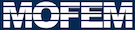 |
| v0.14.0
|
Go to the documentation of this file.
8 #ifndef __EDGE_FORCE_HPP__
9 #define __EDGE_FORCE_HPP__
41 boost::ptr_vector<MethodForForceScaling> &
methodsOp;
45 boost::ptr_vector<MethodForForceScaling> &methods_op,
46 bool use_snes_f =
false);
56 bool use_snes_f =
false);
64 Range *intersect_ptr = NULL) {
72 "FORCE_FE",
"MESH_NODE_POSITIONS");
77 CHKERR m_field.
get_moab().get_entities_by_type(it->meshset, MBTRI, tris,
80 CHKERR m_field.
get_moab().get_entities_by_type(it->meshset, MBEDGE, edges,
83 CHKERR m_field.
get_moab().get_adjacencies(tris, 1,
false, tris_edges,
84 moab::Interface::UNION);
85 edges = subtract(edges, tris_edges);
87 edges = intersect(edges, *intersect_ptr);
98 boost::ptr_map<std::string, EdgeForce> &edge_forces,
Vec F,
100 std::string mesh_node_positions =
"MESH_NODE_POSITIONS") {
102 string fe_name =
"FORCE_FE";
103 edge_forces.insert(fe_name,
new EdgeForce(m_field));
105 auto &fe = edge_forces.at(fe_name).getLoopFe();
108 if (nb_coefficients == 3) {
109 fe.getOpPtrVector().push_back(
110 new OpGetHOTangentsOnEdge<3>(mesh_node_positions));
112 else if (nb_coefficients == 2) {
113 fe.getOpPtrVector().push_back(
114 new OpGetHOTangentsOnEdge<2>(mesh_node_positions));
126 #endif //__EDGE_FORCE_HPP__
MoFEMErrorCode doWork(int side, EntityType type, EntitiesFieldData::EntData &data)
boost::ptr_vector< MethodForForceScaling > & methodsOp
MyFE(MoFEM::Interface &m_field, int add_to_rule)
FieldCoefficientsNumber getNbOfCoeffs() const
Get number of field coefficients.
Force on edges and lines.
boost::ptr_vector< MethodForForceScaling > methodsOp
virtual MoFEMErrorCode modify_finite_element_add_field_row(const std::string &fe_name, const std::string name_row)=0
set field row which finite element use
virtual const Field * get_field_structure(const std::string &name, enum MoFEMTypes bh=MF_EXIST) const =0
get field structure
PetscErrorCode MoFEMErrorCode
MoFEM/PETSc error code.
#define _IT_CUBITMESHSETS_BY_BCDATA_TYPE_FOR_LOOP_(MESHSET_MANAGER, CUBITBCTYPE, IT)
Iterator that loops over a specific Cubit MeshSet in a moFEM field.
Deprecated interface functions.
#define CHKERR
Inline error check.
virtual MoFEMErrorCode add_finite_element(const std::string &fe_name, enum MoFEMTypes bh=MF_EXCL, int verb=DEFAULT_VERBOSITY)=0
add finite element
virtual moab::Interface & get_moab()=0
virtual MoFEMErrorCode add_ents_to_finite_element_by_type(const EntityHandle entities, const EntityType type, const std::string name, const bool recursive=true)=0
add entities to finite element
virtual MoFEMErrorCode modify_finite_element_add_field_col(const std::string &fe_name, const std::string name_row)=0
set field col which finite element use
EdgeForce(MoFEM::Interface &m_field)
virtual bool check_field(const std::string &name) const =0
check if field is in database
virtual MoFEMErrorCode modify_finite_element_add_field_data(const std::string &fe_name, const std::string name_field)=0
set finite element field data
EntitiesFieldData::EntData EntData
constexpr auto field_name
MoFEM::Interface & mField
default operator for EDGE element
MoFEMErrorCode addForce(const std::string field_name, Vec F, int ms_id, bool use_snes_f=false)
const FTensor::Tensor2< T, Dim, Dim > Vec
UBlasVector< double > VectorDouble
#define MoFEMFunctionReturn(a)
Last executable line of each PETSc function used for error handling. Replaces return()
OpEdgeForce(const std::string field_name, Vec f, bCForce &data, boost::ptr_vector< MethodForForceScaling > &methods_op, bool use_snes_f=false)
std::map< int, bCForce > mapForce
#define MoFEMFunctionBegin
First executable line of each MoFEM function, used for error handling. Final line of MoFEM functions ...