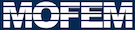 |
| v0.14.0
|
#include <users_modules/fracture_mechanics/src/CrackPropagation.hpp>
|
MoFEMErrorCode | getInterfaceVersion (Version &version) const |
|
MoFEMErrorCode | query_interface (boost::typeindex::type_index type_index, UnknownInterface **iface) const |
| Getting interface of core database. More...
|
|
| CrackPropagation (MoFEM::Interface &m_field, const int approx_order=2, const int geometry_order=1) |
| Constructor of Crack Propagation, prints fracture module version and registers the three interfaces used to solve fracture problem, i.e. CrackPropagation, CPSolvers and CPMeshCut. More...
|
|
virtual | ~CrackPropagation () |
|
MoFEMErrorCode | getOptions () |
| Get options form command line. More...
|
|
MoFEMErrorCode | tetsingReleaseEnergyCalculation () |
| This is run with ctest. More...
|
|
int & | getNbLoadSteps () |
|
int | getNbLoadSteps () const |
|
double & | getLoadScale () |
|
double | getLoadScale () const |
|
int & | getNbCutSteps () |
|
int | getNbCutSteps () const |
|
MoFEMErrorCode | readMedFile () |
| read mesh file More...
|
|
MoFEMErrorCode | saveEachPart (const std::string prefix, const Range &ents) |
| Save entities on ech processor. More...
|
|
virtual MoFEMErrorCode | query_interface (boost::typeindex::type_index type_index, UnknownInterface **iface) const =0 |
|
template<class IFACE > |
MoFEMErrorCode | registerInterface (bool error_if_registration_failed=true) |
| Register interface. More...
|
|
template<class IFACE > |
MoFEMErrorCode | getInterface (IFACE *&iface) const |
| Get interface refernce to pointer of interface. More...
|
|
template<class IFACE > |
MoFEMErrorCode | getInterface (IFACE **const iface) const |
| Get interface pointer to pointer of interface. More...
|
|
template<class IFACE , typename boost::enable_if< boost::is_pointer< IFACE >, int >::type = 0> |
IFACE | getInterface () const |
| Get interface pointer to pointer of interface. More...
|
|
template<class IFACE , typename boost::enable_if< boost::is_reference< IFACE >, int >::type = 0> |
IFACE | getInterface () const |
| Get reference to interface. More...
|
|
template<class IFACE > |
IFACE * | getInterface () const |
| Function returning pointer to interface. More...
|
|
virtual | ~UnknownInterface ()=default |
|
|
MoFEMErrorCode | buildProblemFields (const BitRefLevel &bit1, const BitRefLevel &mask1, const BitRefLevel &bit2, const int verb=QUIET, const bool debug=false) |
| Build problem fields. More...
|
|
MoFEMErrorCode | buildProblemFiniteElements (BitRefLevel bit1, BitRefLevel bit2, std::vector< int > surface_ids, const int verb=QUIET, const bool debug=false) |
| Build problem finite elements. More...
|
|
MoFEMErrorCode | createProblemDataStructures (const std::vector< int > surface_ids, const int verb=QUIET, const bool debug=false) |
| Construct problem data structures. More...
|
|
MoFEMErrorCode | createDMs (SmartPetscObj< DM > &dm_elastic, SmartPetscObj< DM > &dm_eigen_elastic, SmartPetscObj< DM > &dm_material, SmartPetscObj< DM > &dm_crack_propagation, SmartPetscObj< DM > &dm_material_forces, SmartPetscObj< DM > &dm_surface_projection, SmartPetscObj< DM > &dm_crack_srf_area, std::vector< int > surface_ids, std::vector< std::string > fe_surf_proj_list) |
| Crate DMs for all problems and sub problems. More...
|
|
MoFEMErrorCode | deleteEntities (const int verb=QUIET, const bool debug=false) |
|
|
MoFEMErrorCode | buildElasticFields (const BitRefLevel bit, const BitRefLevel mask=BitRefLevel().set(), const bool proc_only=true, const bool build_fields=true, const int verb=QUIET, const bool debug=false) |
| Declate fields for elastic analysis. More...
|
|
MoFEMErrorCode | buildArcLengthField (const BitRefLevel bit, const bool build_fields=true, const int verb=QUIET) |
| Declate field for arc-length. More...
|
|
MoFEMErrorCode | buildSurfaceFields (const BitRefLevel bit, const bool proc_only=true, const bool build_fields=true, const int verb=QUIET, const bool debug=false) |
| build fields with Lagrange multipliers to constrain surfaces More...
|
|
MoFEMErrorCode | buildEdgeFields (const BitRefLevel bit, const bool proc_only=true, const bool build_fields=true, const int verb=QUIET, const bool debug=false) |
| build fields with Lagrange multipliers to constrain edges More...
|
|
MoFEMErrorCode | buildCrackSurfaceFieldId (const BitRefLevel bit, const bool proc_only=true, const bool build_fields=true, const int verb=QUIET, const bool debug=false) |
| declare crack surface files More...
|
|
MoFEMErrorCode | buildCrackFrontFieldId (const BitRefLevel bit, const bool build_fields=true, const int verb=QUIET, const bool debug=false) |
| declare crack surface files More...
|
|
MoFEMErrorCode | buildBothSidesFieldId (const BitRefLevel bit_spatial, const BitRefLevel bit_material, const bool proc_only=false, const bool build_fields=true, const int verb=QUIET, const bool debug=false) |
| Lagrange multipliers field which constrains material displacements. More...
|
|
MoFEMErrorCode | zeroLambdaFields () |
| Zero fields with lagrange multipliers. More...
|
|
|
MoFEMErrorCode | declareElasticFE (const BitRefLevel bit1, const BitRefLevel mask1, const BitRefLevel bit2, const BitRefLevel mask2, const bool add_forces=true, const bool proc_only=true, const int verb=QUIET) |
| declare elastic finite elements More...
|
|
MoFEMErrorCode | declareArcLengthFE (const BitRefLevel bits, const int verb=QUIET) |
| create arc-length element entity and declare elemets More...
|
|
MoFEMErrorCode | declareExternalForcesFE (const BitRefLevel bit, const BitRefLevel mask=BitRefLevel().set(), const bool proc_only=true) |
|
MoFEMErrorCode | declareSurfaceForceAleFE (const BitRefLevel bit, const BitRefLevel mask=BitRefLevel().set(), const bool proc_only=true) |
| Declare FE for pressure BC in ALE formulation (in material domain) More...
|
|
MoFEMErrorCode | declarePressureAleFE (const BitRefLevel bit, const BitRefLevel mask=BitRefLevel().set(), const bool proc_only=true) |
| Declare FE for pressure BC in ALE formulation (in material domain) More...
|
|
MoFEMErrorCode | declareSpringsAleFE (const BitRefLevel bit, const BitRefLevel mask=BitRefLevel().set(), const bool proc_only=true) |
| Declare FE for spring BC in ALE formulation (in material domain) More...
|
|
MoFEMErrorCode | declareSimpleContactAleFE (const BitRefLevel bit, const BitRefLevel mask=BitRefLevel().set(), const bool proc_only=true) |
| Declare FE for pressure BC in ALE formulation (in material domain) More...
|
|
MoFEMErrorCode | declareMaterialFE (const BitRefLevel bit, const BitRefLevel mask=BitRefLevel().set(), const bool proc_only=true, const bool verb=QUIET) |
| declare material finite elements More...
|
|
MoFEMErrorCode | declareFrontFE (const BitRefLevel bit, const BitRefLevel mask=BitRefLevel().set(), const bool proc_only=true, const bool verb=QUIET) |
|
MoFEMErrorCode | declareBothSidesFE (const BitRefLevel bit_spatial, const BitRefLevel bit_material, const BitRefLevel mask=BitRefLevel().set(), const bool proc_only=true, const bool verb=QUIET) |
|
MoFEMErrorCode | declareSmoothingFE (const BitRefLevel bit, const BitRefLevel mask=BitRefLevel().set(), const bool proc_only=true, const bool verb=QUIET) |
| declare mesh smoothing finite elements More...
|
|
MoFEMErrorCode | declareSurfaceFE (std::string fe_name, const BitRefLevel bit, const BitRefLevel mask, const std::vector< int > &ids, const bool proc_only=true, const int verb=QUIET, const bool debug=false) |
| declare surface sliding elements More...
|
|
MoFEMErrorCode | declareEdgeFE (std::string fe_name, const BitRefLevel bit, const BitRefLevel mask, const bool proc_only=true, const int verb=QUIET, const bool debug=false) |
|
MoFEMErrorCode | declareCrackSurfaceFE (std::string fe_name, const BitRefLevel bit, const BitRefLevel mask, const bool proc_only=true, const int verb=QUIET, const bool debug=false) |
|
|
MoFEMErrorCode | createCrackPropagationDM (SmartPetscObj< DM > &dm, const std::string prb_name, SmartPetscObj< DM > dm_elastic, SmartPetscObj< DM > dm_material, const BitRefLevel bit, const BitRefLevel mask, const std::vector< std::string > fe_list) |
| Create DM by composition of elastic DM and material DM. More...
|
|
MoFEMErrorCode | createElasticDM (SmartPetscObj< DM > &dm, const std::string prb_name, const BitRefLevel bit, const BitRefLevel mask=BitRefLevel().set()) |
| Create elastic problem DM. More...
|
|
MoFEMErrorCode | createEigenElasticDM (SmartPetscObj< DM > &dm, const std::string prb_name, const BitRefLevel bit, const BitRefLevel mask=BitRefLevel().set()) |
| Create elastic problem DM. More...
|
|
MoFEMErrorCode | createBcDM (SmartPetscObj< DM > &dm, const std::string prb_name, const BitRefLevel bit, const BitRefLevel mask=BitRefLevel().set()) |
| Create problem to calculate boundary conditions. More...
|
|
MoFEMErrorCode | createMaterialDM (SmartPetscObj< DM > &dm, const std::string prb_name, const BitRefLevel bit, const BitRefLevel mask, const std::vector< std::string > fe_list, const bool debug=false) |
| Create DM fto calculate material problem. More...
|
|
MoFEMErrorCode | createMaterialForcesDM (SmartPetscObj< DM > &dm, SmartPetscObj< DM > dm_material, const std::string prb_name, const int verb=QUIET) |
| Create DM for calculation of material forces (sub DM of DM material) More...
|
|
MoFEMErrorCode | createSurfaceProjectionDM (SmartPetscObj< DM > &dm, SmartPetscObj< DM > dm_material, const std::string prb_name, const std::vector< int > surface_ids, const std::vector< std::string > fe_list, const int verb=QUIET) |
| create DM to calculate projection matrices (sub DM of DM material) More...
|
|
MoFEMErrorCode | createCrackFrontAreaDM (SmartPetscObj< DM > &dm, SmartPetscObj< DM > dm_material, const std::string prb_name, const bool verb=QUIET) |
| create DM to calculate Griffith energy More...
|
|
|
MoFEMErrorCode | assembleElasticDM (const std::string mwls_stress_tag_name, const int verb=QUIET, const bool debug=false) |
| create elastic finite element instance for spatial assembly More...
|
|
MoFEMErrorCode | assembleElasticDM (const int verb=QUIET, const bool debug=false) |
| create elastic finite element instance for spatial assembly More...
|
|
MoFEMErrorCode | addElasticFEInstancesToSnes (DM dm, Mat m, Vec q, Vec f, boost::shared_ptr< FEMethod > arc_method=boost::shared_ptr< FEMethod >(), boost::shared_ptr< ArcLengthCtx > arc_ctx=nullptr, const int verb=QUIET, const bool debug=false) |
|
MoFEMErrorCode | assembleMaterialForcesDM (DM dm, const int verb=QUIET, const bool debug=false) |
| create material element instance More...
|
|
MoFEMErrorCode | assembleSmootherForcesDM (DM dm, const std::vector< int > ids, const int verb=QUIET, const bool debug=false) |
| create smoothing element instance More...
|
|
MoFEMErrorCode | assembleCouplingForcesDM (DM dm, const int verb=QUIET, const bool debug=false) |
| assemble coupling element instances More...
|
|
MoFEMErrorCode | addMaterialFEInstancesToSnes (DM dm, const bool fix_crack_front, const int verb=QUIET, const bool debug=false) |
| add material elements instances to SNES More...
|
|
MoFEMErrorCode | addSmoothingFEInstancesToSnes (DM dm, const bool fix_crack_front, const int verb=QUIET, const bool debug=false) |
| add softening elements instances to SNES More...
|
|
MoFEMErrorCode | addPropagationFEInstancesToSnes (DM dm, boost::shared_ptr< FEMethod > arc_method, boost::shared_ptr< ArcLengthCtx > arc_ctx, const std::vector< int > &surface_ids, const int verb=QUIET, const bool debug=false) |
| add finite element to SNES for crack propagation problem More...
|
|
MoFEMErrorCode | testJacobians (const BitRefLevel bit, const BitRefLevel mask, tangent_tests test) |
| test LHS Jacobians More...
|
|
MoFEMErrorCode | addMWLSStressOperators (boost::shared_ptr< CrackFrontElement > &fe_rhs, boost::shared_ptr< CrackFrontElement > &fe_lhs) |
|
MoFEMErrorCode | addMWLSDensityOperators (boost::shared_ptr< CrackFrontElement > &fe_rhs, boost::shared_ptr< CrackFrontElement > &fe_lhs) |
|
MoFEMErrorCode | updateMaterialFixedNode (const bool fix_front, const bool fix_small_g, const bool debug=false) |
| Update fixed nodes. More...
|
|
|
MoFEMErrorCode | calculateElasticEnergy (DM dm, const std::string msg="") |
| assemble elastic part of matrix, by running elastic finite element instance More...
|
|
MoFEMErrorCode | calculateMaterialForcesDM (DM dm, Vec q, Vec f, const int verb=QUIET, const bool debug=false) |
| assemble material forces, by running material finite element instance More...
|
|
MoFEMErrorCode | calculateSmoothingForcesDM (DM dm, Vec q, Vec f, const int verb=QUIET, const bool debug=false) |
| assemble smoothing forces, by running material finite element instance More...
|
|
MoFEMErrorCode | calculateSmoothingForceFactor (const int verb=QUIET, const bool debug=true) |
|
MoFEMErrorCode | calculateSurfaceProjectionMatrix (DM dm_front, DM dm_project, const std::vector< int > &ids, const int verb=QUIET, const bool debug=false) |
| assemble projection matrices More...
|
|
MoFEMErrorCode | calculateFrontProjectionMatrix (DM dm_surface, DM dm_project, const int verb=QUIET, const bool debug=false) |
| assemble crack front projection matrix (that constrains crack area growth) More...
|
|
MoFEMErrorCode | projectMaterialForcesDM (DM dm_project, Vec f, Vec f_proj, const int verb=QUIET, const bool debug=false) |
| project material forces along the crack elongation direction More...
|
|
MoFEMErrorCode | calculateGriffithForce (DM dm, const double gc, Vec f_griffith, const int verb=QUIET, const bool debug=false) |
| calculate Griffith (driving) force More...
|
|
MoFEMErrorCode | projectGriffithForce (DM dm, Vec f_griffith, Vec f_griffith_proj, const int verb=QUIET, const bool debug=false) |
| project Griffith forces More...
|
|
MoFEMErrorCode | calculateReleaseEnergy (DM dm, Vec f_material_proj, Vec f_griffith_proj, Vec f_lambda, const double gc, const int verb=QUIET, const bool debug=true) |
| calculate release energy More...
|
|
|
MoFEMErrorCode | solveElasticDM (DM dm, SNES snes, Mat m, Vec q, Vec f, bool snes_set_up, Mat *shell_m) |
| solve elastic problem More...
|
|
MoFEMErrorCode | solvePropagationDM (DM dm, DM dm_elastic, SNES snes, Mat m, Vec q, Vec f) |
| solve crack propagation problem More...
|
|
|
bool | onlyHooke |
| True if only Hooke material is applied. More...
|
|
PetscBool | onlyHookeFromOptions |
| True if only Hooke material is applied. More...
|
|
boost::shared_ptr< NonlinearElasticElement > | elasticFe |
|
boost::shared_ptr< NonlinearElasticElement > | materialFe |
|
boost::shared_ptr< CrackFrontElement > | feLhs |
| Integrate elastic FE. More...
|
|
boost::shared_ptr< CrackFrontElement > | feRhs |
| Integrate elastic FE. More...
|
|
boost::shared_ptr< CrackFrontElement > | feMaterialRhs |
| Integrate material stresses, assemble vector. More...
|
|
boost::shared_ptr< CrackFrontElement > | feMaterialLhs |
| Integrate material stresses, assemble matrix. More...
|
|
boost::shared_ptr< CrackFrontElement > | feEnergy |
| Integrate energy. More...
|
|
boost::shared_ptr< ConstrainMatrixCtx > | projSurfaceCtx |
| Data structure to project on the body surface. More...
|
|
boost::shared_ptr< ConstrainMatrixCtx > | projFrontCtx |
| Data structure to project on crack front. More...
|
|
boost::shared_ptr< MWLSApprox > | mwlsApprox |
|
boost::shared_ptr< NeumannForcesSurface::MyTriangleFE > | feMaterialAnaliticalTraction |
| Surface elment to calculate tractions in material space. More...
|
|
boost::shared_ptr< DirichletSpatialPositionsBc > | spatialDirichletBc |
| apply Dirichlet BC to sparial positions More...
|
|
boost::shared_ptr< AnalyticalDirichletBC::DirichletBC > | analyticalDirichletBc |
|
boost::shared_ptr< boost::ptr_map< string, NeumannForcesSurface > > | surfaceForces |
| assemble surface forces More...
|
|
boost::shared_ptr< boost::ptr_map< string, NeumannForcesSurface > > | surfaceForceAle |
| assemble surface pressure (ALE) More...
