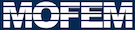 |
| v0.14.0
|
Go to the documentation of this file.
63 boost::shared_ptr<DofEntity> arc_length_dof =
nullptr);
118 bool global_derivatives);
136 bool global_derivatives);
146 bool global_derivatives);
170 Tag
th,
double &total_stress_at_node,
double &total_stress_error_at_node,
171 double &hydro_static_error_at_node,
double &deviatoric_error_at_node,
172 double &total_energy,
double &total_energy_error,
175 typedef ublas::vector<double, ublas::bounded_array<double, 9>>
VecVals;
177 ublas::bounded_array<double, 27>>
180 ublas::bounded_array<double, 81>>
223 std::map<EntityHandle, std::vector<MatrixDouble>>
invABMap;
229 std::map<EntityHandle, std::vector<std::vector<EntityHandle>>>
244 OpMWLSBase(boost::shared_ptr<MatrixDouble> mat_pos_at_pts_ptr,
245 boost::shared_ptr<MatrixDouble> mat_grad_pos_at_pts_ptr,
246 boost::shared_ptr<CrackFrontElement> fe_singular_ptr,
247 boost::shared_ptr<MWLSApprox> mwls_approx,
bool testing =
false)
281 boost::shared_ptr<MatrixDouble> mat_pos_at_pts_ptr,
282 boost::shared_ptr<MatrixDouble> mat_grad_pos_at_pts_ptr,
283 boost::shared_ptr<CrackFrontElement> fe_singular_ptr,
284 boost::shared_ptr<MWLSApprox> mwls_approx,
bool testing =
false)
285 :
OpMWLSBase<T>(mat_pos_at_pts_ptr, mat_grad_pos_at_pts_ptr,
286 fe_singular_ptr, mwls_approx,
testing),
294 typedef OpMWLSCalculateBaseCoeffcientsAtGaussPtsTmpl<
295 VolumeElementForcesAndSourcesCore>
303 :
public OpMWLSBase<VolumeElementForcesAndSourcesCore> {
310 boost::shared_ptr<MatrixDouble> mat_pos_at_pts_ptr,
311 boost::shared_ptr<MatrixDouble> mat_grad_pos_at_pts_ptr,
312 boost::shared_ptr<CrackFrontElement> fe_singular_ptr,
313 boost::shared_ptr<MWLSApprox> mwls_approx,
314 const std::string rho_tag_name,
const bool calculate_derivative,
315 const bool calculate_2nd_derivative,
bool testing =
false)
316 :
OpMWLSBase<VolumeElementForcesAndSourcesCore>(
317 mat_pos_at_pts_ptr, mat_grad_pos_at_pts_ptr, fe_singular_ptr,
337 boost::shared_ptr<MatrixDouble> mat_grad_pos_at_pts_ptr,
338 boost::shared_ptr<CrackFrontElement> fe_singular_ptr,
339 boost::shared_ptr<MWLSApprox> mwls_approx,
340 const std::string rho_tag_name,
341 const bool calculate_derivative,
342 const bool calculate_2nd_derivative,
344 :
OpMWLSBase<VolumeElementForcesAndSourcesCore>(
345 mat_pos_at_pts_ptr, mat_grad_pos_at_pts_ptr, fe_singular_ptr,
364 boost::shared_ptr<moab::Interface> &post_proc_mesh,
365 boost::shared_ptr<std::vector<EntityHandle>> &map_gauss_pts,
366 boost::shared_ptr<MWLSApprox> mwls_approx,
373 PetscErrorCode
doWork(
int side, EntityType
type,
378 :
public OpMWLSBase<VolumeElementForcesAndSourcesCore> {
385 boost::shared_ptr<MatrixDouble> mat_pos_at_pts_ptr,
386 boost::shared_ptr<MatrixDouble> mat_grad_pos_at_pts_ptr,
387 boost::shared_ptr<CrackFrontElement> fe_singular_ptr,
388 boost::shared_ptr<MWLSApprox> mwls_approx,
389 const std::string stress_tag_name,
bool calculate_derivative,
391 :
OpMWLSBase<VolumeElementForcesAndSourcesCore>(
392 mat_pos_at_pts_ptr, mat_grad_pos_at_pts_ptr, fe_singular_ptr,
405 :
OpMWLSBase<VolumeElementForcesAndSourcesCore> {
