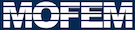 |
| v0.14.0
|
Go to the documentation of this file. 1 #ifndef __NONLINEARPOISSON2D_HPP__
2 #define __NONLINEARPOISSON2D_HPP__
18 PETSC>::LinearForm<GAUSS>::OpBaseTimesScalar<1>;
20 PETSC>::LinearForm<GAUSS>::OpSource<1, 1>;
35 typedef boost::function<
double(
const double,
const double,
const double)>
42 std::string row_field_name, std::string col_field_name,
43 boost::shared_ptr<VectorDouble> field_vec,
44 boost::shared_ptr<MatrixDouble> field_grad_mat)
47 fieldVec(field_vec), fieldGradMat(field_grad_mat) {}
55 const int nb_row_dofs = row_data.
getIndices().size();
56 const int nb_col_dofs = col_data.
getIndices().size();
58 const double area = getMeasure();
61 const int nb_integration_points = getGaussPts().size2();
63 auto t_w = getFTensor0IntegrationWeight();
67 auto t_field_grad = getFTensor1FromMat<2>(*fieldGradMat);
75 for (
int gg = 0; gg != nb_integration_points; gg++) {
76 const double a = t_w * area;
78 for (
int rr = 0; rr != nb_row_dofs; ++rr) {
84 for (
int cc = 0; cc != nb_col_dofs; cc++) {
85 locLhs(rr, cc) += (((1 + t_field * t_field) * t_row_diff_base(
i) *
87 (2.0 * t_field * t_field_grad(
i) *
88 t_row_diff_base(
i) * t_col_base)) *
123 boost::shared_ptr<VectorDouble> field_vec,
124 boost::shared_ptr<MatrixDouble> field_grad_mat)
126 sourceTermFunc(source_term_function), fieldVec(field_vec),
127 fieldGradMat(field_grad_mat) {}
136 const double area = getMeasure();
139 const int nb_integration_points = getGaussPts().size2();
141 auto t_w = getFTensor0IntegrationWeight();
143 auto t_coords = getFTensor1CoordsAtGaussPts();
147 auto t_field_grad = getFTensor1FromMat<2>(*fieldGradMat);
155 for (
int gg = 0; gg != nb_integration_points; gg++) {
156 const double a = t_w * area;
158 sourceTermFunc(t_coords(0), t_coords(1), t_coords(2));
163 (-t_base * body_source +
164 t_grad_base(
i) * t_field_grad(
i) * (1 + t_field * t_field)) *
196 #endif //__NONLINEARPOISSON2D_HPP__
Data on single entity (This is passed as argument to DataOperator::doWork)
ElementsAndOps< SPACE_DIM >::BoundaryEle BoundaryEle
MatrixDouble locMat
local entity block matrix
FormsIntegrators< BoundaryEleOp >::Assembly< PETSC >::LinearForm< GAUSS >::OpBaseTimesScalar< 1 > OpBoundaryRhs
PetscErrorCode MoFEMErrorCode
MoFEM/PETSc error code.
boost::shared_ptr< MatrixDouble > fieldGradMat
OpBaseImpl< PETSC, EdgeEleOp > OpBase
FTensor::Tensor0< FTensor::PackPtr< double *, 1 > > getFTensor0N(const FieldApproximationBase base)
Get base function as Tensor0.
MoFEM::EdgeElementForcesAndSourcesCore EdgeEle
VectorDouble locF
local entity vector
MoFEMErrorCode iNtegrate(EntData &data)
Class dedicated to integrate operator.
MoFEMErrorCode iNtegrate(EntData &row_data, EntData &col_data)
Integrate grad-grad operator.
implementation of Data Operators for Forces and Sources
FormsIntegrators< BoundaryEleOp >::Assembly< PETSC >::LinearForm< GAUSS >::OpSource< 1, 1 > OpBoundaryRhsSource
MoFEM::FaceElementForcesAndSourcesCore FaceEle
static auto getFTensor0FromVec(ublas::vector< T, A > &data)
Get tensor rank 0 (scalar) form data vector.
OpDomainLhs(std::string row_field_name, std::string col_field_name, boost::shared_ptr< VectorDouble > field_vec, boost::shared_ptr< MatrixDouble > field_grad_mat)
FTensor::Index< 'i', 2 > i
const VectorInt & getIndices() const
Get global indices of dofs on entity.
boost::shared_ptr< VectorDouble > fieldVec
OpDomainRhs(std::string field_name, ScalarFunc source_term_function, boost::shared_ptr< VectorDouble > field_vec, boost::shared_ptr< MatrixDouble > field_grad_mat)
FormsIntegrators< BoundaryEleOp >::Assembly< PETSC >::BiLinearForm< GAUSS >::OpMass< 1, 1 > OpBoundaryLhs
boost::shared_ptr< VectorDouble > fieldVec
EntitiesFieldData::EntData EntData
constexpr auto field_name
boost::shared_ptr< MatrixDouble > fieldGradMat
FTensor::Tensor1< FTensor::PackPtr< double *, Tensor_Dim >, Tensor_Dim > getFTensor1DiffN(const FieldApproximationBase base)
Get derivatives of base functions.
int nbRows
number of dofs on rows
ScalarFunc sourceTermFunc
ForcesAndSourcesCore::UserDataOperator UserDataOperator
ElementsAndOps< SPACE_DIM >::DomainEle DomainEle
boost::function< double(const double, const double, const double)> ScalarFunc
#define MoFEMFunctionReturn(a)
Last executable line of each PETSc function used for error handling. Replaces return()
#define MoFEMFunctionBegin
First executable line of each MoFEM function, used for error handling. Final line of MoFEM functions ...