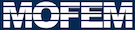 |
| v0.14.0
|
Go to the documentation of this file.
21 using namespace MoFEM;
24 #include <boost/numeric/ublas/vector_proxy.hpp>
25 #include <boost/numeric/ublas/matrix.hpp>
26 #include <boost/numeric/ublas/matrix_proxy.hpp>
27 #include <boost/numeric/ublas/vector.hpp>
28 #include <adolc/adolc.h>
32 #include <boost/iostreams/tee.hpp>
33 #include <boost/iostreams/stream.hpp>
36 namespace bio = boost::iostreams;
37 using bio::tee_device;
42 static char help[] =
"...\n\n";
44 int main(
int argc,
char *argv[]) {
53 MPI_Comm_rank(PETSC_COMM_WORLD,&rank);
60 map<int,Gel::BlockMaterialData> material_data;
63 material_data[0].gAlpha = 1;
64 material_data[0].vAlpha = 0.3;
65 material_data[0].gBeta = 1;
66 material_data[0].vBeta = 0.3;
67 material_data[0].gBetaHat = 1;
68 material_data[0].vBetaHat = 0.3;
69 material_data[0].oMega = 1;
70 material_data[0].vIscosity = 1;
71 material_data[0].pErmeability = 2.;
79 F(0,0) = 0.01;
F(0,1) = 0.02;
F(0,2) = 0.03;
80 F(1,0) = 0.04;
F(1,1) = 0.05;
F(1,2) = 0.06;
81 F(2,0) = 0.07;
F(2,1) = 0.08;
F(2,2) = 0.09;
86 F_dot(0,0) = 0.01; F_dot(0,1) = 0.02; F_dot(0,2) = 0.03;
87 F_dot(1,0) = 0.04; F_dot(1,1) = 0.05; F_dot(1,2) = 0.06;
88 F_dot(2,0) = 0.07; F_dot(2,1) = 0.08; F_dot(2,2) = 0.09;
91 strainHat.resize(3,3);
93 strainHat(0,0) = 0.01; strainHat(0,1) = 0.02; strainHat(0,2) = 0.03;
94 strainHat(1,0) = 0.04; strainHat(1,1) = 0.05; strainHat(1,2) = 0.06;
95 strainHat(2,0) = 0.07; strainHat(2,1) = 0.08; strainHat(2,2) = 0.09;
98 strainHatDot.resize(3,3);
100 strainHatDot(0,0) = 0.01; strainHatDot(0,1) = 0.02; strainHatDot(0,2) = 0.03;
101 strainHatDot(1,0) = 0.04; strainHatDot(1,1) = 0.05; strainHatDot(1,2) = 0.06;
102 strainHatDot(2,0) = 0.07; strainHatDot(2,1) = 0.08; strainHatDot(2,2) = 0.09;
106 gradient_mu.resize(3);
114 typedef tee_device<ostream, ofstream>
TeeDevice;
116 ofstream ofs(
"gel_constitutive_model_test.txt");
123 my_split <<
"C\n" << ce.
C << endl << endl;
126 my_split <<
"strainTotal\n" << ce.
strainTotal << endl << endl;
135 my_split <<
"stressAlpha\n" << ce.
stressAlpha << endl << endl;
138 my_split <<
"stressBeta\n" << ce.
stressBeta << endl << endl;
141 my_split <<
"stressBeta\n" << ce.
stressBetaHat << endl << endl;
144 my_split <<
"stressTotal\n" << ce.
stressTotal << endl << endl;
147 my_split <<
"solventFlux\n" << ce.
solventFlux << endl << endl;
150 my_split <<
"strainHatDot\n" << ce.
strainHatDot << endl << endl;
virtual PetscErrorCode calculateCauchyDefromationTensor()
TYPE solventConcentrationDot
Volume rate change.
ublas::vector< TYPE, ublas::bounded_array< TYPE, 9 > > solventFlux
Solvent flux.
VectorBoundedArray< double, 3 > VectorDouble3
virtual PetscErrorCode calculateStrainHatFlux()
Calculate rate of strain hat.
ublas::vector< TYPE, ublas::bounded_array< TYPE, 3 > > gradientMu
Gradient of solvent concentration.
static MoFEMErrorCode Finalize()
Checks for options to be called at the conclusion of the program.
ublas::matrix< TYPE, ublas::row_major, ublas::bounded_array< TYPE, 9 > > stressTotal
Total stress.
virtual PetscErrorCode calculateStressTotal()
Implementation of Gel constitutive model.
Deprecated interface functions.
DeprecatedCoreInterface Interface
virtual PetscErrorCode calculateStressAlpha()
Calculate stress in spring alpha.
ublas::matrix< TYPE, ublas::row_major, ublas::bounded_array< TYPE, 9 > > C
Cauchy deformation.
virtual PetscErrorCode calculateStrainTotal()
Calculate total strain.
ublas::matrix< TYPE, ublas::row_major, ublas::bounded_array< TYPE, 9 > > stressAlpha
Stress generated by spring alpha.
int main(int argc, char *argv[])
implementation of Data Operators for Forces and Sources
virtual PetscErrorCode calculateStressBetaHat()
Calculate stress due to concentration of solvent molecules.
ublas::matrix< TYPE, ublas::row_major, ublas::bounded_array< TYPE, 9 > > residualStrainHat
Residual for calculation epsilon hat.
virtual PetscErrorCode calculateSolventFlux()
Calculate flux.
Post-process fields on refined mesh.
ublas::matrix< TYPE, ublas::row_major, ublas::bounded_array< TYPE, 9 > > strainTotal
Total strain applied at integration point.
ublas::matrix< TYPE, ublas::row_major, ublas::bounded_array< TYPE, 9 > > strainHatDot
Internal variable, strain in dashpot beta.
virtual PetscErrorCode calculateStressBeta()
Calculate stress in spring beta.
Implementation of Gel finite element.
static MoFEMErrorCode Initialize(int *argc, char ***args, const char file[], const char help[])
Initializes the MoFEM database PETSc, MOAB and MPI.
#define CATCH_ERRORS
Catch errors.
virtual PetscErrorCode calculateTraceStrainTotalDot()
static MoFEMErrorCodeGeneric< PetscErrorCode > ierr
TYPE mU
Solvent concentration.
virtual PetscErrorCode calculateSolventConcentrationDot()
Calculate solvent concentration rate.
MatrixBoundedArray< double, 9 > MatrixDouble3by3
Constitutive model functions.
ublas::matrix< TYPE, ublas::row_major, ublas::bounded_array< TYPE, 9 > > stressBetaHat
Stress as result of volume change due to solvent concentration.
ublas::matrix< TYPE, ublas::row_major, ublas::bounded_array< TYPE, 9 > > FDot
Rate of gradient of deformation.
ublas::matrix< TYPE, ublas::row_major, ublas::bounded_array< TYPE, 9 > > strainHat
Internal variable, strain in dashpot beta.
ublas::matrix< TYPE, ublas::row_major, ublas::bounded_array< TYPE, 9 > > stressBeta
Stress generated by spring beta.
ublas::matrix< TYPE, ublas::row_major, ublas::bounded_array< TYPE, 9 > > F
Gradient of deformation.
virtual PetscErrorCode calculateResidualStrainHat()