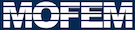 |
| v0.14.0
|
Go to the documentation of this file.
10 #ifndef __NONLINEAR_ELASTIC_HPP
11 #define __NONLINEAR_ELASTIC_HPP
14 #error "MoFEM need to be compiled with ADOL-C"
91 boost::shared_ptr<FunctionsToCalculatePiolaKirchhoffI<adouble>>
93 boost::shared_ptr<FunctionsToCalculatePiolaKirchhoffI<double>>
99 std::map<int, BlockData>
112 std::vector<MatrixDouble>
147 MatrixBoundedArray<TYPE, 9>
F,
C,
E,
S,
invF,
P,
sIGma,
h,
H,
invH,
206 boost::shared_ptr<const NumeredEntFiniteElement> fe_ptr) {
242 boost::shared_ptr<const NumeredEntFiniteElement> fe_ptr) {
294 boost::shared_ptr<const NumeredEntFiniteElement> fe_ptr) {
302 for (
int ii = 0; ii < 3; ii++) {
304 for (
int jj = 0; jj < 3; jj++) {
317 boost::shared_ptr<const NumeredEntFiniteElement> fe_ptr) {
329 std::map<std::string, std::vector<VectorDouble>> &field_map,
330 std::map<std::string, std::vector<MatrixDouble>> &grad_map) {
337 m.resize(3, 3,
false);
350 std::vector<VectorDouble> &values_at_gauss_pts,
351 std::vector<MatrixDouble> &gradient_at_gauss_pts);
401 int tag,
bool jacobian,
bool ale,
407 std::vector<MatrixDouble> *
ptrh;
408 std::vector<MatrixDouble> *
ptrH;
475 bool gradient,
bool hessian,
bool ale,
bool field_disp);
480 std::vector<MatrixDouble> *
ptrh;
481 std::vector<MatrixDouble> *
ptrH;
549 CommonData &common_data, SmartPetscObj<Vec> ghost_vec,
588 EntityType row_type, EntityType col_type,
616 CommonData &common_data,
int tag,
bool jacobian,
656 const std::string element_name,
657 const std::string spatial_position_field_name,
658 const std::string material_position_field_name =
"MESH_NODE_POSITIONS",
659 const bool ale =
false);
672 const std::string spatial_position_field_name,
673 const std::string material_position_field_name =
"MESH_NODE_POSITIONS",
674 const bool ale =
false,
const bool field_disp =
false);
677 #endif //__NONLINEAR_ELASTIC_HPP
#define MoFEMFunctionReturnHot(a)
Last executable line of each PETSc function used for error handling. Replaces return()
std::vector< double > eNergy
OpGetCommonDataAtGaussPts(const std::string field_name, CommonData &common_data)
FTensor::Tensor2< FTensor::PackPtr< TYPE *, 0 >, 3, 3 > t_H
OpGetDataAtGaussPts(const std::string field_name, std::vector< VectorDouble > &values_at_gauss_pts, std::vector< MatrixDouble > &gradient_at_gauss_pts)
FunctionsToCalculatePiolaKirchhoffI()
virtual MoFEMErrorCode calculateP_PiolaKirchhoffI(const BlockData block_data, boost::shared_ptr< const NumeredEntFiniteElement > fe_ptr)
Function overload to implement user material.
MoFEM::VolumeElementForcesAndSourcesCore::UserDataOperator * opPtr
pointer to finite element tetrahedral operator
boost::shared_ptr< FunctionsToCalculatePiolaKirchhoffI< adouble > > materialAdoublePtr
bool fieldDisp
true if displacements instead spatial positions used
MoFEM::Interface & mField
FTensor::Tensor2< FTensor::PackPtr< TYPE *, 0 >, 3, 3 > t_P
Implementation of elastic (non-linear) St. Kirchhoff equation.
