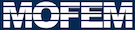 |
| v0.14.0
|
Go to the documentation of this file.
9 #ifndef __NODE_MERGER_HPP__
10 #define __NODE_MERGER_HPP__
58 const bool only_if_improve_quality =
false,
59 const double move = 0,
const int line_search = 0,
60 Tag
th = NULL,
const int verb = 0);
79 const bool only_if_improve_quality =
false,
80 const double move = 0, Tag
th = NULL);
99 const bool only_if_improve_quality =
false,
100 const double move = 0, Tag
th = NULL);
109 typedef multi_index_container<
111 indexed_by<ordered_unique<
112 member<ParentChild, EntityHandle, &ParentChild::pArent>>,
114 member<ParentChild, EntityHandle, &ParentChild::cHild>>>>
141 double *coords_move,
double &min_quality, Tag
th = NULL,
142 boost::function<
double(
double,
double)>
f =
143 [](
double a,
double b) ->
double {
return std::min(
a, b); });
166 typedef multi_index_container<
167 FaceMap, indexed_by<hashed_unique<composite_key<
168 FaceMap, member<FaceMap, EntityHandle, &FaceMap::n0>,
169 member<FaceMap, EntityHandle, &FaceMap::n1>>>>>
176 #endif //__NODE_MERGER_HPP__
bool errorIfNoCommonEdge
Send error if no common edge.
MoFEMErrorCode check_tests(int ss, int test_num, double &expected_energy, double &expected_contact_area, int &expected_nb_gauss_pts)
MoFEMErrorCode query_interface(boost::typeindex::type_index type_index, UnknownInterface **iface) const
multi_index_container< FaceMap, indexed_by< hashed_unique< composite_key< FaceMap, member< FaceMap, EntityHandle, &FaceMap::n0 >, member< FaceMap, EntityHandle, &FaceMap::n1 > > > > > FaceMapIdx
ParentChildMap & getParentChildMap()
Get map of parent cand child.
MoFEMErrorCode mergeNodes(EntityHandle father, EntityHandle mother, Range &out_tets, Range *tets_ptr=NULL, const bool only_if_improve_quality=false, const double move=0, const int line_search=0, Tag th=NULL, const int verb=0)
merge nodes which sharing edge
PetscErrorCode MoFEMErrorCode
MoFEM/PETSc error code.
NodeMergerInterface(const MoFEM::Core &core)
Merge node by collapsing edge between them.
boost::function< double(const double a, const double b)> minQualityFunction
ParentChildMap parentChildMap
implementation of Data Operators for Forces and Sources
multi_index_container< ParentChild, indexed_by< ordered_unique< member< ParentChild, EntityHandle, &ParentChild::pArent > >, ordered_non_unique< member< ParentChild, EntityHandle, &ParentChild::cHild > > > > ParentChildMap
FaceMap(const EntityHandle e, const EntityHandle n0, const EntityHandle n1)
bool getSuccessMerge()
Return true if successful merge.
base class for all interface classes
MoFEMErrorCode lineSearch(Range &check_tests, EntityHandle father, EntityHandle mother, int line_search, FTensor::Tensor1< double, 3 > &t_move, Tag th=NULL)
Use bisection method to find point of edge collapse.
MoFEMErrorCode getSubInterfaceOptions()
bool successMerge
True if marge is success.
std::bitset< BITREFLEVEL_SIZE > BitRefLevel
Bit structure attached to each entity identifying to what mesh entity is attached.
ParentChild(const EntityHandle parent, const EntityHandle child)
void setErrorIfNoCommonEdge(const bool b=true)
Set error if no common edge.
MoFEMErrorCode minQuality(Range &check_tests, EntityHandle father, EntityHandle mother, double *coords_move, double &min_quality, Tag th=NULL, boost::function< double(double, double)> f=[](double a, double b) -> double { return std::min(a, b);})
Calualte quality if nodes merged.