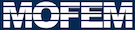 |
| v0.14.0
|
Go to the documentation of this file.
9 #ifndef __CONVECTIVE_MASS_ELEMENT_HPP
10 #define __CONVECTIVE_MASS_ELEMENT_HPP
13 #error "MoFEM need to be compiled with ADOL-C"
31 struct MyVolumeFE :
public VolumeElementForcesAndSourcesCore {
94 auto add_ops = [&](
auto &fe) {
121 std::map<int, BlockData>
144 std::vector<VectorDouble>
valT;
146 std::vector<MatrixDouble>
jacT;
160 std::vector<VectorDouble> &values_at_gauss_pts,
161 std::vector<MatrixDouble> &gardient_at_gauss_pts);
192 boost::ptr_vector<MethodForForceScaling> &methods_op,
193 int tag,
bool linear =
false);
232 Range *forcesonlyonentities_ptr = NULL);
266 SmartPetscObj<Vec>
V;
289 CommonData &common_data,
int tag,
bool jacobian =
true);
360 bool jacobian =
true);
362 VectorBoundedArray<adouble, 3>
a,
v,
a_T;
381 Range *forcesonlyonentities_ptr);
395 Range *forcesonlyonentities_ptr);
408 Range *forcesonlyonentities_ptr);
421 Range *forcesonlyonentities_ptr);
443 const std::string velocity_field,
444 const std::string spatial_position_field);
461 boost::shared_ptr<map<int, BlockData>> &block_sets_ptr);
464 string element_name,
string velocity_field_name,
465 string spatial_position_field_name,
466 string material_position_field_name =
"MESH_NODE_POSITIONS",
470 string element_name,
string velocity_field_name,
471 string spatial_position_field_name,
472 string material_position_field_name =
"MESH_NODE_POSITIONS",
476 string element_name,
string velocity_field_name,
477 string spatial_position_field_name,
478 string material_position_field_name =
"MESH_NODE_POSITIONS",
480 Range *intersected = NULL);
483 string velocity_field_name,
string spatial_position_field_name,
484 string material_position_field_name =
"MESH_NODE_POSITIONS",
485 bool ale =
false,
bool linear =
false);
488 string velocity_field_name,
string spatial_position_field_name,
489 string material_position_field_name =
"MESH_NODE_POSITIONS",
493 string velocity_field_name,
string spatial_position_field_name,
494 string material_position_field_name =
"MESH_NODE_POSITIONS",
495 Range *forces_on_entities_ptr = NULL);
498 string velocity_field_name,
string spatial_position_field_name,
499 string material_position_field_name =
"MESH_NODE_POSITIONS",
500 bool linear =
false);
550 CHKERR MatShellGetContext(
A, &void_ctx);
593 CHKERR MatShellGetContext(
A, &void_ctx);
622 CHKERR PCShellGetContext(pc, &void_ctx);
637 CHKERR PCShellGetContext(pc, &void_ctx);
675 CHKERR PCShellGetContext(pc, &void_ctx);
681 INSERT_VALUES, SCATTER_FORWARD);
683 INSERT_VALUES, SCATTER_FORWARD);
685 INSERT_VALUES, SCATTER_FORWARD);
687 INSERT_VALUES, SCATTER_FORWARD);
690 CHKERR MatMult(shell_mat_ctx->
M, shell_mat_ctx->
v,
692 CHKERR VecAXPY(shell_mat_ctx->
Ku, -1, shell_mat_ctx->
Mv);
697 CHKERR VecAXPY(shell_mat_ctx->
v, shell_mat_ctx->
ts_a,
703 INSERT_VALUES, SCATTER_REVERSE);
705 INSERT_VALUES, SCATTER_REVERSE);
707 INSERT_VALUES, SCATTER_REVERSE);
709 INSERT_VALUES, SCATTER_REVERSE);
710 CHKERR VecAssemblyBegin(x);
732 #ifdef __DIRICHLET_HPP__
740 struct ShellMatrixElement :
public FEMethod {
745 typedef std::pair<std::string, FEMethod *> PairNameFEMethodPtr;
746 typedef std::vector<PairNameFEMethodPtr> LoopsToDoType;
749 LoopsToDoType loopAuxM;
760 #endif //__DIRICHLET_HPP__
763 #endif //__CONVECTIVE_MASS_ELEMENT_HPP
#define MoFEMFunctionReturnHot(a)
Last executable line of each PETSc function used for error handling. Replaces return()