MoFEMErrorCode postProcess()
function is run at the end of loop
Operator performs automatic differentiation.
std::vector< MatrixDouble > jacStress
this is simply material tangent operator
OpJacobianEnergy(const std::string field_name, BlockData &data, CommonData &common_data, int tag, bool gradient, bool hessian, bool ale, bool field_disp)
virtual MoFEMErrorCode getDataOnPostProcessor(std::map< std::string, std::vector< VectorDouble >> &field_map, std::map< std::string, std::vector< MatrixDouble >> &grad_map)
Do operations when pre-process.
FTensor::Tensor2< FTensor::PackPtr< TYPE *, 0 >, 3, 3 > t_E
structure grouping operators and data used for calculation of nonlinear elastic element
MyVolumeFE feRhs
calculate right hand side for tetrahedral elements
VectorDouble activeVariables
PetscErrorCode MoFEMErrorCode
MoFEM/PETSc error code.
DEPRECATED typedef OpRhsEshelbyStress OpRhsEshelbyStrees
UBlasMatrix< double > MatrixDouble
MoFEMErrorCode aSemble(int row_side, int col_side, EntityType row_type, EntityType col_type, EntitiesFieldData::EntData &row_data, EntitiesFieldData::EntData &col_data)
ublas::vector< int > colIndices
OpJacobianEshelbyStress(const std::string field_name, BlockData &data, CommonData &common_data, int tag, bool jacobian, bool ale)
virtual MoFEMErrorCode setUserActiveVariables(int &nb_active_variables)
add additional active variables
MoFEMErrorCode doWork(int row_side, EntityType row_type, EntitiesFieldData::EntData &row_data)
MatrixBoundedArray< TYPE, 9 > h
FTensor::Tensor2< FTensor::PackPtr< TYPE *, 0 >, 3, 3 > t_sigmaCauchy
FTensor::Index< 'j', 3 > j
MoFEMErrorCode doWork(int row_side, int col_side, EntityType row_type, EntityType col_type, EntitiesFieldData::EntData &row_data, EntitiesFieldData::EntData &col_data)
OpRhsPiolaKirchhoff(const std::string field_name, BlockData &data, CommonData &common_data)
MoFEMErrorCode preProcess()
function is run at the beginning of loop
FTensor::Index< 'i', 3 > i
MatrixBoundedArray< TYPE, 9 > sIGma
VectorDouble activeVariables
SmartPetscObj< Vec > ghostVec
FTensor::Index< 'i', 3 > i
Deprecated interface functions.
bool aLe
true if arbitrary Lagrangian-Eulerian formulation
FTensor::Index< 'j', 3 > j
Range forcesOnlyOnEntitiesRow
FTensor::Tensor2< FTensor::PackPtr< TYPE *, 0 >, 3, 3 > t_h
MoFEMErrorCode calculateStress(const int gg)
Calculate Paola-Kirchhoff I stress.
FTensor::Tensor2< FTensor::PackPtr< TYPE *, 0 >, 3, 3 > t_sIGma
virtual MoFEMErrorCode setUserActiveVariables(VectorDouble &activeVariables)
Add additional independent variables More complex physical models depend on gradient of defamation an...
virtual bool recordTagForIntegrationPoint(const int gg)
Cgeck if tape is recorded for given integration point.
#define CHKERR
Inline error check.
OpLhsPiolaKirchhoff_dx(const std::string vel_field, const std::string field_name, BlockData &data, CommonData &common_data)
std::vector< MatrixDouble > * ptrh
OpJacobianPiolaKirchhoffStress(const std::string field_name, BlockData &data, CommonData &common_data, int tag, bool jacobian, bool ale, bool field_disp)
Construct operator to calculate Piola-Kirchhoff stress or its derivatives over gradient deformation.
std::vector< VectorDouble > jacEnergy
OpLhsEshelby_dx(const std::string vel_field, const std::string field_name, BlockData &data, CommonData &common_data)
FTensor::Tensor2< FTensor::PackPtr< TYPE *, 0 >, 3, 3 > t_invH
MatrixBoundedArray< TYPE, 9 > H
virtual bool recordTagForIntegrationPoint(const int gg)
Check if tape is recorded for given integration point.
