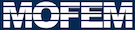 |
| v0.14.0
|
|
| HMHHencky (MoFEM::Interface &m_field, const double E, const double nu) |
|
MoFEMErrorCode | recordTape (const int tag, DTensor2Ptr *t_h) |
|
virtual OpJacobian * | returnOpJacobian (const int tag, const bool eval_rhs, const bool eval_lhs, boost::shared_ptr< DataAtIntegrationPts > data_ptr, boost::shared_ptr< PhysicalEquations > physics_ptr) |
|
virtual VolUserDataOperator * | returnOpSpatialPhysical (const std::string &field_name, boost::shared_ptr< DataAtIntegrationPts > data_ptr, const double alpha_u) |
|
VolUserDataOperator * | returnOpSpatialPhysical_du_du (std::string row_field, std::string col_field, boost::shared_ptr< DataAtIntegrationPts > data_ptr, const double alpha) |
|
VolUserDataOperator * | returnOpCalculateEnergy (boost::shared_ptr< DataAtIntegrationPts > data_ptr, boost::shared_ptr< double > total_energy_ptr) |
|
VolUserDataOperator * | returnOpCalculateStretchFromStress (boost::shared_ptr< DataAtIntegrationPts > data_ptr, boost::shared_ptr< PhysicalEquations > physics_ptr) |
|
VolUserDataOperator * | returnOpCalculateVarStretchFromStress (boost::shared_ptr< DataAtIntegrationPts > data_ptr, boost::shared_ptr< PhysicalEquations > physics_ptr) |
|
MoFEMErrorCode | getOptions (boost::shared_ptr< DataAtIntegrationPts > data_ptr) |
|
MoFEMErrorCode | extractBlockData (Sev sev) |
|
MoFEMErrorCode | extractBlockData (std::vector< const CubitMeshSets * > meshset_vec_ptr, Sev sev) |
|
MoFEMErrorCode | getMatDPtr (boost::shared_ptr< MatrixDouble > mat_D_ptr, boost::shared_ptr< MatrixDouble > mat_axiator_D_ptr, boost::shared_ptr< MatrixDouble > mat_deviator_D_ptr, double bulk_modulus_K, double shear_modulus_G) |
|
MoFEMErrorCode | getInvMatDPtr (boost::shared_ptr< MatrixDouble > mat_inv_D_ptr, double bulk_modulus_K, double shear_modulus_G) |
|
| PhysicalEquations ()=delete |
|
| PhysicalEquations (const int size_active, const int size_dependent) |
|
virtual | ~PhysicalEquations ()=default |
|
virtual VolUserDataOperator * | returnOpSetScale (boost::shared_ptr< double > scale_ptr, boost::shared_ptr< PhysicalEquations > physics_ptr) |
|
DTensor2Ptr | get_P () |
|
DTensor3Ptr | get_P_dh0 () |
|
DTensor3Ptr | get_P_dh1 () |
|
DTensor3Ptr | get_P_dh2 () |
|
DTensor2Ptr | get_h () |
|
|
typedef FTensor::Tensor1< adouble, 3 > | ATensor1 |
|
typedef FTensor::Tensor2< adouble, 3, 3 > | ATensor2 |
|
typedef FTensor::Tensor3< adouble, 3, 3, 3 > | ATensor3 |
|
typedef FTensor::Tensor1< double, 3 > | DTensor1 |
|
typedef FTensor::Tensor2< double, 3, 3 > | DTensor2 |
|
typedef FTensor::Tensor3< double, 3, 3, 3 > | DTensor3 |
|
typedef FTensor::Tensor0< FTensor::PackPtr< double *, 1 > > | DTensor0Ptr |
|
typedef FTensor::Tensor2< FTensor::PackPtr< double *, 1 >, 3, 3 > | DTensor2Ptr |
|
typedef FTensor::Tensor3< FTensor::PackPtr< double *, 1 >, 3, 3, 3 > | DTensor3Ptr |
|
template<int S> |
static DTensor2Ptr | get_VecTensor2 (std::vector< double > &v) |
|
template<int S> |
static DTensor0Ptr | get_VecTensor0 (std::vector< double > &v) |
|
template<int S0> |
static DTensor3Ptr | get_vecTensor3 (std::vector< double > &v, const int nba) |
|
std::vector< double > | activeVariables |
|
std::vector< double > | dependentVariablesPiola |
|
std::vector< double > | dependentVariablesPiolaDirevatives |
|
Definition at line 12 of file HMHHencky.cpp.
