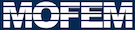 |
| v0.14.0
|
Go to the documentation of this file.
7 #ifndef __SPRINGELEMENT_HPP__
8 #define __SPRINGELEMENT_HPP__
33 :
public boost::enable_shared_from_this<DataAtIntegrationPtsSprings> {
36 boost::make_shared<MatrixDouble>();
37 boost::shared_ptr<MatrixDouble>
xAtPts = boost::make_shared<MatrixDouble>();
39 boost::make_shared<MatrixDouble>();
41 boost::shared_ptr<MatrixDouble>
hMat = boost::make_shared<MatrixDouble>();
42 boost::shared_ptr<MatrixDouble>
FMat = boost::make_shared<MatrixDouble>();
43 boost::shared_ptr<MatrixDouble>
HMat = boost::make_shared<MatrixDouble>();
45 boost::make_shared<MatrixDouble>();
47 boost::make_shared<VectorDouble>();
67 CHKERRABORT(PETSC_COMM_WORLD,
ierr);
72 CHKERR PetscOptionsBegin(PETSC_COMM_WORLD,
"",
"Problem",
"none");
74 ierr = PetscOptionsEnd();
92 if (
bit->getName().compare(0, 9,
"SPRING_BC") == 0) {
94 const int id =
bit->getMeshsetId();
99 std::vector<double> attributes;
100 bit->getAttributes(attributes);
101 if (attributes.size() < 2) {
103 "Springs should have 2 attributes but there is %d",
107 mapSpring[id].springStiffnessNormal = attributes[0];
108 mapSpring[id].springStiffnessTangent = attributes[1];
111 MOFEM_LOG_C(
"WORLD", Sev::verbose,
"\nSpring block %d\n",
id);
112 MOFEM_LOG_C(
"WORLD", Sev::verbose,
"\tNormal stiffness %3.4g\n",
114 MOFEM_LOG_C(
"WORLD", Sev::verbose,
"\tTangent stiffness %3.4g\n",
180 OpSpringFs(boost::shared_ptr<MetaSpringBC::DataAtIntegrationPtsSprings>
187 if (
field_name.compare(0, 16,
"SPATIAL_POSITION") != 0)
232 EntityType col_type,
EntData &row_data,
243 OpSpringKs(boost::shared_ptr<MetaSpringBC::DataAtIntegrationPtsSprings>
318 EntityType col_type,
EntData &row_data,
323 const std::string field_name_1,
324 const std::string field_name_2)
326 field_name_1.c_str(), field_name_2.c_str(),
OPROWCOL),
355 EntityType col_type,
EntData &row_data,
372 const string field_name_1,
const string field_name_2,
373 boost::shared_ptr<DataAtIntegrationPtsSprings> data_at_spring_pts)
427 const string field_name_1,
const string field_name_2,
428 boost::shared_ptr<DataAtIntegrationPtsSprings> data_at_spring_pts)
430 data_at_spring_pts) {
466 boost::shared_ptr<VolumeElementForcesAndSourcesCoreOnSide>
sideFe;
483 EntityType row_type, EntityType col_type,
497 const string field_name_1,
const string field_name_2,
498 boost::shared_ptr<DataAtIntegrationPtsSprings> data_at_spring_pts,
499 boost::shared_ptr<VolumeElementForcesAndSourcesCoreOnSide> side_fe,
500 std::string &side_fe_name)
522 EntityType col_type,
EntData &row_data,
526 const string field_name_1,
const string field_name_2,
527 boost::shared_ptr<DataAtIntegrationPtsSprings> data_at_pts,
528 boost::shared_ptr<VolumeElementForcesAndSourcesCoreOnSide> side_fe,
529 std::string &side_fe_name)
531 side_fe, side_fe_name) {
552 EntityType col_type,
EntData &row_data,
613 const string field_name_1,
const string field_name_2,
614 boost::shared_ptr<DataAtIntegrationPtsSprings> data_at_spring_pts,
615 boost::shared_ptr<VolumeElementForcesAndSourcesCoreOnSide> side_fe,
616 std::string &side_fe_name)
618 side_fe, side_fe_name) {
667 const string field_name_1,
