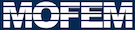 |
| v0.14.0
|
#include <src/finite_elements/FormsIntegrators.hpp>
|
| OpBaseImpl (const std::string row_field_name, const std::string col_field_name, const OpType type, boost::shared_ptr< Range > ents_ptr=nullptr) |
|
MoFEMErrorCode | doWork (int row_side, int col_side, EntityType row_type, EntityType col_type, EntData &row_data, EntData &col_data) |
| Do calculations for the left hand side. More...
|
|
MoFEMErrorCode | doWork (int row_side, EntityType row_type, EntData &row_data) |
| Do calculations for the right hand side. More...
|
|
template<AssemblyType A, typename EleOp>
struct MoFEM::OpBaseImpl< A, EleOp >
- Examples
- ADOLCPlasticity.hpp, approx_sphere.cpp, EshelbianPlasticity.cpp, free_surface.cpp, level_set.cpp, phase.cpp, PlasticOps.hpp, PlasticOpsGeneric.hpp, PlasticOpsLargeStrains.hpp, PlasticOpsSmallStrains.hpp, poisson_2d_homogeneous.hpp, schur_test_diag_mat.cpp, seepage.cpp, SeepageOps.hpp, shallow_wave.cpp, tensor_divergence_operator.cpp, thermo_elastic.cpp, and ThermoElasticOps.hpp.
Definition at line 178 of file FormsIntegrators.hpp.
◆ EntData
template<AssemblyType A, typename EleOp >
◆ MatSetValuesHook
template<AssemblyType A, typename EleOp >
◆ OpType
template<AssemblyType A, typename EleOp >
◆ OpBaseImpl()
template<AssemblyType A, typename EleOp >
◆ aSsemble() [1/2]
template<AssemblyType A, typename EleOp >
Definition at line 490 of file FormsIntegrators.hpp.
496 return VecSetValues<AssemblyTypeSelector<A>>(this->getKSPf(), data,
497 this->
locF, ADD_VALUES);
◆ aSsemble() [2/2]
template<AssemblyType A, typename EleOp >
◆ doWork() [1/2]
template<AssemblyType A, typename EleOp >
Do calculations for the right hand side.
- Parameters
-
row_side | |
row_type | |
row_data | |
- Returns
- MoFEMErrorCode
Definition at line 432 of file FormsIntegrators.hpp.
◆ doWork() [2/2]
template<AssemblyType A, typename EleOp >
Do calculations for the left hand side.
- Parameters
-
row_side | row side number (local number) of entity on element |
col_side | column side number (local number) of entity on element |
row_type | type of row entity |
col_type | type of column entity |
row_data | data for row |
col_data | data for column |
- Returns
- error code
Definition at line 377 of file FormsIntegrators.hpp.
389 nbRows = row_data.getIndices().size();
396 nbCols = col_data.getIndices().size();
415 auto check_if_assemble_transpose = [&] {
417 if (row_side != col_side || row_type != col_type)
427 CHKERR aSsemble(row_data, col_data, check_if_assemble_transpose());
◆ getLocMat()
template<AssemblyType A, typename EleOp >
template<int DIM>
◆ getNbOfBaseFunctions()
template<AssemblyType A, typename EleOp >
Get number of base functions.
- Parameters
-
- Returns
- number of base functions
Definition at line 345 of file FormsIntegrators.hpp.
346 auto nb_base_functions = data.getN().size2();
348 switch (data.getSpace()) {
357 nb_base_functions /= 3;
359 if (data.getN().size2() % 3) {
361 "Number of base functions is not divisible by 3");
372 return nb_base_functions;
◆ getNf()
template<AssemblyType A, typename EleOp >
template<int DIM>
◆ iNtegrate() [1/2]
template<AssemblyType A, typename EleOp >
Class dedicated to integrate operator.
