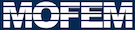 |
| v0.14.0
|
Go to the documentation of this file.
11 #ifndef _ESSENTIAL_DISPLACEMENTCUBITBCDATA_HPP__
12 #define _ESSENTIAL_DISPLACEMENTCUBITBCDATA_HPP__
50 rotated_displacement(
i) = 0;
52 auto get_rotation = [&](
auto &
omega) {
59 if (std::abs(angle) < std::numeric_limits<double>::epsilon())
60 return rotation_matrix;
63 t_omega(
i,
j) = FTensor::levi_civita<double>(
i,
j,
k) *
omega(
k);
64 const double a = sin(angle) / angle;
65 const double sin_squared = sin(angle / 2.) * sin(angle / 2.);
66 const double b = 2. * sin_squared / (angle * angle);
67 rotation_matrix(
i,
j) +=
a * t_omega(
i,
j);
68 rotation_matrix(
i,
j) += b * t_omega(
i,
k) * t_omega(
k,
j);
70 return rotation_matrix;
73 auto rotation = get_rotation(angles);
75 coordinate_distance(
i) = offset(
i) - coordinates(
i);
76 rotated_displacement(
i) =
77 coordinate_distance(
i) - rotation(
j,
i) * coordinate_distance(
j);
79 return rotated_displacement;
93 boost::shared_ptr<FEMethod> fe_ptr,
94 std::vector<boost::shared_ptr<ScalingMethod>> smv,
95 bool get_coords =
false);
113 boost::shared_ptr<FEMethod> fe_ptr,
double diag,
132 boost::shared_ptr<FEMethod> fe_ptr,
double diag,
153 boost::shared_ptr<FEMethod> fe_ptr,
167 template <
int FIELD_DIM, AssemblyType A, IntegrationType I,
typename OpBase>
170 I>::template
OpSource<1, FIELD_DIM> {
176 boost::shared_ptr<DisplacementCubitBcData> bc_data,
177 boost::shared_ptr<Range> ents_ptr,
178 std::vector<boost::shared_ptr<ScalingMethod>> smv);
192 t_ret(
i) = tVal(
i) + rot(
i);
198 if (bc_data->data.flag4 || bc_data->data.flag5 || bc_data->data.flag6) {
199 return [
this](
double x,
double y,
double z) {
200 return this->rotFunction(x, y, z);
203 return [
this](
double x,
double y,
double z) {
return tVal; };
207 template <
int FIELD_DIM, AssemblyType A, IntegrationType I,
typename OpBase>
208 OpEssentialRhsImpl<DisplacementCubitBcData, 1, FIELD_DIM, A, I, OpBase>::
210 boost::shared_ptr<DisplacementCubitBcData> bc_data,
211 boost::shared_ptr<Range> ents_ptr,
212 std::vector<boost::shared_ptr<ScalingMethod>> smv)
214 vecOfTimeScalingMethods(smv) {
215 static_assert(
FIELD_DIM > 1,
"Is not implemented for scalar field");
222 if (bc_data->data.flag1 == 1)
223 tVal(0) = -bc_data->data.value1;
224 if (bc_data->data.flag2 == 1 &&
FIELD_DIM > 1)
225 tVal(1) = -bc_data->data.value2;
226 if (bc_data->data.flag3 == 1 &&
FIELD_DIM > 2)
227 tVal(2) = -bc_data->data.value3;
228 if (bc_data->data.flag4 == 1 &&
FIELD_DIM > 2)
229 tAngles(0) = -bc_data->data.value4;
230 if (bc_data->data.flag5 == 1 &&
FIELD_DIM > 2)
231 tAngles(1) = -bc_data->data.value5;
232 if (bc_data->data.flag6 == 1 &&
FIELD_DIM > 1)
233 tAngles(2) = -bc_data->data.value6;
235 if (
auto ext_bc_data =
238 for (
int a = 0;
a != 3; ++
a)
239 tOffset(
a) = ext_bc_data->rotOffset[
a];
242 this->timeScalingFun = [
this](
const double t) {
244 for (
auto &o : vecOfTimeScalingMethods) {
256 I>::template
OpMass<BASE_DIM, FIELD_DIM> {
262 boost::shared_ptr<Range> ents_ptr);
269 boost::shared_ptr<Range>
293 boost::ptr_deque<ForcesAndSourcesCore::UserDataOperator> &pipeline,
294 const std::string problem_name, std::string
field_name,
295 boost::shared_ptr<MatrixDouble> field_mat_ptr,
296 std::vector<boost::shared_ptr<ScalingMethod>> smv
306 A>::template LinearForm<I>::template OpBaseTimesVector<
BASE_DIM,
309 auto add_op = [&](
auto &bcs) {
311 for (
auto &
m : bcs) {
312 if (
auto bc =
m.second->dispBcPtr) {
313 auto &bc_id =
m.first;
315 (boost::format(
"%s_%s_(.*)") % problem_name %
field_name).str();
316 if (std::regex_match(bc_id, std::regex(regex_str))) {
322 pipeline.push_back(
new OpInternal(
324 [](
double,
double,
double) constexpr {
return 1.; },
325 m.second->getBcEntsPtr()));
355 boost::ptr_deque<ForcesAndSourcesCore::UserDataOperator> &pipeline,
356 const std::string problem_name, std::string
field_name
365 auto add_op = [&](
auto &bcs) {
367 for (
auto &
m : bcs) {
368 if (
auto bc =
m.second->dispBcPtr) {
369 auto &bc_id =
m.first;
371 (boost::format(
"%s_%s_(.*)") % problem_name %
field_name).str();
372 if (std::regex_match(bc_id, std::regex(regex_str))) {
376 pipeline.push_back(
new OP(
field_name,
m.second->getBcEntsPtr()));
393 #endif // _ESSENTIAL_DISPLACEMENTCUBITBCDATA_HPP__
#define MoFEMFunctionReturnHot(a)
Last executable line of each PETSc function used for error handling. Replaces return()
MoFEMErrorCode getInterface(IFACE *&iface) const
Get interface refernce to pointer of interface.