MatrixBoundedArray< TYPE, 9 > F
ublas::vector< int > rowIndices
FTensor::Index< 'k', 3 > k
std::vector< MatrixDouble > * ptrH
int getRule(int order)
it is used to calculate nb. of Gauss integration points
virtual MoFEMErrorCode calculateStress(const int gg)
Calculate Paola-Kirchhoff I stress.
bool hEssian
if set true hessian of energy is calculated
Calculate explicit derivative of free energy.
FTensor::Tensor2< FTensor::PackPtr< TYPE *, 0 >, 3, 3 > t_F
std::vector< VectorDouble > hessianEnergy
OpLhsPiolaKirchhoff_dX(const std::string vel_field, const std::string field_name, BlockData &data, CommonData &common_data)
FTensor::Tensor2< FTensor::PackPtr< TYPE *, 0 >, 3, 3 > t_S
FTensor::Index< 'i', 3 > i
bool fUnction
if true stress i calculated
MoFEMErrorCode doWork(int side, EntityType type, EntitiesFieldData::EntData &data)
operator calculating deformation gradient
virtual MoFEMErrorCode calculateElasticEnergy(const BlockData block_data, boost::shared_ptr< const NumeredEntFiniteElement > fe_ptr)
Calculate elastic energy density.
MoFEMErrorCode addElement(const std::string element_name, const std::string spatial_position_field_name, const std::string material_position_field_name="MESH_NODE_POSITIONS", const bool ale=false)
FTensor::Index< 'i', 3 > i
virtual MoFEMErrorCode playTag(const int gg)
Play ADOL-C tape.
data for calculation heat conductivity and heat capacity elements
virtual MoFEMErrorCode playTag(const int gg)
Play ADOL-C tape.
MatrixBoundedArray< TYPE, 9 > C
const EntityType zeroAtType
virtual MoFEMErrorCode recordTag(const int gg)
Record ADOL-C tape.
Volume finite element base.
MoFEMErrorCode doWork(int row_side, EntityType row_type, EntitiesFieldData::EntData &row_data)
FTensor::Index< 'k', 3 > k
FTensor::Tensor2< FTensor::PackPtr< TYPE *, 0 >, 3, 3 > t_C
std::vector< MatrixDouble > & gradientAtGaussPts
MoFEMErrorCode setOperators(const std::string spatial_position_field_name, const std::string material_position_field_name="MESH_NODE_POSITIONS", const bool ale=false, const bool field_disp=false)
Set operators to calculate left hand tangent matrix and right hand residual.
EntitiesFieldData::EntData EntData
bool aLe
true if arbitrary Lagrangian-Eulerian formulation
constexpr auto field_name
virtual MoFEMErrorCode calculatesIGma_EshelbyStress(const BlockData block_data, boost::shared_ptr< const NumeredEntFiniteElement > fe_ptr)
Calculate Eshelby stress.
OpEnergy(const std::string field_name, BlockData &data, CommonData &common_data, SmartPetscObj< Vec > ghost_vec, bool field_disp)
virtual MoFEMErrorCode recordTag(const int gg)
Record ADOL-C tape.
MatrixBoundedArray< TYPE, 9 > invH
auto getFTensor2FromArray3by3(ublas::matrix< T, L, A > &data, const FTensor::Number< S > &, const size_t rr, const size_t cc=0)
std::vector< MatrixDouble3by3 > sTress
definition of volume element
static auto determinantTensor3by3(T &t)
Calculate the determinant of a 3x3 matrix or a tensor of rank 2.
