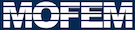 |
| v0.14.0
|
Go to the documentation of this file.
13 #ifndef __THERMAL_ELEMENT_HPP
14 #define __THERMAL_ELEMENT_HPP
99 std::map<int, BlockData>
110 std::map<int, FluxData>
123 std::map<int, ConvectionData>
138 std::map<int, RadiationData>
176 template <
typename OP>
195 if (data.getFieldData().size() == 0)
197 int nb_dofs = data.getFieldData().size();
198 int nb_gauss_pts = data.getN().size1();
202 if (
type == MBVERTEX) {
209 for (
int gg = 0; gg < nb_gauss_pts; gg++) {
211 inner_prod(data.getN(gg, nb_dofs), data.getFieldData());
214 }
catch (
const std::exception &ex) {
215 std::ostringstream ss;
216 ss <<
"throw in method: " << ex.what() << std::endl;
232 field_name, common_data.temperatureAtGaussPts) {}
243 field_name, common_data.temperatureAtGaussPts) {}
254 field_name, common_data.temperatureRateAtGaussPts) {}
387 bool ho_geometry =
false)
394 bool ho_geometry =
false)
424 CommonData &common_data,
bool ho_geometry =
false)
432 CommonData &common_data,
bool ho_geometry =
false)
464 CommonData &common_data,
bool ho_geometry =
false)
472 CommonData &common_data,
bool ho_geometry =
false)
501 CommonData &common_data,
bool ho_geometry =
false)
509 CommonData &common_data,
bool ho_geometry =
false)
534 bool ho_geometry =
false)
541 bool ho_geometry =
false)
569 const std::string rate_name)
586 using Ele = ForcesAndSourcesCore;
587 using VolEle = VolumeElementForcesAndSourcesCore;
597 const std::string temp_name,
598 std::vector<std::array<double, 3>> eval_points = {})
608 CHKERRABORT(PETSC_COMM_WORLD,
ierr);
610 auto no_rule = [](
int,
int,
int) {
return -1; };
612 tempPtr = boost::make_shared<VectorDouble>();
614 boost::shared_ptr<Ele> vol_ele(
dataFieldEval->feMethodPtr.lock());
615 vol_ele->getRuleHook = no_rule;
617 vol_ele->getOpPtrVector().push_back(
618 new OpCalculateScalarFieldValues(
"TEMP",
tempPtr));
636 const std::string mesh_nodals_positions =
"MESH_NODE_POSITIONS");
650 const std::string mesh_nodals_positions =
"MESH_NODE_POSITIONS");
664 const std::string mesh_nodals_positions =
"MESH_NODE_POSITIONS");
678 const std::string mesh_nodals_positions =
"MESH_NODE_POSITIONS");
695 const std::string mesh_nodals_positions =
"MESH_NODE_POSITIONS");
701 const std::string mesh_nodals_positions =
"MESH_NODE_POSITIONS");
707 const std::string mesh_nodals_positions =
"MESH_NODE_POSITIONS");
714 const std::string mesh_nodals_positions =
"MESH_NODE_POSITIONS");
721 const std::string mesh_nodals_positions =
"MESH_NODE_POSITIONS");
724 #endif //__THERMAL_ELEMENT_HPP
#define MoFEMFunctionReturnHot(a)
Last executable line of each PETSc function used for error handling. Replaces return()
MoFEMErrorCode getInterface(IFACE *&iface) const
Get interface refernce to pointer of interface.
OpGetTetTemperatureAtGaussPts(const std::string field_name, CommonData &common_data)
OpRadiationRhs(const std::string field_name, RadiationData &data, CommonData &common_data, bool ho_geometry=false)
data for calculation heat flux
MyTriFE & getLoopFeRadiationLhs()
operator to calculate right hand side of heat capacity terms
MoFEM::Interface & mField
operator to calculate radiation therms on body surface and assemble to rhs of transient equations(Res...
@ MOFEM_STD_EXCEPTION_THROW
std::map< int, BlockData > setOfBlocks
maps block set id with appropriate BlockData
std::map< int, ConvectionData > setOfConvection
MoFEMErrorCode doWork(int side, EntityType type, EntitiesFieldData::EntData &data)
calculate thermal conductivity matrix
OpThermalRhs(const std::string field_name, BlockData &data, CommonData &common_data)
MyTriFE & getLoopFeRadiationRhs()
std::vector< std::array< double, 3 > > evalPoints
MoFEM::Interface & mField
MoFEMErrorCode addThermalElements(const std::string field_name, const std::string mesh_nodals_positions="MESH_NODE_POSITIONS")
add thermal element on tets
PetscErrorCode MoFEMErrorCode
MoFEM/PETSc error code.
