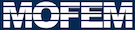 |
| v0.14.0
|
Go to the documentation of this file.
23 #ifndef __DIRICHLET_HPP__
24 #define __DIRICHLET_HPP__
26 using namespace boost::numeric;
44 : scaled_values(3), initial_values(3), bc_flags(3), is_rotation(false) {}
65 string blockset_name =
"DISPLACEMENT");
68 string blockset_name =
"DISPLACEMENT");
91 MoFEMErrorCode getBcDataFromSetsAndBlocks(std::vector<DataFromBc> &bc_data);
101 MoFEMErrorCode getRotationBcFromBlock(std::vector<DataFromBc> &bc_data);
121 : dirichletBcPtr(dirichlet_bc_ptr), dataFromDirichletBc(data_from_dirichlet_bc) {}
127 auto &mField = dirichletBcPtr->
mField;
128 auto &bc_it = dataFromDirichletBc;
131 int coeff = fieldPtr->getNbOfCoeffs();
133 for (
int i = 0;
i != coeff;
i++) {
134 if (bc_it.bc_flags[
i]) {
135 if (entPtr->getEntType() == MBVERTEX) {
136 entPtr->getEntFieldData()[
i] = bc_it.scaled_values[
i];
137 }
else if (!entPtr->getEntFieldData().empty()) {
138 entPtr->getEntFieldData()[
i] = 0;
154 string material_positions)
155 : dirichletBcPtr(dirichlet_bc_ptr),
156 dataFromDirichletBc(data_from_dirichlet_bc),
157 materialPositions(material_positions) {}
165 auto &mField = dirichletBcPtr->
mField;
166 auto &bc_it = dataFromDirichletBc;
170 CHKERR mField.get_field_ents(&field_ents);
171 auto &field_ents_by_uid = field_ents->get<Unique_mi_tag>();
173 auto get_coords = [&]() {
175 if (entPtr->getEntType() == MBVERTEX) {
177 field_ents_by_uid.find(FieldEntity::getLocalUniqueIdCalculate(
178 mField.get_field_bit_number(materialPositions), ent));
179 if (eit != field_ents_by_uid.end())
180 noalias(coords) = (*eit)->getEntFieldData();
182 CHKERR mField.get_moab().get_coords(&ent, 1, &*coords.data().begin());
187 int coeff = fieldPtr->getNbOfCoeffs();
188 auto coords = get_coords();
191 for (
int i = 0;
i != coeff;
i++) {
192 if (bc_it.bc_flags[
i]) {
193 if (entPtr->getEntType() == MBVERTEX) {
194 entPtr->getEntFieldData()[
i] = coords(
i) + bc_it.scaled_values[
i];
195 }
else if (!entPtr->getEntFieldData().empty()) {
196 entPtr->getEntFieldData()[
i] = 0;
215 Vec f,
const std::string material_positions =
"MESH_NODE_POSITIONS",
216 const std::string blockset_name =
"DISPLACEMENT")
218 materialPositions(material_positions) {}
222 const std::string material_positions =
"MESH_NODE_POSITIONS",
223 const std::string blockset_name =
"DISPLACEMENT")
225 materialPositions(material_positions) {}
266 fieldNames.push_back(fieldName);
272 fieldNames.push_back(fieldName);
291 const std::string &problem_name,
292 string blockset_name =
"DISPLACEMENT",
293 bool is_partitioned =
false)
295 problemName(problem_name), isPartitioned(is_partitioned) {}
300 return boost::make_shared<BcEntMethodDisp>(
this, data);
317 const std::string &problem_name,
318 const std::string material_positions =
"MESH_NODE_POSITIONS",
319 string blockset_name =
"DISPLACEMENT",
bool is_partitioned =
false)
322 materialPositions(material_positions) {}
325 return boost::make_shared<BcEntMethodSpatial>(
this, data,
360 const std::string &blockset_name, Mat aij,
367 const std::string &blockset_name)
410 : mField(m_field), problemName(problem_name), fieldName(
field_name) {}
427 return reactionsMap.at(
id);
443 #endif //__DIRICHLET_HPP__
std::vector< std::string > fieldNames
Set Dirichlet boundary conditions on spatial positions by removing dofs.
VectorBoundedArray< double, 3 > VectorDouble3
const std::string blocksetName
Data structure to exchange data between mofem and User Loop Methods on entities.
structure for User Loop Methods on finite elements
std::vector< std::string > fixFields
PetscErrorCode MoFEMErrorCode
MoFEM/PETSc error code.
this struct keeps basic methods for moab meshset about material and boundary conditions
DEPRECATED typedef DirichletSetFieldFromBlockWithFlags DirichletBCFromBlockSetFEMethodPreAndPostProc
DirichletDisplacementBc * dirichletBcPtr
MoFEMErrorCode operator()()
function is run for every finite element
multi_index_container< boost::shared_ptr< FieldEntity >, indexed_by< ordered_unique< tag< Unique_mi_tag >, member< FieldEntity, UId, &FieldEntity::localUId > >, ordered_non_unique< tag< Ent_mi_tag >, const_mem_fun< FieldEntity::interface_type_RefEntity, EntityHandle, &FieldEntity::getEnt > > > > FieldEntity_multiIndex
MultiIndex container keeps FieldEntity.
Add boundary conditions form block set having 6 attributes.
Reactions(MoFEM::Interface &m_field, string problem_name, string field_name)
calculate reactions from vector of internal forces on meshsets
Set Dirichlet boundary conditions on displacements.
