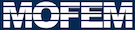 |
| v0.14.0
|
Go to the documentation of this file.
10 #ifndef __TETGENINTERFACE_HPP__
11 #define __TETGENINTERFACE_HPP__
85 std::map<int, Range> &type_ents);
102 bool id_in_tags =
false,
bool error_if_created =
false,
103 bool assume_first_nodes_the_same =
false,
120 bool id_in_tags =
false,
bool error_if_created =
false,
121 bool assume_first_nodes_the_same =
false,
145 tetgenio &out,
Range *ents = NULL,
147 bool only_non_zero =
true);
152 setRegionData(std::vector<std::pair<EntityHandle, int> > ®ions, tetgenio &in,
172 const double eps = 1e-9);
174 const double eps = 1e-9, Tag
th = NULL);
184 std::vector<std::vector<Range> > &sorted,
185 const double eps = 1e-9);
199 bool reduce_edges =
false,
200 Range *not_reducable_nodes = NULL,
201 const double eps = 1e-9,Tag
th = NULL);
205 #endif //__TETGENINTERFACE_HPP__
std::map< unsigned long, EntityHandle > tetGenMoab_Map
MoFEMErrorCode getRegionData(tetGenMoab_Map &tetgen_moab_map, tetgenio &out, Range *ents=NULL, idxRange_Map *ents_map=NULL)
get region data to tetrahedral
MoFEMErrorCode setFaceData(std::vector< std::pair< Range, int > > &markers, tetgenio &in, moabTetGen_Map &moab_tetgen_map, tetGenMoab_Map &tetgen_moab_map)
set markers to faces
PetscErrorCode MoFEMErrorCode
MoFEM/PETSc error code.
MoFEMErrorCode query_interface(boost::typeindex::type_index type_index, UnknownInterface **iface) const
std::map< EntityHandle, unsigned long > moabTetGen_Map
MoFEMErrorCode tetRahedralize(char switches[], tetgenio &in, tetgenio &out)
run tetgen
std::map< int, Range > idxRange_Map
MoFEMErrorCode outData(tetgenio &in, tetgenio &out, moabTetGen_Map &moab_tetgen_map, tetGenMoab_Map &tetgen_moab_map, Range *ents=NULL, bool id_in_tags=false, bool error_if_created=false, bool assume_first_nodes_the_same=false, Tag th=nullptr)
get entities for TetGen data structure
implementation of Data Operators for Forces and Sources
MoFEMErrorCode checkPlanar_Trinagle(double coords[], bool *result, const double eps=1e-9)
base class for all interface classes
MoFEMErrorCode groupRegion_Triangle(Range &tris, std::vector< std::vector< Range > > &sorted, const double eps=1e-9)
Group surface triangles in planar regions.
MoFEMErrorCode groupPlanar_Triangle(Range &tris, std::vector< Range > &sorted, const double eps=1e-9, Tag th=NULL)
MoFEMErrorCode inData(Range &ents, tetgenio &in, moabTetGen_Map &moab_tetgen_map, tetGenMoab_Map &tetgen_moab_map, Tag th=NULL)
create TetGen data structure form range of moab entities
TetGenInterface(const MoFEM::Core &core)
std::bitset< BITREFLEVEL_SIZE > BitRefLevel
Bit structure attached to each entity identifying to what mesh entity is attached.
MoFEMErrorCode setRegionData(std::vector< std::pair< EntityHandle, int > > ®ions, tetgenio &in, Tag th=NULL)
set region data to tetrahedral
MoFEMErrorCode loadPoly(char file_name[], tetgenio &in)
load poly file
MoFEMErrorCode setGeomData(tetgenio &in, moabTetGen_Map &moab_tetgen_map, tetGenMoab_Map &tetgen_moab_map, std::map< int, Range > &type_ents)
set point tags and type
MoFEMErrorCode getTriangleMarkers(tetGenMoab_Map &tetgen_moab_map, tetgenio &out, Range *ents=NULL, idxRange_Map *ents_map=NULL, bool only_non_zero=true)
get markers to faces
MoFEMErrorCode makePolygonFacet(Range &ents, Range &polygons, bool reduce_edges=false, Range *not_reducable_nodes=NULL, const double eps=1e-9, Tag th=NULL)