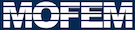 |
| v0.14.0
|
Go to the documentation of this file.
10 static char help[] =
"...\n\n";
12 static const bool debug =
false;
14 int main(
int argc,
char *argv[]) {
21 PetscBool flg = PETSC_TRUE;
23 #if PETSC_VERSION_GE(3, 6, 4)
30 if (flg != PETSC_TRUE) {
31 SETERRQ(PETSC_COMM_SELF, 1,
"*** ERROR -my_file (MESH FILE NEEDED)");
35 DMType dm_name =
"DMMOFEM";
37 DMType dm_name_sub =
"DMSUB";
42 CHKERR DMCreate(PETSC_COMM_WORLD, &dm);
43 CHKERR DMSetType(dm, dm_name);
46 CHKERR DMCreate(PETSC_COMM_WORLD, &subdm0);
47 CHKERR DMSetType(subdm0, dm_name_sub);
48 CHKERR DMCreate(PETSC_COMM_WORLD, &subdm1);
49 CHKERR DMSetType(subdm1, dm_name_sub);
54 ParallelComm *pcomm = ParallelComm::get_pcomm(&moab,
MYPCOMM_INDEX);
56 boost::make_shared<WrapMPIComm>(PETSC_COMM_WORLD,
false);
58 pcomm =
new ParallelComm(&moab, moab_comm_wrap->get_comm());
61 option =
"PARALLEL=BCAST_DELETE;PARALLEL_RESOLVE_SHARED_ENTS;PARTITION="
62 "PARALLEL_PARTITION;";
73 root_set, 3, bit_level0);
75 const int field_rank = 1;
126 CHKERR DMSetFromOptions(dm);
132 ->checkMPIAIJWithArraysMatrixFillIn<PetscGlobalIdx_mi_tag>(
133 "MAIN_PROBLEM", -1, -1, 1);
138 CHKERR DMCreateFieldIS(dm, &nf, &field_names, &fields);
139 for (
int f = 0;
f != nf;
f++) {
140 PetscPrintf(PETSC_COMM_WORLD,
"%d field %s\n",
f, field_names[
f]);
141 CHKERR PetscFree(field_names[
f]);
142 CHKERR ISDestroy(&(fields[
f]));
144 CHKERR PetscFree(field_names);
151 auto verts_ptr = boost::make_shared<Range>();
152 CHKERR moab.get_entities_by_type(0, MBVERTEX, *verts_ptr,
true);
158 ->checkMPIAIJWithArraysMatrixFillIn<PetscGlobalIdx_mi_tag>(
"SUB0", -1,
162 CHKERR DMCreateMatrix(subdm0, &
A);
163 MatView(
A, PETSC_VIEWER_DRAW_WORLD);
176 ->checkMPIAIJWithArraysMatrixFillIn<PetscGlobalIdx_mi_tag>(
"SUB1", -1,
181 CHKERR DMCreateMatrix(subdm1, &B);
182 MatView(B, PETSC_VIEWER_DRAW_WORLD);
190 CHKERR DMDestroy(&subdm0);
191 CHKERR DMDestroy(&subdm1);
MoFEMErrorCode getInterface(IFACE *&iface) const
Get interface reference to pointer of interface.
#define MYPCOMM_INDEX
default communicator number PCOMM
PetscErrorCode DMMoFEMAddSubFieldCol(DM dm, const char field_name[])
virtual MoFEMErrorCode modify_finite_element_add_field_row(const std::string &fe_name, const std::string name_row)=0
set field row which finite element use
PetscErrorCode DMMoFEMSetSquareProblem(DM dm, PetscBool square_problem)
set squared problem
static MoFEMErrorCode Finalize()
Checks for options to be called at the conclusion of the program.
virtual MoFEMErrorCode add_ents_to_field_by_type(const Range &ents, const EntityType type, const std::string &name, int verb=DEFAULT_VERBOSITY)=0
Add entities to field meshset.
PetscErrorCode DMMoFEMAddElement(DM dm, std::string fe_name)
add element to dm
Deprecated interface functions.
DeprecatedCoreInterface Interface
int main(int argc, char *argv[])
virtual MoFEMErrorCode add_ents_to_finite_element_by_type(const EntityHandle entities, const EntityType type, const std::string &name, const bool recursive=true)=0
add entities to finite element
#define CHKERR
Inline error check.
virtual MoFEMErrorCode add_finite_element(const std::string &fe_name, enum MoFEMTypes bh=MF_EXCL, int verb=DEFAULT_VERBOSITY)=0
add finite element
implementation of Data Operators for Forces and Sources
virtual MoFEMErrorCode modify_finite_element_add_field_col(const std::string &fe_name, const std::string name_row)=0
set field col which finite element use
virtual MoFEMErrorCode build_finite_elements(int verb=DEFAULT_VERBOSITY)=0
Build finite elements.
PetscErrorCode DMMoFEMCreateSubDM(DM subdm, DM dm, const char problem_name[])
Must be called by user to set Sub DM MoFEM data structures.
PetscErrorCode DMRegister_MoFEM(const char sname[])
Register MoFEM problem.
PetscErrorCode DMMoFEMCreateMoFEM(DM dm, MoFEM::Interface *m_field_ptr, const char problem_name[], const MoFEM::BitRefLevel bit_level, const MoFEM::BitRefLevel bit_mask=MoFEM::BitRefLevel().set())
Must be called by user to set MoFEM data structures.
virtual MoFEMErrorCode modify_finite_element_add_field_data(const std::string &fe_name, const std::string name_field)=0
set finite element field data
Matrix manager is used to build and partition problems.
static MoFEMErrorCode Initialize(int *argc, char ***args, const char file[], const char help[])
Initializes the MoFEM database PETSc, MOAB and MPI.
#define CATCH_ERRORS
Catch errors.
@ AINSWORTH_LEGENDRE_BASE
Ainsworth Cole (Legendre) approx. base .
PetscErrorCode PetscOptionsGetString(PetscOptions *, const char pre[], const char name[], char str[], size_t size, PetscBool *set)
virtual MoFEMErrorCode build_fields(int verb=DEFAULT_VERBOSITY)=0
std::bitset< BITREFLEVEL_SIZE > BitRefLevel
Bit structure attached to each entity identifying to what mesh entity is attached.
virtual MoFEMErrorCode build_adjacencies(const Range &ents, int verb=DEFAULT_VERBOSITY)=0
build adjacencies
PetscErrorCode DMMoFEMAddSubFieldRow(DM dm, const char field_name[])
virtual MoFEMErrorCode set_field_order(const EntityHandle meshset, const EntityType type, const std::string &name, const ApproximationOrder order, int verb=DEFAULT_VERBOSITY)=0
Set order approximation of the entities in the field.
virtual MoFEMErrorCode add_field(const std::string name, const FieldSpace space, const FieldApproximationBase base, const FieldCoefficientsNumber nb_of_coefficients, const TagType tag_type=MB_TAG_SPARSE, const enum MoFEMTypes bh=MF_EXCL, int verb=DEFAULT_VERBOSITY)=0
Add field.