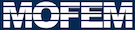 |
| v0.14.0
|
Go to the documentation of this file.
23 struct AddFluxToRhsPipelineImpl<
30 AddFluxToRhsPipelineImpl() =
delete;
33 typename NaturalBC<OpBase>::template Assembly<A>::template LinearForm<I>;
39 boost::ptr_deque<ForcesAndSourcesCore::UserDataOperator> &pipeline,
41 std::vector<boost::shared_ptr<ScalingMethod>> smv,
Sev sev
45 CHKERR T::template AddFluxToPipeline<OpBodyForce>::add(
46 pipeline, m_field,
field_name, smv,
"BODY_FORCE", sev);
53 struct AddFluxToRhsPipelineImpl<
60 AddFluxToRhsPipelineImpl() =
delete;
63 typename NaturalBC<OpBase>::template Assembly<A>::template LinearForm<I>;
73 boost::ptr_deque<ForcesAndSourcesCore::UserDataOperator> &pipeline,
75 std::vector<boost::shared_ptr<ScalingMethod>> smv,
Sev sev
79 CHKERR T::template AddFluxToPipeline<OpForce>::add(
80 pipeline, m_field,
field_name, smv,
"FORCE", sev);
81 auto u_ptr = boost::make_shared<MatrixDouble>();
83 new OpCalculateVectorFieldValues<SPACE_DIM>(
field_name, u_ptr));
84 CHKERR T::template AddFluxToPipeline<OpSpringRhs>::add(
85 pipeline, m_field,
field_name, u_ptr, 1,
"SPRING", sev);
92 struct AddFluxToLhsPipelineImpl<
99 AddFluxToLhsPipelineImpl() =
delete;
101 using T =
typename NaturalBC<OpBase>::template Assembly<
110 boost::ptr_deque<ForcesAndSourcesCore::UserDataOperator> &pipeline,
115 CHKERR T::template AddFluxToPipeline<OpSpringLhs>::add(
Implementation of elastic spring bc.
PetscErrorCode MoFEMErrorCode
MoFEM/PETSc error code.
typename NaturalBC< OpBase >::template Assembly< A >::template LinearForm< I > T
typename T::template OpFlux< ElasticExample::SpringBcType< BLOCKSET >, 1, SPACE_DIM > OpSpringRhs
static MoFEMErrorCode add(boost::ptr_deque< ForcesAndSourcesCore::UserDataOperator > &pipeline, MoFEM::Interface &m_field, std::string field_name, Sev sev)
Deprecated interface functions.
constexpr IntegrationType I
MoFEM::LogManager::SeverityLevel Sev
#define CHKERR
Inline error check.
static MoFEMErrorCode add(boost::ptr_deque< ForcesAndSourcesCore::UserDataOperator > &pipeline, MoFEM::Interface &m_field, std::string field_name, std::vector< boost::shared_ptr< ScalingMethod >> smv, Sev sev)
typename NaturalBC< OpBase >::template Assembly< A >::template BiLinearForm< I > T
constexpr auto field_name
typename NaturalBC< OpBase >::template Assembly< A >::template LinearForm< I > T
typename T::template OpFlux< ElasticExample::SpringBcType< BLOCKSET >, BASE_DIM, FIELD_DIM > OpSpringLhs
static MoFEMErrorCode add(boost::ptr_deque< ForcesAndSourcesCore::UserDataOperator > &pipeline, MoFEM::Interface &m_field, std::string field_name, std::vector< boost::shared_ptr< ScalingMethod >> smv, Sev sev)
#define MoFEMFunctionReturn(a)
Last executable line of each PETSc function used for error handling. Replaces return()
typename T::template OpFlux< NaturalForceMeshsets, 1, SPACE_DIM > OpForce
#define MoFEMFunctionBegin
First executable line of each MoFEM function, used for error handling. Final line of MoFEM functions ...
typename T::template OpFlux< NaturalMeshsetType< BLOCKSET >, BASE_DIM, FIELD_DIM > OpBodyForce