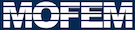 |
| v0.14.0
|
Atom test testing calculation of element residual vectors and tangent matrices.
More...
#include <MoFEM.hpp>
#include <PostProcOnRefMesh.hpp>
#include <Projection10NodeCoordsOnField.hpp>
#include <boost/numeric/ublas/vector_proxy.hpp>
#include <boost/numeric/ublas/matrix.hpp>
#include <boost/numeric/ublas/matrix_proxy.hpp>
#include <boost/numeric/ublas/vector.hpp>
#include <adolc/adolc.h>
#include <Gels.hpp>
#include <boost/iostreams/tee.hpp>
#include <boost/iostreams/stream.hpp>
#include <fstream>
#include <iostream>
Go to the source code of this file.
|
int | main (int argc, char *argv[]) |
|
|
static char | help [] = "...\n\n" |
|
Atom test testing calculation of element residual vectors and tangent matrices.
Definition in file gel_jacobian_test.cpp.
◆ main()
int main |
( |
int |
argc, |
|
|
char * |
argv[] |
|
) |
| |
Definition at line 43 of file gel_jacobian_test.cpp.
53 PetscBool flg = PETSC_TRUE;
56 if(flg != PETSC_TRUE) {
57 SETERRQ(PETSC_COMM_SELF,1,
"*** ERROR -my_file (MESH FILE NEEDED)");
62 ParallelComm* pcomm = ParallelComm::get_pcomm(&moab,
MYPCOMM_INDEX);
63 if(pcomm == NULL) pcomm =
new ParallelComm(&moab,PETSC_COMM_WORLD);
80 bool check_if_spatial_field_exist = m_field.
check_field(
"SPATIAL_POSITION");
105 if(flg != PETSC_TRUE) {
146 if (!check_if_spatial_field_exist) {
148 ierr = m_field.
loop_dofs(
"MESH_NODE_POSITIONS", ent_method_material); CHKERRQ(
ierr);
187 map<int,Gel::BlockMaterialData> &material_data = gel.blockMaterialData;
188 gel.constitutiveEquationPtr = boost::shared_ptr<Gel::ConstitutiveEquation<adouble> >(
193 material_data[0].gAlpha = 1;
194 material_data[0].vAlpha = 0.3;
195 material_data[0].gBeta = 1;
196 material_data[0].vBeta = 0.3;
197 material_data[0].gBetaHat = 1;
198 material_data[0].vBetaHat = 0.3;
199 material_data[0].oMega = 1;
200 material_data[0].vIscosity = 1;
201 material_data[0].pErmeability = 2.;
205 rval = moab.tag_get_handle(
206 "BLOCK_ID",1,MB_TYPE_INTEGER,th_block_id,MB_TAG_CREAT|MB_TAG_SPARSE,&def_block
212 common_data.
muName =
"CHEMICAL_LOAD";
213 common_data.
