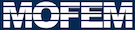 |
| v0.14.0
|
Go to the documentation of this file.
7 #ifndef __SERIESMULTIINDICES_HPP__
8 #define __SERIESMULTIINDICES_HPP__
12 struct FieldSeriesStep;
59 std::vector<FieldData>
data;
68 for (; it != hi_it; it++) {
69 ierr =
push_dofs((*it)->getEnt(), (*it)->getNonNonuniqueShortId(),
70 (*it)->getFieldData());
96 inline boost::string_ref
getNameRef()
const {
return ptr->getNameRef(); }
98 inline std::string
getName()
const {
return ptr->getName(); }
101 return ptr->get_FieldSeries_ptr();
118 const int _step_number);
135 typedef multi_index_container<
137 indexed_by<ordered_unique<tag<SeriesID_mi_tag>,
138 const_mem_fun<FieldSeries, EntityID,
140 ordered_unique<tag<SeriesName_mi_tag>,
141 const_mem_fun<FieldSeries, boost::string_ref,
150 typedef multi_index_container<
154 tag<Composite_SeriesID_And_Step_mi_tag>,
159 member<FieldSeriesStep, int, &FieldSeriesStep::step_number>>>,
161 tag<Composite_SeriesName_And_Step_mi_tag>,
166 member<FieldSeriesStep, int, &FieldSeriesStep::step_number>>>,
168 tag<SeriesName_mi_tag>,
172 tag<Composite_SeriesName_And_Time_mi_tag>,
199 #endif // __SERIESMULTIINDICES_HPP__
#define MoFEMFunctionReturnHot(a)
Last executable line of each PETSc function used for error handling. Replaces return()
EntityHandle getMeshset() const
get meshset
std::vector< ShortId > uids
MoFEMErrorCode push_dofs(const EntityHandle ent, const ShortId uid, const FieldData val)
boost::string_ref getNameRef() const
get string_ref of series
PetscErrorCode MoFEMErrorCode
MoFEM/PETSc error code.
int tagNameSize
number of bits necessary to keep field series
MoFEMErrorCode set_time(double time)
std::string getName() const
get series name
std::string getName() const
get series name
EntityID get_meshset_id() const
EntityID get_meshset_id() const
Deprecated interface functions.
MoFEMErrorCode end(double time=0)
std::vector< double > time
boost::string_ref getNameRef() const
get string_ref of series
std::vector< FieldData > data
implementation of Data Operators for Forces and Sources
int ShortId
Unique Id in the field.
MoFEMErrorCode save(Interface &moab) const
friend std::ostream & operator<<(std::ostream &os, const FieldSeries &e)
FieldSeries(Interface &moab, const EntityHandle _meshset)
multi_index_container< FieldSeriesStep, indexed_by< ordered_unique< tag< Composite_SeriesID_And_Step_mi_tag >, composite_key< FieldSeriesStep, const_mem_fun< FieldSeriesStep::interface_type_FieldSeries, EntityID, &FieldSeriesStep::get_meshset_id >, member< FieldSeriesStep, int, &FieldSeriesStep::step_number > > >, ordered_unique< tag< Composite_SeriesName_And_Step_mi_tag >, composite_key< FieldSeriesStep, const_mem_fun< FieldSeriesStep::interface_type_FieldSeries, boost::string_ref, &FieldSeriesStep::getNameRef >, member< FieldSeriesStep, int, &FieldSeriesStep::step_number > > >, ordered_non_unique< tag< SeriesName_mi_tag >, const_mem_fun< FieldSeriesStep::interface_type_FieldSeries, boost::string_ref, &FieldSeriesStep::getNameRef > >, ordered_non_unique< tag< Composite_SeriesName_And_Time_mi_tag >, composite_key< FieldSeriesStep, const_mem_fun< FieldSeriesStep::interface_type_FieldSeries, boost::string_ref, &FieldSeriesStep::getNameRef >, const_mem_fun< FieldSeriesStep, double, &FieldSeriesStep::get_time > > > > > SeriesStep_multiIndex
Step multi index.
MoFEMErrorCode get_nb_steps(Interface &moab, int &nb_setps) const
MoFEMErrorCode get(Interface &moab, DofEntity_multiIndex &dofsField) const
Structure for recording (time) series.
const FieldSeries * get_FieldSeries_ptr() const
std::vector< EntityHandle > handles
Structure for keeping time and step.
const void * tagName
tag keeps name of the series
int get_step_number() const
MoFEMErrorCode read(Interface &moab)
static MoFEMErrorCodeGeneric< PetscErrorCode > ierr
constexpr IntegrationType IT
#define MoFEMFunctionBeginHot
First executable line of each MoFEM function, used for error handling. Final line of MoFEM functions ...
multi_index_container< boost::shared_ptr< DofEntity >, indexed_by< ordered_unique< tag< Unique_mi_tag >, const_mem_fun< DofEntity, UId, &DofEntity::getLocalUniqueId > >, ordered_non_unique< tag< Ent_mi_tag >, const_mem_fun< DofEntity, EntityHandle, &DofEntity::getEnt > > > > DofEntity_multiIndex
MultiIndex container keeps DofEntity.
MoFEMErrorCode get_time_init(Interface &moab)
MoFEMErrorCode push_dofs(IT it, IT hi_it)
EntityHandle getMeshset() const
get meshset
interface_FieldSeries< FieldSeries > interface_type_FieldSeries
const FieldSeries * get_FieldSeries_ptr() const
interface_FieldSeries(const T *_ptr)
#define CHKERRG(n)
Check error code of MoFEM/MOAB/PETSc function.
friend std::ostream & operator<<(std::ostream &os, const FieldSeriesStep &e)
multi_index_container< FieldSeries, indexed_by< ordered_unique< tag< SeriesID_mi_tag >, const_mem_fun< FieldSeries, EntityID, &FieldSeries::get_meshset_id > >, ordered_unique< tag< SeriesName_mi_tag >, const_mem_fun< FieldSeries, boost::string_ref, &FieldSeries::getNameRef > > > > Series_multiIndex
Series multi index.
FieldSeriesStep(Interface &moab, const FieldSeries *_FieldSeries_ptr, const int _step_number)