411 boost::shared_ptr<MatrixDouble> mat_pos_at_pts_ptr,
412 boost::shared_ptr<MatrixDouble> mat_grad_pos_at_pts_ptr,
413 boost::shared_ptr<CrackFrontElement> fe_singular_ptr,
414 boost::shared_ptr<MWLSApprox> mwls_approx,
415 const std::string stress_tag_name,
bool calculate_derivative,
417 :
OpMWLSBase<VolumeElementForcesAndSourcesCore>(
418 mat_pos_at_pts_ptr, mat_grad_pos_at_pts_ptr, fe_singular_ptr,
439 boost::shared_ptr<moab::Interface> post_proc_mesh,
440 boost::shared_ptr<std::vector<EntityHandle>> map_gauss_pts,
441 boost::shared_ptr<MWLSApprox> mwls_approx)
447 PetscErrorCode
doWork(
int side, EntityType
type,
461 const std::string stress_tag_name,
482 boost::shared_ptr<MatrixDouble> mat_grad_pos_at_pts_ptr,
483 boost::shared_ptr<MWLSApprox> mwls_approx,
const bool testing =
false)
505 boost::shared_ptr<MatrixDouble> space_grad_pos_at_pts_ptr,
506 boost::shared_ptr<MatrixDouble> mat_grad_pos_at_pts_ptr,
507 boost::shared_ptr<MWLSApprox> mwls_approx,
508 Range *forces_only_on_entities_row = NULL)
514 if (forces_only_on_entities_row)
528 boost::shared_ptr<MatrixDouble> mat_grad_pos_at_pts_ptr,
529 boost::shared_ptr<MWLSApprox> mwls_approx)
531 "SPATIAL_POSITION",
"MESH_NODE_POSITIONS",
551 boost::shared_ptr<MatrixDouble> space_grad_pos_at_pts_ptr,
552 boost::shared_ptr<MatrixDouble> mat_grad_pos_at_pts_ptr,
553 boost::shared_ptr<MWLSApprox> mwls_approx,
554 Range *forces_only_on_entities_row =
nullptr)
556 "MESH_NODE_POSITIONS",
"SPATIAL_POSITION",
561 if (forces_only_on_entities_row)
579 boost::shared_ptr<MatrixDouble> space_grad_pos_at_pts_ptr,
580 boost::shared_ptr<MatrixDouble> mat_grad_pos_at_pts_ptr,
581 boost::shared_ptr<MWLSApprox> mwls_approx,
582 Range *forces_only_on_entities_row =
nullptr)
584 "MESH_NODE_POSITIONS",
"MESH_NODE_POSITIONS",
589 if (forces_only_on_entities_row)
601 boost::function<
VectorDouble(
const double,
const double,
const double)>
641 ublas::symmetric_matrix<double, ublas::lower>
A;
642 ublas::symmetric_matrix<double, ublas::lower>
testA;
644 ublas::triangular_matrix<double, ublas::lower>
L;
674 template <
class T = FTensor::Tensor1<
double, 3>>
689 baseFun[4] = t_coords(0) * t_coords(0);
690 baseFun[5] = t_coords(1) * t_coords(1);
691 baseFun[6] = t_coords(2) * t_coords(2);
692 baseFun[7] = t_coords(0) * t_coords(1);
693 baseFun[8] = t_coords(0) * t_coords(2);
694 baseFun[9] = t_coords(1) * t_coords(2);
700 template <
class T = FTensor::Tensor1<
double, 3>>
705 for (
int d = 0;
d != 3; ++
d) {
710 for (
int d = 0;
d != 3;
d++) {
749 template <
class T = FTensor::Tensor1<
double, 3>>
754 for (
int d = 0;
d != 9; ++
d) {
763 const double dm2 = dm * dm;
782 const double rr =
r *
r;
783 const double rrr = rr *
r;
784 const double r4 = rrr *
r;
786 return 1 - 6 * rr + 8 * rrr - 3 * r4;
798 const double rr =
r *
r;
799 const double rrr = rr *
r;
801 return -12 *
r + 24 * rr - 12 * rrr;
813 const double rr =
r *
r;
815 return -36 * rr + 48 *
r - 12;
832 #endif //__MWLS_HPP__
MoFEMErrorCode calculateApproxCoeff(bool trim_influence_nodes, bool global_derivatives)
Calculate approximation function coefficients.
