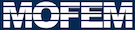 |
| v0.14.0
|
Go to the documentation of this file.
10 using namespace MoFEM;
14 static char help[] =
"-my_file mesh file name\n"
15 "-my_order default approximation order\n"
16 "-my_is_partitioned set if mesh is partitioned\n"
17 "-regression_test check solution vector against expected value\n"
20 int main(
int argc,
char *argv[]) {
22 const string default_options =
"-ksp_type fgmres \n"
24 "-pc_factor_mat_solver_type mumps \n"
25 "-mat_mumps_icntl_20 0 \n"
26 "-mat_mumps_icntl_13 1 \n"
28 "-mat_mumps_icntl_24 1 \n"
29 "-mat_mumps_icntl_13 1 \n";
31 string param_file =
"param_file.petsc";
32 if (!
static_cast<bool>(ifstream(param_file))) {
33 std::ofstream file(param_file.c_str(), std::ios::ate);
35 file << default_options;
47 ParallelComm *pcomm = ParallelComm::get_pcomm(&moab,
MYPCOMM_INDEX);
49 boost::make_shared<WrapMPIComm>(PETSC_COMM_WORLD,
false);
52 new ParallelComm(&moab, moab_comm_wrap->get_comm());
58 PetscBool regression_test = PETSC_FALSE;
60 CHKERR PetscOptionsBegin(PETSC_COMM_WORLD,
"",
"Magnetostatics options",
62 CHKERR PetscOptionsString(
"-my_file",
"mesh file name",
"",
"mesh.h5m",
64 CHKERR PetscOptionsInt(
"-my_order",
"default approximation order",
"",
68 "if set norm of solution vector is check agains expected value ",
70 PETSC_FALSE, ®ression_test, PETSC_NULL);
72 ierr = PetscOptionsEnd();
77 option =
"PARALLEL=READ_PART;"
78 "PARALLEL_RESOLVE_SHARED_ENTS;"
79 "PARTITION=PARALLEL_PARTITION;";
98 CHKERR moab.write_file(
"solution.h5m",
"MOAB",
"PARALLEL=WRITE_PART");
#define MYPCOMM_INDEX
default communicator number PCOMM
MoFEMErrorCode destroyProblem()
destroy Distributed mesh manager
static MoFEMErrorCode Finalize()
Checks for options to be called at the conclusion of the program.
Deprecated interface functions.
DeprecatedCoreInterface Interface
#define CHKERR
Inline error check.
MoFEMErrorCode getEssentialBc()
get essential boundary conditions (only homogenous case is considered)
MoFEMErrorCode createFields()
build problem data structures
implementation of Data Operators for Forces and Sources
MoFEMErrorCode postProcessResults()
post-process results, i.e. save solution on the mesh
MoFEMErrorCode createProblem()
create problem
static MoFEMErrorCode Initialize(int *argc, char ***args, const char file[], const char help[])
Initializes the MoFEM database PETSc, MOAB and MPI.
#define CATCH_ERRORS
Catch errors.
static MoFEMErrorCodeGeneric< PetscErrorCode > ierr
Implementation of magnetic element.
MoFEMErrorCode getNaturalBc()
get natural boundary conditions
int main(int argc, char *argv[])
MoFEMErrorCode createElements()
create finite elements
Implementation of magneto-static problem (basic Implementation)
#define CHKERRG(n)
Check error code of MoFEM/MOAB/PETSc function.
MoFEMErrorCode solveProblem(const bool regression_test=false)
solve problem