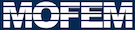 |
| v0.14.0
|
Implementation of magneto-static problem (basic Implementation)
More...
#include <users_modules/tutorials/max-0/src/MagneticElement.hpp>
Implementation of magneto-static problem (basic Implementation)
Look for theory and details here:
[35] <www.hpfem.jku.at/publications/szthesis.pdf> https://pdfs.semanticscholar.org/0574/e5763d9b64bff16f908e9621f23af3b3dc86.pdf
Election file and all other problem related file are here maxwell_element.
- Todo:
Extension for mix formulation
Use appropriate pre-conditioner for large problems
- Examples
- MagneticElement.hpp, and magnetostatic.cpp.
Definition at line 28 of file MagneticElement.hpp.
◆ MagneticElement()
◆ ~MagneticElement()
virtual MagneticElement::~MagneticElement |
( |
| ) |
|
|
virtualdefault |
◆ createElements()
MoFEMErrorCode MagneticElement::createElements |
( |
| ) |
|
|
inline |
◆ createFields()
MoFEMErrorCode MagneticElement::createFields |
( |
| ) |
|
|
inline |
◆ createProblem()
MoFEMErrorCode MagneticElement::createProblem |
( |
| ) |
|
|
inline |
create problem
Problem is collection of finite elements. With the information on which fields finite elements operates the matrix and left and right hand side vector could be created.
Here we use Distributed mesh manager from PETSc as a inteface.
- Returns
- error code
- Examples
- MagneticElement.hpp, and magnetostatic.cpp.
Definition at line 278 of file MagneticElement.hpp.
◆ destroyProblem()
MoFEMErrorCode MagneticElement::destroyProblem |
( |
| ) |
|
|
inline |
◆ getEssentialBc()
MoFEMErrorCode MagneticElement::getEssentialBc |
( |
| ) |
|
|
inline |
get essential boundary conditions (only homogenous case is considered)
- Returns
- error code
- Examples
- MagneticElement.hpp, and magnetostatic.cpp.
Definition at line 121 of file MagneticElement.hpp.
122 ParallelComm *pcomm =
126 if (
bit->getName().compare(0, 10,
"ESSENTIALBC") == 0) {
140 CHKERR skin.find_skin(0, tets,
false, skin_faces);
143 CHKERR pcomm->filter_pstatus(skin_faces,
144 PSTATUS_SHARED | PSTATUS_MULTISHARED,
145 PSTATUS_NOT, -1, &proc_skin);
◆ getNaturalBc()
MoFEMErrorCode MagneticElement::getNaturalBc |
( |
| ) |
|
|
inline |
◆ postProcessResults()
MoFEMErrorCode MagneticElement::postProcessResults |
( |
| ) |
|
|
inline |
post-process results, i.e. save solution on the mesh
- Returns
- [description]
- Examples
- MagneticElement.hpp, and magnetostatic.cpp.
Definition at line 406 of file MagneticElement.hpp.
409 PostProcBrokenMeshInMoab<VolumeElementForcesAndSourcesCore> post_proc(
415 auto pos_ptr = boost::make_shared<MatrixDouble>();
416 auto field_val_ptr = boost::make_shared<MatrixDouble>();
418 post_proc.getOpPtrVector().push_back(
419 new OpCalculateVectorFieldValues<3>(
"MESH_NODE_POSITIONS", pos_ptr));
420 post_proc.getOpPtrVector().push_back(
423 using OpPPMap = OpPostProcMapInMoab<3, 3>;
425 post_proc.getOpPtrVector().push_back(
429 post_proc.getPostProcMesh(), post_proc.getMapGaussPts(),
433 {{
"MESH_NODE_POSITIONS", pos_ptr},
434 {blockData.fieldName, field_val_ptr}},
444 post_proc.getOpPtrVector().push_back(
new OpPostProcessCurl(
445 blockData, post_proc.getPostProcMesh(), post_proc.getMapGaussPts()));
448 CHKERR post_proc.writeFile(
"out_values.h5m");
◆ solveProblem()
MoFEMErrorCode MagneticElement::solveProblem |
( |
const bool |
regression_test = false | ) |
|
|
inline |
solve problem
Create matrices; integrate over elements; solve linear system of equations
- Examples
- MagneticElement.hpp, and magnetostatic.cpp.
Definition at line 319 of file MagneticElement.hpp.
323 auto material_grad_mat = boost::make_shared<MatrixDouble>();
324 auto material_det_vec = boost::make_shared<VectorDouble>();
325 auto material_inv_grad_mat = boost::make_shared<MatrixDouble>();
327 vol_fe.getOpPtrVector().push_back(
new OpCurlCurl(
blockData));
328 vol_fe.getOpPtrVector().push_back(
new OpStab(
blockData));
330 tri_fe.meshPositionsFieldName =
"none";
332 tri_fe.getOpPtrVector().push_back(
333 new OpGetHONormalsOnFace(
"MESH_NODE_POSITIONS"));
334 tri_fe.getOpPtrVector().push_back(
335 new OpCalculateHOCoords(
"MESH_NODE_POSITIONS"));
336 tri_fe.getOpPtrVector().push_back(
337 new OpHOSetCovariantPiolaTransformOnFace3D(
HCURL));
338 tri_fe.getOpPtrVector().push_back(
new OpNaturalBC(
blockData));
349 ->createMPIAIJ<PetscGlobalIdx_mi_tag>(problem_ptr->
getName(),
370 CHKERR KSPCreate(PETSC_COMM_WORLD, &solver);
372 CHKERR KSPSetFromOptions(solver);
375 CHKERR KSPDestroy(&solver);
382 if (regression_test) {
386 const double expected_value = 4.6772e+01;
387 const double tol = 1e-4;
388 if ((nrm2 - expected_value) / expected_value >
tol) {
390 "Regression test failed %6.4e != %6.4e", nrm2, expected_value);
◆ blockData
◆ mField
The documentation for this struct was generated from the following file:
MoFEMErrorCode getInterface(IFACE *&iface) const
Get interface refernce to pointer of interface.