MoFEMErrorCode preProcess()
UBlasMatrix< double > MatrixDouble
MoFEMErrorCode addThermalConvectionElement(const std::string field_name, const std::string mesh_nodals_positions="MESH_NODE_POSITIONS")
add convection element
MoFEMErrorCode doWork(int row_side, int col_side, EntityType row_type, EntityType col_type, EntitiesFieldData::EntData &row_data, EntitiesFieldData::EntData &col_data)
calculate thermal conductivity matrix
MatrixDouble cOnductivity_mat
operator for calculate heat flux and assemble to right hand side
OpThermalLhs(const std::string field_name, Mat _A, BlockData &data, CommonData &common_data)
boost::shared_ptr< VectorDouble > tempPtr
TimeSeriesMonitor(MoFEM::Interface &m_field, const std::string series_name, const std::string temp_name, std::vector< std::array< double, 3 >> eval_points={})
ublas::matrix_row< MatrixDouble > getGradAtGaussPts(const int gg)
MyVolumeFE feRhs
cauclate right hand side for tetrahedral elements
OpConvectionRhs(const std::string field_name, Vec _F, ConvectionData &data, CommonData &common_data, bool ho_geometry=false)
MoFEMErrorCode doWork(int row_side, int col_side, EntityType row_type, EntityType col_type, EntitiesFieldData::EntData &row_data, EntitiesFieldData::EntData &col_data)
calculate thermal convection term in the lhs of equations
OpGetGradAtGaussPts(const std::string field_name, CommonData &common_data)
operator to calculate temperature gradient at Gauss points
@ OPROWCOL
operator doWork is executed on FE rows &columns
OpGetTetRateAtGaussPts(const std::string field_name, CommonData &common_data)
Deprecated interface functions.
MyVolumeFE(MoFEM::Interface &m_field)
MyTriFE & getLoopFeFlux()
FieldEvaluatorInterface::SetPtsData SetPtsData
const std::string seriesName
OpConvectionLhs(const std::string field_name, Mat _A, ConvectionData &data, bool ho_geometry=false)
OpThermalLhs(const std::string field_name, BlockData &data, CommonData &common_data)
VolumeElementForcesAndSourcesCore(Interface &m_field, const EntityType type=MBTET)
MyTriFE & getLoopFeConvectionLhs()
MoFEMErrorCode doWork(int side, EntityType type, EntitiesFieldData::EntData &data)
operator calculating temperature and rate of temperature
VolumeElementForcesAndSourcesCore VolEle
MoFEMErrorCode setThermalFluxFiniteElementRhsOperators(string field_name, Vec &F, const std::string mesh_nodals_positions="MESH_NODE_POSITIONS")
this function is used in case of stationary problem for heat flux terms
implementation of Data Operators for Forces and Sources
data for calculation heat conductivity and heat capacity elements
MyTriFE(MoFEM::Interface &m_field)
boost::shared_ptr< SetPtsData > dataFieldEval
default operator for TRI element
OpRadiationRhs(const std::string field_name, Vec _F, RadiationData &data, CommonData &common_data, bool ho_geometry=false)
OpRadiationLhs(const std::string field_name, RadiationData &data, CommonData &common_data, bool ho_geometry=false)
OpHeatFlux(const std::string field_name, Vec _F, FluxData &data, bool ho_geometry=false)
OpGetFieldAtGaussPts(const std::string field_name, VectorDouble &field_at_gauss_pts)
common data used by volume elements
definition of volume element
operator to calculate convection therms on body surface and assemble to rhs of equations
MoFEMErrorCode doWork(int side, EntityType type, EntitiesFieldData::EntData &data)
MoFEMErrorCode setTimeSteppingProblem(string field_name, string rate_name, const std::string mesh_nodals_positions="MESH_NODE_POSITIONS")
set up operators for unsteady heat flux; convection; radiation problem
MoFEMErrorCode doWork(int row_side, int col_side, EntityType row_type, EntityType col_type, EntitiesFieldData::EntData &row_data, EntitiesFieldData::EntData &col_data)
calculate thermal radiation term in the lhs of equations(Tangent Matrix) for transient Thermal Proble...