DirichletTemperatureBc(MoFEM::Interface &m_field, const std::string &field_name)
DirichletSetFieldFromBlockWithFlags(MoFEM::Interface &m_field, const std::string &field_name, const std::string &blockset_name)
MoFEMErrorCode postProcess()
function is run at the end of loop
Deprecated interface functions.
MoFEMErrorCode preProcess()
function is run at the beginning of loop
DirichletSetFieldFromBlockWithFlags(MoFEM::Interface &m_field, const std::string &field_name, const std::string &blockset_name, Mat aij, Vec x, Vec f)
const ReactionsMap & getReactionsMap() const
Get the Reactions Map.
MoFEMErrorCode calculateRotationForDof(VectorDouble3 &coords, DataFromBc &bc_data)
Calculate displacements from rotation for particular dof.
virtual boost::shared_ptr< EntityMethod > getEntMethodPtr(DataFromBc &data)
#define CHKERR
Inline error check.
FTensor::Tensor1< double, 3 > t_normal
DirichletDisplacementRemoveDofsBc(MoFEM::Interface &m_field, const std::string &field_name, const std::string &problem_name, string blockset_name="DISPLACEMENT", bool is_partitioned=false)
MoFEMErrorCode operator()()
function is run for every finite element
DEPRECATED typedef DirichletSetFieldFromBlockWithFlags DirichletSetFieldFromBlock
BcEntMethodSpatial(DirichletDisplacementBc *dirichlet_bc_ptr, DataFromBc &data_from_dirichlet_bc, string material_positions)
FTensor::Tensor1< double, 3 > t_centr
std::string materialPositions
std::vector< double > dofsValues
DirichletDisplacementBc * dirichletBcPtr
DataFromBc & dataFromDirichletBc
Data from Cubit blocksets.
DirichletSpatialRemoveDofsBc(MoFEM::Interface &m_field, const std::string &field_name, const std::string &problem_name, const std::string material_positions="MESH_NODE_POSITIONS", string blockset_name="DISPLACEMENT", bool is_partitioned=false)
DirichletSpatialPositionsBc(MoFEM::Interface &m_field, const std::string &field_name, const std::string material_positions="MESH_NODE_POSITIONS", const std::string blockset_name="DISPLACEMENT")
MoFEMErrorCode preProcess()
function is run at the beginning of loop
VectorDouble scaled_values
DirichletFixFieldAtEntitiesBc(MoFEM::Interface &m_field, const std::string field_name, Mat aij, Vec x, Vec f, Range &ents)
MoFEMErrorCode postProcess()
function is run at the end of loop
std::string materialPositions
FTensor::Index< 'i', SPACE_DIM > i
MoFEMErrorCode postProcess()
function is run at the end of loop
DirichletTemperatureBc(MoFEM::Interface &m_field, const std::string &field_name, Mat aij, Vec x, Vec f)
constexpr auto field_name
ReactionsMap reactionsMap
DEPRECATED typedef DirichletSetFieldFromBlockWithFlags DirichletBCFromBlockSetFEMethodPreAndPostProcWithFlags
MoFEMErrorCode operator()()
function is run for every finite element
BcEntMethodDisp(DirichletDisplacementBc *dirichlet_bc_ptr, DataFromBc &data_from_dirichlet_bc)
boost::shared_ptr< vector< DataFromBc > > bcDataPtr
const double v
phase velocity of light in medium (cm/ns)
std::vector< double > dofsXValues
MoFEM::Interface & mField
UBlasVector< int > VectorInt
const std::string fieldName
field name to set Dirichlet BC
DataFromBc & dataFromDirichletBc
MoFEM::Interface & mField
const VectorDouble & getReactionsFromSet(const int &id) const
Get the Reactions at specified meshset id.
DEPRECATED typedef DirichletSpatialPositionsBc SpatialPositionsBCFEMethodPreAndPostProc
const FTensor::Tensor2< T, Dim, Dim > Vec
VectorDouble initial_values
double dIag
diagonal value set on zeroed column and rows
boost::ptr_vector< MethodForForceScaling > methodsOp
DEPRECATED typedef DirichletFixFieldAtEntitiesBc FixBcAtEntities
DirichletSpatialPositionsBc(MoFEM::Interface &m_field, const std::string &field_name, Mat aij, Vec x, Vec f, const std::string material_positions="MESH_NODE_POSITIONS", const std::string blockset_name="DISPLACEMENT")
UBlasVector< double > VectorDouble
DirichletFixFieldAtEntitiesBc(MoFEM::Interface &m_field, const std::string field_name, Range &ents)
Set Dirichlet boundary conditions on displacements by removing dofs.
std::map< DofIdx, FieldData > mapZeroRows
std::map< int, VectorDouble > ReactionsMap
#define MoFEMFunctionReturn(a)
Last executable line of each PETSc function used for error handling. Replaces return()
DEPRECATED typedef DirichletTemperatureBc TemperatureBCFEMethodPreAndPostProc
MoFEMErrorCode operator()()
function is run for every finite element
std::vector< int > dofsIndices
#define MoFEMFunctionBegin
First executable line of each MoFEM function, used for error handling. Final line of MoFEM functions ...
DEPRECATED typedef DirichletDisplacementBc DisplacementBCFEMethodPreAndPostProc
Set Dirichlet boundary conditions on spatial displacements.
boost::shared_ptr< EntityMethod > getEntMethodPtr(DataFromBc &data) override