|
|
boost::shared_ptr< NeumannForcesSurface::DataAtIntegrationPts > | commonDataSurfaceForceAle |
| common data at integration points (ALE) More...
|
|
boost::shared_ptr< boost::ptr_map< string, NeumannForcesSurface > > | surfacePressure |
| assemble surface pressure More...
|
|
boost::shared_ptr< boost::ptr_map< string, NeumannForcesSurface > > | surfacePressureAle |
| assemble surface pressure (ALE) More...
|
|
boost::shared_ptr< NeumannForcesSurface::DataAtIntegrationPts > | commonDataSurfacePressureAle |
| common data at integration points (ALE) More...
|
|
boost::shared_ptr< boost::ptr_map< string, EdgeForce > > | edgeForces |
| assemble edge forces More...
|
|
boost::shared_ptr< boost::ptr_map< string, NodalForce > > | nodalForces |
| assemble nodal forces More...
|
|
boost::shared_ptr< FEMethod > | assembleFlambda |
| assemble F_lambda vector More...
|
|
boost::shared_ptr< FEMethod > | zeroFlambda |
| assemble F_lambda vector More...
|
|
boost::shared_ptr< CrackFrontElement > | feCouplingElasticLhs |
| FE instance to assemble coupling terms. More...
|
|
boost::shared_ptr< CrackFrontElement > | feCouplingMaterialLhs |
| FE instance to assemble coupling terms. More...
|
|
boost::shared_ptr< Smoother > | smootherFe |
|
boost::shared_ptr< Smoother::MyVolumeFE > | feSmootherRhs |
| Integrate smoothing operators. More...
|
|
boost::shared_ptr< Smoother::MyVolumeFE > | feSmootherLhs |
| Integrate smoothing operators. More...
|
|
boost::shared_ptr< VolumeLengthQuality< double > > | volumeLengthDouble |
|
boost::shared_ptr< VolumeLengthQuality< adouble > > | volumeLengthAdouble |
|
boost::shared_ptr< ObosleteUsersModules::TangentWithMeshSmoothingFrontConstrain > | tangentConstrains |
| Constrains crack front in tangent directiona. More...
|
|
boost::shared_ptr< SurfaceSlidingConstrains::DriverElementOrientation > | skinOrientation |
|
boost::shared_ptr< SurfaceSlidingConstrains::DriverElementOrientation > | crackOrientation |
|
boost::shared_ptr< SurfaceSlidingConstrains::DriverElementOrientation > | contactOrientation |
|
map< int, boost::shared_ptr< SurfaceSlidingConstrains > > | surfaceConstrain |
|
map< int, boost::shared_ptr< EdgeSlidingConstrains > > | edgeConstrains |
|
boost::shared_ptr< BothSurfaceConstrains > | bothSidesConstrains |
|
boost::shared_ptr< BothSurfaceConstrains > | closeCrackConstrains |
|
boost::shared_ptr< BothSurfaceConstrains > | bothSidesContactConstrains |
|
boost::shared_ptr< DirichletFixFieldAtEntitiesBc > | fixMaterialEnts |
|
boost::shared_ptr< GriffithForceElement > | griffithForceElement |
|
boost::shared_ptr< GriffithForceElement::MyTriangleFE > | feGriffithForceRhs |
|
boost::shared_ptr< GriffithForceElement::MyTriangleFE > | feGriffithForceLhs |
|
boost::shared_ptr< GriffithForceElement::MyTriangleFEConstrainsDelta > | feGriffithConstrainsDelta |
|
boost::shared_ptr< GriffithForceElement::MyTriangleFEConstrains > | feGriffithConstrainsRhs |
|
boost::shared_ptr< GriffithForceElement::MyTriangleFEConstrains > | feGriffithConstrainsLhs |
|
boost::shared_ptr< boost::ptr_map< string, NeumannForcesSurface > > | analiticalSurfaceElement |
|
boost::shared_ptr< FaceElementForcesAndSourcesCore > | feSpringLhsPtr |
|
boost::shared_ptr< FaceElementForcesAndSourcesCore > | feSpringRhsPtr |
|
Crack propagation data and methods
- Functions strarting with build, for example buildElastic, add and build fields.
- Functions starting with declare, for example declareElasticFE, add and build finite elements.
- Functions starting with assemble, for example assembleElasticDM, create finite element instances and operators.
- Functions starting with create, for example createElasticDM, create problems.
Definition at line 77 of file CrackPropagation.hpp.
◆ CrackPropagation()
FractureMechanics::CrackPropagation::CrackPropagation |
( |
MoFEM::Interface & |
m_field, |
|
|
const int |
approx_order = 2 , |
|
|
const int |
geometry_order = 1 |
|
) |
| |
Constructor of Crack Propagation, prints fracture module version and registers the three interfaces used to solve fracture problem, i.e. CrackPropagation, CPSolvers and CPMeshCut.
- Parameters
-
m_field | interface to MoAB database |
approx_order | order of approximation space, default is 2 |
geometry_order | order of geometry, default is 1 (can be up to 2) |
Definition at line 521 of file CrackPropagation.cpp.
535 if (!LogManager::checkIfChannelExist(
"CPWorld")) {
536 auto core_log = logging::core::get();
539 LogManager::createSink(LogManager::getStrmWorld(),
"CPWorld"));
541 LogManager::createSink(LogManager::getStrmSync(),
"CPSync"));
543 LogManager::createSink(LogManager::getStrmSelf(),
"CPSelf"));
545 LogManager::setLog(
"CPWorld");
546 LogManager::setLog(
"CPSync");
547 LogManager::setLog(
"CPSelf");
554 MOFEM_LOG(
"CPWorld", Sev::noisy) <<
"CPSolve created";
556 #ifdef GIT_UM_SHA1_NAME
557 MOFEM_LOG_C(
"CPWorld", Sev::inform,
"User module git commit id %s",
564 <<
"Fracture module version " << version.
strVersion();
566 #ifdef GIT_FM_SHA1_NAME
567 MOFEM_LOG_C(
"CPWorld", Sev::inform,
"Fracture module git commit id %s",
571 ierr = registerInterface<CrackPropagation>();
572 CHKERRABORT(PETSC_COMM_SELF,
ierr);
573 ierr = registerInterface<CPSolvers>();
574 CHKERRABORT(PETSC_COMM_SELF,
ierr);
575 ierr = registerInterface<CPMeshCut>();
576 CHKERRABORT(PETSC_COMM_SELF,
ierr);
583 moabCommWorld = boost::make_shared<WrapMPIComm>(PETSC_COMM_WORLD,
false);
◆ ~CrackPropagation()
FractureMechanics::CrackPropagation::~CrackPropagation |
( |
| ) |
|
|
virtual |
◆ addElasticFEInstancesToSnes()
MoFEMErrorCode FractureMechanics::CrackPropagation::addElasticFEInstancesToSnes |
( |
DM |
dm, |
|
|
Mat |
m, |
|
|
Vec |
q, |
|
|
Vec |
f, |
|
|
boost::shared_ptr< FEMethod > |
arc_method = boost::shared_ptr<FEMethod>() , |
|
|
boost::shared_ptr< ArcLengthCtx > |
arc_ctx = nullptr , |
|
|
const int |
verb = QUIET , |
|
|
const bool |
debug = false |
|
) |
| |
Definition at line 4610 of file CrackPropagation.cpp.
4613 boost::shared_ptr<FEMethod>
null;
4629 VecSetOption(arc_ctx->F_lambda, VEC_IGNORE_NEGATIVE_INDICES, PETSC_TRUE);
4634 auto oit = fit->second->getLoopFe().getOpPtrVector().begin();
4635 auto hi_oit = fit->second->getLoopFe().getOpPtrVector().end();
4636 for (; oit != hi_oit; oit++) {
4637 if (boost::typeindex::type_id_runtime(*oit) ==
4638 boost::typeindex::type_id<NeumannForcesSurface::OpNeumannForce>()) {
4646 auto oit = fit->second->getLoopFe().getOpPtrVector().begin();
4647 auto hi_oit = fit->second->getLoopFe().getOpPtrVector().end();
4648 for (; oit != hi_oit; oit++) {
4649 if (boost::typeindex::type_id_runtime(*oit) ==
4650 boost::typeindex::type_id<
4655 if (boost::typeindex::type_id_runtime(*oit) ==
4656 boost::typeindex::type_id<
4664 auto oit = fit->second->getLoopFe().getOpPtrVector().begin();
4665 auto hi_oit = fit->second->getLoopFe().getOpPtrVector().end();
4666 for (; oit != hi_oit; oit++) {
4667 if (boost::typeindex::type_id_runtime(*oit) ==
4668 boost::typeindex::type_id<EdgeForce::OpEdgeForce>()) {
4674 auto oit = fit->second->getLoopFe().getOpPtrVector().begin();
4675 auto hi_oit = fit->second->getLoopFe().getOpPtrVector().end();
4676 for (; oit != hi_oit; oit++) {
4677 if (boost::typeindex::type_id_runtime(*oit) ==
4678 boost::typeindex::type_id<NodalForce::OpNodalForce>()) {
4687 #ifdef __ANALITICAL_DISPLACEMENT__
4692 #endif //__ANALITICAL_DISPLACEMENT__
4701 if (problem_ptr->
getName() ==
"EIGEN_ELASTIC") {
4707 #ifdef __ANALITICAL_DISPLACEMENT__
4712 #endif //__ANALITICAL_DISPLACEMENT__
4718 auto fe_set_option = boost::make_shared<FEMethod>();
4719 fe_set_option->preProcessHook = [fe_set_option]() {
4720 return VecSetOption(fe_set_option->snes_f, VEC_IGNORE_NEGATIVE_INDICES,
4727 #ifdef __ANALITICAL_DISPLACEMENT__
4732 #endif //__ANALITICAL_DISPLACEMENT__
4741 if (problem_ptr->
getName() ==
"EIGEN_ELASTIC") {
4749 dm, fit->first.c_str(),
4750 boost::shared_ptr<FEMethod>(
surfaceForces, &fit->second->getLoopFe()),
4756 dm, fit->first.c_str(),
4757 boost::shared_ptr<FEMethod>(
surfacePressure, &fit->second->getLoopFe()),
4762 dm, fit->first.c_str(),
4763 boost::shared_ptr<FEMethod>(
edgeForces, &fit->second->getLoopFe()),
4768 dm, fit->first.c_str(),
4769 boost::shared_ptr<FEMethod>(
nodalForces, &fit->second->getLoopFe()),
4772 #ifdef __ANALITICAL_TRACTION__
4777 dm, fit->first.c_str(),
4783 #endif //__ANALITICAL_TRACTION__
4784 #ifdef __ANALITICAL_DISPLACEMENT__
4789 #endif //__ANALITICAL_DISPLACEMENT__
4796 if (
m == PETSC_NULL || q == PETSC_NULL ||
f == PETSC_NULL) {
4798 "problem matrix or vectors are set to null");
4801 CHKERR SNESCreate(PETSC_COMM_WORLD, &snes);
4808 CHKERR SNESDestroy(&snes);
4809 MatView(
m, PETSC_VIEWER_DRAW_WORLD);
◆ addMaterialFEInstancesToSnes()
MoFEMErrorCode FractureMechanics::CrackPropagation::addMaterialFEInstancesToSnes |
( |
DM |
dm, |
|
|
const bool |
fix_crack_front, |
|
|
const int |
verb = QUIET , |
|
|
const bool |
debug = false |
|
) |
| |
add material elements instances to SNES
Definition at line 6631 of file CrackPropagation.cpp.
6633 boost::shared_ptr<FEMethod>
null;
6637 Vec front_f, tangent_front_f;
6638 CHKERR DMCreateGlobalVector(dm, &front_f);
6639 CHKERR VecDuplicate(front_f, &tangent_front_f);
6640 CHKERR VecSetOption(front_f, VEC_IGNORE_NEGATIVE_INDICES, PETSC_TRUE);
6641 CHKERR VecSetOption(tangent_front_f, VEC_IGNORE_NEGATIVE_INDICES, PETSC_TRUE);
6643 if (
smootherFe->smootherData.frontF != PETSC_NULL)
6645 if (
smootherFe->smootherData.tangentFrontF != PETSC_NULL)
6649 smootherFe->smootherData.tangentFrontF = tangent_front_f;
6651 CHKERR PetscObjectReference((PetscObject)front_f);
6652 CHKERR PetscObjectReference((PetscObject)tangent_front_f);
6662 CHKERR PetscObjectReference((PetscObject)front_f);
6663 CHKERR PetscObjectReference((PetscObject)tangent_front_f);
6664 CHKERR VecDestroy(&front_f);
6665 CHKERR VecDestroy(&tangent_front_f);
6672 VEC_IGNORE_NEGATIVE_INDICES, PETSC_TRUE);
6684 cerr <<
"Node " << endl;
6685 FieldEntity_multiIndex::index<Ent_mi_tag>::type::iterator eit, hi_eit;
6686 eit = field_ents->get<
Ent_mi_tag>().lower_bound(*vit);
6687 hi_eit = field_ents->get<
Ent_mi_tag>().upper_bound(*vit);
6688 for (; eit != hi_eit; ++eit) {
6689 cerr << **eit << endl;
6707 if (
m.first != getInterface<CPMeshCut>()->getCrackSurfaceId()) {
6737 if (
m.first != getInterface<CPMeshCut>()->getCrackSurfaceId()) {
6760 CHKERR DMCreateGlobalVector(dm, &q);
6763 CHKERR VecSetOption(
f, VEC_IGNORE_NEGATIVE_INDICES, PETSC_TRUE);
6765 CHKERR DMCreateMatrix(dm, &
m);
6767 CHKERR SNESCreate(PETSC_COMM_WORLD, &snes);
6774 CHKERR SNESDestroy(&snes);
6776 MatView(
m, PETSC_VIEWER_STDOUT_WORLD);
◆ addMWLSDensityOperators()
Definition at line 5027 of file CrackPropagation.cpp.
