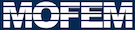 |
| v0.14.0
|
Go to the source code of this file.
|
int | main (int argc, char *argv[]) |
|
|
static char | help [] = "...\n\n" |
|
◆ main()
int main |
( |
int |
argc, |
|
|
char * |
argv[] |
|
) |
| |
< database
< interface
This has no real effect, folling line are only for atom test purpose
- Examples
- hdiv_divergence_operator.cpp.
Definition at line 44 of file hdiv_divergence_operator.cpp.
50 enum bases { AINSWORTH, DEMKOWICZ,
LASTOP };
52 const char *list[] = {
"ainsworth",
"demkowicz"};
55 PetscInt choice_value = AINSWORTH;
58 if (flg != PETSC_TRUE) {
62 PetscBool ho_geometry = PETSC_FALSE;
66 PetscInt ho_choice_value = AINSWORTH;
68 &ho_choice_value, &flg);
70 DMType dm_name =
"DMMOFEM";
87 switch (choice_value) {
102 if (ho_geometry == PETSC_TRUE) {
103 switch (ho_choice_value) {
113 constexpr
int order = 3;
115 if (ho_geometry == PETSC_TRUE)
120 pipeline_mng->getDomainLhsFE().reset();
121 pipeline_mng->getDomainRhsFE().reset();
122 pipeline_mng->getBoundaryLhsFE().reset();
123 pipeline_mng->getBoundaryRhsFE().reset();
124 pipeline_mng->getSkeletonLhsFE().reset();
125 pipeline_mng->getSkeletonRhsFE().reset();
131 double divergence_vol = 0;
132 double divergence_skin = 0;
134 auto jac_ptr = boost::make_shared<MatrixDouble>();
135 auto inv_jac_ptr = boost::make_shared<MatrixDouble>();
136 auto det_ptr = boost::make_shared<VectorDouble>();
137 pipeline_mng->getOpDomainRhsPipeline().push_back(
139 pipeline_mng->getOpDomainRhsPipeline().push_back(
141 pipeline_mng->getOpDomainRhsPipeline().push_back(
143 pipeline_mng->getOpDomainRhsPipeline().push_back(
145 pipeline_mng->getOpDomainRhsPipeline().push_back(
147 pipeline_mng->getOpDomainRhsPipeline().push_back(
151 pipeline_mng->getOpBoundaryRhsPipeline().push_back(
153 pipeline_mng->getOpBoundaryRhsPipeline().push_back(
155 pipeline_mng->getOpBoundaryRhsPipeline().push_back(
159 if (ho_geometry == PETSC_TRUE) {
163 CHKERR pipeline_mng->loopFiniteElements();
166 <<
"divergence_vol " << std::setprecision(12) << divergence_vol;
168 <<
"divergence_skin " << std::setprecision(12) << divergence_skin;
170 constexpr
double eps = 1e-8;
171 const double error = divergence_skin - divergence_vol;
172 if (fabs(error) >
eps)
174 "invalid surface flux or divergence or both error = %3.4e",
◆ help
MoFEMErrorCode getInterface(IFACE *&iface) const
Get interface reference to pointer of interface.
virtual MoFEMErrorCode loop_dofs(const Problem *problem_ptr, const std::string &field_name, RowColData rc, DofMethod &method, int lower_rank, int upper_rank, int verb=DEFAULT_VERBOSITY)=0
Make a loop over dofs.
MoFEMErrorCode addDataField(const std::string name, const FieldSpace space, const FieldApproximationBase base, const FieldCoefficientsNumber nb_of_coefficients, const TagType tag_type=MB_TAG_SPARSE, const enum MoFEMTypes bh=MF_ZERO, int verb=-1)
Add field on domain.
MoFEMErrorCode loadFile(const std::string options, const std::string mesh_file_name, LoadFileFunc loadFunc=defaultLoadFileFunc)
Load mesh file.
PipelineManager interface.
Projection of edge entities with one mid-node on hierarchical basis.
static MoFEMErrorCode Finalize()
Checks for options to be called at the conclusion of the program.
Simple interface for fast problem set-up.
Deprecated interface functions.
DeprecatedCoreInterface Interface
MoFEMErrorCode getOptions()
get options
#define CHKERR
Inline error check.
PetscErrorCode DMRegister_MoFEM(const char sname[])
Register MoFEM problem.
MoFEMErrorCode addBoundaryField(const std::string name, const FieldSpace space, const FieldApproximationBase base, const FieldCoefficientsNumber nb_of_coefficients, const TagType tag_type=MB_TAG_SPARSE, const enum MoFEMTypes bh=MF_ZERO, int verb=-1)
Add field on boundary.
Calculate normals at Gauss points of triangle element.
virtual bool check_field(const std::string &name) const =0
check if field is in database
MoFEMErrorCode setFieldOrder(const std::string field_name, const int order, const Range *ents=NULL)
Set field order.
static MoFEMErrorCode Initialize(int *argc, char ***args, const char file[], const char help[])
Initializes the MoFEM database PETSc, MOAB and MPI.
#define MOFEM_LOG(channel, severity)
Log.
#define CATCH_ERRORS
Catch errors.
Set inverse jacobian to base functions.
@ AINSWORTH_LEGENDRE_BASE
Ainsworth Cole (Legendre) approx. base .
PetscErrorCode PetscOptionsGetEList(PetscOptions *, const char pre[], const char name[], const char *const *list, PetscInt next, PetscInt *value, PetscBool *set)
transform local reference derivatives of shape function to global derivatives if higher order geometr...
@ MOFEM_ATOM_TEST_INVALID
MoFEMErrorCode setUp(const PetscBool is_partitioned=PETSC_TRUE)
Setup problem.
@ HDIV
field with continuous normal traction
MoFEMErrorCode addDomainField(const std::string name, const FieldSpace space, const FieldApproximationBase base, const FieldCoefficientsNumber nb_of_coefficients, const TagType tag_type=MB_TAG_SPARSE, const enum MoFEMTypes bh=MF_ZERO, int verb=-1)
Add field on domain.
PetscErrorCode PetscOptionsGetBool(PetscOptions *, const char pre[], const char name[], PetscBool *bval, PetscBool *set)