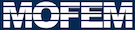 |
| v0.14.0
|
Go to the documentation of this file.
13 #ifndef __MED_INTERFACE_HPP__
14 #define __MED_INTERFACE_HPP__
103 boost::shared_ptr<std::vector<const CubitMeshSets *>> meshsets_ptr,
104 boost::shared_ptr<Range> range_ptr =
nullptr,
int verb = 1);
116 const bool load_series =
false,
117 const int only_step = -1,
int verb = 1);
151 std::map<int, Range> &family_elem_map,
int verb = 1);
163 const std::map<int, Range> &family_elem_mapint,
164 std::map<string, Range> &group_elem_map,
182 #endif // __MED_INTERFACE_HPP__
MoFEMErrorCode query_interface(boost::typeindex::type_index type_index, UnknownInterface **iface) const
std::ostream & operator<<(std::ostream &os, const EntitiesFieldData::EntData &e)
MoFEMErrorCode getMeshsets(boost::shared_ptr< std::vector< const CubitMeshSets * >> &meshsets_ptr)
Interface for load MED files.
MoFEMErrorCode makeBlockSets(const std::map< string, Range > &group_elem_map, int verb=1)
make from groups meshsets
std::string medFileName
MED file name.
multi_index_container< CubitMeshSets, indexed_by< hashed_unique< tag< Meshset_mi_tag >, member< CubitMeshSets, EntityHandle, &CubitMeshSets::meshset > >, ordered_non_unique< tag< CubitMeshsetType_mi_tag >, const_mem_fun< CubitMeshSets, unsigned long int, &CubitMeshSets::getBcTypeULong > >, ordered_non_unique< tag< CubitMeshsetMaskedType_mi_tag >, const_mem_fun< CubitMeshSets, unsigned long int, &CubitMeshSets::getMaskedBcTypeULong > >, ordered_non_unique< tag< CubitMeshsets_name >, const_mem_fun< CubitMeshSets, std::string, &CubitMeshSets::getName > >, hashed_unique< tag< Composite_Cubit_msId_And_MeshsetType_mi_tag >, composite_key< CubitMeshSets, const_mem_fun< CubitMeshSets, int, &CubitMeshSets::getMeshsetId >, const_mem_fun< CubitMeshSets, unsigned long int, &CubitMeshSets::getMaskedBcTypeULong > > > > > CubitMeshSet_multiIndex
Stores data about meshsets (see CubitMeshSets) storing data about boundary conditions,...
std::vector< std::string > meshNames
list of meshes in MED file
PetscErrorCode MoFEMErrorCode
MoFEM/PETSc error code.
MoFEMErrorCode readFamily(const string &file, const int index, const std::map< int, Range > &family_elem_mapint, std::map< string, Range > &group_elem_map, int verb=1)
read family and groups
MoFEMErrorCode readMed(const string &file, int verb=1)
read MED file
std::map< std::string, FieldData > fieldNames
PetscBool getFlgFile() const
Check if file name is given in command line.
MoFEMErrorCode writeMed(boost::shared_ptr< Range > range_ptr=nullptr, int verb=1)
write MED file
implementation of Data Operators for Forces and Sources
PetscBool flgFile
true if file name given in command line
MoFEMErrorCode medGetFieldNames(const string &file, int verb=1)
Get field names in MED file.
MoFEMErrorCode getFileNameFromCommandLine(int verb=1)
Get MED file name from command line.
MoFEMErrorCode readFields(const std::string &file_name, const std::string &field_name, const bool load_series=false, const int only_step=-1, int verb=1)
std::vector< EntityHandle > meshMeshsets
meshset for each mesh
constexpr auto field_name
base class for all interface classes
MoFEMErrorCode readMesh(const string &file, const int index, std::map< int, Range > &family_elem_map, int verb=1)
read mesh from MED file
MedInterface(const MoFEM::Core &core)
std::vector< std::string > componentNames
std::vector< std::string > componentUnits
MoFEM::Core & cOre
core database
CubitMeshSet_multiIndex cubitMeshsets
cubit meshsets