5037 "Elastic element instance not created");
5042 fe_rhs = boost::make_shared<CrackFrontElement>(
5046 fe_lhs = boost::make_shared<CrackFrontElement>(
5050 bool test_with_mwls =
false;
5054 fe_rhs->meshPositionsFieldName =
"NONE";
5055 fe_lhs->meshPositionsFieldName =
"NONE";
5056 fe_rhs->addToRule = 0;
5057 fe_lhs->addToRule = 0;
5059 boost::shared_ptr<HookeElement::DataAtIntegrationPts>
5060 data_hooke_element_at_pts(
new HookeElement::DataAtIntegrationPts());
5061 boost::shared_ptr<map<int, NonlinearElasticElement::BlockData>>
5077 "Density tag name %s cannot be found. Please "
5078 "provide the correct name.",
5089 auto mat_pos_at_pts_ptr = boost::make_shared<MatrixDouble>();
5091 "MESH_NODE_POSITIONS", mat_pos_at_pts_ptr));
5093 "MESH_NODE_POSITIONS", mat_pos_at_pts_ptr));
5095 boost::shared_ptr<MatrixDouble> mat_pos_at_pts_MWLS_ptr(
5098 "MESH_NODE_POSITIONS", mat_pos_at_pts_MWLS_ptr));
5100 "MESH_NODE_POSITIONS", mat_pos_at_pts_MWLS_ptr));
5101 auto mat_grad_pos_at_pts_ptr = boost::make_shared<MatrixDouble>();
5103 "MESH_NODE_POSITIONS", mat_grad_pos_at_pts_ptr));
5105 "MESH_NODE_POSITIONS", mat_grad_pos_at_pts_ptr));
5107 "MESH_NODE_POSITIONS", data_hooke_element_at_pts->HMat));
5109 "MESH_NODE_POSITIONS", data_hooke_element_at_pts->HMat));
5110 fe_rhs->getOpPtrVector().push_back(
new OpGetCrackFrontDataGradientAtGaussPts(
5111 "SPATIAL_POSITION", data_hooke_element_at_pts->hMat,
5112 fe_rhs->singularElement, fe_rhs->invSJac));
5113 fe_lhs->getOpPtrVector().push_back(
new OpGetCrackFrontDataGradientAtGaussPts(
5114 "SPATIAL_POSITION", data_hooke_element_at_pts->hMat,
5115 fe_lhs->singularElement, fe_lhs->invSJac));
5116 auto space_grad_pos_at_pts_ptr = boost::make_shared<MatrixDouble>();
5117 fe_rhs->getOpPtrVector().push_back(
new OpGetCrackFrontDataGradientAtGaussPts(
5118 "SPATIAL_POSITION", space_grad_pos_at_pts_ptr, fe_rhs->singularElement,
5120 fe_lhs->getOpPtrVector().push_back(
new OpGetCrackFrontDataGradientAtGaussPts(
5121 "SPATIAL_POSITION", space_grad_pos_at_pts_ptr, fe_lhs->singularElement,
5124 fe_rhs->getOpPtrVector().push_back(
new OpTransfromSingularBaseFunctions(
5125 fe_rhs->singularElement, fe_rhs->detS, fe_rhs->invSJac));
5131 if (test_with_mwls) {
5132 if (
mwlsApprox->getUseGlobalBaseAtMaterialReferenceConfiguration())
5133 fe_rhs->getOpPtrVector().push_back(
new MWLSApprox::OpMWLSRhoAtGaussPts(
5134 mat_pos_at_pts_ptr, mat_grad_pos_at_pts_ptr, fe_rhs,
mwlsApprox,
5137 fe_rhs->getOpPtrVector().push_back(
5139 mat_pos_at_pts_ptr, mat_grad_pos_at_pts_ptr, fe_rhs,
mwlsApprox));
5140 fe_rhs->getOpPtrVector().push_back(
5141 new MWLSApprox::OpMWLSRhoAtGaussUsingPrecalulatedCoeffs(
5142 mat_pos_at_pts_ptr, mat_grad_pos_at_pts_ptr, fe_rhs,
mwlsApprox,
5147 fe_rhs->getOpPtrVector().push_back(
new OpGetDensityFieldForTesting(
5148 "MESH_NODE_POSITIONS", mat_pos_at_pts_ptr,
mwlsApprox->rhoAtGaussPts,
5150 fe_rhs,
mwlsApprox->singularInitialDisplacement,
5151 mat_grad_pos_at_pts_ptr));
5154 fe_rhs->getOpPtrVector().push_back(
5155 new HookeElement::OpCalculateStiffnessScaledByDensityField(
5156 "SPATIAL_POSITION",
"SPATIAL_POSITION", block_sets_ptr,
5158 fe_rhs->getOpPtrVector().push_back(
new HookeElement::OpCalculateStrainAle(
5159 "MESH_NODE_POSITIONS",
"MESH_NODE_POSITIONS", data_hooke_element_at_pts));
5160 fe_rhs->getOpPtrVector().push_back(
new HookeElement::OpCalculateStress<1>(
5161 "MESH_NODE_POSITIONS",
"MESH_NODE_POSITIONS", data_hooke_element_at_pts));
5162 fe_rhs->getOpPtrVector().push_back(
new HookeElement::OpCalculateEnergy(
5163 "MESH_NODE_POSITIONS",
"MESH_NODE_POSITIONS", data_hooke_element_at_pts));
5165 fe_rhs->getOpPtrVector().push_back(
new HookeElement::OpCalculateEshelbyStress(
5166 "MESH_NODE_POSITIONS",
"MESH_NODE_POSITIONS", data_hooke_element_at_pts));
5168 fe_rhs->getOpPtrVector().push_back(
new HookeElement::OpAleRhs_dX(
5169 "MESH_NODE_POSITIONS",
"MESH_NODE_POSITIONS", data_hooke_element_at_pts));
5170 fe_rhs->getOpPtrVector().push_back(
new HookeElement::OpAleRhs_dx(
5171 "SPATIAL_POSITION",
"SPATIAL_POSITION", data_hooke_element_at_pts));
5173 fe_rhs->getOpPtrVector().push_back(
new OpRhsBoneExplicitDerivariveWithHooke(
5174 *data_hooke_element_at_pts,
mwlsApprox->rhoAtGaussPts,
5179 fe_lhs->getOpPtrVector().push_back(
new OpGetCrackFrontDataGradientAtGaussPts(
5180 "SPATIAL_POSITION", space_grad_pos_at_pts_ptr, fe_lhs->singularElement,
5182 fe_lhs->getOpPtrVector().push_back(
new OpTransfromSingularBaseFunctions(
5183 fe_lhs->singularElement, fe_lhs->detS, fe_lhs->invSJac));
5185 if (test_with_mwls) {
5186 if (
mwlsApprox->getUseGlobalBaseAtMaterialReferenceConfiguration())
5187 fe_lhs->getOpPtrVector().push_back(
new MWLSApprox::OpMWLSRhoAtGaussPts(
5188 mat_pos_at_pts_ptr, mat_grad_pos_at_pts_ptr, fe_lhs,
mwlsApprox,
5191 fe_lhs->getOpPtrVector().push_back(
5193 mat_pos_at_pts_ptr, mat_grad_pos_at_pts_ptr, fe_lhs,
mwlsApprox));
5194 fe_lhs->getOpPtrVector().push_back(
5195 new MWLSApprox::OpMWLSRhoAtGaussUsingPrecalulatedCoeffs(
5196 mat_pos_at_pts_ptr, mat_grad_pos_at_pts_ptr, fe_lhs,
mwlsApprox,
5200 fe_lhs->getOpPtrVector().push_back(
new OpGetDensityFieldForTesting(
5201 "MESH_NODE_POSITIONS", mat_pos_at_pts_ptr,
mwlsApprox->rhoAtGaussPts,
5203 fe_lhs,
mwlsApprox->singularInitialDisplacement,
5204 mat_grad_pos_at_pts_ptr));
5207 fe_lhs->getOpPtrVector().push_back(
5208 new HookeElement::OpCalculateStiffnessScaledByDensityField(
5209 "SPATIAL_POSITION",
"SPATIAL_POSITION", block_sets_ptr,
5212 fe_lhs->getOpPtrVector().push_back(
new HookeElement::OpCalculateStrainAle(
5213 "MESH_NODE_POSITIONS",
"MESH_NODE_POSITIONS", data_hooke_element_at_pts));
5214 fe_lhs->getOpPtrVector().push_back(
new HookeElement::OpCalculateStress<1>(
5215 "MESH_NODE_POSITIONS",
"MESH_NODE_POSITIONS", data_hooke_element_at_pts));
5216 fe_lhs->getOpPtrVector().push_back(
new HookeElement::OpCalculateEnergy(
5217 "MESH_NODE_POSITIONS",
"MESH_NODE_POSITIONS", data_hooke_element_at_pts));
5218 fe_lhs->getOpPtrVector().push_back(
new HookeElement::OpCalculateEshelbyStress(
5219 "MESH_NODE_POSITIONS",
"MESH_NODE_POSITIONS", data_hooke_element_at_pts));
5221 fe_lhs->getOpPtrVector().push_back(
new HookeElement::OpAleLhs_dX_dX<1>(
5222 "MESH_NODE_POSITIONS",
"MESH_NODE_POSITIONS", data_hooke_element_at_pts));
5223 fe_lhs->getOpPtrVector().push_back(
new HookeElement::OpAleLhsPre_dX_dx<1>(
5224 "MESH_NODE_POSITIONS",
"SPATIAL_POSITION", data_hooke_element_at_pts));
5225 fe_lhs->getOpPtrVector().push_back(
new HookeElement::OpAleLhs_dX_dx(
5226 "MESH_NODE_POSITIONS",
"SPATIAL_POSITION", data_hooke_element_at_pts));
5227 fe_lhs->getOpPtrVector().push_back(
new HookeElement::OpAleLhs_dx_dx<1>(
5228 "SPATIAL_POSITION",
"SPATIAL_POSITION", data_hooke_element_at_pts));
5229 fe_lhs->getOpPtrVector().push_back(
new HookeElement::OpAleLhs_dx_dX<1>(
5230 "SPATIAL_POSITION",
"MESH_NODE_POSITIONS", data_hooke_element_at_pts));
5232 fe_lhs->getOpPtrVector().push_back(
5233 new HookeElement::OpAleLhsWithDensity_dX_dX(
5234 "MESH_NODE_POSITIONS",
"MESH_NODE_POSITIONS",
5235 data_hooke_element_at_pts,
mwlsApprox->rhoAtGaussPts,
5238 fe_lhs->getOpPtrVector().push_back(
5239 new HookeElement::OpAleLhsWithDensity_dx_dX(
5240 "SPATIAL_POSITION",
"MESH_NODE_POSITIONS", data_hooke_element_at_pts,
5244 boost::shared_ptr<MatrixDouble> mat_singular_disp_ptr =
nullptr;
5246 mat_singular_disp_ptr = boost::shared_ptr<MatrixDouble>(
5249 fe_lhs->getOpPtrVector().push_back(
5250 new OpAleLhsWithDensitySingularElement_dX_dX(
5251 "MESH_NODE_POSITIONS",
"MESH_NODE_POSITIONS",
5252 data_hooke_element_at_pts,
mwlsApprox->rhoAtGaussPts,
5255 fe_lhs->getOpPtrVector().push_back(
5256 new OpAleLhsWithDensitySingularElement_dx_dX(
5257 "SPATIAL_POSITION",
"MESH_NODE_POSITIONS",
5258 data_hooke_element_at_pts,
mwlsApprox->rhoAtGaussPts,
5262 fe_lhs->getOpPtrVector().push_back(
5263 new OpLhsBoneExplicitDerivariveWithHooke_dX(
5264 *data_hooke_element_at_pts,
mwlsApprox->rhoAtGaussPts,
5269 fe_lhs->getOpPtrVector().push_back(
5270 new OpLhsBoneExplicitDerivariveWithHooke_dx(
5271 *data_hooke_element_at_pts,
mwlsApprox->rhoAtGaussPts,
◆ addMWLSStressOperators()
Definition at line 4902 of file CrackPropagation.cpp.
4912 "Elastic element instance not created");
4917 fe_rhs = boost::make_shared<CrackFrontElement>(
4921 fe_lhs = boost::make_shared<CrackFrontElement>(
4927 fe_rhs->meshPositionsFieldName =
"NONE";
4928 fe_lhs->meshPositionsFieldName =
"NONE";
4929 fe_rhs->addToRule = 0;
4930 fe_lhs->addToRule = 0;
4945 "Internal stress tag name %s cannot be found. Please "
4946 "provide the correct name.",
4957 auto mat_pos_at_pts_ptr = boost::make_shared<MatrixDouble>();
4959 "MESH_NODE_POSITIONS", mat_pos_at_pts_ptr));
4961 "MESH_NODE_POSITIONS", mat_pos_at_pts_ptr));
4962 boost::shared_ptr<MatrixDouble> mat_grad_pos_at_pts_ptr(
new MatrixDouble());
4964 "MESH_NODE_POSITIONS", mat_grad_pos_at_pts_ptr));
4966 "MESH_NODE_POSITIONS", mat_grad_pos_at_pts_ptr));
4968 boost::shared_ptr<MatrixDouble> space_grad_pos_at_pts_ptr(
new MatrixDouble());
4969 fe_rhs->getOpPtrVector().push_back(
new OpGetCrackFrontDataGradientAtGaussPts(
4970 "SPATIAL_POSITION", space_grad_pos_at_pts_ptr, fe_rhs->singularElement,
4972 fe_rhs->getOpPtrVector().push_back(
new OpTransfromSingularBaseFunctions(
4973 fe_rhs->singularElement, fe_rhs->detS, fe_rhs->invSJac));
4975 if (
mwlsApprox->getUseGlobalBaseAtMaterialReferenceConfiguration())
4976 fe_rhs->getOpPtrVector().push_back(
new MWLSApprox::OpMWLSStressAtGaussPts(
4977 mat_pos_at_pts_ptr, mat_grad_pos_at_pts_ptr, fe_rhs,
mwlsApprox,
4980 fe_rhs->getOpPtrVector().push_back(
4982 mat_pos_at_pts_ptr, mat_grad_pos_at_pts_ptr, fe_rhs,
mwlsApprox));
4983 fe_rhs->getOpPtrVector().push_back(
4984 new MWLSApprox::OpMWLSStressAtGaussUsingPrecalulatedCoeffs(
4985 mat_pos_at_pts_ptr, mat_grad_pos_at_pts_ptr, fe_rhs,
mwlsApprox,
4989 fe_rhs->getOpPtrVector().push_back(
new MWLSApprox::OpMWLSSpatialStressRhs(
4990 mat_grad_pos_at_pts_ptr,
mwlsApprox,
false));
4991 fe_rhs->getOpPtrVector().push_back(
new MWLSApprox::OpMWLSMaterialStressRhs(
4992 space_grad_pos_at_pts_ptr, mat_grad_pos_at_pts_ptr,
mwlsApprox,
4995 fe_lhs->getOpPtrVector().push_back(
new OpGetCrackFrontDataGradientAtGaussPts(
4996 "SPATIAL_POSITION", space_grad_pos_at_pts_ptr, fe_lhs->singularElement,
4998 fe_lhs->getOpPtrVector().push_back(
new OpTransfromSingularBaseFunctions(
4999 fe_lhs->singularElement, fe_lhs->detS, fe_lhs->invSJac));
5001 if (
mwlsApprox->getUseGlobalBaseAtMaterialReferenceConfiguration())
5002 fe_lhs->getOpPtrVector().push_back(
new MWLSApprox::OpMWLSStressAtGaussPts(
5003 mat_pos_at_pts_ptr, mat_grad_pos_at_pts_ptr, fe_lhs,
mwlsApprox,
5006 fe_lhs->getOpPtrVector().push_back(
5008 mat_pos_at_pts_ptr, mat_grad_pos_at_pts_ptr, fe_lhs,
mwlsApprox));
5009 fe_lhs->getOpPtrVector().push_back(
5010 new MWLSApprox::OpMWLSStressAtGaussUsingPrecalulatedCoeffs(
5011 mat_pos_at_pts_ptr, mat_grad_pos_at_pts_ptr, fe_lhs,
mwlsApprox,
5015 fe_lhs->getOpPtrVector().push_back(
new MWLSApprox::OpMWLSSpatialStressLhs_DX(
5017 fe_lhs->getOpPtrVector().push_back(
new MWLSApprox::OpMWLSMaterialStressLhs_DX(
5018 space_grad_pos_at_pts_ptr, mat_grad_pos_at_pts_ptr,
mwlsApprox,
5020 fe_lhs->getOpPtrVector().push_back(
new MWLSApprox::OpMWLSMaterialStressLhs_Dx(
5021 space_grad_pos_at_pts_ptr, mat_grad_pos_at_pts_ptr,
mwlsApprox,
◆ addPropagationFEInstancesToSnes()
MoFEMErrorCode FractureMechanics::CrackPropagation::addPropagationFEInstancesToSnes |
( |
DM |
dm, |
|
|
boost::shared_ptr< FEMethod > |
arc_method, |
|
|
boost::shared_ptr< ArcLengthCtx > |
arc_ctx, |
|
|
const std::vector< int > & |
surface_ids, |
|
|
const int |
verb = QUIET , |
|
|
const bool |
debug = false |
|
) |
| |
add finite element to SNES for crack propagation problem
Definition at line 6921 of file CrackPropagation.cpp.
6925 boost::shared_ptr<FEMethod>
null;
6944 for (boost::ptr_map<string, NeumannForcesSurface>::iterator fit =
6947 auto oit = fit->second->getLoopFe().getOpPtrVector().begin();
6948 auto hi_oit = fit->second->getLoopFe().getOpPtrVector().end();
6949 for (; oit != hi_oit; oit++) {
6950 if (boost::typeindex::type_id_runtime(*oit) ==
6951 boost::typeindex::type_id<NeumannForcesSurface::OpNeumannForce>()) {
6959 for (boost::ptr_map<string, NeumannForcesSurface>::iterator fit =
6966 auto oit = fit->second->getLoopFeMatRhs().getOpPtrVector().begin();
6967 auto hi_oit = fit->second->getLoopFeMatRhs().getOpPtrVector().end();
6968 for (; oit != hi_oit; oit++) {
6969 if (boost::typeindex::type_id_runtime(*oit) ==
6970 boost::typeindex::type_id<
6974 .
F = arc_ctx->F_lambda;
6981 for (boost::ptr_map<string, NeumannForcesSurface>::iterator fit =
6985 auto oit = fit->second->getLoopFe().getOpPtrVector().begin();
6986 auto hi_oit = fit->second->getLoopFe().getOpPtrVector().end();
6987 for (; oit != hi_oit; oit++) {
6988 if (boost::typeindex::type_id_runtime(*oit) ==
6989 boost::typeindex::type_id<
6994 if (boost::typeindex::type_id_runtime(*oit) ==
6995 boost::typeindex::type_id<
7004 for (boost::ptr_map<string, NeumannForcesSurface>::iterator fit =
7011 auto oit = fit->second->getLoopFeMatRhs().getOpPtrVector().begin();
7012 auto hi_oit = fit->second->getLoopFeMatRhs().getOpPtrVector().end();
7013 for (; oit != hi_oit; oit++) {
7014 if (boost::typeindex::type_id_runtime(*oit) ==
7015 boost::typeindex::type_id<
7019 .
F = arc_ctx->F_lambda;
7025 for (boost::ptr_map<string, EdgeForce>::iterator fit =
edgeForces->begin();
7027 auto oit = fit->second->getLoopFe().getOpPtrVector().begin();
7028 auto hi_oit = fit->second->getLoopFe().getOpPtrVector().end();
7029 for (; oit != hi_oit; oit++) {
7030 if (boost::typeindex::type_id_runtime(*oit) ==
7031 boost::typeindex::type_id<EdgeForce::OpEdgeForce>()) {
7037 for (boost::ptr_map<string, NodalForce>::iterator fit =
nodalForces->begin();
7039 auto oit = fit->second->getLoopFe().getOpPtrVector().begin();
7040 auto hi_oit = fit->second->getLoopFe().getOpPtrVector().end();
7041 for (; oit != hi_oit; oit++) {
7042 if (boost::typeindex::type_id_runtime(*oit) ==
7043 boost::typeindex::type_id<NodalForce::OpNodalForce>()) {
7049 Vec front_f, tangent_front_f;
7050 CHKERR DMCreateGlobalVector(dm, &front_f);
7051 CHKERR VecDuplicate(front_f, &tangent_front_f);
7052 CHKERR VecSetOption(front_f, VEC_IGNORE_NEGATIVE_INDICES, PETSC_TRUE);
7053 CHKERR VecSetOption(tangent_front_f, VEC_IGNORE_NEGATIVE_INDICES, PETSC_TRUE);
7057 if (
smootherFe->smootherData.frontF != PETSC_NULL)
7059 if (
smootherFe->smootherData.tangentFrontF != PETSC_NULL)
7063 smootherFe->smootherData.tangentFrontF = tangent_front_f;
7082 VEC_IGNORE_NEGATIVE_INDICES, PETSC_TRUE);
7110 if (
m.first != getInterface<CPMeshCut>()->getCrackSurfaceId()) {
7150 if (
m.first != getInterface<CPMeshCut>()->getCrackSurfaceId()) {
7172 for (boost::ptr_map<string, NeumannForcesSurface>::iterator fit =
7176 dm, fit->first.c_str(),
7177 boost::shared_ptr<FEMethod>(
surfaceForces, &fit->second->getLoopFe()),
7183 for (boost::ptr_map<string, NeumannForcesSurface>::iterator fit =
7187 dm, fit->first.c_str(),
7189 &fit->second->getLoopFeLhs()),
7193 dm, fit->first.c_str(),
7195 &fit->second->getLoopFeMatRhs()),
7198 dm, fit->first.c_str(),
7200 &fit->second->getLoopFeMatLhs()),
7207 for (boost::ptr_map<string, NeumannForcesSurface>::iterator fit =
7211 dm, fit->first.c_str(),
7212 boost::shared_ptr<FEMethod>(
surfacePressure, &fit->second->getLoopFe()),
7217 for (boost::ptr_map<string, NeumannForcesSurface>::iterator fit =
7221 dm, fit->first.c_str(),
7223 &fit->second->getLoopFeLhs()),
7226 dm, fit->first.c_str(),
7228 &fit->second->getLoopFeMatRhs()),
7231 dm, fit->first.c_str(),
7233 &fit->second->getLoopFeMatLhs()),
7250 PETSC_NULL, PETSC_NULL);
7257 for (boost::ptr_map<string, EdgeForce>::iterator fit =
edgeForces->begin();
7260 dm, fit->first.c_str(),
7261 boost::shared_ptr<FEMethod>(
edgeForces, &fit->second->getLoopFe()),
7264 for (boost::ptr_map<string, NodalForce>::iterator fit =
nodalForces->begin();
7267 dm, fit->first.c_str(),
7268 boost::shared_ptr<FEMethod>(
nodalForces, &fit->second->getLoopFe()),
◆ addSmoothingFEInstancesToSnes()
MoFEMErrorCode FractureMechanics::CrackPropagation::addSmoothingFEInstancesToSnes |
( |
DM |
dm, |
|
|
const bool |
fix_crack_front, |
|
|
const int |
verb = QUIET , |
|
|
const bool |
debug = false |
|
) |
| |
add softening elements instances to SNES
Definition at line 6786 of file CrackPropagation.cpp.