#define MYPCOMM_INDEX
default communicator number PCOMM
virtual MoFEMErrorCode loop_dofs(const Problem *problem_ptr, const std::string &field_name, RowColData rc, DofMethod &method, int lower_rank, int upper_rank, int verb=DEFAULT_VERBOSITY)=0
Make a loop over dofs.
MoFEMErrorCode addHOOpsVol(const std::string field, E &e, bool h1, bool hcurl, bool hdiv, bool l2)
virtual MoFEMErrorCode modify_finite_element_add_field_row(const std::string &fe_name, const std::string name_row)=0
set field row which finite element use
int oRder
approximation order
PetscErrorCode DMoFEMMeshToLocalVector(DM dm, Vec l, InsertMode mode, ScatterMode scatter_mode)
set local (or ghosted) vector values on mesh for partition only
virtual MoFEMErrorCode add_ents_to_field_by_type(const Range &ents, const EntityType type, const std::string &name, int verb=DEFAULT_VERBOSITY)=0
Add entities to field meshset.
PetscErrorCode DMMoFEMAddElement(DM dm, std::string fe_name)
add element to dm
virtual MoFEMErrorCode add_ents_to_finite_element_by_type(const EntityHandle entities, const EntityType type, const std::string &name, const bool recursive=true)=0
add entities to finite element
#define CHKERR
Inline error check.
virtual MoFEMErrorCode add_finite_element(const std::string &fe_name, enum MoFEMTypes bh=MF_EXCL, int verb=DEFAULT_VERBOSITY)=0
add finite element
virtual moab::Interface & get_moab()=0
virtual MoFEMErrorCode modify_finite_element_add_field_col(const std::string &fe_name, const std::string name_row)=0
set field col which finite element use
virtual MoFEMErrorCode build_finite_elements(int verb=DEFAULT_VERBOSITY)=0
Build finite elements.
virtual MoFEMErrorCode add_field(const std::string &name, const FieldSpace space, const FieldApproximationBase base, const FieldCoefficientsNumber nb_of_coefficients, const TagType tag_type=MB_TAG_SPARSE, const enum MoFEMTypes bh=MF_EXCL, int verb=DEFAULT_VERBOSITY)=0
Add field.
OpPostProcMapInMoab< SPACE_DIM, SPACE_DIM > OpPPMap
PetscErrorCode DMRegister_MoFEM(const char sname[])
Register MoFEM problem.
const string feNaturalBCName
PetscErrorCode DMMoFEMCreateMoFEM(DM dm, MoFEM::Interface *m_field_ptr, const char problem_name[], const MoFEM::BitRefLevel bit_level, const MoFEM::BitRefLevel bit_mask=MoFEM::BitRefLevel().set())
Must be called by user to set MoFEM data structures.
MoFEM::Interface & mField
#define _IT_CUBITMESHSETS_BY_SET_TYPE_FOR_LOOP_(MESHSET_MANAGER, CUBITBCTYPE, IT)
Iterator that loops over a specific Cubit MeshSet having a particular BC meshset in a moFEM field.
@ AINSWORTH_LEGENDRE_BASE
Ainsworth Cole (Legendre) approx. base .
@ HCURL
field with continuous tangents
@ MOFEM_DATA_INCONSISTENCY
PetscErrorCode DMMoFEMGetProblemPtr(DM dm, const MoFEM::Problem **problem_ptr)
Get pointer to problem data structure.
virtual MoFEMErrorCode build_fields(int verb=DEFAULT_VERBOSITY)=0
virtual MoFEMErrorCode modify_finite_element_add_field_data(const std::string &fe_name, const std::string name_filed)=0
set finite element field data
std::bitset< BITREFLEVEL_SIZE > BitRefLevel
Bit structure attached to each entity identifying to what mesh entity is attached.
virtual MoFEMErrorCode build_adjacencies(const Range &ents, int verb=DEFAULT_VERBOSITY)=0
build adjacencies
keeps basic data about problem
virtual MoFEMErrorCode set_field_order(const EntityHandle meshset, const EntityType type, const std::string &name, const ApproximationOrder order, int verb=DEFAULT_VERBOSITY)=0
Set order approximation of the entities in the field.
PetscErrorCode DMoFEMLoopFiniteElements(DM dm, const char fe_name[], MoFEM::FEMethod *method, CacheTupleWeakPtr cache_ptr=CacheTupleSharedPtr())
Executes FEMethod for finite elements in DM.
#define MoFEMFunctionReturn(a)
Last executable line of each PETSc function used for error handling. Replaces return()
#define MoFEMFunctionBegin
First executable line of each MoFEM function, used for error handling. Final line of MoFEM functions ...
PetscErrorCode DMMoFEMSetIsPartitioned(DM dm, PetscBool is_partitioned)
Post post-proc data at points from hash maps.