constexpr double omega
Save field DOFS on vertices/tags.
DisplacementCubitBcDataWithRotation()
FTensor::Tensor1< double, FIELD_DIM > rotFunction(double x, double y, double z)
#define MOFEM_LOG_CHANNEL(channel)
Set and reset channel.
FTensor::Tensor1< double, FIELD_DIM > tVal
VecOfTimeScalingMethods vecOfTimeScalingMethods
FTensor::Tensor1< double, 3 > tAngles
VecOfTimeScalingMethods vecOfTimeScalingMethods
std::array< double, 3 > rotOffset
Essential boundary conditions.
PetscErrorCode MoFEMErrorCode
MoFEM/PETSc error code.
SmartPetscObj< Vec > vRhs
Definition of the displacement bc data structure.
typename FormsIntegrators< OpBase >::template Assembly< A >::template LinearForm< I >::template OpSource< 1, FIELD_DIM > OpSource
boost::weak_ptr< FEMethod > fePtr
MoFEM::Interface & mField
Deprecated interface functions.
constexpr IntegrationType I
#define CHKERR
Inline error check.
implementation of Data Operators for Forces and Sources
Simple interface for fast problem set-up.
boost::weak_ptr< FEMethod > fePtr
SmartPetscObj< Mat > vLhs
MoFEM::Interface & mField
Enforce essential constrains on lhs.
BcMapByBlockName & getBcMapByBlockName()
Get the bc map.
Function (factory) for setting operators for rhs pipeline.
Class (Function) to enforce essential constrains on the left hand side diagonal.
static MoFEMErrorCode add(MoFEM::Interface &m_field, boost::ptr_deque< ForcesAndSourcesCore::UserDataOperator > &pipeline, const std::string problem_name, std::string field_name)
Function (factory) for setting operators for lhs pipeline.
MoFEM::Interface & mField
boost::weak_ptr< FEMethod > fePtr
static FTensor::Tensor1< double, 3 > GetRotDisp(const FTensor::Tensor1< double, 3 > &angles, FTensor::Tensor1< double, 3 > coordinates, FTensor::Tensor1< double, 3 > offset=FTensor::Tensor1< double, 3 >{ 0., 0., 0.})
Calculates the rotated displacement given the rotation angles, coordinates, and an optional offset.
SeverityLevel
Severity levels.
SmartPetscObj< Vec > vRhs
constexpr double t
plate stiffness
FTensor::Index< 'i', SPACE_DIM > i
Class (Function) to calculate residual side diagonal.
static MoFEMErrorCode add(MoFEM::Interface &m_field, boost::ptr_deque< ForcesAndSourcesCore::UserDataOperator > &pipeline, const std::string problem_name, std::string field_name, boost::shared_ptr< MatrixDouble > field_mat_ptr, std::vector< boost::shared_ptr< ScalingMethod >> smv)
Class (Function) to enforce essential constrains on the right hand side diagonal.
constexpr auto field_name
VectorFun< FIELD_DIM > initDispFunction(boost::shared_ptr< DisplacementCubitBcData > bc_data)
typename FormsIntegrators< OpBase >::template Assembly< A >::template BiLinearForm< I >::template OpMass< BASE_DIM, FIELD_DIM > OpMass
#define MOFEM_TAG_AND_LOG(channel, severity, tag)
Tag and log in channel.
Enforce essential constrains on rhs.
Tensor2_Expr< Kronecker_Delta< T >, T, Dim0, Dim1, i, j > kronecker_delta(const Index< i, Dim0 > &, const Index< j, Dim1 > &)
Rank 2.
FTensor::Index< 'j', 3 > j
LogManager::SeverityLevel sevLevel
std::vector< boost::shared_ptr< ScalingMethod > > VecOfTimeScalingMethods
Vector of time scaling methods.
Set indices on entities on finite element.
FTensor::Tensor1< double, 3 > tOffset
FTensor::Index< 'm', 3 > m
#define MoFEMFunctionBeginHot
First executable line of each MoFEM function, used for error handling. Final line of MoFEM functions ...
A specialized version of DisplacementCubitBcData that includes an additional rotation offset.
Class (Function) to enforce essential constrains.
FTensor::Index< 'k', 3 > k
#define MoFEMFunctionReturn(a)
Last executable line of each PETSc function used for error handling. Replaces return()
#define MoFEMFunctionBegin
First executable line of each MoFEM function, used for error handling. Final line of MoFEM functions ...
boost::weak_ptr< FEMethod > fePtr
MoFEM::Interface & mField