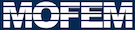 |
| v0.14.0
|
Go to the documentation of this file.
9 #ifndef __ANALYTICALDIRICHLETBC_HPP__
10 #define __ANALYTICALDIRICHLETBC_HPP__
12 using namespace boost::numeric;
13 using namespace MoFEM;
47 MoFEMErrorCode doWork(
int row_side,
int col_side, EntityType row_type,
55 template <
typename FUNEVAL>
64 boost::shared_ptr<FUNEVAL> function_evaluator,
int field_number)
67 functionEvaluator(function_evaluator), fieldNumber(field_number) {}
76 unsigned int nb_row = data.
getIndices().size();
81 unsigned int rank = dof_ptr->getNbOfCoeffs();
83 const auto &gauss_pts = getGaussPts();
84 const auto &coords_at_gauss_pts = getCoordsAtGaussPts();
86 NTf.resize(nb_row / rank);
87 iNdices.resize(nb_row / rank);
89 for (
unsigned int gg = 0; gg < data.
getN().size1(); gg++) {
91 const double area = norm_2(getNormalsAtGaussPts(gg)) * 0.5;
92 const double val = gauss_pts(2, gg) * area;
93 const double x = coords_at_gauss_pts(gg, 0);
94 const double y = coords_at_gauss_pts(gg, 1);
95 const double z = coords_at_gauss_pts(gg, 2);
99 if (
a.size() != rank) {
101 "data inconsistency");
104 for (
unsigned int rr = 0; rr < rank; rr++) {
106 ublas::noalias(iNdices) = ublas::vector_slice<VectorInt>(
108 ublas::slice(rr, rank, data.
getIndices().size() / rank));
110 noalias(NTf) = data.
getN(gg, nb_row / rank) *
a[rr] * val;
112 &iNdices[0], &*NTf.data().begin(), ADD_VALUES);
156 template <
typename FUNEVAL>
159 boost::shared_ptr<FUNEVAL> function_evaluator,
160 const int field_number = 0,
161 const string nodals_positions =
"MESH_NODE_POSITIONS") {
204 string nodals_positions =
"MESH_NODE_POSITIONS");
286 #endif //__ANALYTICALDIRICHLETBC_HPP__
#define MoFEMFunctionReturnHot(a)
Last executable line of each PETSc function used for error handling. Replaces return()
MoFEMErrorCode addHOOpsFace3D(const std::string field, E &e, bool hcurl, bool hdiv)
Data on single entity (This is passed as argument to DataOperator::doWork)
PetscErrorCode MoFEMErrorCode
MoFEM/PETSc error code.
UBlasMatrix< double > MatrixDouble
MyTriFE(MoFEM::Interface &m_field)
boost::shared_ptr< Range > trisPtr
Set Dirichlet boundary conditions on displacements.
MoFEMErrorCode VecSetValues(Vec V, const EntitiesFieldData::EntData &data, const double *ptr, InsertMode iora)
Assemble PETSc vector.
Deprecated interface functions.
#define CHKERR
Inline error check.
OpRhs(const std::string field_name, boost::shared_ptr< FUNEVAL > function_evaluator, int field_number)
implementation of Data Operators for Forces and Sources
Structure used to enforce analytical boundary conditions.
default operator for TRI element
MoFEMErrorCode setApproxOps(MoFEM::Interface &m_field, const std::string field_name, boost::shared_ptr< FUNEVAL > function_evaluator, const int field_number=0, const string nodals_positions="MESH_NODE_POSITIONS")
Set operators used to calculate the rhs vector and the lhs matrix.
const VectorInt & getIndices() const
Get global indices of dofs on entity.
virtual bool check_field(const std::string &name) const =0
check if field is in database
const VectorDofs & getFieldDofs() const
get dofs data stature FEDofEntity
constexpr auto field_name
MoFEMErrorCode doWork(int side, EntityType type, EntitiesFieldData::EntData &data)
Operator for linear form, usually to calculate values on right hand side.
structure to get information form mofem into EntitiesFieldData
finite element to approximate analytical solution on surface
MatrixDouble & getN(const FieldApproximationBase base)
get base functions this return matrix (nb. of rows is equal to nb. of Gauss pts, nb....
UBlasVector< int > VectorInt
boost::ptr_deque< UserDataOperator > & getOpPtrVector()
Use to push back operator for row operator.
const FTensor::Tensor2< T, Dim, Dim > Vec
@ MOFEM_DATA_INCONSISTENCY
boost::shared_ptr< FUNEVAL > functionEvaluator
Rhs operator used to build matrix.
ApproxField(MoFEM::Interface &m_field)
Lhs operator used to build matrix.
UBlasVector< double > VectorDouble
Analytical Dirichlet boundary conditions.
const double D
diffusivity
#define MoFEMFunctionBeginHot
First executable line of each MoFEM function, used for error handling. Final line of MoFEM functions ...
#define MoFEMFunctionReturn(a)
Last executable line of each PETSc function used for error handling. Replaces return()
#define MoFEMFunctionBegin
First executable line of each MoFEM function, used for error handling. Final line of MoFEM functions ...
MyTriFE & getLoopFeApprox()