const string field_name_2,
668 boost::shared_ptr<DataAtIntegrationPtsSprings> data_at_spring_pts)
670 data_at_spring_pts) {
680 :
public VolumeElementForcesAndSourcesCoreOnContactPrismSide::
691 boost::shared_ptr<MetaSpringBC::DataAtIntegrationPtsSprings>
693 bool ho_geometry =
false)
718 boost::shared_ptr<MetaSpringBC::DataAtIntegrationPtsSprings>
dataAtPts;
719 boost::shared_ptr<VolumeElementForcesAndSourcesCoreOnSide>
sideFe;
789 const string material_field,
790 boost::shared_ptr<MetaSpringBC::DataAtIntegrationPtsSprings>
792 boost::shared_ptr<VolumeElementForcesAndSourcesCoreOnSide> side_fe,
804 boost::shared_ptr<MetaSpringBC::DataAtIntegrationPtsSprings>
817 boost::shared_ptr<MetaSpringBC::DataAtIntegrationPtsSprings>
863 boost::shared_ptr<MetaSpringBC::DataAtIntegrationPtsSprings>
877 boost::shared_ptr<MetaSpringBC::DataAtIntegrationPtsSprings>
878 data_at_integration_pts)
933 const std::string mesh_nodals_positions =
"MESH_NODE_POSITIONS");
952 const std::string mesh_nodals_positions,
953 Range &spring_triangles);
968 boost::shared_ptr<FaceElementForcesAndSourcesCore> fe_spring_lhs_ptr,
969 boost::shared_ptr<FaceElementForcesAndSourcesCore> fe_spring_rhs_ptr,
971 const std::string mesh_nodals_positions =
"MESH_NODE_POSITIONS",
972 double stiffness_scale = 1.);
987 boost::shared_ptr<FaceElementForcesAndSourcesCore> fe_spring_lhs_ptr_dx,
988 boost::shared_ptr<FaceElementForcesAndSourcesCore> fe_spring_lhs_ptr_dX,
989 boost::shared_ptr<FaceElementForcesAndSourcesCore> fe_spring_rhs_ptr,
990 boost::shared_ptr<DataAtIntegrationPtsSprings> data_at_integration_pts,
991 const std::string
field_name,
const std::string mesh_nodals_positions,
992 std::string side_fe_name);
995 #endif //__SPRINGELEMENT_HPP__
default operator for TET element
Data on single entity (This is passed as argument to DataOperator::doWork)
PetscErrorCode MoFEMErrorCode
MoFEM/PETSc error code.
UBlasMatrix< double > MatrixDouble
@ OPROWCOL
operator doWork is executed on FE rows &columns
Deprecated interface functions.
#define CHKERR
Inline error check.
friend class UserDataOperator
virtual moab::Interface & get_moab()=0
implementation of Data Operators for Forces and Sources
default operator for TRI element
#define MOFEM_LOG_C(channel, severity, format,...)
@ OPCOL
operator doWork function is executed on FE columns
friend class UserDataOperator
constexpr auto field_name
EntitiesFieldData::EntData EntData
#define _IT_CUBITMESHSETS_BY_SET_TYPE_FOR_LOOP_(MESHSET_MANAGER, CUBITBCTYPE, IT)
Iterator that loops over a specific Cubit MeshSet having a particular BC meshset in a moFEM field.
UBlasVector< int > VectorInt
static MoFEMErrorCodeGeneric< PetscErrorCode > ierr
const FTensor::Tensor2< T, Dim, Dim > Vec
UBlasVector< double > VectorDouble
@ MOFEM_ATOM_TEST_INVALID
bool sYmm
If true assume that matrix is symmetric structure.
#define MoFEMFunctionReturn(a)
Last executable line of each PETSc function used for error handling. Replaces return()
#define MoFEMFunctionBegin
First executable line of each MoFEM function, used for error handling. Final line of MoFEM functions ...
@ OPROW
operator doWork function is executed on FE rows