- Parameters
-
data | entity data on element row |
- Returns
- error code
Reimplemented in MoFEM::OpBrokenSpaceConstrainDHybridImpl< FIELD_DIM, GAUSS, OpBase >, MoFEM::OpBrokenSpaceConstrainDFluxImpl< FIELD_DIM, GAUSS, OpBase >, OpURhs, OpRhs, MoFEM::OpConvectiveTermRhsImpl< 1, FIELD_DIM, SPACE_DIM, GAUSS, OpBase >, MoFEM::OpConvectiveTermRhsImpl< 1, 1, SPACE_DIM, GAUSS, OpBase >, MoFEM::OpNormalMixVecTimesVectorFieldImpl< SPACE_DIM, GAUSS, OpBase >, MoFEM::OpNormalMixVecTimesScalarImpl< 2, GAUSS, OpBase >, MoFEM::OpNormalMixVecTimesScalarImpl< 3, GAUSS, OpBase >, MoFEM::OpMixTensorTimesGradUImpl< SPACE_DIM, GAUSS, OpBase >, MoFEM::OpMixVecTimesDivLambdaImpl< SPACE_DIM, GAUSS, OpBase >, MoFEM::OpMixDivTimesUImpl< 1, FIELD_DIM, FIELD_DIM, GAUSS, OpBase, CoordSys >, MoFEM::OpMixDivTimesUImpl< 3, 1, SPACE_DIM, GAUSS, OpBase, CoordSys >, MoFEM::OpMixDivTimesUImpl< 3, FIELD_DIM, SPACE_DIM, GAUSS, OpBase, CoordSys >, MoFEM::OpGradTimesSymTensorImpl< 1, SPACE_DIM, SPACE_DIM, S, GAUSS, OpBase >, MoFEM::OpGradTimesTensorImpl< 1, SPACE_DIM, SPACE_DIM, S, GAUSS, OpBase >, MoFEM::OpGradTimesTensorImpl< 1, 1, SPACE_DIM, S, GAUSS, OpBase >, MoFEM::OpBaseTimesVectorImpl< 3, FIELD_DIM, S, GAUSS, OpBase >, MoFEM::OpBaseTimesVectorImpl< 1, FIELD_DIM, S, GAUSS, OpBase >, MoFEM::OpBaseTimesScalarImpl< 1, S, GAUSS, OpBase >, MoFEM::OpSourceImpl< 3, FIELD_DIM, GAUSS, SourceFunctionSpecialization::S< OpBase > >, MoFEM::OpSourceImpl< 1, FIELD_DIM, GAUSS, SourceFunctionSpecialization::S< OpBase > >, MoFEM::OpSourceImpl< 1, 1, GAUSS, SourceFunctionSpecialization::S< OpBase > >, Example::OpFluxRhs, Example::OpRadiationRhs, LevelSet::OpRhsDomain, and OpDomainResidualVector.
Definition at line 271 of file FormsIntegrators.hpp.
◆ iNtegrate() [2/2]
template<AssemblyType A, typename EleOp >
Integrate grad-grad operator.
- Parameters
-
row_data | row data (consist base functions on row entity) |
col_data | column data (consist base functions on column entity) |
- Returns
- error code
Reimplemented in MoFEM::OpMixTensorTimesGradImpl< SPACE_DIM, GAUSS, OpBase >, MoFEM::OpMixVectorTimesGradImpl< 1, SPACE_DIM, SPACE_DIM, GAUSS, OpBase >, MoFEM::OpMixVectorTimesGradImpl< 3, SPACE_DIM, SPACE_DIM, GAUSS, OpBase >, MoFEM::OpMixScalarTimesDivImpl< SPACE_DIM, GAUSS, OpBase, COORDINATE_SYSTEM >, MoFEM::OpMixDivTimesVecImpl< SPACE_DIM, GAUSS, OpBase, CoordSys >, OpULhs_dH, MoFEM::OpMixDivTimesScalarImpl< SPACE_DIM, GAUSS, OpBase >, MoFEM::OpGradGradSymTensorGradGradImpl< 1, 1, SPACE_DIM, S, GAUSS, OpBase >, MoFEM::OpGradTensorGradImpl< 1, SPACE_DIM, SPACE_DIM, S, GAUSS, OpBase >, MoFEM::OpBrokenSpaceConstrainImpl< FIELD_DIM, GAUSS, OpBase >, MoFEM::OpGradSymTensorGradImpl< 1, SPACE_DIM, SPACE_DIM, S, GAUSS, OpBase >, OpULhs_dU, MoFEM::OpMassImpl< 3, 9, GAUSS, OpBase >, OpLhs, MoFEM::OpMassImpl< 3, 4, GAUSS, OpBase >, MoFEM::OpMassImpl< 3, FIELD_DIM, GAUSS, OpBase >, MoFEM::OpConvectiveTermLhsDyImpl< 1, FIELD_DIM, SPACE_DIM, GAUSS, OpBase >, MoFEM::OpMassImpl< 1, FIELD_DIM, GAUSS, OpBase >, MoFEM::OpMassImpl< 1, 1, GAUSS, OpBase >, MoFEM::OpConvectiveTermLhsDuImpl< 1, FIELD_DIM, SPACE_DIM, GAUSS, OpBase >, MoFEM::OpGradGradImpl< 1, FIELD_DIM, SPACE_DIM, GAUSS, OpBase >, MoFEM::OpConvectiveTermLhsDyImpl< 1, 1, SPACE_DIM, GAUSS, OpBase >, MoFEM::OpGradGradImpl< 1, 1, SPACE_DIM, GAUSS, OpBase >, MoFEM::OpConvectiveTermLhsDuImpl< 1, 1, SPACE_DIM, GAUSS, OpBase >, LevelSet::OpLhsDomain, Example::OpRadiationLhs, and OpDomainTangentMatrix.
