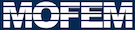 |
| v0.14.0
|
Go to the documentation of this file.
32 std::map<std::string, boost::shared_ptr<Range>>
mapTypeRow;
33 std::map<std::string, boost::shared_ptr<Range>>
mapTypeCol;
54 #endif //__DMCTX_IMPL_H
std::vector< std::string > colSubFields
std::vector< std::string > colCompPrb
std::map< std::string, boost::shared_ptr< Range > > mapTypeRow
PetscBool isPartitioned
true if read mesh is on parts
boost::shared_ptr< NestSchurData > nestedSchurDataPtr
std::string problemName
Problem name.
Deprecated interface functions.
PetscBool isSquareMatrix
true if rows equals to cols
implementation of Data Operators for Forces and Sources
boost::shared_ptr< BlockStructure > blocMatDataPtr
std::vector< std::string > rowSubFields
Interface * mField_ptr
MoFEM interface.
PetscBool destroyProblem
If true destroy problem with DM.
PETSc Discrete Manager data structure.
std::vector< std::string > rowCompPrb
const Problem * problemMainOfSubPtr
pointer to main problem to sub-problem
PetscBool isProblemBuild
True if problem is build.
keeps basic data about problem
const Problem * problemPtr
pointer to problem data structure
std::map< std::string, boost::shared_ptr< Range > > mapTypeCol