◆ HMHHencky()
◆ extractBlockData() [1/2]
MoFEMErrorCode EshelbianPlasticity::HMHHencky::extractBlockData |
( |
Sev |
sev | ) |
|
|
inline |
Definition at line 204 of file HMHHencky.cpp.
209 (boost::format(
"%s(.*)") %
"MAT_ELASTIC").str()
◆ extractBlockData() [2/2]
MoFEMErrorCode EshelbianPlasticity::HMHHencky::extractBlockData |
( |
std::vector< const CubitMeshSets * > |
meshset_vec_ptr, |
|
|
Sev |
sev |
|
) |
| |
|
inline |
Definition at line 217 of file HMHHencky.cpp.
221 for (
auto m : meshset_vec_ptr) {
223 std::vector<double> block_data;
224 CHKERR m->getAttributes(block_data);
225 if (block_data.size() != 2) {
227 "Expected that block has two attribute");
229 auto get_block_ents = [&]() {
◆ getInvMatDPtr()
MoFEMErrorCode EshelbianPlasticity::HMHHencky::getInvMatDPtr |
( |
boost::shared_ptr< MatrixDouble > |
mat_inv_D_ptr, |
|
|
double |
bulk_modulus_K, |
|
|
double |
shear_modulus_G |
|
) |
| |
|
inline |
[Calculate elasticity tensor]
[Calculate elasticity tensor]
Definition at line 283 of file HMHHencky.cpp.
287 auto set_material_compilance = [&]() {
296 auto t_inv_D = getFTensor4DdgFromPtr<SPACE_DIM, SPACE_DIM, 0>(
297 &*(mat_inv_D_ptr->data().begin()));
298 t_inv_D(
i,
j,
k,
l) =
304 set_material_compilance();
◆ getMatDPtr()
MoFEMErrorCode EshelbianPlasticity::HMHHencky::getMatDPtr |
( |
boost::shared_ptr< MatrixDouble > |
mat_D_ptr, |
|
|
boost::shared_ptr< MatrixDouble > |
mat_axiator_D_ptr, |
|
|
boost::shared_ptr< MatrixDouble > |
mat_deviator_D_ptr, |
|
|
double |
bulk_modulus_K, |
|
|
double |
shear_modulus_G |
|
) |
| |
|
inline |
[Calculate elasticity tensor]
[Calculate elasticity tensor]
Definition at line 249 of file HMHHencky.cpp.
255 auto set_material_stiffness = [&]() {
261 auto t_D = getFTensor4DdgFromPtr<SPACE_DIM, SPACE_DIM, 0>(
262 &*(mat_D_ptr->data().begin()));
263 auto t_axiator_D = getFTensor4DdgFromPtr<SPACE_DIM, SPACE_DIM, 0>(
264 &*mat_axiator_D_ptr->data().begin());
265 auto t_deviator_D = getFTensor4DdgFromPtr<SPACE_DIM, SPACE_DIM, 0>(
266 &*mat_deviator_D_ptr->data().begin());
269 t_deviator_D(
i,
j,
k,
l) =
271 t_D(
i,
j,
k,
l) = t_axiator_D(
i,
j,
k,
l) + t_deviator_D(
i,
j,
k,
l);
278 set_material_stiffness();
◆ getOptions()
MoFEMErrorCode EshelbianPlasticity::HMHHencky::getOptions |
( |
boost::shared_ptr< DataAtIntegrationPts > |
data_ptr | ) |
|
|
inline |
Definition at line 186 of file HMHHencky.cpp.