6788 boost::shared_ptr<FEMethod>
null;
6793 if (
smootherFe->smootherData.frontF != PETSC_NULL)
6795 if (
smootherFe->smootherData.tangentFrontF != PETSC_NULL)
6798 smootherFe->smootherData.frontF = PETSC_NULL;
6799 smootherFe->smootherData.tangentFrontF = PETSC_NULL;
6810 cerr <<
"Node " << endl;
6811 FieldEntity_multiIndex::index<Ent_mi_tag>::type::iterator eit, hi_eit;
6812 eit = field_ents->get<
Ent_mi_tag>().lower_bound(*vit);
6813 hi_eit = field_ents->get<
Ent_mi_tag>().upper_bound(*vit);
6814 for (; eit != hi_eit; ++eit) {
6815 cerr << **eit << endl;
6831 if (
m.first != getInterface<CPMeshCut>()->getCrackSurfaceId()) {
6852 if (
m.first != getInterface<CPMeshCut>()->getCrackSurfaceId()) {
6872 CHKERR DMCreateGlobalVector(dm, &q);
6875 CHKERR VecSetOption(
f, VEC_IGNORE_NEGATIVE_INDICES, PETSC_TRUE);
6877 CHKERR DMCreateMatrix(dm, &
m);
6879 CHKERR SNESCreate(PETSC_COMM_WORLD, &snes);
6886 CHKERR SNESDestroy(&snes);
6888 MatView(
m, PETSC_VIEWER_STDOUT_WORLD);
◆ analyticalStrainFunction()
Definition at line 10030 of file CrackPropagation.cpp.
10036 constexpr
double alpha = 1.e-5;
10041 double temp = 250.;
10042 double z = t_coords(2);
10043 if ((-10. < z && z < -1.) || std::abs(z + 1.) < 1e-15) {
10044 temp = 10. / 3. * (35. - 4. * z);
10046 if ((-1. < z && z < 2.) || std::abs(z - 2.) < 1e-15) {
10047 temp = 10. / 3. * (34. - 5. * z);
10049 if (2. < z && z < 10.) {
10050 temp = 5. / 4. * (30. + 17. * z);
10053 t_thermal_strain(
i,
j) = alpha * (
temp - 250.) *
t_kd(
i,
j);
10054 return t_thermal_strain;
◆ assembleCouplingForcesDM()
MoFEMErrorCode FractureMechanics::CrackPropagation::assembleCouplingForcesDM |
( |
DM |
dm, |
|
|
const int |
verb = QUIET , |
|
|
const bool |
debug = false |
|
) |
| |
assemble coupling element instances
Definition at line 6178 of file CrackPropagation.cpp.
6184 "Elastic element instance not created");
6193 boost::shared_ptr<map<int, BlockData>> block_sets_ptr(
6201 new OpGetCrackFrontCommonDataAtGaussPts(
6202 "SPATIAL_POSITION",
elasticFe->commonData,
6208 "MESH_NODE_POSITIONS",
elasticFe->commonData));
6211 new OpTransfromSingularBaseFunctions(
6215 map<int, NonlinearElasticElement::BlockData>::iterator sit =
6217 for (; sit !=
elasticFe->setOfBlocks.end(); sit++) {
6221 "SPATIAL_POSITION", sit->second,
elasticFe->commonData,
6226 "SPATIAL_POSITION",
"SPATIAL_POSITION", sit->second,
6231 "SPATIAL_POSITION",
"MESH_NODE_POSITIONS", sit->second,
6235 boost::shared_ptr<HookeElement::DataAtIntegrationPts>
6236 data_hooke_element_at_pts(
new HookeElement::DataAtIntegrationPts());
6239 "MESH_NODE_POSITIONS", data_hooke_element_at_pts->HMat));
6241 boost::shared_ptr<MatrixDouble> mat_pos_at_pts_ptr(
new MatrixDouble());
6244 mat_pos_at_pts_ptr));
6249 "mwlsApprox not allocated");
6252 boost::shared_ptr<MatrixDouble> mat_grad_pos_at_pts_ptr;
6253 mat_grad_pos_at_pts_ptr =
6257 mat_grad_pos_at_pts_ptr));
6259 new OpGetCrackFrontDataGradientAtGaussPts(
6260 "SPATIAL_POSITION", data_hooke_element_at_pts->hMat,
6264 if (
mwlsApprox->getUseGlobalBaseAtMaterialReferenceConfiguration())
6266 new MWLSApprox::OpMWLSRhoAtGaussPts(
6267 mat_pos_at_pts_ptr, mat_grad_pos_at_pts_ptr,
6272 mat_pos_at_pts_ptr, mat_grad_pos_at_pts_ptr,
6275 new MWLSApprox::OpMWLSRhoAtGaussUsingPrecalulatedCoeffs(
6276 mat_pos_at_pts_ptr, mat_grad_pos_at_pts_ptr,
6280 new HookeElement::OpCalculateStiffnessScaledByDensityField(
6281 "SPATIAL_POSITION",
"SPATIAL_POSITION", block_sets_ptr,
6288 new HookeElement::OpCalculateStrainAle(
"SPATIAL_POSITION",
6290 data_hooke_element_at_pts));
6292 new HookeElement::OpCalculateStress<1>(
"SPATIAL_POSITION",
6294 data_hooke_element_at_pts));
6297 new OpTransfromSingularBaseFunctions(
6301 new HookeElement::OpAleLhs_dx_dx<1>(
"SPATIAL_POSITION",
6303 data_hooke_element_at_pts));
6305 new HookeElement::OpAleLhs_dx_dX<1>(
"SPATIAL_POSITION",
6306 "MESH_NODE_POSITIONS",
6307 data_hooke_element_at_pts));
6310 new HookeElement::OpAleLhsWithDensity_dx_dX(
6311 "SPATIAL_POSITION",
"MESH_NODE_POSITIONS",
6312 data_hooke_element_at_pts,
mwlsApprox->rhoAtGaussPts,
6315 boost::shared_ptr<MatrixDouble> mat_singular_disp_ptr =
nullptr;
6318 mat_singular_disp_ptr = boost::shared_ptr<MatrixDouble>(
6322 new OpAleLhsWithDensitySingularElement_dx_dX(
6323 "SPATIAL_POSITION",
"MESH_NODE_POSITIONS",
6324 data_hooke_element_at_pts,
mwlsApprox->rhoAtGaussPts,
6326 mat_singular_disp_ptr));
6332 new HookeElement::OpCalculateHomogeneousStiffness<0>(
6333 "SPATIAL_POSITION",
"SPATIAL_POSITION", block_sets_ptr,
6334 data_hooke_element_at_pts));
6339 new OpGetCrackFrontDataGradientAtGaussPts(
6340 "SPATIAL_POSITION", data_hooke_element_at_pts->hMat,
6344 new HookeElement::OpCalculateStrainAle(
"SPATIAL_POSITION",
6346 data_hooke_element_at_pts));
6348 new HookeElement::OpCalculateStress<0>(
"SPATIAL_POSITION",
6350 data_hooke_element_at_pts));
6353 new OpTransfromSingularBaseFunctions(
6357 new HookeElement::OpAleLhs_dx_dx<0>(
"SPATIAL_POSITION",
6359 data_hooke_element_at_pts));
6361 new HookeElement::OpAleLhs_dx_dX<0>(
"SPATIAL_POSITION",
6362 "MESH_NODE_POSITIONS",
6363 data_hooke_element_at_pts));
6369 "Material element instance not created");
6378 boost::shared_ptr<MatrixDouble> mat_pos_at_pts_ptr(
new MatrixDouble());
6381 mat_pos_at_pts_ptr));
6382 boost::shared_ptr<MatrixDouble> mat_grad_pos_at_pts_ptr;
6384 mat_grad_pos_at_pts_ptr =
6388 mat_grad_pos_at_pts_ptr));
6390 boost::shared_ptr<MatrixDouble> space_grad_pos_at_pts_ptr;
6392 space_grad_pos_at_pts_ptr =
6395 new OpGetCrackFrontDataGradientAtGaussPts(
6396 "SPATIAL_POSITION", space_grad_pos_at_pts_ptr,
6404 new OpGetCrackFrontCommonDataAtGaussPts(
6410 "MESH_NODE_POSITIONS",
materialFe->commonData));
6412 new OpTransfromSingularBaseFunctions(
6415 for (map<int, NonlinearElasticElement::BlockData>::iterator sit =
6417 sit !=
materialFe->setOfBlocks.end(); sit++) {
6420 "SPATIAL_POSITION", sit->second,
materialFe->commonData,
6424 "MESH_NODE_POSITIONS",
"MESH_NODE_POSITIONS", sit->second,
6428 "MESH_NODE_POSITIONS",
"SPATIAL_POSITION", sit->second,
6433 boost::shared_ptr<HookeElement::DataAtIntegrationPts>
6434 data_hooke_element_at_pts(
new HookeElement::DataAtIntegrationPts());
6439 "MESH_NODE_POSITIONS", data_hooke_element_at_pts->HMat));
6440 mat_grad_pos_at_pts_ptr = data_hooke_element_at_pts->HMat;
6445 "mwlsApprox not allocated");
6449 new OpGetCrackFrontDataGradientAtGaussPts(
6450 "SPATIAL_POSITION", data_hooke_element_at_pts->hMat,
6454 if (
mwlsApprox->getUseGlobalBaseAtMaterialReferenceConfiguration())
6456 new MWLSApprox::OpMWLSRhoAtGaussPts(
6457 mat_pos_at_pts_ptr, mat_grad_pos_at_pts_ptr,
6462 mat_pos_at_pts_ptr, mat_grad_pos_at_pts_ptr,
6465 new MWLSApprox::OpMWLSRhoAtGaussUsingPrecalulatedCoeffs(
6466 mat_pos_at_pts_ptr, mat_grad_pos_at_pts_ptr,
6471 new HookeElement::OpCalculateStiffnessScaledByDensityField(
6472 "SPATIAL_POSITION",
"SPATIAL_POSITION", block_sets_ptr,
6475 space_grad_pos_at_pts_ptr = data_hooke_element_at_pts->hMat;
6477 new HookeElement::OpCalculateStrainAle(
"MESH_NODE_POSITIONS",
6478 "MESH_NODE_POSITIONS",
6479 data_hooke_element_at_pts));
6481 new HookeElement::OpCalculateStress<1>(
"MESH_NODE_POSITIONS",
6482 "MESH_NODE_POSITIONS",
6483 data_hooke_element_at_pts));
6485 new HookeElement::OpCalculateEnergy(
"MESH_NODE_POSITIONS",
6486 "MESH_NODE_POSITIONS",
6487 data_hooke_element_at_pts));
6489 new HookeElement::OpCalculateEshelbyStress(
6490 "MESH_NODE_POSITIONS",
"MESH_NODE_POSITIONS",
6491 data_hooke_element_at_pts));
6493 new OpTransfromSingularBaseFunctions(
6497 new HookeElement::OpAleLhs_dX_dX<1>(
"MESH_NODE_POSITIONS",
6498 "MESH_NODE_POSITIONS",
6499 data_hooke_element_at_pts));
6501 new HookeElement::OpAleLhsPre_dX_dx<1>(
"MESH_NODE_POSITIONS",
6503 data_hooke_element_at_pts));
6505 new HookeElement::OpAleLhs_dX_dx(
"MESH_NODE_POSITIONS",
6507 data_hooke_element_at_pts));
6510 new HookeElement::OpAleLhsWithDensity_dX_dX(
6511 "MESH_NODE_POSITIONS",
"MESH_NODE_POSITIONS",
6512 data_hooke_element_at_pts,
mwlsApprox->rhoAtGaussPts,
6515 boost::shared_ptr<MatrixDouble> mat_singular_disp_ptr =
nullptr;
6518 mat_singular_disp_ptr = boost::shared_ptr<MatrixDouble>(
6522 new OpAleLhsWithDensitySingularElement_dX_dX(
6523 "MESH_NODE_POSITIONS",
"MESH_NODE_POSITIONS",
6524 data_hooke_element_at_pts,
mwlsApprox->rhoAtGaussPts,
6526 mat_singular_disp_ptr));
6530 new OpLhsBoneExplicitDerivariveWithHooke_dX(
6531 *data_hooke_element_at_pts,
mwlsApprox->rhoAtGaussPts,
6537 new OpLhsBoneExplicitDerivariveWithHooke_dx(
6538 *data_hooke_element_at_pts,
mwlsApprox->rhoAtGaussPts,
6546 new HookeElement::OpCalculateHomogeneousStiffness<0>(
6547 "SPATIAL_POSITION",
"SPATIAL_POSITION", block_sets_ptr,
6548 data_hooke_element_at_pts));
6553 new OpGetCrackFrontDataGradientAtGaussPts(
6554 "SPATIAL_POSITION", data_hooke_element_at_pts->hMat,
6557 space_grad_pos_at_pts_ptr = data_hooke_element_at_pts->hMat;
6559 new HookeElement::OpCalculateStrainAle(
"MESH_NODE_POSITIONS",
6560 "MESH_NODE_POSITIONS",
6561 data_hooke_element_at_pts));
6563 new HookeElement::OpCalculateStress<0>(
"MESH_NODE_POSITIONS",
6564 "MESH_NODE_POSITIONS",
6565 data_hooke_element_at_pts));
6567 new HookeElement::OpCalculateEnergy(
"MESH_NODE_POSITIONS",
6568 "MESH_NODE_POSITIONS",
6569 data_hooke_element_at_pts));
6571 new HookeElement::OpCalculateEshelbyStress(
6572 "MESH_NODE_POSITIONS",
"MESH_NODE_POSITIONS",
6573 data_hooke_element_at_pts));
6575 new OpTransfromSingularBaseFunctions(
6579 new HookeElement::OpAleLhs_dX_dX<0>(
"MESH_NODE_POSITIONS",
6580 "MESH_NODE_POSITIONS",
6581 data_hooke_element_at_pts));
6583 new HookeElement::OpAleLhsPre_dX_dx<0>(
"MESH_NODE_POSITIONS",
6585 data_hooke_element_at_pts));
6587 new HookeElement::OpAleLhs_dX_dx(
"MESH_NODE_POSITIONS",
6589 data_hooke_element_at_pts));
6596 "mwlsApprox not allocated");
6599 if (
mwlsApprox->getUseGlobalBaseAtMaterialReferenceConfiguration())
6601 new MWLSApprox::OpMWLSStressAtGaussPts(
6602 mat_pos_at_pts_ptr, mat_grad_pos_at_pts_ptr,
6607 mat_pos_at_pts_ptr, mat_grad_pos_at_pts_ptr,
6610 new MWLSApprox::OpMWLSStressAtGaussUsingPrecalulatedCoeffs(
6611 mat_pos_at_pts_ptr, mat_grad_pos_at_pts_ptr,
6616 new MWLSApprox::OpMWLSSpatialStressLhs_DX(mat_grad_pos_at_pts_ptr,
6619 new MWLSApprox::OpMWLSMaterialStressLhs_DX(
6620 space_grad_pos_at_pts_ptr, mat_grad_pos_at_pts_ptr,
mwlsApprox,
6623 new MWLSApprox::OpMWLSMaterialStressLhs_Dx(
6624 space_grad_pos_at_pts_ptr, mat_grad_pos_at_pts_ptr,
mwlsApprox,
◆ assembleElasticDM() [1/2]
MoFEMErrorCode FractureMechanics::CrackPropagation::assembleElasticDM |
( |
const int |
verb = QUIET , |
|
|
const bool |
debug = false |
|
) |
| |
create elastic finite element instance for spatial assembly
- Parameters
-
verb | compilation parameter determining the amount of information printed (not in use here) |
debug | flag for debugging |
- Returns
- error code
Definition at line 4601 of file CrackPropagation.cpp.
◆ assembleElasticDM() [2/2]
MoFEMErrorCode FractureMechanics::CrackPropagation::assembleElasticDM |
( |
const std::string |
mwls_stress_tag_name, |
|
|
const int |
verb = QUIET , |
|
|
const bool |
debug = false |
|
) |
| |
create elastic finite element instance for spatial assembly
- Parameters
-
mwls_stress_tag_name | Name of internal stress tag |
close_crack | if true, crack surface is closed |
verb | compilation parameter determining the amount of information printed (not in use here) |
debug | flag for debugging |
- Returns
- error code
Rhs
Lhs
Definition at line 3795 of file CrackPropagation.cpp.
