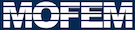 |
| v0.14.0
|
Go to the documentation of this file.
17 boost::shared_ptr<std::vector<unsigned char>> boundary_marker)
20 yesSet(yes_set), boundaryMarker(boundary_marker) {}
45 boost::make_shared<EssentialBcStorage>(indices));
46 e_ptr->getWeakStoragePtr() =
78 const double *ptr, InsertMode iora) {
83 "Pointer to PETSc vector is null");
85 CHKERR VecSetOption(V, VEC_IGNORE_NEGATIVE_INDICES, PETSC_TRUE);
88 if (
auto stored_data_ptr =
91 &*stored_data_ptr->entityIndices.begin(), ptr,
119 "Pointer to PETSc matrix is null");
123 if (
auto stored_data_ptr =
126 &*stored_data_ptr->entityIndices.begin(),
OpSetBc(std::string field_name, bool yes_set, boost::shared_ptr< std::vector< unsigned char >> boundary_marker)
Data on single entity (This is passed as argument to DataOperator::doWork)
const VectorFieldEntities & getFieldEntities() const
get field entities
MoFEMErrorCode MatSetValues(Mat M, const EntitiesFieldData::EntData &row_data, const EntitiesFieldData::EntData &col_data, const double *ptr, InsertMode iora)
Assemble PETSc matrix.
const VectorInt & getLocalIndices() const
get local indices of dofs on entity
PetscErrorCode MoFEMErrorCode
MoFEM/PETSc error code.
map< std::string, std::vector< boost::shared_ptr< EssentialBcStorage > >> HashVectorStorage
Store modifed indices by field.
OpUnSetBc(std::string field_name)
MoFEMErrorCode VecSetValues(Vec V, const EntitiesFieldData::EntData &data, const double *ptr, InsertMode iora)
Assemble PETSc vector.
boost::shared_ptr< std::vector< unsigned char > > boundaryMarker
FTensor::Index< 'M', 3 > M
#define CHKERR
Inline error check.
MoFEMErrorCode doWork(int side, EntityType type, EntitiesFieldData::EntData &data)
Operator for linear form, usually to calculate values on right hand side.
implementation of Data Operators for Forces and Sources
MoFEMErrorCode MatSetValues< EssentialBcStorage >(Mat M, const EntitiesFieldData::EntData &row_data, const EntitiesFieldData::EntData &col_data, const double *ptr, InsertMode iora)
Set values to matrix in operator.
const VectorInt & getIndices() const
Get global indices of dofs on entity.
static HashVectorStorage feStorage
[Storage and set boundary conditions]
constexpr auto field_name
MoFEMErrorCode doWork(int side, EntityType type, EntitiesFieldData::EntData &data)
Operator for linear form, usually to calculate values on right hand side.
structure to get information form mofem into EntitiesFieldData
[Storage and set boundary conditions]
const FTensor::Tensor2< T, Dim, Dim > Vec
#define MoFEMFunctionReturn(a)
Last executable line of each PETSc function used for error handling. Replaces return()
#define MoFEMFunctionBegin
First executable line of each MoFEM function, used for error handling. Final line of MoFEM functions ...