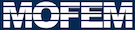 |
| v0.14.0
|
Go to the documentation of this file.
20 static char help[] =
"...\n\n";
35 double operator()(
const double x,
const double y,
const double z)
const {
36 return 1 + x + y + pow(z, 3);
45 const double z)
const {
59 double operator()(
const double x,
const double y,
const double z)
const {
60 return 0.4e1 + (
double)(4 * x) + (
double)(4 * y) + 0.4e1 * pow(z, 0.3e1) +
62 (0.6e1 * z * z + 0.6e1 * (
double)x * z * z +
63 0.6e1 * (
double)y * z * z + 0.6e1 * pow(z, 0.5e1)) *
66 (0.2e1 + (
double)(2 * x) + (
double)(2 * y) +
67 0.2e1 * pow(z, 0.3e1) + (
double)(x * x) + (
double)(2 * x * y) +
69 0.2e1 * (
double)y * pow(z, 0.3e1) + pow(z, 0.6e1)) *
83 int operator()(
int,
int,
int p)
const {
return 2 * (p + 1); }
86 int main(
int argc,
char *argv[]) {
98 PetscBool flg_test = PETSC_FALSE;
99 CHKERR PetscOptionsBegin(PETSC_COMM_WORLD,
"",
"Poisson's problem options",
105 CHKERR PetscOptionsBool(
"-test",
"if true is ctest",
"", flg_test,
106 &flg_test, PETSC_NULL);
107 ierr = PetscOptionsEnd();
127 boost::shared_ptr<ForcesAndSourcesCore>
129 boost::shared_ptr<ForcesAndSourcesCore>
131 boost::shared_ptr<ForcesAndSourcesCore>
133 boost::shared_ptr<ForcesAndSourcesCore>
135 boost::shared_ptr<ForcesAndSourcesCore>
137 boost::shared_ptr<PoissonExample::PostProcFE>
139 boost::shared_ptr<ForcesAndSourcesCore>
null;
146 domain_lhs_fe, boundary_lhs_fe, domain_rhs_fe, boundary_rhs_fe,
152 global_error, domain_error);
233 domain_lhs_fe,
null,
null);
235 boundary_lhs_fe,
null,
null);
238 domain_rhs_fe,
null,
null);
240 boundary_rhs_fe,
null,
null);
248 CHKERR DMCreateGlobalVector(dm, &
F);
255 CHKERR SNESCreate(PETSC_COMM_WORLD, &solver);
256 CHKERR SNESSetFromOptions(solver);
257 CHKERR SNESSetDM(solver, dm);
270 CHKERR SNESDestroy(&solver);
284 if (flg_test == PETSC_TRUE) {
295 post_proc_volume->writeFile(
"out_vol.h5m");
302 CHKERR VecDestroy(&global_error);
MoFEMErrorCode getInterface(IFACE *&iface) const
Get interface reference to pointer of interface.
int main(int argc, char *argv[])
int operator()(int, int, int p) const
double operator()(const double u)
@ L2
field with C-1 continuity
MoFEMErrorCode loadFile(const std::string options, const std::string mesh_file_name, LoadFileFunc loadFunc=defaultLoadFileFunc)
Load mesh file.
static MoFEMErrorCode Finalize()
Checks for options to be called at the conclusion of the program.
Create finite elements instances.
Simple interface for fast problem set-up.
Deprecated interface functions.
DeprecatedCoreInterface Interface
MoFEMErrorCode getOptions()
get options
MoFEMErrorCode createGhostVec(Vec *ghost_vec) const
double operator()(const double x, const double y, const double z) const
double operator()(const double x, const double y, const double z) const
MoFEMErrorCode getDM(DM *dm)
Get DM.
#define CHKERR
Inline error check.
MoFEMErrorCode creatFEToPostProcessResults(boost::shared_ptr< PostProcFE > &post_proc_volume) const
Create finite element to post-process results.
MoFEMErrorCode testError(Vec ghost_vec)
Test error.