MoFEMErrorCode addEshelbyDynamicMaterialMomentum(string element_name, string velocity_field_name, string spatial_position_field_name, string material_position_field_name="MESH_NODE_POSITIONS", bool ale=false, BitRefLevel bit=BitRefLevel(), Range *intersected=NULL)
MoFEMErrorCode doWork(int row_side, EntityType row_type, EntitiesFieldData::EntData &row_data)
MoFEMErrorCode doWork(int row_side, EntityType row_type, EntitiesFieldData::EntData &row_data)
VectorBoundedArray< double, 3 > VectorDouble3
PCShellCtx(Mat shell_mat)
VectorBoundedArray< adouble, 3 > v
MoFEMErrorCode setShellMatrixMassOperators(string velocity_field_name, string spatial_position_field_name, string material_position_field_name="MESH_NODE_POSITIONS", bool linear=false)
OpMassRhs(const std::string field_name, BlockData &data, CommonData &common_data)
Range forcesOnlyOnEntities
MoFEMErrorCode addHOOpsVol(const std::string field, E &e, bool h1, bool hcurl, bool hdiv, bool l2)
MyVolumeFE feVelLhs
calculate left hand side for tetrahedral elements
MoFEMErrorCode setVelocityOperators(string velocity_field_name, string spatial_position_field_name, string material_position_field_name="MESH_NODE_POSITIONS", bool ale=false)
VectorBoundedArray< adouble, 3 > dot_W
friend MoFEMErrorCode MultOpA(Mat A, Vec x, Vec f)
OpMassLhs_dM_dx(const std::string field_name, const std::string col_field, BlockData &data, CommonData &common_data)
std::vector< double > active
friend MoFEMErrorCode ZeroEntriesOp(Mat A)
VectorBoundedArray< adouble, 3 > a_res
PetscErrorCode MoFEMErrorCode
MoFEM/PETSc error code.
UBlasMatrix< double > MatrixDouble
std::vector< std::vector< double * > > jacVelRowPtr
std::vector< MatrixDouble > jacT
OpVelocityLhs_dV_dx(const std::string vel_field, const std::string field_name, BlockData &data, CommonData &common_data)
FTensor::Index< 'j', 3 > j
Range tEts
elements in block set
MyVolumeFE feEnergy
calculate kinetic energy
VectorBoundedArray< adouble, 3 > dot_W
MoFEMErrorCode setConvectiveMassOperators(string velocity_field_name, string spatial_position_field_name, string material_position_field_name="MESH_NODE_POSITIONS", bool ale=false, bool linear=false)
friend MoFEMErrorCode PCShellApplyOp(PC pc, Vec f, Vec x)
std::map< std::string, std::vector< VectorDouble > > dataAtGaussPts
MoFEM::Interface & mField
VectorBoundedArray< adouble, 3 > dp_dt
std::vector< std::vector< double * > > jacTRowPtr
Set Dirichlet boundary conditions on displacements.
const std::string velocityField
MatrixBoundedArray< adouble, 9 > H
Deprecated interface functions.
virtual MoFEMErrorCode getJac(EntitiesFieldData::EntData &col_data, int gg)
MoFEMErrorCode addVelocityElement(string element_name, string velocity_field_name, string spatial_position_field_name, string material_position_field_name="MESH_NODE_POSITIONS", bool ale=false, BitRefLevel bit=BitRefLevel())
structure grouping operators and data used for calculation of mass (convective) element
virtual MoFEMErrorCode getJac(EntitiesFieldData::EntData &col_data, int gg)
std::vector< std::vector< double * > > jacMassRowPtr
VectorBoundedArray< adouble, 3 > v
std::map< std::string, std::vector< MatrixDouble > > gradAtGaussPts
OpGetDataAtGaussPts(const std::string field_name, std::vector< VectorDouble > &values_at_gauss_pts, std::vector< MatrixDouble > &gardient_at_gauss_pts)
definition of volume element
#define CHKERR
Inline error check.