#define MoFEMFunctionReturnHot(a)
Last executable line of each PETSc function used for error handling. Replaces return()
double evalWeight(const double r)
double evalDiffWeight(const double r)
boost::function< VectorDouble(const double, const double, const double)> functionTestHack
This is used to set up analytical function for testing approximation.
boost::shared_ptr< MWLSApprox > mwlsApprox
MatrixDouble diffStressAtGaussPts
std::map< EntityHandle, std::array< double, 3 > > elemCentreMap
boost::shared_ptr< MWLSApprox > mwlsApprox
VectorBoundedArray< double, 3 > VectorDouble3
OpMWLSRhoAtGaussPts(boost::shared_ptr< MatrixDouble > mat_pos_at_pts_ptr, boost::shared_ptr< MatrixDouble > mat_grad_pos_at_pts_ptr, boost::shared_ptr< CrackFrontElement > fe_singular_ptr, boost::shared_ptr< MWLSApprox > mwls_approx, const std::string rho_tag_name, const bool calculate_derivative, const bool calculate_2nd_derivative, bool testing=false)
boost::shared_ptr< MatrixDouble > spaceGradPosAtPtsPtr
VecVals outDeviatoricDifference
const VecVals & getDataApprox()
Evaluate density at integration points.
virtual ~OpMWLSStressAtGaussPts()=default
MoFEMErrorCode doWork(int side, EntityType type, DataForcesAndSourcesCore::EntData &data)
boost::shared_ptr< MWLSApprox > mwlsApprox
double young_modulus
Young modulus.
const MatDiffDiffVals & getDiffDiffDataApprox()
MoFEMErrorCode doWork(int side, EntityType type, DataForcesAndSourcesCore::EntData &data)
MoFEMErrorCode doWork(int row_side, int col_side, EntityType row_type, EntityType col_type, DataForcesAndSourcesCore::EntData &row_data, DataForcesAndSourcesCore::EntData &col_data)
OpMWLSStressPostProcess(boost::shared_ptr< moab::Interface > post_proc_mesh, boost::shared_ptr< std::vector< EntityHandle >> map_gauss_pts, boost::shared_ptr< MWLSApprox > mwls_approx)
MatrixDouble diffDiffApproxFun
MoFEMErrorCode getMWLSOptions()
get options from command line for MWLS
MoFEMErrorCode getErrorAtNode(Tag th, double &total_stress_at_node, double &total_stress_error_at_node, double &hydro_static_error_at_node, double &deviatoric_error_at_node, double &total_energy, double &total_energy_error, const double young_modulus, const double poisson_ratio)
Get the norm of the error at nod.
boost::shared_ptr< BVHTree > myTree
const bool calculateDerivative
OpMWLSCalculateBaseCoeffcientsAtGaussPtsTmpl< FaceElementForcesAndSourcesCore > OpMWLSCalculateBaseCoeffcientsForFaceAtGaussPts
int splitsPerDir
Split per direction in the tree.
MoFEMErrorCode loadMWLSMesh(std::string file_name)
virtual ~OpMWLSBase()=default
PetscErrorCode MoFEMErrorCode
MoFEM/PETSc error code.
boost::shared_ptr< MatrixDouble > diffRhoAtGaussPts
boost::shared_ptr< MatrixDouble > matGradPosAtPtsPtr
UBlasMatrix< double > MatrixDouble
MoFEMErrorCode doMWLSWork(int side, EntityType type, DataForcesAndSourcesCore::EntData &data)
Do specific work in operator.
