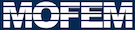 |
| v0.14.0
|
Go to the documentation of this file.
19 #ifndef __CP_CUTMESH_HPP__
20 #define __CP_CUTMESH_HPP__
67 const int verb =
QUIET,
const bool debug =
false);
81 const std::string save_mesh =
"",
82 const int verb =
QUIET,
100 const bool debug =
false,
101 std::string file_name =
"out_body_skin.vtk");
104 const bool debug =
false);
108 const bool debug =
false);
113 const bool debug =
false);
120 const bool debug =
false);
124 const bool debug =
false);
128 const bool debug =
false);
149 const bool debug =
false);
215 #endif //__CP_CUTMESH_HPP__
const Range & getCuttingSurface() const
MoFEMErrorCode getInterfacesPtr()
MoFEMErrorCode refineCrackTip(const int front_id, const int verb=1, const bool debug=false)
refine elements at crack tip
MoFEMErrorCode findCrackFromPrisms(const BitRefLevel bit, const int verb=1, const bool debug=false)
get crack front
MoFEMErrorCode rebuildCrackSurface(const double factor, const std::string save_mesh="", const int verb=QUIET, bool debug=false)
MoFEMErrorCode cutMesh(int &first_bit, const bool debug=false)
Ref, cut and trim, merge and do TetGen, if option is on.
MoFEMErrorCode refineMesh(const Range *vol, const bool make_front, const int verb=QUIET, const bool debug=false)
Refine mesh close to crack surface and crack front.
PetscErrorCode MoFEMErrorCode
MoFEM/PETSc error code.
MoFEMErrorCode cutRefineAndSplit(const int verb=VERBOSE, bool debug=false)
Refine, cut, trim, merge and ref front and split.
MoFEMErrorCode copySurface(const std::string save_mesh="")
MoFEMErrorCode splitFaces(const int verb=1, const bool debug=false)
split crack faces
std::vector< double > originalCoords
MoFEMErrorCode getOptions()
Get options from command line.
MoFEMErrorCode setCutSurfaceFromFile()
Set the cut surface from file.
MoFEMErrorCode insertContactInterface(const int verb=1, const bool debug=false)
insert contact interface
int getCrackFrontId() const
MoFEMErrorCode query_interface(boost::typeindex::type_index type_index, MoFEM::UnknownInterface **iface) const
Getting interface of core database.
std::vector< double > & getOrgCoords()
const std::string & getCutSurfMeshName() const
MoFEMErrorCode setMeshOrgCoords()
int contactPrismsBlockSetId
MoFEMErrorCode setFixEdgesAndCorners(const BitRefLevel bit)
PetscBool removePathologicalFrontTris
MoFEMErrorCode findBodySkin(const BitRefLevel bit, const int verb=1, const bool debug=false, std::string file_name="out_body_skin.vtk")
find body skin
const std::vector< double > & getOrgCoords() const
int cuttingSurfaceSidesetId
MoFEMErrorCode findCrack(const BitRefLevel bit, const int verb=1, const bool debug=false)
get crack front
MeshsetsManager * meshsetMngPtr
const Range & getCornerNodes() const
int getSkinOfTheBodyId() const
MoFEMErrorCode refineAndSplit(const int verb=1, const bool debug=false)
MoFEMErrorCode getMeshOrgCoords()
CutMeshInterface * cutMeshPtr
int crackedBodyBlockSetId
base class for all interface classes
const Range & getFixedEdges() const
std::string cutSurfMeshName
MoFEMErrorCode getFrontEdgesAndElements(const BitRefLevel bit, const int verb=1, const bool debug=false)
get crack front edges and finite elements
MoFEMErrorCode findContactFromPrisms(const BitRefLevel bit, const int verb=1, const bool debug=false)
get contact elements
CPMeshCut(CrackPropagation &cp)
BitRefManager * bitRefPtr
int getContactPrismsBlockSetId() const
int getCrackSurfaceId() const
MoFEMErrorCode addMortarContactPrisms(const int verb=1, const bool debug=false)
insert contact interface
PetscBool edgesBlockSetFlg
MoFEMErrorCode setVolume(const BitRefLevel &bit)
Set cutting volume from bit ref level.
std::bitset< BITREFLEVEL_SIZE > BitRefLevel
Bit structure attached to each entity identifying to what mesh entity is attached.
int getCuttingSurfaceSidesetId() const
const int getEdgesBlockSet() const
MoFEMErrorCode clearData()