muNameDot =
"CHEMICAL_LOAD_DOT";
217 Gel::GelFE *fe_ptr[] = { &gel.feRhs, &gel.feLhs };
218 for(
int ss = 0;ss<2;ss++) {
234 gel.feRhs.getOpPtrVector().push_back(
235 new Gel::OpJacobian(
"SPATIAL_POSITION", tags,gel.constitutiveEquationPtr,gel.commonData,
true,
false)
237 gel.feRhs.getOpPtrVector().push_back(
240 gel.feRhs.getOpPtrVector().push_back(
243 gel.feRhs.getOpPtrVector().push_back(
246 gel.feRhs.getOpPtrVector().push_back(
250 gel.feLhs.getOpPtrVector().push_back(
251 new Gel::OpJacobian(
"SPATIAL_POSITION",tags,gel.constitutiveEquationPtr,gel.commonData,
false,
true)
253 gel.feLhs.getOpPtrVector().push_back(
256 gel.feLhs.getOpPtrVector().push_back(
259 gel.feLhs.getOpPtrVector().push_back(
262 gel.feLhs.getOpPtrVector().push_back(
265 gel.feLhs.getOpPtrVector().push_back(
268 gel.feLhs.getOpPtrVector().push_back(
271 gel.feLhs.getOpPtrVector().push_back(
279 DMType dm_name =
"DMGEL";
282 ierr = DMCreate(PETSC_COMM_WORLD,&dm);CHKERRQ(
ierr);
283 ierr = DMSetType(dm,dm_name);CHKERRQ(
ierr);
285 ierr = DMSetFromOptions(dm); CHKERRQ(
ierr);
299 ierr = VecGhostUpdateBegin(
F,INSERT_VALUES,SCATTER_FORWARD); CHKERRQ(
ierr);
300 ierr = VecGhostUpdateEnd(
F,INSERT_VALUES,SCATTER_FORWARD); CHKERRQ(
ierr);
301 ierr = VecZeroEntries(U_t); CHKERRQ(
ierr);
302 ierr = VecGhostUpdateBegin(U_t,INSERT_VALUES,SCATTER_FORWARD); CHKERRQ(
ierr);
303 ierr = VecGhostUpdateEnd(U_t,INSERT_VALUES,SCATTER_FORWARD); CHKERRQ(
ierr);
306 gel.feRhs.ts_u_t = U_t;
307 gel.feRhs.ts_ctx = TSMethod::CTX_TSSETIFUNCTION;
310 gel.feLhs.ts_a = 1.0;
311 gel.feLhs.ts_ctx = TSMethod::CTX_TSSETIJACOBIAN;
313 ierr = VecAssemblyBegin(
F); CHKERRQ(
ierr);
315 ierr = MatAssemblyBegin(
M,MAT_FINAL_ASSEMBLY); CHKERRQ(
ierr);
316 ierr = MatAssemblyEnd(
M,MAT_FINAL_ASSEMBLY); CHKERRQ(
ierr);
323 ierr = PetscViewerASCIIOpen(PETSC_COMM_WORLD,
"gel_jacobian_test.txt",&viewer); CHKERRQ(
ierr);
324 ierr = PetscViewerDestroy(&viewer); CHKERRQ(
ierr);
332 ierr = MatNorm(
M,NORM_1,&mnorm); CHKERRQ(
ierr);
333 ierr = PetscPrintf(PETSC_COMM_WORLD,
"mnorm = %9.8e\n",mnorm); CHKERRQ(
ierr);
335 if(fabs(mnorm-6.54443978e+01)>1e-6) {
342 ierr = VecDestroy(&U_t); CHKERRQ(
ierr);
344 ierr = DMDestroy(&dm); CHKERRQ(
ierr);
◆ help
#define MYPCOMM_INDEX
default communicator number PCOMM
virtual MoFEMErrorCode loop_dofs(const Problem *problem_ptr, const std::string &field_name, RowColData rc, DofMethod &method, int lower_rank, int upper_rank, int verb=DEFAULT_VERBOSITY)=0
Make a loop over dofs.
#define CHKERRQ_MOAB(a)
check error code of MoAB function
string spatialPositionName
virtual MoFEMErrorCode modify_finite_element_add_field_row(const std::string &fe_name, const std::string name_row)=0
set field row which finite element use
@ L2
field with C-1 continuity
Projection of edge entities with one mid-node on hierarchical basis.
static MoFEMErrorCode Finalize()
Checks for options to be called at the conclusion of the program.
string spatialPositionNameDot
virtual MoFEMErrorCode add_ents_to_field_by_type(const Range &ents, const EntityType type, const std::string &name, int verb=DEFAULT_VERBOSITY)=0
Add entities to field meshset.
PetscErrorCode DMMoFEMAddElement(DM dm, std::string fe_name)
add element to dm
Implementation of Gel constitutive model.
Deprecated interface functions.