Definition at line 259 of file FormsIntegrators.hpp.
◆ assembleTranspose
template<AssemblyType A, typename EleOp >
◆ colSide
template<AssemblyType A, typename EleOp >
◆ colType
template<AssemblyType A, typename EleOp >
◆ entsPtr
template<AssemblyType A, typename EleOp >
◆ feScalingFun
template<AssemblyType A, typename EleOp >
◆ locF
template<AssemblyType A, typename EleOp >
◆ locMat
template<AssemblyType A, typename EleOp >
◆ locMatTranspose
template<AssemblyType A, typename EleOp >
◆ matSetValuesHook
template<AssemblyType A, typename EleOp >
Initial value:=
return MatSetValues<AssemblyTypeSelector<A>>(
op_ptr->getKSPB(), row_data, col_data,
m, ADD_VALUES);
}
Definition at line 220 of file FormsIntegrators.hpp.
◆ nbCols
template<AssemblyType A, typename EleOp >
◆ nbIntegrationPts
template<AssemblyType A, typename EleOp >
◆ nbRowBaseFunctions
template<AssemblyType A, typename EleOp >
◆ nbRows
template<AssemblyType A, typename EleOp >
◆ onlyTranspose
template<AssemblyType A, typename EleOp >
◆ rowSide
template<AssemblyType A, typename EleOp >
◆ rowType
template<AssemblyType A, typename EleOp >
◆ timeScalingFun
template<AssemblyType A, typename EleOp >
The documentation for this struct was generated from the following file:
FEFun feScalingFun
assumes that time variable is set
#define MoFEMFunctionReturnHot(a)
Last executable line of each PETSc function used for error handling. Replaces return()
int nbIntegrationPts
number of integration points
MatrixDouble locMat
local entity block matrix
@ L2
field with C-1 continuity
UBlasMatrix< double > MatrixDouble
@ USER_BASE
user implemented approximation base
VectorDouble locF
local entity vector
#define CHKERR
Inline error check.
TimeFun timeScalingFun
assumes that time variable is set
virtual MoFEMErrorCode iNtegrate(EntData &row_data, EntData &col_data)
Integrate grad-grad operator.
const VectorInt & getIndices() const
Get global indices of dofs on entity.
int colSide
column side number
MatrixDouble locMatTranspose
local entity block matrix
friend class UserDataOperator
EntitiesFieldData::EntData EntData
int nbRowBaseFunctions
number or row base functions
int rowSide
row side number
int nbCols
number if dof on column
const static char *const FieldSpaceNames[]
int nbRows
number of dofs on rows
boost::shared_ptr< Range > entsPtr
Entities on which element is run.
static MatSetValuesHook matSetValuesHook
@ HCURL
field with continuous tangents
@ MOFEM_DATA_INCONSISTENCY
virtual MoFEMErrorCode aSsemble(EntData &row_data, EntData &col_data, const bool trans)
FTensor::Index< 'm', 3 > m
virtual size_t getNbOfBaseFunctions(EntitiesFieldData::EntData &data)
Get number of base functions.
EntityType rowType
row type
#define MoFEMFunctionReturn(a)
Last executable line of each PETSc function used for error handling. Replaces return()
@ HDIV
field with continuous normal traction
#define MoFEMFunctionBegin
First executable line of each MoFEM function, used for error handling. Final line of MoFEM functions ...
EntityType colType
column type
@ NOFIELD
scalar or vector of scalars describe (no true field)