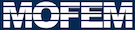 |
| v0.14.0
|
Go to the documentation of this file.
11 using namespace MoFEM;
13 static char help[] =
"testing mesh cut test\n\n";
15 int main(
int argc,
char *argv[]) {
21 auto run_test = [](
auto w,
auto v,
auto k,
auto l,
auto expected_result,
22 double expected_t0,
double expected_t1) {
24 double t0 = 0, t1 = 0;
33 (t_w(
i) + t0 * (t_v(
i) - t_w(
i))) - (t_k(
i) + t1 * (t_l(
i) - t_k(
i)));
34 std::cout <<
"Result " << result <<
" : " << t0 <<
" " << t1 <<
" dist "
35 << sqrt(t_delta(
i) * t_delta(
i)) << std::endl;
37 if (result != expected_result)
39 if (fabs(t0 - expected_t0) > 1e-12)
41 "Wrong value of t0 %3.4e != %3.4e", t0, expected_t0);
42 if (fabs(t1 - expected_t1) > 1e-12)
44 "Wrong value of t1 %3.4e != %3.4e", t1, expected_t1);
49 const double w[] = {-1, 0, 0};
50 const double v[] = {1, 0, 0};
51 const double k[] = {0, -1, 1};
52 const double l[] = {0, 1, 1};
57 const double w[] = {-1, 0, 0};
58 const double v[] = {1, 0, 0};
59 const double k[] = {0, 1, 0};
60 const double l[] = {0, 2, 0};
65 const double w[] = {-1, 0, 0};
66 const double v[] = {1, 0, 0};
67 const double k[] = {-1, -1, 0};
68 const double l[] = {1, 1, 0};
73 const double w[] = {-1, 0, 1};
74 const double v[] = {1, 0, 1};
75 const double k[] = {-1, -1, 0};
76 const double l[] = {1, 1, 0};
81 const double w[] = {0, 0, 0};
82 const double v[] = {0, 0, 0};
83 const double k[] = {0, 0, 0};
84 const double l[] = {0, 1, 0};
89 const double w[] = {1, 0, 0};
90 const double v[] = {1, 0, 0};
91 const double k[] = {0, 0, 0};
92 const double l[] = {1, 0, 0};
97 const double w[] = {0, 0, 0};
98 const double v[] = {1, 0, 0};
99 const double k[] = {1, 0, 0};
100 const double l[] = {1, 0, 0};
105 const double w[] = {-1, 0, 0};
106 const double v[] = {1, 0, 0};
107 const double k[] = {0, 1, 0};
108 const double l[] = {0, 1, 0};
113 const double w[] = {0, 0, 0};
114 const double v[] = {0, 0, 0};
115 const double k[] = {1, 1, -1};
116 const double l[] = {1, 1, 1};
121 const double w[] = {0, 0, 0};
122 const double v[] = {0, 0, 1};
123 const double k[] = {0, 0, 0};
124 const double l[] = {0, 0, 1};
129 const double w[] = {1, 0, 0};
130 const double v[] = {1, 0, 1};
131 const double k[] = {0, 0, 0};
132 const double l[] = {0, 0, 1};
137 const double w[] = {1, -1, 0};
138 const double v[] = {1, 1, 1};
139 const double k[] = {0, 1, 0};
140 const double l[] = {0, -1, 1};
145 const double w[] = {0, 1, 0};
146 const double v[] = {0, 2, 0};
147 const double k[] = {0, 0, 0};
148 const double l[] = {1, 0, 0};
153 const double w[] = {0, 0, 0};
154 const double v[] = {1, 0, 0};
155 const double k[] = {0, 1, 0};
156 const double l[] = {0, 2, 0};
static MoFEMErrorCode Finalize()
Checks for options to be called at the conclusion of the program.
#define CHKERR
Inline error check.
implementation of Data Operators for Forces and Sources
FTensor::Index< 'i', SPACE_DIM > i
const double v
phase velocity of light in medium (cm/ns)
static MoFEMErrorCode Initialize(int *argc, char ***args, const char file[], const char help[])
Initializes the MoFEM database PETSc, MOAB and MPI.
#define CATCH_ERRORS
Catch errors.
int main(int argc, char *argv[])
@ MOFEM_ATOM_TEST_INVALID
FTensor::Index< 'k', 3 > k
#define MoFEMFunctionReturn(a)
Last executable line of each PETSc function used for error handling. Replaces return()
#define MoFEMFunctionBegin
First executable line of each MoFEM function, used for error handling. Final line of MoFEM functions ...
FTensor::Index< 'l', 3 > l