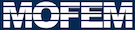 |
| v0.14.0
|
#include <tutorials/cor-7/src/ElasticityMixedFormulation.hpp>
◆ DataAtIntegrationPts() [1/3]
◆ DataAtIntegrationPts() [2/3]
◆ DataAtIntegrationPts() [3/3]
DataAtIntegrationPts::DataAtIntegrationPts |
( |
| ) |
|
|
inline |
◆ getBlockData()
MoFEMErrorCode DataAtIntegrationPts::getBlockData |
( |
BlockData & |
data | ) |
|
|
inline |
◆ getParameters()
MoFEMErrorCode DataAtIntegrationPts::getParameters |
( |
| ) |
|
|
inline |
◆ setBlocks()
MoFEMErrorCode DataAtIntegrationPts::setBlocks |
( |
| ) |
|
|
inline |
◆ blockChrgDens
double DataAtIntegrationPts::blockChrgDens |
◆ blockPermittivity
double DataAtIntegrationPts::blockPermittivity |
◆ cauchyStressMat
boost::shared_ptr<MatrixDouble> DataAtIntegrationPts::cauchyStressMat |
◆ detHVec
boost::shared_ptr<VectorDouble> DataAtIntegrationPts::detHVec |
◆ energyVec
boost::shared_ptr<VectorDouble> DataAtIntegrationPts::energyVec |
◆ eshelbyStress_dx
boost::shared_ptr<MatrixDouble> DataAtIntegrationPts::eshelbyStress_dx |
◆ eshelbyStressMat
boost::shared_ptr<MatrixDouble> DataAtIntegrationPts::eshelbyStressMat |
◆ FMat
boost::shared_ptr<MatrixDouble> DataAtIntegrationPts::FMat |
◆ forcesOnlyOnEntitiesCol
Range DataAtIntegrationPts::forcesOnlyOnEntitiesCol |
◆ forcesOnlyOnEntitiesRow
Range DataAtIntegrationPts::forcesOnlyOnEntitiesRow |
◆ gradDispPtr
boost::shared_ptr<MatrixDouble> DataAtIntegrationPts::gradDispPtr |
◆ hMat
boost::shared_ptr<MatrixDouble> DataAtIntegrationPts::hMat |
◆ HMat
boost::shared_ptr<MatrixDouble> DataAtIntegrationPts::HMat |
◆ invHMat
boost::shared_ptr<MatrixDouble> DataAtIntegrationPts::invHMat |
◆ lAmbda
double DataAtIntegrationPts::lAmbda |
◆ mField
◆ mU
double DataAtIntegrationPts::mU |
◆ petscVec
◆ pOisson
double DataAtIntegrationPts::pOisson |
◆ pPtr
boost::shared_ptr<VectorDouble> DataAtIntegrationPts::pPtr |
◆ setOfBlocksData
std::map<int, BlockData> DataAtIntegrationPts::setOfBlocksData |
◆ smallStrainMat
boost::shared_ptr<MatrixDouble> DataAtIntegrationPts::smallStrainMat |
◆ stiffnessMat
boost::shared_ptr<MatrixDouble> DataAtIntegrationPts::stiffnessMat |
◆ tD
◆ yOung
double DataAtIntegrationPts::yOung |
The documentation for this struct was generated from the following files:
boost::shared_ptr< VectorDouble > energyVec
boost::shared_ptr< MatrixDouble > FMat
virtual MPI_Comm & get_comm() const =0
#define _IT_CUBITMESHSETS_BY_BCDATA_TYPE_FOR_LOOP_(MESHSET_MANAGER, CUBITBCTYPE, IT)
Iterator that loops over a specific Cubit MeshSet in a moFEM field.
UBlasMatrix< double > MatrixDouble
virtual int get_comm_rank() const =0
boost::shared_ptr< MatrixDouble > eshelbyStressMat
std::map< int, BlockData > setOfBlocksData
boost::shared_ptr< MatrixDouble > hMat
#define CHKERR
Inline error check.
SmartPetscObj< Vec > petscVec
auto createGhostVector(MPI_Comm comm, PetscInt n, PetscInt N, PetscInt nghost, const PetscInt ghosts[])
Create smart ghost vector.
virtual moab::Interface & get_moab()=0
@ MAT_ELASTICSET
block name is "MAT_ELASTIC"
MoFEM::Interface & mField
FTensor::Index< 'i', SPACE_DIM > i
boost::shared_ptr< MatrixDouble > invHMat
boost::shared_ptr< MatrixDouble > HMat
boost::shared_ptr< VectorDouble > pPtr
boost::shared_ptr< MatrixDouble > gradDispPtr
FTensor::Index< 'j', 3 > j
boost::shared_ptr< MatrixDouble > eshelbyStress_dx
static MoFEMErrorCodeGeneric< PetscErrorCode > ierr
FTensor::Ddg< double, 3, 3 > tD
MoFEMErrorCode setBlocks()
UBlasVector< double > VectorDouble
FTensor::Index< 'k', 3 > k
#define MoFEMFunctionReturn(a)
Last executable line of each PETSc function used for error handling. Replaces return()
boost::shared_ptr< MatrixDouble > smallStrainMat
boost::shared_ptr< MatrixDouble > stiffnessMat
#define MoFEMFunctionBegin
First executable line of each MoFEM function, used for error handling. Final line of MoFEM functions ...
FTensor::Index< 'l', 3 > l
boost::shared_ptr< VectorDouble > detHVec
boost::shared_ptr< MatrixDouble > cauchyStressMat