MoFEMErrorCode addConvectiveMassElement(string element_name, string velocity_field_name, string spatial_position_field_name, string material_position_field_name="MESH_NODE_POSITIONS", bool ale=false, BitRefLevel bit=BitRefLevel())
static MoFEMErrorCode PCShellSetUpOp(PC pc)
OpVelocityRhs(const std::string field_name, BlockData &data, CommonData &common_data)
MatrixBoundedArray< adouble, 9 > H
MatrixBoundedArray< adouble, 9 > G
const std::string spatialPositionField
std::vector< double > active
VectorBoundedArray< adouble, 3 > a
std::map< int, BlockData > setOfBlocks
maps block set id with appropriate BlockData
MoFEMErrorCode doWork(int row_side, EntityType row_type, EntitiesFieldData::EntData &row_data)
FTensor::Index< 'i', 3 > i
MoFEMErrorCode setKinematicEshelbyOperators(string velocity_field_name, string spatial_position_field_name, string material_position_field_name="MESH_NODE_POSITIONS", Range *forces_on_entities_ptr=NULL)
MyVolumeFE feVelRhs
calculate right hand side for tetrahedral elements
MoFEMErrorCode preProcess()
Scatter values from t_u_dt on the fields.
static MoFEMErrorCode PCShellDestroy(PC pc)
virtual MoFEMErrorCode getJac(EntitiesFieldData::EntData &col_data, int gg)
MoFEMErrorCode preProcess()
Calculate inconsistency between approximation of velocities and velocities calculated from displaceme...
MoFEMErrorCode getJac(EntitiesFieldData::EntData &col_data, int gg)
const EntityType zeroAtType
MoFEMErrorCode doWork(int row_side, EntityType row_type, EntitiesFieldData::EntData &row_data)
OpEnergy(const std::string field_name, BlockData &data, CommonData &common_data, SmartPetscObj< Vec > v)
MoFEMErrorCode doWork(int side, EntityType type, EntitiesFieldData::EntData &data)
operator calculating deformation gradient
MatrixBoundedArray< adouble, 9 > invH
MatShellCtx * shellMatCtx
pointer to shell matrix
friend MoFEMErrorCode PCShellDestroy(PC pc)
MatrixBoundedArray< adouble, 9 > H
Range forcesOnlyOnEntities
MatrixBoundedArray< adouble, 9 > g
FTensor::Index< 'k', 3 > k
VectorBoundedArray< adouble, 3 > dot_u
virtual MoFEMErrorCode getJac(EntitiesFieldData::EntData &col_data, int gg)
MoFEMErrorCode doWork(int row_side, int col_side, EntityType row_type, EntityType col_type, EntitiesFieldData::EntData &row_data, EntitiesFieldData::EntData &col_data)
OpVelocityJacobian(const std::string field_name, BlockData &data, CommonData &common_data, int tag, bool jacobian=true)
VectorBoundedArray< adouble, 3 > a
OpMassLhs_dM_dX(const std::string field_name, const std::string col_field, BlockData &data, CommonData &common_data)
std::vector< MatrixDouble > jacMass
data for calculation inertia forces
MatrixBoundedArray< adouble, 9 > F
OpEshelbyDynamicMaterialMomentumJacobian(const std::string field_name, BlockData &data, CommonData &common_data, int tag, bool jacobian=true)
virtual MoFEMErrorCode getJac(EntitiesFieldData::EntData &col_data, int gg)
MatrixBoundedArray< adouble, 9 > h
static MoFEMErrorCode ZeroEntriesOp(Mat A)
boost::ptr_vector< MethodForForceScaling > & methodsOp
static MoFEMErrorCode PCShellApplyOp(PC pc, Vec f, Vec x)
apply pre-conditioner for shell matrix
std::vector< MatrixDouble > jacVel
virtual MoFEMErrorCode getJac(EntitiesFieldData::EntData &col_data, int gg)
OpEshelbyDynamicMaterialMomentumRhs(const std::string field_name, BlockData &data, CommonData &common_data, Range *forcesonlyonentities_ptr)
MoFEMErrorCode getJac(EntitiesFieldData::EntData &col_data, int gg)
OpEshelbyDynamicMaterialMomentumLhs_dx(const std::string vel_field, const std::string field_name, BlockData &data, CommonData &common_data, Range *forcesonlyonentities_ptr)
OpEshelbyDynamicMaterialMomentumLhs_dX(const std::string vel_field, const std::string field_name, BlockData &data, CommonData &common_data, Range *forcesonlyonentities_ptr)