3804 feRhs = boost::make_shared<CrackFrontElement>(
3809 feLhs = boost::make_shared<CrackFrontElement>(
3815 feRhs->meshPositionsFieldName =
"NONE";
3816 feLhs->meshPositionsFieldName =
"NONE";
3817 feRhs->addToRule = 0;
3818 feLhs->addToRule = 0;
3823 boost::shared_ptr<map<int, BlockData>> block_sets_ptr(
3831 CHKERR it->getAttributeDataStructure(mydata);
3832 int id = it->getMeshsetId();
3835 meshset, MBTET,
elasticFe->setOfBlocks[
id].tEts,
true);
3838 elasticFe->setOfBlocks[id].PoissonRatio = mydata.
data.Poisson;
3841 CHKERR PetscPrintf(PETSC_COMM_WORLD,
"\nMaterial block %d \n",
id);
3842 CHKERR PetscPrintf(PETSC_COMM_WORLD,
"\tYoung's modulus %6.4e \n",
3844 CHKERR PetscPrintf(PETSC_COMM_WORLD,
"\tPoisson's ratio %6.4e \n\n",
3845 mydata.
data.Poisson);
3850 elasticFe->setOfBlocks[id].materialDoublePtr =
3851 boost::make_shared<Hooke<double>>();
3852 elasticFe->setOfBlocks[id].materialAdoublePtr =
3853 boost::make_shared<Hooke<adouble>>();
3856 elasticFe->setOfBlocks[id].materialDoublePtr = boost::make_shared<
3859 elasticFe->setOfBlocks[id].materialAdoublePtr = boost::make_shared<
3865 elasticFe->setOfBlocks[id].materialDoublePtr =
3866 boost::make_shared<NeoHookean<double>>();
3867 elasticFe->setOfBlocks[id].materialAdoublePtr =
3868 boost::make_shared<NeoHookean<adouble>>();
3872 elasticFe->setOfBlocks[id].materialDoublePtr =
3873 boost::make_shared<Hooke<double>>();
3874 elasticFe->setOfBlocks[id].materialAdoublePtr =
3875 boost::make_shared<Hooke<adouble>>();
3879 "Material type not yet implemented");
3886 elasticFe->commonData.spatialPositions =
"SPATIAL_POSITION";
3887 elasticFe->commonData.meshPositions =
"MESH_NODE_POSITIONS";
3889 auto data_hooke_element_at_pts =
3890 boost::make_shared<HookeElement::DataAtIntegrationPts>();
3893 auto mat_pos_at_pts_ptr = boost::make_shared<MatrixDouble>();
3895 "MESH_NODE_POSITIONS", mat_pos_at_pts_ptr));
3896 boost::shared_ptr<MatrixDouble> mat_grad_pos_at_pts_ptr;
3898 mat_grad_pos_at_pts_ptr = boost::make_shared<MatrixDouble>();
3899 feRhs->getOpPtrVector().push_back(
3901 mat_grad_pos_at_pts_ptr));
3909 feRhs->getOpPtrVector().push_back(
new OpGetCrackFrontCommonDataAtGaussPts(
3910 "SPATIAL_POSITION",
elasticFe->commonData,
feRhs->singularElement,
3913 feRhs->getOpPtrVector().push_back(
3915 "MESH_NODE_POSITIONS",
elasticFe->commonData));
3917 feRhs->getOpPtrVector().push_back(
new OpTransfromSingularBaseFunctions(
3920 map<int, NonlinearElasticElement::BlockData>::iterator sit =
3922 for (; sit !=
elasticFe->setOfBlocks.end(); sit++) {
3924 feRhs->getOpPtrVector().push_back(
3926 "SPATIAL_POSITION", sit->second,
elasticFe->commonData,
3929 feRhs->getOpPtrVector().push_back(
3931 "SPATIAL_POSITION", sit->second,
elasticFe->commonData));
3934 feRhs->getOpPtrVector().push_back(
3936 "MESH_NODE_POSITIONS", data_hooke_element_at_pts->HMat));
3937 mat_grad_pos_at_pts_ptr = data_hooke_element_at_pts->HMat;
3952 th_rho) != MB_SUCCESS) {
3954 "Density tag name %s cannot be found. Please "
3955 "provide the correct name.",
3969 feRhs->getOpPtrVector().push_back(
3970 new OpGetCrackFrontDataGradientAtGaussPts(
3971 "SPATIAL_POSITION", data_hooke_element_at_pts->hMat,
3974 if (
mwlsApprox->getUseGlobalBaseAtMaterialReferenceConfiguration())
3975 feRhs->getOpPtrVector().push_back(
new MWLSApprox::OpMWLSRhoAtGaussPts(
3979 feRhs->getOpPtrVector().push_back(
3981 mat_pos_at_pts_ptr, mat_grad_pos_at_pts_ptr,
feRhs,
3983 feRhs->getOpPtrVector().push_back(
3984 new MWLSApprox::OpMWLSRhoAtGaussUsingPrecalulatedCoeffs(
3985 mat_pos_at_pts_ptr, mat_grad_pos_at_pts_ptr,
feRhs,
3989 feRhs->getOpPtrVector().push_back(
3990 new HookeElement::OpCalculateStiffnessScaledByDensityField(
3991 "SPATIAL_POSITION",
"SPATIAL_POSITION", block_sets_ptr,
3994 feRhs->getOpPtrVector().push_back(
3995 new HookeElement::OpCalculateStrainAle(
"SPATIAL_POSITION",
3997 data_hooke_element_at_pts));
3998 feRhs->getOpPtrVector().push_back(
3999 new HookeElement::OpCalculateStress<1>(
"SPATIAL_POSITION",
4001 data_hooke_element_at_pts));
4003 feRhs->getOpPtrVector().push_back(
4004 new HookeElement::OpCalculateHomogeneousStiffness<0>(
4005 "SPATIAL_POSITION",
"SPATIAL_POSITION", block_sets_ptr,
4006 data_hooke_element_at_pts));
4010 feRhs->getOpPtrVector().push_back(
4011 new OpGetCrackFrontDataGradientAtGaussPts(
4012 "SPATIAL_POSITION", data_hooke_element_at_pts->hMat,
4014 feRhs->getOpPtrVector().push_back(
4015 new HookeElement::OpCalculateStrainAle(
"SPATIAL_POSITION",
4017 data_hooke_element_at_pts));
4018 feRhs->getOpPtrVector().push_back(
4019 new HookeElement::OpCalculateStress<0>(
"SPATIAL_POSITION",
4021 data_hooke_element_at_pts));
4024 feRhs->getOpPtrVector().push_back(
new OpTransfromSingularBaseFunctions(
4026 feRhs->getOpPtrVector().push_back(
new HookeElement::OpAleRhs_dx(
4027 "SPATIAL_POSITION",
"SPATIAL_POSITION", data_hooke_element_at_pts));
4042 if (
mwlsApprox->mwlsMoab.tag_get_handle(mwls_stress_tag_name.c_str(),
4043 th) != MB_SUCCESS) {
4045 "Internal stress tag name %s cannot be found. Please "
4046 "provide the correct name.",
4047 mwls_stress_tag_name.substr(4).c_str());
4052 if (
mwlsApprox->mwlsMoab.tag_get_handle(mwls_stress_tag_name.c_str(),
4053 th) != MB_SUCCESS) {
4055 "Internal stress tag name %s cannot be found. Please "
4056 "provide the correct name.",
4057 mwls_stress_tag_name.substr(4).c_str());
4064 if (
mwlsApprox->getUseGlobalBaseAtMaterialReferenceConfiguration())
4065 feRhs->getOpPtrVector().push_back(
4066 new MWLSApprox::OpMWLSStressAtGaussPts(
4068 mwls_stress_tag_name,
false));
4070 feRhs->getOpPtrVector().push_back(
4072 mat_pos_at_pts_ptr, mat_grad_pos_at_pts_ptr,
feRhs,
4074 feRhs->getOpPtrVector().push_back(
4075 new MWLSApprox::OpMWLSStressAtGaussUsingPrecalulatedCoeffs(
4077 mwls_stress_tag_name,
false));
4081 feRhs->getOpPtrVector().push_back(
new MWLSApprox::OpMWLSSpatialStressRhs(
4084 feRhs->getOpPtrVector().push_back(
4085 new HookeElement::OpAnalyticalInternalAleStrain_dx<0>(
4086 "SPATIAL_POSITION", data_hooke_element_at_pts,
4088 boost::shared_ptr<MatrixDouble>(
4098 feLhs->getOpPtrVector().push_back(
new OpGetCrackFrontCommonDataAtGaussPts(
4099 "SPATIAL_POSITION",
elasticFe->commonData,
feLhs->singularElement,
4102 feLhs->getOpPtrVector().push_back(
4104 "MESH_NODE_POSITIONS",
elasticFe->commonData));
4106 feLhs->getOpPtrVector().push_back(
new OpTransfromSingularBaseFunctions(
4109 map<int, NonlinearElasticElement::BlockData>::iterator sit =
4111 for (; sit !=
elasticFe->setOfBlocks.end(); sit++) {
4113 feLhs->getOpPtrVector().push_back(
4115 "SPATIAL_POSITION", sit->second,
elasticFe->commonData,
4118 feLhs->getOpPtrVector().push_back(
4120 "SPATIAL_POSITION",
"SPATIAL_POSITION", sit->second,
4126 "MESH_NODE_POSITIONS", mat_pos_at_pts_ptr));
4127 boost::shared_ptr<MatrixDouble> mat_grad_pos_at_pts_ptr;
4129 mat_grad_pos_at_pts_ptr = boost::make_shared<MatrixDouble>();
4130 feLhs->getOpPtrVector().push_back(
4132 mat_grad_pos_at_pts_ptr));
4135 feLhs->getOpPtrVector().push_back(
4137 "MESH_NODE_POSITIONS", data_hooke_element_at_pts->HMat));
4138 mat_grad_pos_at_pts_ptr = data_hooke_element_at_pts->HMat;
4143 "mwlsApprox not allocated");
4154 feLhs->getOpPtrVector().push_back(
4155 new OpGetCrackFrontDataGradientAtGaussPts(
4156 "SPATIAL_POSITION", data_hooke_element_at_pts->hMat,
4160 if (
mwlsApprox->getUseGlobalBaseAtMaterialReferenceConfiguration())
4161 feLhs->getOpPtrVector().push_back(
new MWLSApprox::OpMWLSRhoAtGaussPts(
4165 feLhs->getOpPtrVector().push_back(
4167 mat_pos_at_pts_ptr, mat_grad_pos_at_pts_ptr,
feLhs,
4169 feLhs->getOpPtrVector().push_back(
4170 new MWLSApprox::OpMWLSRhoAtGaussUsingPrecalulatedCoeffs(
4171 mat_pos_at_pts_ptr, mat_grad_pos_at_pts_ptr,
feLhs,
4175 feLhs->getOpPtrVector().push_back(
4176 new HookeElement::OpCalculateStiffnessScaledByDensityField(
4177 "SPATIAL_POSITION",
"SPATIAL_POSITION", block_sets_ptr,
4180 feLhs->getOpPtrVector().push_back(
4181 new HookeElement::OpCalculateStrainAle(
"SPATIAL_POSITION",
4183 data_hooke_element_at_pts));
4184 feLhs->getOpPtrVector().push_back(
4185 new HookeElement::OpCalculateStress<1>(
"SPATIAL_POSITION",
4187 data_hooke_element_at_pts));
4189 feLhs->getOpPtrVector().push_back(
new OpTransfromSingularBaseFunctions(
4191 feLhs->getOpPtrVector().push_back(
new HookeElement::OpAleLhs_dx_dx<1>(
4192 "SPATIAL_POSITION",
"SPATIAL_POSITION", data_hooke_element_at_pts));
4195 feLhs->getOpPtrVector().push_back(
4196 new HookeElement::OpCalculateHomogeneousStiffness<0>(
4197 "SPATIAL_POSITION",
"SPATIAL_POSITION", block_sets_ptr,
4198 data_hooke_element_at_pts));
4202 feLhs->getOpPtrVector().push_back(
4203 new OpGetCrackFrontDataGradientAtGaussPts(
4204 "SPATIAL_POSITION", data_hooke_element_at_pts->hMat,
4206 feLhs->getOpPtrVector().push_back(
4207 new HookeElement::OpCalculateStrainAle(
"SPATIAL_POSITION",
4209 data_hooke_element_at_pts));
4210 feLhs->getOpPtrVector().push_back(
4211 new HookeElement::OpCalculateStress<0>(
"SPATIAL_POSITION",
4213 data_hooke_element_at_pts));
4216 feLhs->getOpPtrVector().push_back(
new OpTransfromSingularBaseFunctions(
4218 feLhs->getOpPtrVector().push_back(
new HookeElement::OpAleLhs_dx_dx<0>(
4219 "SPATIAL_POSITION",
"SPATIAL_POSITION", data_hooke_element_at_pts));
4227 boost::make_shared<boost::ptr_map<string, NeumannForcesSurface>>();
4230 string fe_name_str =
"FORCE_FE";
4233 auto &fe = nf.getLoopFe();
4240 .addForce(
"SPATIAL_POSITION", PETSC_NULL, it->getMeshsetId(),
true);
4243 const string block_set_force_name(
"FORCE");
4246 if (it->getName().compare(0, block_set_force_name.length(),
4247 block_set_force_name) == 0) {
4249 .addForce(
"SPATIAL_POSITION", PETSC_NULL, (it->getMeshsetId()),
4257 boost::make_shared<boost::ptr_map<string, NeumannForcesSurface>>();
4259 boost::make_shared<NeumannForcesSurface::DataAtIntegrationPts>();
4262 string fe_name_str =
"FORCE_FE_ALE";
4266 auto &fe_lhs = nf.getLoopFeLhs();
4267 auto &fe_mat_rhs = nf.getLoopFeMatRhs();
4268 auto &fe_mat_lhs = nf.getLoopFeMatLhs();
4277 .addForceAle(
"SPATIAL_POSITION",
"MESH_NODE_POSITIONS",
4279 PETSC_NULL,
bit->getMeshsetId(),
true,
false,
4283 const string block_set_force_name(
"FORCE");
4286 if (it->getName().compare(0, block_set_force_name.length(),
4287 block_set_force_name) == 0) {
4289 .addForceAle(
"SPATIAL_POSITION",
"MESH_NODE_POSITIONS",
4291 PETSC_NULL, it->getMeshsetId(),
true,
true,
4299 boost::make_shared<boost::ptr_map<string, NeumannForcesSurface>>();
4301 string fe_name_str =
"PRESSURE_FE";
4305 auto &fe = nf.getLoopFe();
4313 .addPressure(
"SPATIAL_POSITION", PETSC_NULL, it->getMeshsetId(),
4317 const string block_set_pressure_name(
"PRESSURE");
4319 if (it->getName().compare(0, block_set_pressure_name.length(),
4320 block_set_pressure_name) == 0) {
4322 .addPressure(
"SPATIAL_POSITION", PETSC_NULL, it->getMeshsetId(),
4327 const string block_set_linear_pressure_name(
"LINEAR_PRESSURE");
4329 if (it->getName().compare(0, block_set_linear_pressure_name.length(),
4330 block_set_linear_pressure_name) == 0) {
4332 .addLinearPressure(
"SPATIAL_POSITION", PETSC_NULL,
4333 it->getMeshsetId(),
true);
4340 boost::make_shared<boost::ptr_map<string, NeumannForcesSurface>>();
4342 boost::make_shared<NeumannForcesSurface::DataAtIntegrationPts>();
4344 string fe_name_str =
"PRESSURE_ALE";
4348 auto &fe_lhs = nf.getLoopFeLhs();
4349 auto &fe_mat_rhs = nf.getLoopFeMatRhs();
4350 auto &fe_mat_lhs = nf.getLoopFeMatLhs();
4360 .addPressureAle(
"SPATIAL_POSITION",
"MESH_NODE_POSITIONS",
4362 PETSC_NULL, it->getMeshsetId(),
true);
4368 boost::make_shared<FaceElementForcesAndSourcesCore>(
mField);
4371 boost::make_shared<FaceElementForcesAndSourcesCore>(
mField);
4373 boost::make_shared<FaceElementForcesAndSourcesCore>(
mField);
4375 boost::make_shared<MetaSpringBC::DataAtIntegrationPtsSprings>(
mField);
4386 feSpringLhsPtr = boost::shared_ptr<FaceElementForcesAndSourcesCore>(
4388 feSpringRhsPtr = boost::shared_ptr<FaceElementForcesAndSourcesCore>(
4393 "MESH_NODE_POSITIONS");
4395 bool use_eigen_pos =
4398 bool use_eigen_pos_simple_contact =
4403 boost::make_shared<SimpleContactProblem::SimpleContactElement>(
mField);
4405 boost::make_shared<SimpleContactProblem::SimpleContactElement>(
mField);
4407 boost::make_shared<SimpleContactProblem::CommonDataSimpleContact>(
mField);
4416 "LAMBDA_CONTACT",
false, use_eigen_pos_simple_contact,
4417 "EIGEN_SPATIAL_POSITIONS");
4420 "LAMBDA_CONTACT",
false, use_eigen_pos_simple_contact,
4421 "EIGEN_SPATIAL_POSITIONS");
4425 "LAMBDA_CONTACT",
false, use_eigen_pos_simple_contact,
4426 "EIGEN_SPATIAL_POSITIONS");
4430 "LAMBDA_CONTACT",
false, use_eigen_pos_simple_contact,
4431 "EIGEN_SPATIAL_POSITIONS");
4437 boost::shared_ptr<BothSurfaceConstrains>(
new BothSurfaceConstrains(
4438 mField,
"LAMBDA_CLOSE_CRACK",
"SPATIAL_POSITION"));
4447 boost::make_shared<SimpleContactProblem::SimpleContactElement>(
mField);
4449 boost::make_shared<SimpleContactProblem::SimpleContactElement>(
mField);
4451 boost::make_shared<SimpleContactProblem::SimpleContactElement>(
mField);
4453 boost::make_shared<SimpleContactProblem::CommonDataSimpleContact>(
4460 "SPATIAL_POSITION",
"MESH_NODE_POSITIONS",
"LAMBDA_CONTACT",
4465 "SPATIAL_POSITION",
"MESH_NODE_POSITIONS",
"LAMBDA_CONTACT",
4470 "MESH_NODE_POSITIONS",
"LAMBDA_CONTACT", use_eigen_pos_simple_contact,
4471 "EIGEN_SPATIAL_POSITIONS");
4475 boost::make_shared<MortarContactProblem::MortarContactElement>(
4477 "MESH_NODE_POSITIONS");
4479 boost::make_shared<MortarContactProblem::MortarContactElement>(
4481 "MESH_NODE_POSITIONS");
4483 boost::make_shared<MortarContactProblem::CommonDataMortarContact>(
mField);
4492 "LAMBDA_CONTACT",
false, use_eigen_pos,
"EIGEN_SPATIAL_POSITIONS");
4496 "LAMBDA_CONTACT",
false, use_eigen_pos,
"EIGEN_SPATIAL_POSITIONS");
4500 "LAMBDA_CONTACT",
false, use_eigen_pos,
"EIGEN_SPATIAL_POSITIONS");
4504 "LAMBDA_CONTACT",
false, use_eigen_pos,
"EIGEN_SPATIAL_POSITIONS");
4510 boost::make_shared<SimpleContactProblem::SimpleContactElement>(
mField);
4513 boost::make_shared<MortarContactProblem::MortarContactElement>(
4515 "MESH_NODE_POSITIONS");
4521 use_eigen_pos_simple_contact,
"EIGEN_SPATIAL_POSITIONS");
4526 use_eigen_pos,
"EIGEN_SPATIAL_POSITIONS");
4530 nodalForces = boost::make_shared<boost::ptr_map<string, NodalForce>>();
4532 string fe_name_str =
"FORCE_FE";
4537 .addForce(
"SPATIAL_POSITION", PETSC_NULL, it->getMeshsetId(),
false);
4541 edgeForces = boost::make_shared<boost::ptr_map<string, EdgeForce>>();
4543 string fe_name_str =
"FORCE_FE";
4548 .addForce(
"SPATIAL_POSITION", PETSC_NULL, it->getMeshsetId(),
false);
4555 PETSC_NULL, PETSC_NULL));
4558 #ifdef __ANALITICAL_DISPLACEMENT__
4561 mField,
"SPATIAL_POSITION", PETSC_NULL, PETSC_NULL, PETSC_NULL));
4562 #endif //__ANALITICAL_DISPLACEMENT__
4565 #ifdef __ANALITICAL_TRACTION__
4568 if (meshset_manager_ptr->
checkMeshset(
"ANALITICAL_TRACTION")) {
4571 boost::make_shared<boost::ptr_map<string, NeumannForcesSurface>>();
4573 string fe_name_str =
"ANALITICAL_TRACTION";
4579 &cubit_meshset_ptr);
4584 .analyticalForceOp.push_back(
new AnalyticalForces());
4586 .fe.getOpPtrVector()
4587 .push_back(
new OpAnalyticalSpatialTraction(
4590 "SPATIAL_POSITION", faces,
4596 #endif //__ANALITICAL_TRACTION__
◆ assembleMaterialForcesDM()
MoFEMErrorCode FractureMechanics::CrackPropagation::assembleMaterialForcesDM |
( |
DM |
dm, |
|
|
const int |
verb = QUIET , |
|
|
const bool |
debug = false |
|
) |
| |
create material element instance
Operators to assemble rhs
Definition at line 5531 of file CrackPropagation.cpp.
