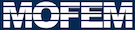 |
| v0.14.0
|
Go to the documentation of this file.
11 using namespace MoFEM;
13 static char help[] =
"...\n\n";
15 int main(
int argc,
char *argv[]) {
22 PetscBool flg_file = PETSC_FALSE;
23 char mesh_out_file[255] =
"out.h5m";
25 CHKERR PetscOptionsBegin(PETSC_COMM_WORLD,
"",
"Field to vertices options",
27 CHKERR PetscOptionsString(
"-file_name",
"mesh file name",
"",
"mesh.h5m",
29 CHKERR PetscOptionsString(
"-output_file",
"output mesh file name",
"",
30 "out.h5m", mesh_out_file, 255, PETSC_NULL);
36 ParallelComm *pcomm = ParallelComm::get_pcomm(&moab,
MYPCOMM_INDEX);
38 pcomm =
new ParallelComm(&moab, PETSC_COMM_WORLD);
48 auto core_log = logging::core::get();
55 std::vector<std::string> prb_list;
56 for(
auto &it : *prb_ptr)
57 prb_list.push_back(it.getName());
59 for (
auto &it : prb_list) {
60 MOFEM_LOG(
"REMOVER", Sev::inform) <<
"Delete problem " << it;
65 std::vector<std::string> fe_list;
66 for (
auto &it : *fe_ptr)
67 fe_list.push_back(it->getName());
69 for (
auto &it : fe_list) {
71 <<
"Delete finite element " << it;
76 std::vector<std::string> field_list;
77 for (
auto &it : *field_ptr)
78 field_list.push_back(it->getName());
80 for (
auto &it : field_list) {
81 MOFEM_LOG(
"REMOVER", Sev::inform) <<
"Delete field " << it;
85 CHKERR moab.write_file(mesh_out_file);
#define MYPCOMM_INDEX
default communicator number PCOMM
virtual const FiniteElement_multiIndex * get_finite_elements() const =0
Get the finite elements object.
static MoFEMErrorCode Finalize()
Checks for options to be called at the conclusion of the program.
static boost::shared_ptr< SinkType > createSink(boost::shared_ptr< std::ostream > stream_ptr, std::string comm_filter)
Create a sink object.
Deprecated interface functions.
DeprecatedCoreInterface Interface
#define CHKERR
Inline error check.
implementation of Data Operators for Forces and Sources
virtual MoFEMErrorCode delete_field(const std::string name, int verb=DEFAULT_VERBOSITY)=0
Delete field.
static boost::shared_ptr< std::ostream > getStrmWorld()
Get the strm world object.
virtual MoFEMErrorCode delete_finite_element(const std::string name, int verb=DEFAULT_VERBOSITY)=0
delete finite element from mofem database
#define MOFEM_LOG_TAG(channel, tag)
Tag channel.
virtual const Problem_multiIndex * get_problems() const =0
Get the problems object.
virtual const Field_multiIndex * get_fields() const =0
Get the fields object.
static MoFEMErrorCode Initialize(int *argc, char ***args, const char file[], const char help[])
Initializes the MoFEM database PETSc, MOAB and MPI.
#define MOFEM_LOG(channel, severity)
Log.
#define CATCH_ERRORS
Catch errors.
static MoFEMErrorCodeGeneric< PetscErrorCode > ierr
static LoggerType & setLog(const std::string channel)
Set ans resset chanel logger.
virtual MoFEMErrorCode delete_problem(const std::string name)=0
Delete problem.
#define CHKERRG(n)
Check error code of MoFEM/MOAB/PETSc function.
int main(int argc, char *argv[])