VectorBoundedArray< adouble, 3 > dot_w
OpMassJacobian(const std::string field_name, BlockData &data, CommonData &common_data, boost::ptr_vector< MethodForForceScaling > &methods_op, int tag, bool linear=false)
EntitiesFieldData::EntData EntData
constexpr auto field_name
std::vector< VectorDouble > valT
MatrixBoundedArray< adouble, 9 > g
double rho0
reference density
boost::ptr_vector< MethodForForceScaling > methodsOp
MatrixBoundedArray< adouble, 9 > h
MatrixBoundedArray< adouble, 9 > invH
VectorBoundedArray< adouble, 3 > a_res
std::vector< MatrixDouble > & gradientAtGaussPts
std::vector< VectorDouble > & valuesAtGaussPts
int getRule(int order)
it is used to calculate nb. of Gauss integration points
MoFEMErrorCode postProcess()
MatrixBoundedArray< adouble, 9 > h
friend MoFEMErrorCode PCShellSetUpOp(PC pc)
MoFEMErrorCode doWork(int row_side, EntityType row_type, EntitiesFieldData::EntData &row_data)
MoFEMErrorCode setBlocks()
OpVelocityLhs_dV_dX(const std::string vel_field, const std::string field_name, BlockData &data, CommonData &common_data)
VectorBoundedArray< adouble, 3 > a_T
ShellResidualElement(MoFEM::Interface &m_field)
common data used by volume elements
MoFEMErrorCode addHOOpsVol()
ForcesAndSourcesCore::UserDataOperator UserDataOperator
MatrixBoundedArray< adouble, 9 > F
OpEshelbyDynamicMaterialMomentumLhs_dv(const std::string vel_field, const std::string field_name, BlockData &data, CommonData &common_data, Range *forcesonlyonentities_ptr)
virtual MoFEMErrorCode getJac(EntitiesFieldData::EntData &col_data, int gg)
static MoFEMErrorCode MultOpA(Mat A, Vec x, Vec f)
Mult operator for shell matrix.
OpMassLhs_dM_dv(const std::string vel_field, const std::string field_name, BlockData &data, CommonData &common_data, Range *forcesonlyonentities_ptr=NULL)
MatrixBoundedArray< adouble, 9 > G
MoFEMErrorCode doWork(int row_side, EntityType row_type, EntitiesFieldData::EntData &row_data)
OpGetCommonDataAtGaussPts(const std::string field_name, CommonData &common_data)
const FTensor::Tensor2< T, Dim, Dim > Vec
MatrixBoundedArray< adouble, 9 > F
std::vector< VectorDouble > valMass
MoFEMErrorCode doWork(int row_side, EntityType row_type, EntitiesFieldData::EntData &row_data)
MatrixBoundedArray< double, 9 > MatrixDouble3by3
UBlasVector< double > VectorDouble
bool initPC
check if PC is initialized
MyVolumeFE feTLhs
calculate left hand side for tetrahedral elements
std::bitset< BITREFLEVEL_SIZE > BitRefLevel
Bit structure attached to each entity identifying to what mesh entity is attached.
#define MoFEMFunctionBeginHot
First executable line of each MoFEM function, used for error handling. Final line of MoFEM functions ...
MoFEMErrorCode postProcess()
MatrixBoundedArray< adouble, 9 > invH
OpVelocityLhs_dV_dv(const std::string vel_field, const std::string field_name, BlockData &data, CommonData &common_data)
MyVolumeFE feMassRhs
calculate right hand side for tetrahedral elements
ConvectiveMassElement(MoFEM::Interface &m_field, short int tag)
MoFEMErrorCode preProcess()
MyVolumeFE(MoFEM::Interface &m_field)
MoFEM::Interface & mField
MyVolumeFE feTRhs
calculate right hand side for tetrahedral elements
#define MoFEMFunctionReturn(a)
Last executable line of each PETSc function used for error handling. Replaces return()
#define MoFEMFunctionBegin
First executable line of each MoFEM function, used for error handling. Final line of MoFEM functions ...
MoFEM::Interface & mField
VectorDouble a0
constant acceleration
std::vector< VectorDouble > valVel
UpdateAndControl(MoFEM::Interface &m_field, TS _ts, const std::string velocity_field, const std::string spatial_position_field)