DeprecatedCoreInterface Interface
PetscErrorCode DMCreateGlobalVector_MoFEM(DM dm, Vec *g)
DMShellSetCreateGlobalVector.
static MoFEMErrorCodeGeneric< moab::ErrorCode > rval
FTensor::Index< 'M', 3 > M
PetscErrorCode DMCreateMatrix_MoFEM(DM dm, Mat *M)
virtual MoFEMErrorCode add_finite_element(const std::string &fe_name, enum MoFEMTypes bh=MF_EXCL, int verb=DEFAULT_VERBOSITY)=0
add finite element
virtual MoFEMErrorCode add_ents_to_finite_element_by_type(const EntityHandle entities, const EntityType type, const std::string name, const bool recursive=true)=0
add entities to finite element
virtual MoFEMErrorCode modify_finite_element_add_field_col(const std::string &fe_name, const std::string name_row)=0
set field col which finite element use
virtual MoFEMErrorCode build_finite_elements(int verb=DEFAULT_VERBOSITY)=0
Build finite elements.
Common data for gel model.
PetscErrorCode DMRegister_MoFEM(const char sname[])
Register MoFEM problem.
virtual bool check_field(const std::string &name) const =0
check if field is in database
PetscErrorCode DMMoFEMCreateMoFEM(DM dm, MoFEM::Interface *m_field_ptr, const char problem_name[], const MoFEM::BitRefLevel bit_level, const MoFEM::BitRefLevel bit_mask=MoFEM::BitRefLevel().set())
Must be called by user to set MoFEM data structures.
virtual MoFEMErrorCode modify_finite_element_add_field_data(const std::string &fe_name, const std::string name_field)=0
set finite element field data
Calculating right hand side.
static MoFEMErrorCode Initialize(int *argc, char ***args, const char file[], const char help[])
Initializes the MoFEM database PETSc, MOAB and MPI.
#define CATCH_ERRORS
Catch errors.
static MoFEMErrorCodeGeneric< PetscErrorCode > ierr
@ AINSWORTH_LEGENDRE_BASE
Ainsworth Cole (Legendre) approx. base .
boost::ptr_deque< UserDataOperator > & getOpPtrVector()
Use to push back operator for row operator.
const FTensor::Tensor2< T, Dim, Dim > Vec
PetscErrorCode PetscOptionsGetString(PetscOptions *, const char pre[], const char name[], char str[], size_t size, PetscBool *set)
virtual MoFEMErrorCode build_fields(int verb=DEFAULT_VERBOSITY)=0
DEPRECATED MoFEMErrorCode seed_ref_level_3D(const EntityHandle meshset, const BitRefLevel &bit, int verb=-1)
seed 2D entities in the meshset and their adjacencies (only TETs adjacencies) in a particular BitRefL...
Assemble internal force vector.
Constitutive model functions.
std::bitset< BITREFLEVEL_SIZE > BitRefLevel
Bit structure attached to each entity identifying to what mesh entity is attached.
@ MOFEM_ATOM_TEST_INVALID
virtual MoFEMErrorCode build_adjacencies(const Range &ents, int verb=DEFAULT_VERBOSITY)=0
build adjacencies
virtual MoFEMErrorCode set_field_order(const EntityHandle meshset, const EntityType type, const std::string &name, const ApproximationOrder order, int verb=DEFAULT_VERBOSITY)=0
Set order approximation of the entities in the field.
PetscErrorCode DMoFEMLoopFiniteElements(DM dm, const char fe_name[], MoFEM::FEMethod *method, CacheTupleWeakPtr cache_ptr=CacheTupleSharedPtr())
Executes FEMethod for finite elements in DM.
PetscErrorCode PetscOptionsGetInt(PetscOptions *, const char pre[], const char name[], PetscInt *ivalue, PetscBool *set)
Calculate internal forces for solvent flux.
virtual MoFEMErrorCode add_field(const std::string name, const FieldSpace space, const FieldApproximationBase base, const FieldCoefficientsNumber nb_of_coefficients, const TagType tag_type=MB_TAG_SPARSE, const enum MoFEMTypes bh=MF_EXCL, int verb=DEFAULT_VERBOSITY)=0
Add field.
definition of volume element