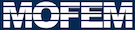 |
| v0.14.0
|
Operator the left hand side matrix.
More...
Operator the left hand side matrix.
- Examples
- plate.cpp.
Definition at line 121 of file plate.cpp.
◆ OpH1LhsSkeleton()
OpH1LhsSkeleton::OpH1LhsSkeleton |
( |
boost::shared_ptr< FaceSideEle > |
side_fe_ptr, |
|
|
boost::shared_ptr< MatrixDouble > |
mat_d_ptr |
|
) |
| |
◆ doWork()
- Examples
- plate.cpp.
Definition at line 511 of file plate.cpp.
517 const auto in_the_loop =
525 auto t_normal = getFTensor1Normal();
526 t_normal.normalize();
530 auto t_D = getFTensor4DdgFromMat<SPACE_DIM, SPACE_DIM, 0>(*
dMatPtr);
533 const size_t nb_integration_pts = getGaussPts().size2();
537 const double beta =
static_cast<double>(
nitsche) / (in_the_loop + 1);
539 auto integrate = [&](
auto sense_row,
auto &row_ind,
auto &row_diff,
540 auto &row_diff2,
auto sense_col,
auto &col_ind,
541 auto &col_diff,
auto &col_diff2) {
546 const auto nb_rows = row_ind.size();
547 const auto nb_cols = col_ind.size();
549 const auto nb_row_base_functions = row_diff.size2() /
SPACE_DIM;
554 locMat.resize(nb_rows, nb_cols,
false);
561 auto t_w = getFTensor0IntegrationWeight();
564 for (
size_t gg = 0; gg != nb_integration_pts; ++gg) {
568 const double alpha = getMeasure() * t_w;
569 auto t_mat =
locMat.data().begin();
573 for (; rr != nb_rows; ++rr) {
576 t_mv(
i,
j) = t_D(
i,
j,
k,
l) * t_diff2_row_base(
k,
l);
580 t_vn_plus(
i,
j) = beta * (
phi * t_mv(
i,
j) / p);
582 t_vn(
i,
j) = (t_diff_row_base(
j) * (t_normal(
i) * sense_row)) -
590 for (
size_t cc = 0; cc != nb_cols; ++cc) {
593 t_mu(
i,
j) = t_D(
i,
j,
k,
l) * t_diff2_col_base(
k,
l);
597 t_un(
i,
j) = -p * ((t_diff_col_base(
j) * (t_normal(
i) * sense_col) -
598 beta * t_mu(
i,
j) / p));
601 *t_mat -= alpha * (t_vn(
i,
j) * t_un(
i,
j));
602 *t_mat -= alpha * (t_vn_plus(
i,
j) * (beta * t_mu(
i,
j)));
620 for (; rr < nb_row_base_functions; ++rr) {
630 col_ind.size(), &*col_ind.begin(),
631 &*
locMat.data().begin(), ADD_VALUES);
640 const auto sense_row =
senseMap[s0];
645 const auto sense_col =
senseMap[s1];
◆ dMatPtr
boost::shared_ptr<MatrixDouble> OpH1LhsSkeleton::dMatPtr |
|
private |
◆ locMat
MatrixDouble OpH1LhsSkeleton::locMat |
|
private |
◆ sideFEPtr
boost::shared_ptr<FaceSideEle> OpH1LhsSkeleton::sideFEPtr |
|
private |
pointer to element to get data on edge/face sides
- Examples
- plate.cpp.
Definition at line 136 of file plate.cpp.
The documentation for this struct was generated from the following file:
#define MoFEMFunctionReturnHot(a)
Last executable line of each PETSc function used for error handling. Replaces return()
std::array< int, 2 > senseMap
MoFEMErrorCode MatSetValues(Mat M, const EntitiesFieldData::EntData &row_data, const EntitiesFieldData::EntData &col_data, const double *ptr, InsertMode iora)
Assemble PETSc matrix.
boost::shared_ptr< FaceSideEle > sideFEPtr
pointer to element to get data on edge/face sides
FTensor::Index< 'k', SPACE_DIM > k
@ OPSPACE
operator do Work is execute on space data
constexpr int SPACE_DIM
dimension of space
FTensor::Index< 'l', SPACE_DIM > l
std::array< std::vector< MatrixDouble >, 2 > diff2BaseSideMap
auto get_diff_ntensor(T &base_mat)
std::array< std::vector< VectorInt >, 2 > indicesSideMap
indices on rows for left hand-side
#define CHKERR
Inline error check.
MatrixDouble locMat
local operator matrix
FTensor::Index< 'i', SPACE_DIM > i
std::array< double, 2 > areaMap
boost::shared_ptr< MatrixDouble > dMatPtr
std::array< std::vector< MatrixDouble >, 2 > diffBaseSideMap
auto get_diff2_ntensor(T &base_mat)
FTensor::Index< 'j', SPACE_DIM > j
#define MoFEMFunctionBeginHot
First executable line of each MoFEM function, used for error handling. Final line of MoFEM functions ...
#define MoFEMFunctionReturn(a)
Last executable line of each PETSc function used for error handling. Replaces return()
BoundaryEle::UserDataOperator BoundaryEleOp
#define MoFEMFunctionBegin
First executable line of each MoFEM function, used for error handling. Final line of MoFEM functions ...