PetscErrorCode DMoFEMMeshToGlobalVector(DM dm, Vec g, InsertMode mode, ScatterMode scatter_mode)
set ghosted vector values on all existing mesh entities
MoFEMErrorCode addDomainField(const std::string &name, const FieldSpace space, const FieldApproximationBase base, const FieldCoefficientsNumber nb_of_coefficients, const TagType tag_type=MB_TAG_SPARSE, const enum MoFEMTypes bh=MF_ZERO, int verb=-1)
Add field on domain.
PetscErrorCode DMRegister_MoFEM(const char sname[])
Register MoFEM problem.
const std::string getDomainFEName() const
Get the Domain FE Name.
PetscErrorCode DMMoFEMSNESSetJacobian(DM dm, const char fe_name[], MoFEM::FEMethod *method, MoFEM::BasicMethod *pre_only, MoFEM::BasicMethod *post_only)
set SNES Jacobian evaluation function
MoFEMErrorCode printError(Vec ghost_vec)
Print error.
MoFEMErrorCode addDataField(const std::string &name, const FieldSpace space, const FieldApproximationBase base, const FieldCoefficientsNumber nb_of_coefficients, const TagType tag_type=MB_TAG_SPARSE, const enum MoFEMTypes bh=MF_ZERO, int verb=-1)
Add field on domain.
MoFEMErrorCode setFieldOrder(const std::string field_name, const int order, const Range *ents=NULL)
Set field order.
double operator()(const double u)
static MoFEMErrorCode Initialize(int *argc, char ***args, const char file[], const char help[])
Initializes the MoFEM database PETSc, MOAB and MPI.
#define CATCH_ERRORS
Catch errors.
MoFEMErrorCode createFEToAssembleMatrixAndVectorForNonlinearProblem(boost::function< double(const double, const double, const double)> f_u, boost::function< double(const double, const double, const double)> f_source, boost::function< double(const double)> a, boost::function< double(const double)> diff_a, boost::shared_ptr< ForcesAndSourcesCore > &domain_lhs_fe, boost::shared_ptr< ForcesAndSourcesCore > &boundary_lhs_fe, boost::shared_ptr< ForcesAndSourcesCore > &domain_rhs_fe, boost::shared_ptr< ForcesAndSourcesCore > &boundary_rhs_fe, ForcesAndSourcesCore::RuleHookFun vol_rule, ForcesAndSourcesCore::RuleHookFun face_rule=FaceRule(), bool trans=true) const
Create finite element to calculate matrix and vectors.
MoFEMErrorCode createFEToEvaluateError(boost::function< double(const double, const double, const double)> f_u, boost::function< FTensor::Tensor1< double, 3 >(const double, const double, const double)> g_u, Vec global_error, boost::shared_ptr< ForcesAndSourcesCore > &domain_error) const
Create finite element to calculate error.
static MoFEMErrorCodeGeneric< PetscErrorCode > ierr
@ AINSWORTH_LEGENDRE_BASE
Ainsworth Cole (Legendre) approx. base .
const std::string getBoundaryFEName() const
Get the Boundary FE Name.
const FTensor::Tensor2< T, Dim, Dim > Vec
MoFEMErrorCode addBoundaryField(const std::string &name, const FieldSpace space, const FieldApproximationBase base, const FieldCoefficientsNumber nb_of_coefficients, const TagType tag_type=MB_TAG_SPARSE, const enum MoFEMTypes bh=MF_ZERO, int verb=-1)
Add field on boundary.
const double D
diffusivity
MoFEMErrorCode setUp(const PetscBool is_partitioned=PETSC_TRUE)
Setup problem.
PetscErrorCode DMoFEMLoopFiniteElements(DM dm, const char fe_name[], MoFEM::FEMethod *method, CacheTupleWeakPtr cache_ptr=CacheTupleSharedPtr())
Executes FEMethod for finite elements in DM.
#define CHKERRG(n)
Check error code of MoFEM/MOAB/PETSc function.
MoFEMErrorCode assembleGhostVector(Vec ghost_vec) const
Assemble error vector.
FTensor::Tensor1< double, 3 > operator()(const double x, const double y, const double z) const
PetscErrorCode DMMoFEMSNESSetFunction(DM dm, const char fe_name[], MoFEM::FEMethod *method, MoFEM::BasicMethod *pre_only, MoFEM::BasicMethod *post_only)
set SNES residual evaluation function