5536 "Elastic element instance not created");
5539 materialFe = boost::shared_ptr<NonlinearElasticElement>(
5543 for (std::map<int, NonlinearElasticElement::BlockData>::iterator sit =
5545 sit !=
elasticFe->setOfBlocks.end(); sit++) {
5546 materialFe->setOfBlocks[sit->first] = sit->second;
5547 materialFe->setOfBlocks[sit->first].forcesOnlyOnEntitiesRow =
5550 materialFe->commonData.spatialPositions =
"SPATIAL_POSITION";
5551 materialFe->commonData.meshPositions =
"MESH_NODE_POSITIONS";
5566 boost::shared_ptr<map<int, BlockData>> block_sets_ptr(
5568 boost::shared_ptr<HookeElement::DataAtIntegrationPts>
5569 data_hooke_element_at_pts(
new HookeElement::DataAtIntegrationPts());
5573 boost::shared_ptr<MatrixDouble> mat_pos_at_pts_ptr(
new MatrixDouble());
5575 "MESH_NODE_POSITIONS", mat_pos_at_pts_ptr));
5577 "MESH_NODE_POSITIONS", mat_pos_at_pts_ptr));
5578 boost::shared_ptr<MatrixDouble> mat_grad_pos_at_pts_ptr;
5580 mat_grad_pos_at_pts_ptr =
5584 mat_grad_pos_at_pts_ptr));
5587 mat_grad_pos_at_pts_ptr));
5589 boost::shared_ptr<MatrixDouble> space_grad_pos_at_pts_ptr;
5591 space_grad_pos_at_pts_ptr =
5594 new OpGetCrackFrontDataGradientAtGaussPts(
5595 "SPATIAL_POSITION", space_grad_pos_at_pts_ptr,
5598 new OpGetCrackFrontDataGradientAtGaussPts(
5599 "SPATIAL_POSITION", space_grad_pos_at_pts_ptr,
5606 new OpGetCrackFrontCommonDataAtGaussPts(
5611 "MESH_NODE_POSITIONS",
materialFe->commonData));
5614 new OpTransfromSingularBaseFunctions(
feMaterialRhs->singularElement,
5617 for (map<int, NonlinearElasticElement::BlockData>::iterator sit =
5619 sit !=
materialFe->setOfBlocks.end(); sit++) {
5622 "SPATIAL_POSITION", sit->second,
materialFe->commonData,
5626 "MESH_NODE_POSITIONS", sit->second,
materialFe->commonData));
5633 "MESH_NODE_POSITIONS", data_hooke_element_at_pts->HMat));
5634 mat_grad_pos_at_pts_ptr = data_hooke_element_at_pts->HMat;
5637 new OpGetCrackFrontDataGradientAtGaussPts(
5638 "SPATIAL_POSITION", data_hooke_element_at_pts->hMat,
5640 space_grad_pos_at_pts_ptr = data_hooke_element_at_pts->hMat;
5645 "mwlsApprox not allocated");
5648 if (
mwlsApprox->getUseGlobalBaseAtMaterialReferenceConfiguration())
5650 new MWLSApprox::OpMWLSRhoAtGaussPts(
5659 new MWLSApprox::OpMWLSRhoAtGaussUsingPrecalulatedCoeffs(
5665 new HookeElement::OpCalculateStiffnessScaledByDensityField(
5666 "SPATIAL_POSITION",
"SPATIAL_POSITION", block_sets_ptr,
5670 new HookeElement::OpCalculateStrainAle(
"MESH_NODE_POSITIONS",
5671 "MESH_NODE_POSITIONS",
5672 data_hooke_element_at_pts));
5674 new HookeElement::OpCalculateStress<1>(
"MESH_NODE_POSITIONS",
5675 "MESH_NODE_POSITIONS",
5676 data_hooke_element_at_pts));
5680 new HookeElement::OpCalculateHomogeneousStiffness<0>(
5681 "SPATIAL_POSITION",
"SPATIAL_POSITION", block_sets_ptr,
5682 data_hooke_element_at_pts));
5684 new HookeElement::OpCalculateStrainAle(
"MESH_NODE_POSITIONS",
5685 "MESH_NODE_POSITIONS",
5686 data_hooke_element_at_pts));
5688 new HookeElement::OpCalculateStress<0>(
"MESH_NODE_POSITIONS",
5689 "MESH_NODE_POSITIONS",
5690 data_hooke_element_at_pts));
5693 new HookeElement::OpCalculateEnergy(
"MESH_NODE_POSITIONS",
5694 "MESH_NODE_POSITIONS",
5695 data_hooke_element_at_pts));
5698 new HookeElement::OpCalculateEshelbyStress(
"MESH_NODE_POSITIONS",
5699 "MESH_NODE_POSITIONS",
5700 data_hooke_element_at_pts));
5702 new OpTransfromSingularBaseFunctions(
feMaterialRhs->singularElement,
5706 feMaterialRhs->getOpPtrVector().push_back(
new HookeElement::OpAleRhs_dX(
5707 "MESH_NODE_POSITIONS",
"MESH_NODE_POSITIONS",
5708 data_hooke_element_at_pts));
5712 new OpRhsBoneExplicitDerivariveWithHooke(
5713 *data_hooke_element_at_pts,
mwlsApprox->rhoAtGaussPts,
5722 "mwlsApprox not allocated");
5729 if (
mwlsApprox->getUseGlobalBaseAtMaterialReferenceConfiguration())
5731 new MWLSApprox::OpMWLSStressAtGaussPts(
5740 new MWLSApprox::OpMWLSStressAtGaussUsingPrecalulatedCoeffs(
5746 new MWLSApprox::OpMWLSMaterialStressRhs(space_grad_pos_at_pts_ptr,
5747 mat_grad_pos_at_pts_ptr,
5751 new HookeElement::OpAnalyticalInternalAleStrain_dX<0>(
5752 "MESH_NODE_POSITIONS", data_hooke_element_at_pts,
5754 boost::shared_ptr<MatrixDouble>(
5760 #ifdef __ANALITICAL_TRACTION__
5764 boost::shared_ptr<NeumannForcesSurface::MyTriangleFE>(
5770 if (meshset_manager_ptr->
checkMeshset(
"ANALITICAL_TRACTION")) {
5773 &cubit_meshset_ptr);
5778 new OpAnalyticalMaterialTraction(
5781 "MESH_NODE_POSITIONS", faces,
5789 #endif //__ANALITICAL_TRACTION__
5794 new OpGetCrackFrontCommonDataAtGaussPts(
5799 "MESH_NODE_POSITIONS",
materialFe->commonData));
5801 new OpTransfromSingularBaseFunctions(
5806 for (
auto sit =
materialFe->setOfBlocks.begin();
5807 sit !=
materialFe->setOfBlocks.end(); sit++) {
5810 "SPATIAL_POSITION", sit->second,
materialFe->commonData,
5814 "MESH_NODE_POSITIONS",
"MESH_NODE_POSITIONS", sit->second,
5820 "MESH_NODE_POSITIONS", data_hooke_element_at_pts->HMat));
5821 mat_grad_pos_at_pts_ptr = data_hooke_element_at_pts->HMat;
5823 new OpGetCrackFrontDataGradientAtGaussPts(
5824 "SPATIAL_POSITION", data_hooke_element_at_pts->hMat,
5826 space_grad_pos_at_pts_ptr = data_hooke_element_at_pts->hMat;
5831 "mwlsApprox not allocated");
5834 if (
mwlsApprox->getUseGlobalBaseAtMaterialReferenceConfiguration())
5836 new MWLSApprox::OpMWLSRhoAtGaussPts(
5845 new MWLSApprox::OpMWLSRhoAtGaussUsingPrecalulatedCoeffs(
5851 new HookeElement::OpCalculateStiffnessScaledByDensityField(
5852 "SPATIAL_POSITION",
"SPATIAL_POSITION", block_sets_ptr,
5857 new HookeElement::OpCalculateStrainAle(
"MESH_NODE_POSITIONS",
5858 "MESH_NODE_POSITIONS",
5859 data_hooke_element_at_pts));
5862 new HookeElement::OpCalculateStress<1>(
"MESH_NODE_POSITIONS",
5863 "MESH_NODE_POSITIONS",
5864 data_hooke_element_at_pts));
5867 new HookeElement::OpCalculateEnergy(
"MESH_NODE_POSITIONS",
5868 "MESH_NODE_POSITIONS",
5869 data_hooke_element_at_pts));
5871 new HookeElement::OpCalculateEshelbyStress(
5872 "MESH_NODE_POSITIONS",
"MESH_NODE_POSITIONS",
5873 data_hooke_element_at_pts));
5875 new OpTransfromSingularBaseFunctions(
feMaterialLhs->singularElement,
5879 new HookeElement::OpAleLhs_dX_dX<1>(
"MESH_NODE_POSITIONS",
5880 "MESH_NODE_POSITIONS",
5881 data_hooke_element_at_pts));
5884 new HookeElement::OpAleLhsWithDensity_dX_dX(
5885 "MESH_NODE_POSITIONS",
"MESH_NODE_POSITIONS",
5886 data_hooke_element_at_pts,
mwlsApprox->rhoAtGaussPts,
5889 boost::shared_ptr<MatrixDouble> mat_singular_disp_ptr =
nullptr;
5892 mat_singular_disp_ptr = boost::shared_ptr<MatrixDouble>(
5896 new OpAleLhsWithDensitySingularElement_dX_dX(
5897 "MESH_NODE_POSITIONS",
"MESH_NODE_POSITIONS",
5898 data_hooke_element_at_pts,
mwlsApprox->rhoAtGaussPts,
5900 mat_singular_disp_ptr));
5904 new OpLhsBoneExplicitDerivariveWithHooke_dX(
5905 *data_hooke_element_at_pts,
mwlsApprox->rhoAtGaussPts,
5912 new HookeElement::OpCalculateHomogeneousStiffness<0>(
5913 "SPATIAL_POSITION",
"SPATIAL_POSITION", block_sets_ptr,
5914 data_hooke_element_at_pts));
5917 new HookeElement::OpCalculateStrainAle(
"MESH_NODE_POSITIONS",
5918 "MESH_NODE_POSITIONS",
5919 data_hooke_element_at_pts));
5921 new HookeElement::OpCalculateStress<0>(
"MESH_NODE_POSITIONS",
5922 "MESH_NODE_POSITIONS",
5923 data_hooke_element_at_pts));
5926 new HookeElement::OpCalculateEnergy(
"MESH_NODE_POSITIONS",
5927 "MESH_NODE_POSITIONS",
5928 data_hooke_element_at_pts));
5930 new HookeElement::OpCalculateEshelbyStress(
5931 "MESH_NODE_POSITIONS",
"MESH_NODE_POSITIONS",
5932 data_hooke_element_at_pts));
5934 new OpTransfromSingularBaseFunctions(
feMaterialLhs->singularElement,
5938 new HookeElement::OpAleLhs_dX_dX<0>(
"MESH_NODE_POSITIONS",
5939 "MESH_NODE_POSITIONS",
5940 data_hooke_element_at_pts));
5946 "mwlsApprox not allocated");
5949 if (
mwlsApprox->getUseGlobalBaseAtMaterialReferenceConfiguration())
5951 new MWLSApprox::OpMWLSStressAtGaussPts(
5960 new MWLSApprox::OpMWLSStressAtGaussUsingPrecalulatedCoeffs(
5966 new MWLSApprox::OpMWLSMaterialStressLhs_DX(
5967 space_grad_pos_at_pts_ptr, mat_grad_pos_at_pts_ptr,
mwlsApprox,
◆ assembleSmootherForcesDM()
MoFEMErrorCode FractureMechanics::CrackPropagation::assembleSmootherForcesDM |
( |
DM |
dm, |
|
|
const std::vector< int > |
ids, |
|
|
const int |
verb = QUIET , |
|
|
const bool |
debug = false |
|
) |
| |
create smoothing element instance
Definition at line 5975 of file CrackPropagation.cpp.
5980 "Elastic element instance not created");
5993 for (std::map<int, NonlinearElasticElement::BlockData>::iterator sit =
5995 sit !=
elasticFe->setOfBlocks.end(); sit++) {
5997 smootherFe->setOfBlocks[0].tEts.merge(sit->second.tEts);
6004 smootherFe->commonData.spatialPositions =
"MESH_NODE_POSITIONS";
6005 smootherFe->commonData.meshPositions =
"NONE";
6008 smootherFe->feRhs.meshPositionsFieldName =
"NONE";
6009 smootherFe->feLhs.meshPositionsFieldName =
"NONE";
6020 "MESH_NODE_POSITIONS",
smootherFe->commonData));
6021 map<int, NonlinearElasticElement::BlockData>::iterator sit =
6024 "MESH_NODE_POSITIONS",
smootherFe->setOfBlocks.at(0),
6027 "MESH_NODE_POSITIONS", sit->second,
smootherFe->commonData,
6033 "MESH_NODE_POSITIONS",
smootherFe->commonData));
6035 "MESH_NODE_POSITIONS",
smootherFe->setOfBlocks.at(0),
6038 "MESH_NODE_POSITIONS",
"MESH_NODE_POSITIONS",
6040 smootherFe->smootherData,
"LAMBDA_CRACKFRONT_AREA_TANGENT"));
6046 mField,
"LAMBDA_CRACKFRONT_AREA_TANGENT"));
6048 Range contact_faces;
6055 boost::shared_ptr<SurfaceSlidingConstrains::DriverElementOrientation>(
6056 new FaceOrientation(
false, contact_faces,
mapBitLevel[
"mesh_cut"]));
6057 for (
auto id : ids) {
6058 if (
id != getInterface<CPMeshCut>()->getCrackSurfaceId()) {
6063 "LAMBDA_SURFACE" + boost::lexical_cast<std::string>(
id),
6069 boost::shared_ptr<SurfaceSlidingConstrains::DriverElementOrientation>(
6070 new FaceOrientation(
true, contact_faces,
mapBitLevel[
"mesh_cut"]));
6076 boost::lexical_cast<std::string>(
6077 getInterface<CPMeshCut>()->getCrackSurfaceId()),
6081 auto get_edges_block_set = [
this]() {
6082 return getInterface<CPMeshCut>()->getEdgesBlockSet();
6084 if (get_edges_block_set()) {
6086 boost::shared_ptr<EdgeSlidingConstrains>(
6093 new BothSurfaceConstrains(
mField));
6097 new BothSurfaceConstrains(
mField,
"LAMBDA_BOTH_SIDES_CONTACT"));
6101 Range fix_material_nodes;
6103 mField,
"MESH_NODE_POSITIONS", fix_material_nodes);
6106 for (
auto f : *fields_ptr) {
6107 if (
f->getName().compare(0, 6,
"LAMBDA") == 0 &&
6108 f->getName() !=
"LAMBDA_ARC_LENGTH" &&
6109 f->getName() !=
"LAMBDA_CONTACT" &&
6110 f->getName() !=
"LAMBDA_CLOSE_CRACK") {
6131 new GriffithForceElement::OpCalculateGriffithForce(
6145 boost::shared_ptr<GriffithForceElement::MyTriangleFEConstrainsDelta>(
6146 new GriffithForceElement::MyTriangleFEConstrainsDelta(
6147 mField,
"LAMBDA_CRACKFRONT_AREA"));
6149 new GriffithForceElement::OpConstrainsDelta(
6154 boost::shared_ptr<GriffithForceElement::MyTriangleFEConstrains>(
6155 new GriffithForceElement::MyTriangleFEConstrains(
6156 mField,
"LAMBDA_CRACKFRONT_AREA",
6160 boost::shared_ptr<GriffithForceElement::MyTriangleFEConstrains>(
6161 new GriffithForceElement::MyTriangleFEConstrains(
6162 mField,
"LAMBDA_CRACKFRONT_AREA",
6166 new GriffithForceElement::OpConstrainsRhs(
6170 new GriffithForceElement::OpConstrainsLhs(
◆ buildArcLengthField()
Declate field for arc-length.