MoFEMErrorCode doWork(int side, EntityType type, EntitiesFieldData::EntData &data)
calculate thermal conductivity matrix
MoFEM::Interface & mField
FieldEvaluatorInterface::SetPtsData SetPtsData
OpHeatCapacityRhs(const std::string field_name, BlockData &data, CommonData &common_data)
OpConvectionRhs(const std::string field_name, ConvectionData &data, CommonData &common_data, bool ho_geometry=false)
OpGetTriTemperatureAtGaussPts(const std::string field_name, CommonData &common_data)
MyTriFE & getLoopFeConvectionRhs()
MoFEMErrorCode addThermalFluxElement(const std::string field_name, const std::string mesh_nodals_positions="MESH_NODE_POSITIONS")
add heat flux element
operator to calculate temperature rate at Gauss pts
MoFEMErrorCode setThermalFiniteElementRhsOperators(string field_name, Vec &F)
this function is used in case of stationary problem to set elements for rhs
Range tEts
contains elements in block set
VectorDouble & fieldAtGaussPts
Volume finite element base.
OpConvectionLhs(const std::string field_name, ConvectionData &data, bool ho_geometry=false)
ThermalElement(MoFEM::Interface &m_field)
MoFEMErrorCode doWork(int side, EntityType type, EntitiesFieldData::EntData &data)
operator calculating temperature gradients
TS monitore it records temperature at time steps.
EntitiesFieldData::EntData EntData
constexpr auto field_name
OpHeatFlux(const std::string field_name, FluxData &data, bool ho_geometry=false)
std::map< int, FluxData > setOfFluxes
maps side set id with appropriate FluxData
const std::string tempName
this calass is to control time stepping
VectorDouble temperatureAtGaussPts
MoFEMErrorCode postProcess()
operator to calculate temperature at Gauss pts
double initTemp
initial temperature
structure grouping operators and data used for thermal problems
std::map< int, RadiationData > setOfRadiation
operator to calculate temperature and rate of temperature at Gauss points
Range tRis
surface triangles where hate flux is applied
MoFEMErrorCode setThermalConvectionFiniteElementLhsOperators(string field_name, Mat A, const std::string mesh_nodals_positions="MESH_NODE_POSITIONS")
ForcesAndSourcesCore::UserDataOperator UserDataOperator
OpRadiationLhs(const std::string field_name, Mat _A, RadiationData &data, CommonData &common_data, bool ho_geometry=false)
OpThermalRhs(const std::string field_name, Vec _F, BlockData &data, CommonData &common_data)
VectorDouble temperatureRateAtGaussPts
int getRule(int order)
it is used to calculate nb. of Gauss integration points
static MoFEMErrorCodeGeneric< PetscErrorCode > ierr
MoFEMErrorCode addThermalRadiationElement(const std::string field_name, const std::string mesh_nodals_positions="MESH_NODE_POSITIONS")
add Non-linear Radiation element
operator to calculate temperature at Gauss pts
const FTensor::Tensor2< T, Dim, Dim > Vec
MoFEMErrorCode doWork(int side, EntityType type, EntitiesFieldData::EntData &data)
calculate Transient Radiation condition on the right hand side residual
const std::string rateName
UBlasVector< double > VectorDouble
std::bitset< BITREFLEVEL_SIZE > BitRefLevel
Bit structure attached to each entity identifying to what mesh entity is attached.
const std::string tempName
MoFEMErrorCode postProcess()
MoFEMErrorCode setThermalConvectionFiniteElementRhsOperators(string field_name, Vec &F, const std::string mesh_nodals_positions="MESH_NODE_POSITIONS")
#define MoFEMFunctionBeginHot
First executable line of each MoFEM function, used for error handling. Final line of MoFEM functions ...
MatrixDouble gradAtGaussPts
MoFEMErrorCode setThermalFiniteElementLhsOperators(string field_name, Mat A)
this function is used in case of stationary heat conductivity problem for lhs
MoFEMErrorCode doWork(int side, EntityType type, EntitiesFieldData::EntData &data)
calculate heat flux
MoFEMErrorCode doWork(int row_side, int col_side, EntityType row_type, EntityType col_type, EntitiesFieldData::EntData &row_data, EntitiesFieldData::EntData &col_data)
calculate heat capacity matrix
UpdateAndControl(MoFEM::Interface &m_field, const std::string temp_name, const std::string rate_name)
OpHeatCapacityLhs(const std::string field_name, BlockData &data, CommonData &common_data)
@ OPROW
operator doWork function is executed on FE rows
operator to calculate left hand side of heat capacity terms