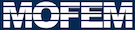 |
| v0.14.0
|
calculate reactions from vector of internal forces on meshsets
More...
#include <users_modules/basic_finite_elements/src/DirichletBC.hpp>
calculate reactions from vector of internal forces on meshsets
example usage
feRhs->snes_ctx = FEMethod::CTX_SNESSETFUNCTION;
feRhs->snes_f = F_int;
VecAssemblyBegin(F_int);
VecAssemblyEnd(F_int);
VecGhostUpdateBegin(F_int, INSERT_VALUES, SCATTER_FORWARD);
VecGhostUpdateEnd(F_int, INSERT_VALUES, SCATTER_FORWARD);
Reactions my_react(m_field,
"DM_ELASTIC",
"U");
my_react.calculateReactions(F_int);
int fix_nodes_meshset_id = 1;
cout << my_react.getReactionsFromSet(fix_nodes_meshset_id) << endl;
Definition at line 407 of file DirichletBC.hpp.
◆ ReactionsMap
◆ Reactions()
Reactions::Reactions |
( |
MoFEM::Interface & |
m_field, |
|
|
string |
problem_name, |
|
|
string |
field_name |
|
) |
| |
|
inline |
◆ calculateReactions()
calculate reactions from a given vector
- Parameters
-
- Returns
- MoFEMErrorCode
Definition at line 782 of file DirichletBC.cpp.
789 CHKERR VecGetArrayRead(
internal, &array);
794 std::vector<int> ghosts(nb_coefficients);
795 for (
int g = 0;
g != nb_coefficients; ++
g)
802 &*ghosts.begin(), &
v);
807 const int id = it->getMeshsetId();
809 reaction_vec.resize(nb_coefficients);
810 reaction_vec.clear();
813 for (
int dim = 0; dim != 3; ++dim) {
819 verts.insert(nodes.begin(), nodes.end());
822 auto for_each_dof = [&](
auto &dof) {
824 reaction_vec[dof->getDofCoeffIdx()] += array[dof->getPetscLocalDofIdx()];
830 verts, for_each_dof);
834 CHKERR VecGetArray(
v, &res_array);
835 for (
int dd = 0;
dd != reaction_vec.size(); ++
dd)
836 res_array[
dd] = reaction_vec[
dd];
837 CHKERR VecRestoreArray(
v, &res_array);
839 CHKERR VecGetArray(
v, &res_array);
840 for (
int dd = 0;
dd != reaction_vec.size(); ++
dd)
841 reaction_vec[
dd] = res_array[
dd];
842 CHKERR VecRestoreArray(
v, &res_array);
845 CHKERR VecGhostUpdateBegin(
v, ADD_VALUES, SCATTER_REVERSE);
846 CHKERR VecGhostUpdateEnd(
v, ADD_VALUES, SCATTER_REVERSE);
847 CHKERR VecGhostUpdateBegin(
v, INSERT_VALUES, SCATTER_FORWARD);
848 CHKERR VecGhostUpdateEnd(
v, INSERT_VALUES, SCATTER_FORWARD);
851 CHKERR VecRestoreArrayRead(
internal, &array);
◆ getReactionsFromSet()
const VectorDouble& Reactions::getReactionsFromSet |
( |
const int & |
id | ) |
const |
|
inline |
Get the Reactions at specified meshset id.
- Parameters
-
id | meshset id (from Cubit) |
- Returns
- const VectorDouble&
Definition at line 426 of file DirichletBC.hpp.
◆ getReactionsMap()
◆ fieldName
std::string Reactions::fieldName |
|
private |
◆ mField
◆ problemName
std::string Reactions::problemName |
|
private |
◆ reactionsMap
The documentation for this struct was generated from the following files:
#define MoFEMFunctionReturnHot(a)
Last executable line of each PETSc function used for error handling. Replaces return()
FieldCoefficientsNumber getNbOfCoeffs() const
Get number of field coefficients.
virtual MPI_Comm & get_comm() const =0
virtual const Field * get_field_structure(const std::string &name, enum MoFEMTypes bh=MF_EXIST) const =0
get field structure
#define _IT_CUBITMESHSETS_BY_BCDATA_TYPE_FOR_LOOP_(MESHSET_MANAGER, CUBITBCTYPE, IT)
Iterator that loops over a specific Cubit MeshSet in a moFEM field.
virtual FieldBitNumber get_field_bit_number(const std::string name) const =0
get field bit number
virtual int get_comm_rank() const =0
virtual const Problem * get_problem(const std::string problem_name) const =0
Get the problem object.
calculate reactions from vector of internal forces on meshsets
PetscErrorCode DMCreateGlobalVector_MoFEM(DM dm, Vec *g)
DMShellSetCreateGlobalVector.
#define CHKERR
Inline error check.
virtual moab::Interface & get_moab()=0
constexpr auto field_name
ReactionsMap reactionsMap
static MoFEMErrorCode set_numered_dofs_on_ents(const Problem *problem_ptr, const FieldBitNumber bit_number, Range &ents, boost::function< MoFEMErrorCode(const boost::shared_ptr< MoFEM::NumeredDofEntity > &dof)> for_each_dof)
const double v
phase velocity of light in medium (cm/ns)
const Tensor2_symmetric_Expr< const ddTensor0< T, Dim, i, j >, typename promote< T, double >::V, Dim, i, j > dd(const Tensor0< T * > &a, const Index< i, Dim > index1, const Index< j, Dim > index2, const Tensor1< int, Dim > &d_ijk, const Tensor1< double, Dim > &d_xyz)
MoFEM::Interface & mField
const FTensor::Tensor2< T, Dim, Dim > Vec
UBlasVector< double > VectorDouble
#define MoFEMFunctionBeginHot
First executable line of each MoFEM function, used for error handling. Final line of MoFEM functions ...
keeps basic data about problem
PetscErrorCode DMoFEMLoopFiniteElements(DM dm, const char fe_name[], MoFEM::FEMethod *method, CacheTupleWeakPtr cache_ptr=CacheTupleSharedPtr())
Executes FEMethod for finite elements in DM.
#define MoFEMFunctionReturn(a)
Last executable line of each PETSc function used for error handling. Replaces return()
#define MoFEMFunctionBegin
First executable line of each MoFEM function, used for error handling. Final line of MoFEM functions ...