- Parameters
-
- Returns
- error code
Definition at line 1726 of file CrackPropagation.cpp.
1736 if (vit == refined_ents_ptr->get<
Ent_mi_tag>().end()) {
1738 "Vertex of arc-length field not found");
1740 *(
const_cast<RefEntity *
>(vit->get())->getBitRefLevelPtr()) |=
bit;
1742 FieldEntity::getLocalUniqueIdCalculate(
1746 "Arc length field entity not found");
1748 MOFEM_LOG(
"CPWorld", Sev::noisy) << **fit;
1749 MOFEM_LOG_C(
"CPWorld", Sev::inform,
"Arc-Length field lambda = %3.4e",
1750 (*fit)->getEntFieldData()[0]);
1755 MB_TAG_SPARSE,
MF_ZERO, verb);
1759 std::pair<RefEntity_multiIndex::iterator, bool> p_ent =
1761 ->insert(boost::make_shared<RefEntity>(
1763 *(
const_cast<RefEntity *
>(p_ent.first->get())->getBitRefLevelPtr()) |=
◆ buildBothSidesFieldId()
Lagrange multipliers field which constrains material displacements.
Nodes on both sides are constrainded to have the same material displacements
Definition at line 1973 of file CrackPropagation.cpp.
1979 auto add_both_sides_field = [&](
const std::string lambda_field_name,
1989 std::ostringstream file;
1990 file << lambda_field_name <<
".vtk";
2000 Range ents_to_remove;
2003 ents_to_remove = subtract(ents_to_remove, master_nodes);
2016 lambda_field_name, 1);
2030 for (
auto const &field : (*fields_ptr)) {
2031 const std::string
field_name = field->getName();
2032 if (
field_name.compare(0, 14,
"LAMBDA_SURFACE") == 0) {
2035 if (
field_name.compare(0, 11,
"LAMBDA_EDGE") == 0) {
2042 auto write_file = [&](
const std::string file_name,
Range ents) {
2055 auto get_prisms_level_nodes = [&](
auto bit) {
2060 prisms_level = intersect(
bitProcEnts, prisms_level);
2062 Range prisms_level_nodes;
2065 return prisms_level_nodes;
2068 auto get_other_side_crack_faces_nodes = [&](
auto prisms_level_nodes) {
2069 Range other_side_crack_faces_nodes;
2072 other_side_crack_faces_nodes =
2073 intersect(other_side_crack_faces_nodes, prisms_level_nodes);
2074 other_side_crack_faces_nodes =
2077 return other_side_crack_faces_nodes;
2080 auto prisms_level_nodes = get_prisms_level_nodes(bit_material);
2081 auto other_side_crack_faces_nodes =
2082 get_other_side_crack_faces_nodes(prisms_level_nodes);
2084 CHKERR add_both_sides_field(
"LAMBDA_BOTH_SIDES", other_side_crack_faces_nodes,
2085 other_side_crack_faces_nodes,
false);
2088 Range other_side_crack_faces_edges;
2091 other_side_crack_faces_edges,
2092 moab::Interface::UNION);
2093 other_side_crack_faces_edges =
2094 subtract(other_side_crack_faces_edges,
crackFront);
2097 other_side_crack_faces_edges.merge(
2098 get_other_side_crack_faces_nodes(get_prisms_level_nodes(bit_spatial)));
2099 CHKERR add_both_sides_field(
"LAMBDA_CLOSE_CRACK",
2100 other_side_crack_faces_edges,
Range(),
true);
2111 Range contact_both_sides_slave_nodes;
2114 Range contact_prisms;
2116 &*nit, 1, 3,
false, contact_prisms, moab::Interface::UNION);
2120 int side_number, other_side_number, sense, offset, number_nodes = 0;
2124 if (side_number < 3)
2125 contact_both_sides_slave_nodes.insert(conn[side_number + 3]);
2127 contact_both_sides_slave_nodes.insert(conn[side_number - 3]);
2129 contact_both_sides_slave_nodes =
2130 intersect(contact_both_sides_slave_nodes, prisms_level_nodes);
2133 CHKERR write_file(
"contact_both_sides_slave_nodes.vtk",
2134 contact_both_sides_slave_nodes);
2137 CHKERR add_both_sides_field(
"LAMBDA_BOTH_SIDES_CONTACT",
2139 contact_both_sides_slave_nodes,
false);
◆ buildCrackFrontFieldId()
declare crack surface files
Definition at line 2145 of file CrackPropagation.cpp.
2156 Range crack_front_nodes;
2157 CHKERR moab.get_connectivity(crack_front_edges, crack_front_nodes,
true);
2160 1, MB_TAG_SPARSE,
MF_ZERO, verb);
2163 Range ents_to_remove;
2166 ents_to_remove = subtract(ents_to_remove, crack_front_nodes);
2168 ents_to_remove, verb);
2170 "LAMBDA_CRACKFRONT_AREA", verb);
2182 Range skin_crack_front;
2183 rval = skin.find_skin(0, crack_front_edges,
false, skin_crack_front);
2184 Range field_ents = subtract(crack_front_nodes, skin_crack_front);
2186 Range ents_to_remove;
2189 ents_to_remove = subtract(ents_to_remove, field_ents);
2191 ents_to_remove, verb);
2193 field_ents, MBVERTEX,
"LAMBDA_CRACKFRONT_AREA_TANGENT", verb);
◆ buildCrackSurfaceFieldId()
MoFEMErrorCode FractureMechanics::CrackPropagation::buildCrackSurfaceFieldId |
( |
const BitRefLevel |
bit, |
|
|
const bool |
proc_only = true , |
|
|
const bool |
build_fields = true , |
|
|
const int |
verb = QUIET , |
|
|
const bool |
debug = false |
|
) |
| |
declare crack surface files
Definition at line 1911 of file CrackPropagation.cpp.
1923 crack_surface_ents = intersect(crack_surface_ents, level_tris);
1928 moab::Interface::UNION);
1929 crack_surface_ents = intersect(crack_surface_ents, proc_tris);
1946 "LAMBDA_SURFACE" + boost::lexical_cast<std::string>(
1947 getInterface<CPMeshCut>()->getCrackSurfaceId());
1949 CHKERR moab.get_connectivity(crack_surface_ents, dofs_nodes,
true);
1956 MB_TAG_SPARSE,
MF_ZERO, verb);
1957 Range ents_to_remove;
1959 ents_to_remove = subtract(ents_to_remove, dofs_nodes);
◆ buildEdgeFields()
build fields with Lagrange multipliers to constrain edges
Definition at line 1836 of file CrackPropagation.cpp.
1847 CHKERR bit_ref_manager_ptr->getEntitiesByTypeAndRefLevel(
1850 if (meshset_manager_ptr->checkMeshset(
1851 getInterface<CPMeshCut>()->getEdgesBlockSet(),
BLOCKSET)) {
1853 CHKERR meshset_manager_ptr->getEntitiesByDimension(
1854 getInterface<CPMeshCut>()->getEdgesBlockSet(),
BLOCKSET, 1,
1855 meshset_ents,
true);
1856 edges_ents = intersect(meshset_ents, level_edges);
1862 moab::Interface::UNION);
1863 edges_ents = intersect(edges_ents, proc_edges);
1867 Range other_side_crack_faces_nodes;
1869 other_side_crack_faces_nodes,
true);
1870 other_side_crack_faces_nodes =
1874 CHKERR moab.get_connectivity(edges_ents, dofs_nodes,
true);
1875 dofs_nodes = subtract(dofs_nodes, other_side_crack_faces_nodes);
1882 MB_TAG_SPARSE,
MF_ZERO, verb);
1884 Range ents_to_remove;
1886 ents_to_remove = subtract(ents_to_remove, dofs_nodes);
1893 auto get_fields_multi_index = [
this]() {
1898 for (
auto field : get_fields_multi_index()) {
1899 if (field->getName().compare(0, 14,
"LAMBDA_SURFACE") == 0) {
◆ buildElasticFields()
Declate fields for elastic analysis.
- Parameters
-
- Returns
- error code
Definition at line 1460 of file CrackPropagation.cpp.
1466 auto write_file = [&](
const std::string file_name,
Range ents) {
1479 Range tets_level, tets_level_all;
1481 bit, mask, MBTET, tets_level_all);
1483 tets_level = intersect(
bitProcEnts, tets_level_all);
1488 bit, mask, MBPRISM, prisms_level);
1490 prisms_level = intersect(prisms_level,
bitProcEnts);
1492 Range prisms_level_tris;
1494 prisms_level, 2,
false, prisms_level_tris, moab::Interface::UNION);
1496 if (
rval != MB_SUCCESS &&
rval != MB_MULTIPLE_ENTITIES_FOUND) {
1501 prisms_level_tris = intersect(prisms_level_tris,
crackFaces);
1502 Range proc_ents = tets_level;
1503 proc_ents.merge(prisms_level_tris);
1506 MB_TAG_SPARSE,
MF_ZERO, verb);
1513 MB_TAG_SPARSE,
MF_ZERO, verb);
1517 dofs_ents.merge(proc_ents);
1518 for (
int dd = 1;
dd != 3; ++
dd) {
1519 CHKERR moab.get_adjacencies(proc_ents,
dd,
false, dofs_ents,
1520 moab::Interface::UNION);
1524 CHKERR moab.get_connectivity(proc_ents, dofs_nodes,
true);
1530 ents_to_remove_from_field;
1533 ents_to_remove_from_field);
1534 ents_to_remove_from_field = subtract(ents_to_remove_from_field, dofs_nodes);
1535 ents_to_remove_from_field = subtract(ents_to_remove_from_field, dofs_ents);
1537 ents_to_remove_from_field, verb);
1539 ents_to_remove_from_field, verb);
1542 ents_to_remove_from_field, verb);
1545 auto add_spatial_field_ents = [&](
const auto field_name) {
1559 CHKERR add_spatial_field_ents(
"SPATIAL_POSITION");
1561 CHKERR add_spatial_field_ents(
"EIGEN_SPATIAL_POSITIONS");
1564 Range current_field_ents;
1566 current_field_ents);
1567 dofs_nodes = current_field_ents.subset_by_type(MBVERTEX);
1568 dofs_ents = subtract(current_field_ents, dofs_nodes);
1570 "MESH_NODE_POSITIONS", verb);
1572 auto set_spatial_field_order = [&](
const auto field_name) {
1578 CHKERR set_spatial_field_order(
"SPATIAL_POSITION");
1580 CHKERR set_spatial_field_order(
"EIGEN_SPATIAL_POSITIONS");
1597 non_ref_ents.merge(dofs_ents);
1599 Range contact_faces;
1609 bit, mask, MBTET, proc_ents);
1610 map<int, Range> layers;
1614 CHKERR moab.get_adjacencies(ref_nodes, 3,
false, tets,
1615 moab::Interface::UNION);
1616 tets = intersect(tets_level_all, tets);
1618 Range ref_order_ents;
1619 CHKERR moab.get_adjacencies(tets, 2,
false, ref_order_ents,
1620 moab::Interface::UNION);
1621 CHKERR moab.get_adjacencies(tets, 1,
false, ref_order_ents,
1622 moab::Interface::UNION);
1623 layers[ll].merge(ref_order_ents);
1624 CHKERR moab.get_connectivity(tets, ref_nodes,
false);
1626 if (!contact_faces.empty()) {
1627 Range contact_layer = intersect(layers[ll], contact_faces);
1628 for (Range::iterator tit = contact_layer.begin();
1629 tit != contact_layer.end(); tit++) {
1630 Range contact_prisms, contact_tris;
1631 CHKERR moab.get_adjacencies(&*tit, 1, 3,
false, contact_prisms,
1632 moab::Interface::UNION);
1633 contact_prisms = contact_prisms.subset_by_type(MBPRISM);
1635 Range contact_elements;
1639 contact_elements = intersect(contact_prisms, contact_elements);
1640 if (contact_elements.empty()) {
1642 "Expecting adjacent contact prism(s), but none found");
1644 CHKERR moab.get_adjacencies(contact_elements, 2,
false,
1645 contact_tris, moab::Interface::UNION);
1646 contact_tris = contact_tris.subset_by_type(MBTRI);
1647 if (contact_tris.size() < 2) {
1650 "Expecting 2 or more adjacent contact tris, but %d found",
1651 contact_tris.size());
1655 layers[ll].merge(contact_tris);
1656 Range contact_edges, contact_nodes;
1657 CHKERR moab.get_adjacencies(contact_tris, 1,
false, contact_edges,
1658 moab::Interface::UNION);
1659 layers[ll].merge(contact_edges);
1660 CHKERR moab.get_connectivity(contact_tris, contact_nodes,
false);
1661 ref_nodes.merge(contact_nodes);
1666 for (map<int, Range>::reverse_iterator mit = layers.rbegin();
1667 mit != layers.rend(); mit++, poo++) {
1668 mit->second = intersect(mit->second, dofs_ents);
1671 non_ref_ents = subtract(non_ref_ents, mit->second);
1683 Range cont_ents, cont_adj_ent;
1689 CHKERR moab.get_adjacencies(cont_ents, 1,
false, cont_adj_ent,
1690 moab::Interface::UNION);
1691 cont_ents.merge(cont_adj_ent);
1692 cont_ents = intersect(cont_ents, non_ref_ents);
◆ buildProblemFields()
Build problem fields.
- Parameters
-
bit1 | bit level for computational domain |
mask1 | bit mask |
bit2 | bit level for elements near crack front |
mask2 | bit mask 2 |
verb | verbosity level |
debug | |
- Returns
- error code
Definition at line 9456 of file CrackPropagation.cpp.
9467 if (getInterface<CPMeshCut>()->getEdgesBlockSet())
◆ buildProblemFiniteElements()
Build problem finite elements.
- Parameters
-
bit1 | bit level for computational domain |
bit2 | bit level for elements near crack front |
surface_ids | meshsets Ids on body skin |
verb | verbosity level |
debug | |
- Returns
- error code
Definition at line 9480 of file CrackPropagation.cpp.
9485 auto all_bits = []() {
return BitRefLevel().set(); };
9487 auto get_edges_block_set = [
this]() {
9488 return getInterface<CPMeshCut>()->getEdgesBlockSet();
9508 if (get_edges_block_set()) {
◆ buildSurfaceFields()
build fields with Lagrange multipliers to constrain surfaces
Definition at line 1771 of file CrackPropagation.cpp.
1785 Range body_skin_proc_tris;
1786 body_skin_proc_tris = intersect(
bodySkin, level_tris);
1791 moab::Interface::UNION);
1792 body_skin_proc_tris = intersect(body_skin_proc_tris, proc_tris);
1809 "LAMBDA_SURFACE" + boost::lexical_cast<std::string>(
1810 getInterface<CPMeshCut>()->getSkinOfTheBodyId());
1812 CHKERR moab.get_connectivity(body_skin_proc_tris, dofs_nodes,
true);
1818 MB_TAG_SPARSE,
MF_ZERO, verb);
1820 Range ents_to_remove;
1822 ents_to_remove = subtract(ents_to_remove, dofs_nodes);
◆ calculateElasticEnergy()
MoFEMErrorCode FractureMechanics::CrackPropagation::calculateElasticEnergy |
( |
DM |
dm, |
|
|
const std::string |
msg = "" |
|
) |
| |
assemble elastic part of matrix, by running elastic finite element instance
Definition at line 7280 of file CrackPropagation.cpp.
