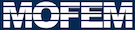 |
| v0.14.0
|
|
| OpULhs_dU (const std::string field_name_row, const std::string field_name_col, boost::shared_ptr< MatrixDouble > u_ptr, boost::shared_ptr< MatrixDouble > grad_u_ptr) |
|
MoFEMErrorCode | iNtegrate (EntitiesFieldData::EntData &row_data, EntitiesFieldData::EntData &col_data) |
| Integrate grad-grad operator. More...
|
|
| OpBaseImpl (const std::string row_field_name, const std::string col_field_name, const OpType type, boost::shared_ptr< Range > ents_ptr=nullptr) |
|
MoFEMErrorCode | doWork (int row_side, int col_side, EntityType row_type, EntityType col_type, EntData &row_data, EntData &col_data) |
| Do calculations for the left hand side. More...
|
|
MoFEMErrorCode | doWork (int row_side, EntityType row_type, EntData &row_data) |
| Do calculations for the right hand side. More...
|
|
|
boost::shared_ptr< MatrixDouble > | uPtr |
|
boost::shared_ptr< MatrixDouble > | uGradPtr |
|
|
using | OpType = typename EleOp::OpType |
|
using | EntData = EntitiesFieldData::EntData |
|
using | MatSetValuesHook = boost::function< MoFEMErrorCode(ForcesAndSourcesCore::UserDataOperator *op_ptr, const EntitiesFieldData::EntData &row_data, const EntitiesFieldData::EntData &col_data, MatrixDouble &m)> |
|
TimeFun | timeScalingFun |
| assumes that time variable is set More...
|
|
FEFun | feScalingFun |
| assumes that time variable is set More...
|
|
boost::shared_ptr< Range > | entsPtr |
| Entities on which element is run. More...
|
|
static MatSetValuesHook | matSetValuesHook |
|
template<int DIM> |
FTensor::Tensor1< FTensor::PackPtr< double *, DIM >, DIM > | getNf () |
|
template<int DIM> |
FTensor::Tensor2< FTensor::PackPtr< double *, DIM >, DIM, DIM > | getLocMat (const int rr) |
|
virtual MoFEMErrorCode | aSsemble (EntData &row_data, EntData &col_data, const bool trans) |
|
virtual MoFEMErrorCode | iNtegrate (EntData &data) |
| Class dedicated to integrate operator. More...
|
|
virtual MoFEMErrorCode | aSsemble (EntData &data) |
|
virtual size_t | getNbOfBaseFunctions (EntitiesFieldData::EntData &data) |
| Get number of base functions. More...
|
|
int | nbRows |
| number of dofs on rows More...
|
|
int | nbCols |
| number if dof on column More...
|
|
int | nbIntegrationPts |
| number of integration points More...
|
|
int | nbRowBaseFunctions |
| number or row base functions More...
|
|
int | rowSide |
| row side number More...
|
|
int | colSide |
| column side number More...
|
|
EntityType | rowType |
| row type More...
|
|
EntityType | colType |
| column type More...
|
|
bool | assembleTranspose |
|
bool | onlyTranspose |
|
MatrixDouble | locMat |
| local entity block matrix More...
|
|
MatrixDouble | locMatTranspose |
| local entity block matrix More...
|
|
VectorDouble | locF |
| local entity vector More...
|
|
- Examples
- shallow_wave.cpp.
Definition at line 168 of file shallow_wave.cpp.
◆ OpULhs_dU()
OpULhs_dU::OpULhs_dU |
( |
const std::string |
field_name_row, |
|
|
const std::string |
field_name_col, |
|
|
boost::shared_ptr< MatrixDouble > |
u_ptr, |
|
|
boost::shared_ptr< MatrixDouble > |
grad_u_ptr |
|
) |
| |
|
inline |
◆ iNtegrate()
Integrate grad-grad operator.
- Parameters
-
row_data | row data (consist base functions on row entity) |
col_data | column data (consist base functions on column entity) |
- Returns
- error code
Reimplemented from MoFEM::OpBaseImpl< A, EleOp >.
Definition at line 179 of file shallow_wave.cpp.
183 const double vol = getMeasure();
184 auto t_w = getFTensor0IntegrationWeight();
187 auto t_coords = getFTensor1CoordsAtGaussPts();
188 auto t_normal = getFTensor1NormalsAtGaussPts();
190 auto t_u = getFTensor1FromMat<3>(*
uPtr);
191 auto t_grad_u = getFTensor2FromMat<3, 3>(*
uGradPtr);
193 auto get_t_mat = [&](
const int rr) {
202 const auto ts_a = getFEMethod()->ts_a;
206 const auto a = std::sqrt(t_coords(
i) * t_coords(
i));
207 const auto sin_fi = t_coords(2) /
a;
208 const auto f = 2 *
omega * sin_fi;
211 t_r(
i) = t_normal(
i);
216 t_P(
i,
j) = t_r(
i) * t_r(
j);
224 t_Q(
m,
i) * (ts_a *
t_kd(
i,
j) + t_grad_u(
i,
j) +
f * t_A(
i,
j)) +
227 const double alpha = t_w * vol;
230 for (; rr !=
nbRows / 3; rr++) {
234 auto t_mat = get_t_mat(3 * rr);
236 for (
int cc = 0; cc !=
nbCols / 3; cc++) {
237 t_mat(
i,
j) += (alpha * t_row_base * t_col_base) * t_rhs_du(
i,
j);
238 t_mat(
i,
j) += (alpha *
mu) * t_Q(
i,
j) *
239 (t_row_diff_base(
m) * t_col_diff_base(
m));
◆ uGradPtr
boost::shared_ptr<MatrixDouble> OpULhs_dU::uGradPtr |
|
private |
◆ uPtr
boost::shared_ptr<MatrixDouble> OpULhs_dU::uPtr |
|
private |
The documentation for this struct was generated from the following file:
int nbIntegrationPts
number of integration points
MatrixDouble locMat
local entity block matrix
FTensor::Index< 'i', 3 > i
constexpr std::enable_if<(Dim0<=2 &&Dim1<=2), Tensor2_Expr< Levi_Civita< T >, T, Dim0, Dim1, i, j > >::type levi_civita(const Index< i, Dim0 > &, const Index< j, Dim1 > &)
levi_civita functions to make for easy adhoc use
FTensor::Tensor0< FTensor::PackPtr< double *, 1 > > getFTensor0N(const FieldApproximationBase base)
Get base function as Tensor0.
FTensor::Index< 'j', 3 > j
Tensor1< T, Tensor_Dim > normalize()
int nbRowBaseFunctions
number or row base functions
int nbCols
number if dof on column
FTensor::Tensor1< FTensor::PackPtr< double *, Tensor_Dim >, Tensor_Dim > getFTensor1DiffN(const FieldApproximationBase base)
Get derivatives of base functions.
int nbRows
number of dofs on rows
boost::shared_ptr< MatrixDouble > uGradPtr
FormsIntegrators< DomainEleOp >::Assembly< PETSC >::OpBase AssemblyDomainEleOp
FTensor::Index< 'm', 3 > m
#define MoFEMFunctionReturn(a)
Last executable line of each PETSc function used for error handling. Replaces return()
boost::shared_ptr< MatrixDouble > uPtr
#define MoFEMFunctionBegin
First executable line of each MoFEM function, used for error handling. Final line of MoFEM functions ...