Range forcesOnlyOnEntitiesRow
boost::shared_ptr< MWLSApprox > mwlsApprox
boost::shared_ptr< MatrixDouble > matGradPosAtPtsPtr
double evalDiffDiffWeight(const double r)
OpMWLSMaterialStressLhs_DX(boost::shared_ptr< MatrixDouble > space_grad_pos_at_pts_ptr, boost::shared_ptr< MatrixDouble > mat_grad_pos_at_pts_ptr, boost::shared_ptr< MWLSApprox > mwls_approx, Range *forces_only_on_entities_row=nullptr)
MatrixDouble approxBasePoint
MoFEMErrorCode calculateBase(const T &t_coords)
MoFEMErrorCode evaluateApproxFun(double eval_point[3])
evaluate approximation function at material point
MatrixDouble diffApproxFun
std::string stressTagName
@ OPROWCOL
operator doWork is executed on FE rows &columns
boost::shared_ptr< MatrixDouble > matGradPosAtPtsPtr
std::vector< EntityHandle > leafsVec
OpMWLSBase(boost::shared_ptr< MatrixDouble > mat_pos_at_pts_ptr, boost::shared_ptr< MatrixDouble > mat_grad_pos_at_pts_ptr, boost::shared_ptr< CrackFrontElement > fe_singular_ptr, boost::shared_ptr< MWLSApprox > mwls_approx, bool testing=false)
std::vector< EntityHandle > leafsTetsVecLeaf
VectorDouble3 approxPointCoords
ublas::matrix< double, ublas::row_major, ublas::bounded_array< double, 27 > > MatDiffVals
Deprecated interface functions.
ublas::triangular_matrix< double, ublas::lower > L
MatrixDouble influenceNodesData
MoFEMErrorCode doMWLSWork(int side, EntityType type, DataForcesAndSourcesCore::EntData &data)
Do specific work in operator.
std::vector< EntityHandle > influenceNodes
boost::shared_ptr< std::vector< EntityHandle > > mapGaussPtsPtr
boost::shared_ptr< MWLSApprox > mwlsApprox
DeprecatedCoreInterface Interface
Evaluate stress at integration points.
OpMWLSMaterialStressRhs(boost::shared_ptr< MatrixDouble > space_grad_pos_at_pts_ptr, boost::shared_ptr< MatrixDouble > mat_grad_pos_at_pts_ptr, boost::shared_ptr< MWLSApprox > mwls_approx, Range *forces_only_on_entities_row=NULL)
double outHydroStaticError
boost::shared_ptr< MatrixDouble > matGradPosAtPtsPtr
MatrixDouble singularCurrentDisplacement
MatrixDouble stressAtGaussPts
const bool calculate2ndDerivative
boost::shared_ptr< MWLSApprox > mwlsApproxPtr
boost::weak_ptr< CrackFrontElement > feSingularPtr
boost::shared_ptr< MWLSApprox > mwlsApprox
std::map< EntityHandle, std::vector< MatrixDouble > > invABMap
Store Coefficient of base functions at integration points.
ublas::symmetric_matrix< double, ublas::lower, ublas::row_major, DoubleAllocator > diffInvA[3]
boost::shared_ptr< VectorDouble > rhoAtGaussPts
OpMWLSStressAtGaussPts(boost::shared_ptr< MatrixDouble > mat_pos_at_pts_ptr, boost::shared_ptr< MatrixDouble > mat_grad_pos_at_pts_ptr, boost::shared_ptr< CrackFrontElement > fe_singular_ptr, boost::shared_ptr< MWLSApprox > mwls_approx, const std::string stress_tag_name, bool calculate_derivative, bool testing=false)
boost::shared_ptr< std::vector< EntityHandle > > mapGaussPtsPtr
implementation of Data Operators for Forces and Sources
std::map< EntityHandle, std::vector< std::vector< EntityHandle > > > influenceNodesMap
Influence nodes on elements on at integration points.