MatrixBoundedArray< TYPE, 9 > S
FTensor::Index< 'k', 3 > k
Range forcesOnlyOnEntitiesCol
virtual ~MyVolumeFE()=default
MoFEMErrorCode calculateE_GreenStrain()
std::map< int, BlockData > setOfBlocks
maps block set id with appropriate BlockData
bool gRadient
if set true gradient of energy is calculated
virtual ~FunctionsToCalculatePiolaKirchhoffI()=default
virtual MoFEMErrorCode calculateCauchyStress(const BlockData block_data, boost::shared_ptr< const NumeredEntFiniteElement > fe_ptr)
Function overload to implement user material.
Range tEts
constrains elements in block set
const FTensor::Tensor2< T, Dim, Dim > Vec
MyVolumeFE(MoFEM::Interface &m_field)
ublas::vector< int > iNdices
MyVolumeFE feEnergy
calculate elastic energy
static auto resizeAndSet(MatrixBoundedArray< TYPE, 9 > &m)
UBlasVector< double > VectorDouble
std::map< std::string, std::vector< VectorDouble > > dataAtGaussPts
MoFEMErrorCode doWork(int row_side, EntityType row_type, EntitiesFieldData::EntData &row_data)
Calculate stress or jacobian at gauss points.
MatrixBoundedArray< TYPE, 9 > sigmaCauchy
MoFEMErrorCode doWork(int row_side, EntityType row_type, EntitiesFieldData::EntData &row_data)
FTensor::Index< 'm', 3 > m
OpRhsEshelbyStress(const std::string field_name, BlockData &data, CommonData &common_data)
double trace(FTensor::Tensor2_symmetric< T, 2 > &t_stress)
MoFEMErrorCode calculateC_CauchyDeformationTensor()
virtual MoFEMErrorCode aSemble(int row_side, EntityType row_type, EntitiesFieldData::EntData &row_data)
#define MoFEMFunctionBeginHot
First executable line of each MoFEM function, used for error handling. Final line of MoFEM functions ...
FTensor::Tensor2< double *, 3, 3 > & getJac()
get element Jacobian
virtual MoFEMErrorCode calculateEnergy(const int gg)
Calculate Paola-Kirchhoff I stress.
FTensor::Index< 'j', 3 > j
NonlinearElasticElement(MoFEM::Interface &m_field, short int tag)
virtual ~NonlinearElasticElement()=default
std::vector< VectorDouble > & valuesAtGaussPts
MatrixBoundedArray< TYPE, 9 > invF
std::vector< MatrixDouble > * ptrH
FTensor::Index< 'j', 3 > j
#define MoFEMFunctionReturn(a)
Last executable line of each PETSc function used for error handling. Replaces return()
OpLhsEshelby_dX(const std::string vel_field, const std::string field_name, BlockData &data, CommonData &common_data)
std::map< std::string, std::vector< MatrixDouble > > gradAtGaussPts
boost::shared_ptr< FunctionsToCalculatePiolaKirchhoffI< double > > materialDoublePtr
MoFEMErrorCode calculateS_PiolaKirchhoffII()
MoFEMErrorCode setBlocks(boost::shared_ptr< FunctionsToCalculatePiolaKirchhoffI< double >> materialDoublePtr, boost::shared_ptr< FunctionsToCalculatePiolaKirchhoffI< adouble >> materialAdoublePtr)
#define MoFEMFunctionBegin
First executable line of each MoFEM function, used for error handling. Final line of MoFEM functions ...
int gG
Gauss point number.
MatrixBoundedArray< TYPE, 9 > P
FTensor::Tensor2< FTensor::PackPtr< TYPE *, 0 >, 3, 3 > t_invF
MatrixBoundedArray< TYPE, 9 > E
CommonData * commonDataPtr
bool jAcobian
if true Jacobian is calculated
std::vector< MatrixDouble > * ptrh
common data used by volume elements
virtual MoFEMErrorCode aSemble(int row_side, int col_side, EntityType row_type, EntityType col_type, EntitiesFieldData::EntData &row_data, EntitiesFieldData::EntData &col_data)
FTensor::Index< 'k', 3 > k