188 CHKERR PetscOptionsBegin(PETSC_COMM_WORLD,
"hencky_",
"",
"none");
190 CHKERR PetscOptionsScalar(
"-young_modulus",
"Young modulus",
"",
E, &
E,
192 CHKERR PetscOptionsScalar(
"-poisson_ratio",
"poisson ratio",
"",
nu, &
nu,
196 MOFEM_LOG(
"WORLD", Sev::verbose) <<
"Hencky: E = " <<
E <<
" nu = " <<
nu;
198 ierr = PetscOptionsEnd();
◆ recordTape()
MoFEMErrorCode EshelbianPlasticity::HMHHencky::recordTape |
( |
const int |
tag, |
|
|
DTensor2Ptr * |
t_h |
|
) |
| |
|
inlinevirtual |
◆ returnOpCalculateEnergy()
◆ returnOpCalculateStretchFromStress()
Reimplemented from EshelbianPlasticity::PhysicalEquations.
Definition at line 169 of file HMHHencky.cpp.
172 return new OpCalculateStretchFromStress(
173 data_ptr, data_ptr->getLogStretchTensorAtPts(),
174 data_ptr->getApproxPAtPts(),
175 boost::dynamic_pointer_cast<HMHHencky>(physics_ptr));
◆ returnOpCalculateVarStretchFromStress()
Reimplemented from EshelbianPlasticity::PhysicalEquations.
Definition at line 178 of file HMHHencky.cpp.
181 return new OpCalculateStretchFromStress(
182 data_ptr, data_ptr->getVarLogStreachPts(), data_ptr->getVarPiolaPts(),
183 boost::dynamic_pointer_cast<HMHHencky>(physics_ptr));
◆ returnOpJacobian()
◆ returnOpSpatialPhysical()
◆ returnOpSpatialPhysical_du_du()
◆ blockData
std::vector<BlockData> EshelbianPlasticity::HMHHencky::blockData |
|
private |
double EshelbianPlasticity::HMHHencky::E |
|
private |
◆ mField
◆ nu
double EshelbianPlasticity::HMHHencky::nu |
|
private |
The documentation for this struct was generated from the following file:
MoFEMErrorCode getInterface(IFACE *&iface) const
Get interface reference to pointer of interface.
constexpr static auto size_symm
#define MOFEM_LOG_CHANNEL(channel)
Set and reset channel.
double young_modulus
Young modulus.
std::vector< BlockData > blockData
#define FTENSOR_INDEX(DIM, I)
#define CHKERR
Inline error check.
virtual moab::Interface & get_moab()=0
double poisson_ratio
Poisson ratio.
MoFEM::Interface & mField
FTensor::Index< 'i', SPACE_DIM > i
MoFEMErrorCode extractBlockData(Sev sev)
constexpr auto field_name
#define MOFEM_TAG_AND_LOG(channel, severity, tag)
Tag and log in channel.
#define MOFEM_LOG(channel, severity)
Log.
FTensor::Index< 'j', 3 > j
static MoFEMErrorCodeGeneric< PetscErrorCode > ierr
@ MOFEM_DATA_INCONSISTENCY
FTensor::Index< 'm', 3 > m
Kronecker Delta class symmetric.
FTensor::Index< 'k', 3 > k
#define MoFEMFunctionReturn(a)
Last executable line of each PETSc function used for error handling. Replaces return()
#define CHKERRG(n)
Check error code of MoFEM/MOAB/PETSc function.
PhysicalEquations()=delete
#define MoFEMFunctionBegin
First executable line of each MoFEM function, used for error handling. Final line of MoFEM functions ...
FTensor::Index< 'l', 3 > l