MoFEMErrorCode evaluateSecondDiffApproxFun(double eval_point[3], bool global_derivatives)
evaluate 2nd derivative of approximation function at material point
boost::shared_ptr< MWLSApprox > mwlsApproxPtr
boost::weak_ptr< DofEntity > arcLengthDof
virtual ~MWLSApprox()=default
boost::shared_ptr< moab::Interface > postProcMeshPtr
ublas::symmetric_matrix< double, ublas::lower > A
double outDeviatoricError
MatrixDouble mwlsMaterialPositions
std::string stressTagName
boost::shared_ptr< MatrixDouble > matGradPosAtPtsPtr
std::vector< double > leafsDist
virtual MoFEMErrorCode doMWLSWork(int side, EntityType type, DataForcesAndSourcesCore::EntData &data)=0
Do specific work in operator.
boost::shared_ptr< MWLSApprox > mwlsApproxPtr
MoFEMErrorCode doMWLSWork(int side, EntityType type, DataForcesAndSourcesCore::EntData &data)
Do specific work in operator.
double dmFactor
Relative value of weight function radius.
double poisson_ratio
Poisson ratio.
int maxThreeDepth
Maximal three depths.
PetscErrorCode doWork(int side, EntityType type, DataForcesAndSourcesCore::EntData &data)
OpMWLSSpatialStressRhs(boost::shared_ptr< MatrixDouble > mat_grad_pos_at_pts_ptr, boost::shared_ptr< MWLSApprox > mwls_approx, const bool testing=false)
moab::Interface & mwlsMoab
ublas::vector< double, ublas::bounded_array< double, 9 > > VecVals
ublas::symmetric_matrix< double, ublas::lower > testA
boost::shared_ptr< MatrixDouble > spaceGradPosAtPtsPtr
MoFEMErrorCode evaluateFirstDiffApproxFun(double eval_point[3], bool global_derivatives)
evaluate 1st derivative of approximation function at material point
OpMWLSRhoPostProcess(boost::shared_ptr< moab::Interface > &post_proc_mesh, boost::shared_ptr< std::vector< EntityHandle >> &map_gauss_pts, boost::shared_ptr< MWLSApprox > mwls_approx, PostProcVolumeOnRefinedMesh::CommonData &common_data)
PetscErrorCode doWork(int side, EntityType type, DataForcesAndSourcesCore::EntData &data)
PostProcVolumeOnRefinedMesh::CommonData & commonData
MoFEMErrorCode doWork(int row_side, int col_side, EntityType row_type, EntityType col_type, DataForcesAndSourcesCore::EntData &row_data, DataForcesAndSourcesCore::EntData &col_data)
MoFEMErrorCode calculateDiffDiffBase(const T &t_coords, const double dm)
const bool calculateDerivative
MatDiffDiffVals outDiffDiffData
Range forcesOnlyOnEntitiesRow
MatrixDouble & getInvAB()
MoFEMErrorCode doWork(int side, EntityType type, DataForcesAndSourcesCore::EntData &data)
const MatDiffVals & getDiffDataApprox()
EntitiesFieldData::EntData EntData
VecAllocator< double > DoubleAllocator
std::string stressTagName
PetscBool mwlsAnalyticalInternalStressTest
OpMWLSStressAndErrorsAtGaussPts(boost::shared_ptr< MWLSApprox > mwls_approx, const std::string stress_tag_name, MoFEM::Interface &m_field)
boost::shared_ptr< MatrixDouble > matPosAtPtsPtr
MoFEM::Interface & mField
OpMWLSRhoAtGaussUsingPrecalulatedCoeffs(boost::shared_ptr< MatrixDouble > mat_pos_at_pts_ptr, boost::shared_ptr< MatrixDouble > mat_grad_pos_at_pts_ptr, boost::shared_ptr< CrackFrontElement > fe_singular_ptr, boost::shared_ptr< MWLSApprox > mwls_approx, const std::string rho_tag_name, const bool calculate_derivative, const bool calculate_2nd_derivative, bool testing=false)
MoFEMErrorCode doWork(int side, EntityType type, DataForcesAndSourcesCore::EntData &data)
ublas::symmetric_matrix< double, ublas::lower, ublas::row_major, DoubleAllocator > diffA[3]
std::vector< double > leafsTetsCentre
VectorDouble diffBaseFun[3]
ForcesAndSourcesCore::UserDataOperator UserDataOperator
MoFEMErrorCode doMWLSWork(int side, EntityType type, DataForcesAndSourcesCore::EntData &data)
Do specific work in operator.
