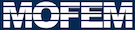 |
| v0.14.0
|
Go to the documentation of this file.
9 #ifndef __VECMANAGER_HPP__
10 #define __VECMANAGER_HPP__
81 const std::string x_field_name,
83 const std::string y_problem,
84 const std::string y_field_name,
92 const std::string y_problem,
const std::string y_field_name,
110 VecScatter *newctx)
const;
136 Vec V, InsertMode mode,
137 ScatterMode scatter_mode)
const;
156 Vec V, InsertMode mode,
157 ScatterMode scatter_mode)
const;
176 Vec V, InsertMode mode,
177 ScatterMode scatter_mode)
const;
196 Vec V, InsertMode mode,
197 ScatterMode scatter_mode)
const;
217 const std::string cpy_field_name,
219 ScatterMode scatter_mode)
const;
239 const std::string cpy_field_name,
241 ScatterMode scatter_mode)
const;
263 const std::string cpy_field_name,
266 ScatterMode scatter_mode)
const;
289 const std::string cpy_field_name,
292 ScatterMode scatter_mode)
const;
297 #endif //__VECMANAGER_HPP__
MoFEMErrorCode vecCreateSeq(const std::string name, RowColData rc, Vec *V) const
create local vector for problem
MoFEMErrorCode setOtherLocalGhostVector(const Problem *problem_ptr, const std::string field_name, const std::string cpy_field_name, RowColData rc, Vec V, InsertMode mode, ScatterMode scatter_mode) const
Copy vector to field which is not part of the problem.
PetscErrorCode MoFEMErrorCode
MoFEM/PETSc error code.
MoFEMErrorCode setOtherGlobalGhostVector(const Problem *problem_ptr, const std::string field_name, const std::string cpy_field_name, RowColData rc, Vec V, InsertMode mode, ScatterMode scatter_mode) const
Copy vector to field which is not part of the problem (collective)
MoFEMErrorCode vecCreateGhost(const std::string name, RowColData rc, Vec *V) const
create ghost vector for problem (collective)
Deprecated interface functions.
implementation of Data Operators for Forces and Sources
const MoFEM::Interface & cOre
MoFEMErrorCode vecScatterCreate(Vec xin, const std::string x_problem, const std::string x_field_name, RowColData x_rc, Vec yin, const std::string y_problem, const std::string y_field_name, RowColData y_rc, VecScatter *newctx) const
create scatter for vectors form one to another problem (collective)
Vector manager is used to create vectors \mofem_vectors.
constexpr auto field_name
MoFEMErrorCode setLocalGhostVector(const Problem *problem_ptr, RowColData rc, Vec V, InsertMode mode, ScatterMode scatter_mode) const
set values of vector from/to mesh database
base class for all interface classes
MoFEMErrorCode setGlobalGhostVector(const Problem *problem_ptr, RowColData rc, Vec V, InsertMode mode, ScatterMode scatter_mode) const
set values of vector from/to mesh database (collective)
const FTensor::Tensor2< T, Dim, Dim > Vec
VecManager(const MoFEM::Core &core)
keeps basic data about problem
MoFEMErrorCode query_interface(boost::typeindex::type_index type_index, UnknownInterface **iface) const