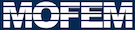 |
| v0.14.0
|
Go to the documentation of this file.
42 static double tetVolume(
const double *coords);
51 const Range &tets,
double &min_quality, Tag
th =
nullptr,
52 boost::function<
double(
double,
double)>
f =
53 [](
double a,
double b) ->
double {
return std::min(
a, b); });
87 template <
int LDB = 1>
123 static inline double shapeFunMBTRI0(
const double x,
const double y);
125 static inline double shapeFunMBTRI1(
const double x,
const double y);
127 static inline double shapeFunMBTRI2(
const double x,
const double y);
142 template <
int LDB = 1>
144 const double *
eta,
const int nb);
281 static inline double shapeFunMBTET0(
const double x,
const double y,
284 static inline double shapeFunMBTET1(
const double x,
const double y,
287 static inline double shapeFunMBTET2(
const double x,
const double y,
290 static inline double shapeFunMBTET3(
const double x,
const double y,
299 N_MBTET0(1. / 3., 1. / 3., 1. / 3.);
301 N_MBTET1(1. / 3., 1. / 3., 1. / 3.);
303 N_MBTET2(1. / 3., 1. / 3., 1. / 3.);
305 N_MBTET3(1. / 3., 1. / 3., 1. / 3.);
321 template <
int LDB = 1>
323 const double *
eta,
const double *
zeta,
363 const double *elem_coords,
const double *glob_coords,
const int nb_nodes,
364 double *local_coords);
389 const double *elem_coords,
const double *glob_coords,
const int nb_nodes,
390 double *local_coords);
394 const double *elem_coords,
const std::complex<double> *glob_coords,
395 const int nb_nodes, std::complex<double> *local_coords);
399 const double *elem_coords,
const double *glob_coords,
const int nb_nodes,
400 double *local_coords) {
402 nb_nodes, local_coords);
426 const double *elem_coords,
const double *glob_coords,
const int nb_nodes,
427 double *local_coords);
440 boost::function<
bool(
double)>
f = [](
double q) ->
bool {
458 const char *file_name,
const char *file_type,
const char *options,
459 const Range &tets, Tag
th =
nullptr,
460 boost::function<
bool(
double)>
f = [](
double q) ->
bool {
477 const double global_coord[],
478 const double tol,
bool &result);
499 double *d_normal =
nullptr);
571 double *
const t_ptr =
nullptr);
590 const double *k_ptr,
const double *l_ptr,
591 double *
const tvw_ptr =
nullptr,
592 double *
const tlk_ptr =
nullptr);
611 double *min_dist_ptr,
double *o_ptr =
nullptr,
632 const int edge1,
const int edge2);
648 std::tuple<std::vector<double>, std::vector<int>, std::vector<int>>;
694 for (
int n = 0;
n != nb; ++
n) {
717 const double *
eta,
const int nb) {
719 for (
int n = 0;
n != nb; ++
n) {
748 const double *
eta,
const double *
zeta,
751 for (
int n = 0;
n != nb; ++
n) {
767 #endif // __TOOLS_HPP__a
#define MoFEMFunctionReturnHot(a)
Last executable line of each PETSc function used for error handling. Replaces return()
PetscErrorCode MoFEMErrorCode
MoFEM/PETSc error code.
UBlasMatrix< double > MatrixDouble
double zeta
Viscous hardening.
implementation of Data Operators for Forces and Sources
base class for all interface classes
static const double edge_coords[6][6]
const double v
phase velocity of light in medium (cm/ns)
const FTensor::Tensor2< T, Dim, Dim > Vec
#define MoFEMFunctionBeginHot
First executable line of each MoFEM function, used for error handling. Final line of MoFEM functions ...
keeps basic data about problem