boost::shared_ptr< MatrixDouble > spaceGradPosAtPtsPtr
UBlasVector< int > VectorInt
ublas::matrix< double, ublas::row_major, ublas::bounded_array< double, 81 > > MatDiffDiffVals
std::vector< EntityHandle > & getInfluenceNodes()
OpMWLSCalculateBaseCoeffcientsAtGaussPtsTmpl(boost::shared_ptr< MatrixDouble > mat_pos_at_pts_ptr, boost::shared_ptr< MatrixDouble > mat_grad_pos_at_pts_ptr, boost::shared_ptr< CrackFrontElement > fe_singular_ptr, boost::shared_ptr< MWLSApprox > mwls_approx, bool testing=false)
MoFEMErrorCode calculateDiffBase(const T &t_coords, const double dm)
const FTensor::Tensor2< T, Dim, Dim > Vec
MWLSApprox(MoFEM::Interface &m_field, Vec F_lambda=PETSC_NULL, boost::shared_ptr< DofEntity > arc_length_dof=nullptr)
boost::shared_ptr< moab::Interface > postProcMeshPtr
MoFEMErrorCode getTagData(Tag th)
std::map< EntityHandle, std::vector< double > > dmNodesMap
Store weight function radius at the nodes.
MatrixDouble singularInitialDisplacement
UBlasVector< double > VectorDouble
Evaluate stress at integration points.
MoFEM::Interface & mField
OpMWLSMaterialStressLhs_Dx(boost::shared_ptr< MatrixDouble > space_grad_pos_at_pts_ptr, boost::shared_ptr< MatrixDouble > mat_grad_pos_at_pts_ptr, boost::shared_ptr< MWLSApprox > mwls_approx, Range *forces_only_on_entities_row=nullptr)
bool getUseGlobalBaseAtMaterialReferenceConfiguration() const
Get the Use Local Base At Material Reference Configuration object.
#define MoFEMFunctionBeginHot
First executable line of each MoFEM function, used for error handling. Final line of MoFEM functions ...
PetscBool useGlobalBaseAtMaterialReferenceConfiguration
boost::shared_ptr< MatrixDouble > matGradPosAtPtsPtr
OpMWLSCalculateBaseCoeffcientsAtGaussPtsTmpl< VolumeElementForcesAndSourcesCore > OpMWLSCalculateBaseCoeffcientsAtGaussPts
const bool calculateDerivative
OpMWLSSpatialStressLhs_DX(boost::shared_ptr< MatrixDouble > mat_grad_pos_at_pts_ptr, boost::shared_ptr< MWLSApprox > mwls_approx)
boost::shared_ptr< MatrixDouble > matGradPosAtPtsPtr
Range forcesOnlyOnEntitiesRow
std::vector< EntityHandle > treeTets
EntityHandle nearestInfluenceNode
bool sYmm
If true assume that matrix is symmetric structure.
MoFEMErrorCode getValuesToNodes(Tag th)
get values form elements to nodes
boost::shared_ptr< MatrixDouble > diffDiffRhoAtGaussPts
MoFEMErrorCode doMWLSWork(int side, EntityType type, DataForcesAndSourcesCore::EntData &data)
Do specific work in operator.
VectorDouble diffDiffBaseFun[9]
const bool calculate2ndDerivative
const bool calculateDerivative
std::vector< std::pair< double, EntityHandle > > distLeafs
MoFEMErrorCode doWork(int row_side, int col_side, EntityType row_type, EntityType col_type, DataForcesAndSourcesCore::EntData &row_data, DataForcesAndSourcesCore::EntData &col_data)
OpMWLSStressAtGaussUsingPrecalulatedCoeffs(boost::shared_ptr< MatrixDouble > mat_pos_at_pts_ptr, boost::shared_ptr< MatrixDouble > mat_grad_pos_at_pts_ptr, boost::shared_ptr< CrackFrontElement > fe_singular_ptr, boost::shared_ptr< MWLSApprox > mwls_approx, const std::string stress_tag_name, bool calculate_derivative, bool testing=false)
@ OPROW
operator doWork function is executed on FE rows