7285 "Elastic element instance not created");
7287 feEnergy = boost::make_shared<CrackFrontElement>(
7291 feEnergy->meshPositionsFieldName =
"NONE";
7292 feRhs->addToRule = 0;
7296 feEnergy->getOpPtrVector().push_back(
7297 new OpGetCrackFrontCommonDataAtGaussPts(
7298 "SPATIAL_POSITION",
elasticFe->commonData,
7300 feEnergy->getOpPtrVector().push_back(
7302 "MESH_NODE_POSITIONS",
elasticFe->commonData));
7303 feEnergy->getOpPtrVector().push_back(
new OpTransfromSingularBaseFunctions(
7305 map<int, NonlinearElasticElement::BlockData>::iterator sit =
7307 for (; sit !=
elasticFe->setOfBlocks.end(); sit++) {
7308 feEnergy->getOpPtrVector().push_back(
7317 boost::shared_ptr<map<int, BlockData>> block_sets_ptr(
7320 boost::shared_ptr<HookeElement::DataAtIntegrationPts>
7321 data_hooke_element_at_pts(
new HookeElement::DataAtIntegrationPts());
7322 boost::shared_ptr<MatrixDouble> mat_grad_pos_at_pts_ptr =
7323 data_hooke_element_at_pts->HMat;
7324 boost::shared_ptr<MatrixDouble> space_grad_pos_at_pts_ptr =
7325 data_hooke_element_at_pts->hMat;
7327 feEnergy->getOpPtrVector().push_back(
7329 "MESH_NODE_POSITIONS", data_hooke_element_at_pts->HMat));
7334 "mwlsApprox not allocated");
7336 boost::shared_ptr<MatrixDouble> mat_pos_at_pts_ptr(
new MatrixDouble());
7338 "MESH_NODE_POSITIONS", mat_pos_at_pts_ptr));
7339 feEnergy->getOpPtrVector().push_back(
7340 new OpGetCrackFrontDataGradientAtGaussPts(
7341 "SPATIAL_POSITION", data_hooke_element_at_pts->hMat,
7343 if (
mwlsApprox->getUseGlobalBaseAtMaterialReferenceConfiguration())
7344 feEnergy->getOpPtrVector().push_back(
7345 new MWLSApprox::OpMWLSRhoAtGaussPts(
7346 mat_pos_at_pts_ptr, mat_grad_pos_at_pts_ptr,
feEnergy,
7349 feEnergy->getOpPtrVector().push_back(
7351 mat_pos_at_pts_ptr, mat_grad_pos_at_pts_ptr,
feEnergy,
7353 feEnergy->getOpPtrVector().push_back(
7354 new MWLSApprox::OpMWLSRhoAtGaussUsingPrecalulatedCoeffs(
7355 mat_pos_at_pts_ptr, mat_grad_pos_at_pts_ptr,
feEnergy,
7358 feEnergy->getOpPtrVector().push_back(
7359 new HookeElement::OpCalculateStiffnessScaledByDensityField(
7360 "SPATIAL_POSITION",
"SPATIAL_POSITION", block_sets_ptr,
7363 feEnergy->getOpPtrVector().push_back(
7364 new HookeElement::OpCalculateStrainAle(
"SPATIAL_POSITION",
7366 data_hooke_element_at_pts));
7368 feEnergy->getOpPtrVector().push_back(
7369 new HookeElement::OpCalculateStress<1>(
"SPATIAL_POSITION",
7371 data_hooke_element_at_pts));
7374 feEnergy->getOpPtrVector().push_back(
7375 new HookeElement::OpCalculateHomogeneousStiffness<0>(
7376 "SPATIAL_POSITION",
"SPATIAL_POSITION", block_sets_ptr,
7377 data_hooke_element_at_pts));
7381 feEnergy->getOpPtrVector().push_back(
7382 new OpGetCrackFrontDataGradientAtGaussPts(
7383 "SPATIAL_POSITION", data_hooke_element_at_pts->hMat,
7385 feEnergy->getOpPtrVector().push_back(
7386 new HookeElement::OpCalculateStrainAle(
"SPATIAL_POSITION",
7388 data_hooke_element_at_pts));
7389 feEnergy->getOpPtrVector().push_back(
7390 new HookeElement::OpCalculateStress<0>(
"SPATIAL_POSITION",
7392 data_hooke_element_at_pts));
7395 feEnergy->getOpPtrVector().push_back(
new OpTransfromSingularBaseFunctions(
7397 feEnergy->getOpPtrVector().push_back(
new HookeElement::OpCalculateEnergy(
7398 "SPATIAL_POSITION",
"SPATIAL_POSITION", data_hooke_element_at_pts,
7402 feEnergy->snes_ctx = SnesMethod::CTX_SNESNONE;
7407 PetscPrintf(PETSC_COMM_WORLD,
"Elastic energy %8.8e\n", msg.c_str(),
7410 PetscPrintf(PETSC_COMM_WORLD,
"%s Elastic energy %8.8e\n", msg.c_str(),
◆ calculateFrontProjectionMatrix()
MoFEMErrorCode FractureMechanics::CrackPropagation::calculateFrontProjectionMatrix |
( |
DM |
dm_surface, |
|
|
DM |
dm_project, |
|
|
const int |
verb = QUIET , |
|
|
const bool |
debug = false |
|
) |
| |
assemble crack front projection matrix (that constrains crack area growth)
Definition at line 7637 of file CrackPropagation.cpp.
7644 PetscPrintf(PETSC_COMM_WORLD,
"No DOFs at crack front\n");
7660 CHKERR MatShellSetOperation(Q, MATOP_MULT,
7669 ConstantArea constant_area(
mField);
7670 constant_area.feLhsPtr = boost::shared_ptr<ConstantArea::MyTriangleFE>(
7671 new ConstantArea::MyTriangleFE(
mField, constant_area.commonData,
7672 "LAMBDA_CRACKFRONT_AREA"));
7675 constant_area.feLhsPtr->petscQ = Q;
7677 constant_area.feLhsPtr->petscQ = PETSC_NULL;
7679 constant_area.feLhsPtr->getOpPtrVector().push_back(
7681 constant_area.commonData));
7682 constant_area.feLhsPtr->getOpPtrVector().push_back(
7686 ConstantArea tangent_constrain(
mField);
7687 tangent_constrain.feLhsPtr = boost::shared_ptr<ConstantArea::MyTriangleFE>(
7688 new ConstantArea::MyTriangleFE(
mField, tangent_constrain.commonData,
7689 "LAMBDA_CRACKFRONT_AREA_TANGENT"));
7692 tangent_constrain.feLhsPtr->petscQ = Q;
7694 tangent_constrain.feLhsPtr->petscQ = PETSC_NULL;
7696 tangent_constrain.feLhsPtr->getOpPtrVector().push_back(
7698 tangent_constrain.commonData));
7699 tangent_constrain.feLhsPtr->getOpPtrVector().push_back(
7701 tangent_constrain.commonData));
7705 constant_area.feLhsPtr.get());
7707 tangent_constrain.feLhsPtr.get());
7717 PetscViewerASCIIOpen(PETSC_COMM_WORLD,
"C.m", &viewer);
7718 PetscViewerPushFormat(viewer, PETSC_VIEWER_ASCII_MATLAB);
7720 PetscViewerPopFormat(viewer);
7721 PetscViewerDestroy(&viewer);
◆ calculateGriffithForce()
MoFEMErrorCode FractureMechanics::CrackPropagation::calculateGriffithForce |
( |
DM |
dm, |
|
|
const double |
gc, |
|
|
Vec |
f_griffith, |
|
|
const int |
verb = QUIET , |
|
|
const bool |
debug = false |
|
) |
| |
calculate Griffith (driving) force
Definition at line 7962 of file CrackPropagation.cpp.
7970 CHKERR VecSetOption(f_griffith, VEC_IGNORE_NEGATIVE_INDICES, PETSC_TRUE);
7972 boost::scoped_ptr<GriffithForceElement> griffith_force_element(
7973 new GriffithForceElement(
mField));
7974 griffith_force_element->blockData[0].gc = gc;
7977 griffith_force_element->blockData[0]);
7978 gC = griffith_force_element->blockData[0].gc;
7980 griffith_force_element->feRhs.getOpPtrVector().push_back(
7981 new GriffithForceElement::OpCalculateGriffithForce(
7983 griffith_force_element->commonData));
7984 griffith_force_element->feRhs.getOpPtrVector().push_back(
7986 griffith_force_element->blockData[0],
7987 griffith_force_element->commonData));
7988 griffith_force_element->feRhs.snes_f = f_griffith;
7989 CHKERR VecZeroEntries(f_griffith);
7990 CHKERR VecGhostUpdateBegin(f_griffith, INSERT_VALUES, SCATTER_FORWARD);
7991 CHKERR VecGhostUpdateEnd(f_griffith, INSERT_VALUES, SCATTER_FORWARD);
7993 &griffith_force_element->feRhs);
7994 CHKERR VecAssemblyBegin(f_griffith);
7995 CHKERR VecAssemblyEnd(f_griffith);
7996 CHKERR VecGhostUpdateBegin(f_griffith, ADD_VALUES, SCATTER_REVERSE);
7997 CHKERR VecGhostUpdateEnd(f_griffith, ADD_VALUES, SCATTER_REVERSE);
7998 CHKERR VecGhostUpdateBegin(f_griffith, INSERT_VALUES, SCATTER_FORWARD);
7999 CHKERR VecGhostUpdateEnd(f_griffith, INSERT_VALUES, SCATTER_FORWARD);
8001 PostProcVertexMethod ent_method_griffith_force(
mField, f_griffith,
8003 CHKERR VecGhostUpdateBegin(f_griffith, INSERT_VALUES, SCATTER_FORWARD);
8004 CHKERR VecGhostUpdateEnd(f_griffith, INSERT_VALUES, SCATTER_FORWARD);
◆ calculateMaterialForcesDM()
MoFEMErrorCode FractureMechanics::CrackPropagation::calculateMaterialForcesDM |
( |
DM |
dm, |
|
|
Vec |
q, |
|
|
Vec |
f, |
|
|
const int |
verb = QUIET , |
|
|
const bool |
debug = false |
|
) |
| |
assemble material forces, by running material finite element instance
- Parameters
-
dm | dm used to calculate material forces, sub-dm of dm_crack_propagation |
q | DoF sub-vector for crack propagation DM |
f | Rhs sub-vector for crack propagation DM |
verb | compilation parameter determining the amount of information printed |
debug | flag for debugging |
- Returns
- error code
Definition at line 7416 of file CrackPropagation.cpp.
7425 CHKERR VecSetOption(
f, VEC_IGNORE_NEGATIVE_INDICES, PETSC_TRUE);
7427 CHKERR VecGhostUpdateBegin(
f, INSERT_VALUES, SCATTER_FORWARD);
7428 CHKERR VecGhostUpdateEnd(
f, INSERT_VALUES, SCATTER_FORWARD);
7433 #ifdef __ANALITICAL_TRACTION__
7439 #endif // __ANALITICAL_TRACTION__
7442 CHKERR VecGhostUpdateBegin(
f, ADD_VALUES, SCATTER_REVERSE);
7443 CHKERR VecGhostUpdateEnd(
f, ADD_VALUES, SCATTER_REVERSE);
7444 CHKERR VecGhostUpdateBegin(
f, INSERT_VALUES, SCATTER_FORWARD);
7445 CHKERR VecGhostUpdateEnd(
f, INSERT_VALUES, SCATTER_FORWARD);
7447 CHKERR VecView(
f, PETSC_VIEWER_STDOUT_WORLD);
7451 PostProcVertexMethod ent_method_material_force(
mField,
f,
"MATERIAL_FORCE");
7452 CHKERR VecGhostUpdateBegin(
f, INSERT_VALUES, SCATTER_FORWARD);
7453 CHKERR VecGhostUpdateEnd(
f, INSERT_VALUES, SCATTER_FORWARD);
7456 PostProcVertexMethod ent_method_material_position(
mField, q,
7457 "MESH_NODE_POSITIONS");
7458 CHKERR VecGhostUpdateBegin(q, INSERT_VALUES, SCATTER_FORWARD);
7459 CHKERR VecGhostUpdateEnd(q, INSERT_VALUES, SCATTER_FORWARD);
7461 ent_method_material_position, 0,
◆ calculateReleaseEnergy()
MoFEMErrorCode FractureMechanics::CrackPropagation::calculateReleaseEnergy |
( |
DM |
dm, |
|
|
Vec |
f_material_proj, |
|
|
Vec |
f_griffith_proj, |
|
|
Vec |
f_lambda, |
|
|
const double |
gc, |
|
|
const int |
verb = QUIET , |
|
|
const bool |
debug = true |
|
) |
| |
calculate release energy
Definition at line 7731 of file CrackPropagation.cpp.
7737 PetscPrintf(PETSC_COMM_WORLD,
"No crack front projection data structure\n");
7747 CHKERR VecGetSize(f_material_proj, &
N);
7748 CHKERR VecGetLocalSize(f_material_proj, &
n);
7749 CHKERR VecGetSize(f_lambda, &
M);
7750 CHKERR VecGetLocalSize(f_lambda, &
m);
7753 CHKERR MatShellSetOperation(RT, MATOP_MULT,
7757 CHKERR MatMult(RT, f_material_proj, f_lambda);
7758 CHKERR VecScale(f_lambda, -1);
7759 CHKERR VecGhostUpdateBegin(f_lambda, INSERT_VALUES, SCATTER_FORWARD);
7760 CHKERR VecGhostUpdateEnd(f_lambda, INSERT_VALUES, SCATTER_FORWARD);
7765 CHKERR VecView(f_lambda, PETSC_VIEWER_STDOUT_WORLD);
7767 PostProcVertexMethod ent_method_g1(
mField, f_lambda,
"G1");
7768 PostProcVertexMethod ent_method_g3(
mField, f_lambda,
"G3");
7781 CHKERR VecGetArray(f_lambda, &a_lambda);
7788 for (; it != hi_it; ++it) {
7789 double val = a_lambda[it->get()->getPetscLocalDofIdx()];
7790 if (!std::isnormal(val)) {
7792 "Value vector is not a number val = %3.4f", val);
7794 if (it->get()->getName() ==
"LAMBDA_CRACKFRONT_AREA") {
7795 mapG1[it->get()->getEnt()] = val;
7796 }
else if (it->get()->getName() ==
"LAMBDA_CRACKFRONT_AREA_TANGENT") {
7797 mapG3[it->get()->getEnt()] = val;
7800 "Should not happen");
7803 CHKERR VecRestoreArray(f_lambda, &a_lambda);
7806 Vec f_mat_scat, f_griffith_scat;
7809 CHKERR VecDuplicate(f_mat_scat, &f_griffith_scat);
7811 INSERT_VALUES, SCATTER_FORWARD);
7813 INSERT_VALUES, SCATTER_FORWARD);
7815 f_griffith_scat, INSERT_VALUES, SCATTER_FORWARD);
7817 f_griffith_scat, INSERT_VALUES, SCATTER_FORWARD);
7818 CHKERR VecGhostUpdateBegin(f_mat_scat, INSERT_VALUES, SCATTER_FORWARD);
7819 CHKERR VecGhostUpdateEnd(f_mat_scat, INSERT_VALUES, SCATTER_FORWARD);
7820 CHKERR VecGhostUpdateBegin(f_griffith_scat, INSERT_VALUES, SCATTER_FORWARD);
7821 CHKERR VecGhostUpdateEnd(f_griffith_scat, INSERT_VALUES, SCATTER_FORWARD);
7822 double *a_material, *a_griffith;
7823 CHKERR VecGetArray(f_mat_scat, &a_material);
7824 CHKERR VecGetArray(f_griffith_scat, &a_griffith);
7831 for (; it != hi_it; it++) {
7832 if (it->get()->getName() ==
"MESH_NODE_POSITIONS") {
7836 a_material[it->get()->getPetscLocalDofIdx()];
7839 a_griffith[it->get()->getPetscLocalDofIdx()];
7842 "Should not happen");
7845 CHKERR VecRestoreArray(f_mat_scat, &a_material);
7846 CHKERR VecRestoreArray(f_griffith_scat, &a_griffith);
7847 CHKERR VecDestroy(&f_mat_scat);
7848 CHKERR VecDestroy(&f_griffith_scat);
7852 for (map<EntityHandle, double>::iterator mit =
mapG1.begin();
7853 mit !=
mapG1.end(); mit++) {
7855 double g1 = mit->second;
7857 double g3 =
mapG3[ent];
7862 double g2 = sqrt(fabs(
j *
j - g1 * g1 - g3 * g3));
7866 ss <<
"griffith force at ent ";
7867 ss << setw(5) << ent;
7870 ss <<
" " << setw(10) << setprecision(4) << coords[0];
7871 ss <<
" " << setw(10) << setprecision(4) << coords[1];
7872 ss <<
" " << setw(10) << setprecision(4) << coords[2];
7874 ss <<
"\t\tg1 " << scientific << setprecision(6) << g1;
7875 ss <<
" / " << scientific << setprecision(6) <<
j;
7876 ss <<
" ( " << scientific << setprecision(6) << g1 /
j <<
" )";
7878 ss <<
"\t\tg2 " << scientific << setprecision(6) << g2;
7879 ss <<
" ( " << scientific << setprecision(6) << g2 /
j <<
" )";
7881 ss <<
"\t\tg3 " << scientific << setprecision(6) << g3;
7882 ss <<
" ( " << scientific << setprecision(6) << g3 /
j <<
" )";
7884 ss <<
"\t relative error (gc-g)/gc ";
7885 ss << scientific << setprecision(6) << (gc - g1) / gc;
7886 ss <<
" / " << scientific << setprecision(6) << (gc -
j) / gc;
7889 MOFEM_LOG(
"CPWorld", Sev::inform) << ss.str();
7900 CHKERR VecDestroy(&vec_max);
◆ calculateSmoothingForceFactor()
MoFEMErrorCode FractureMechanics::CrackPropagation::calculateSmoothingForceFactor |
( |
const int |
verb = QUIET , |
|
|
const bool |
debug = true |
|
) |
| |
Definition at line 7524 of file CrackPropagation.cpp.
7528 PetscFunctionReturn(0);
7529 auto get_tag_data = [
this](std::string tag_name) {
7536 auto griffith_force = get_tag_data(
"GRIFFITH_FORCE");
7537 auto smoothing_force = get_tag_data(
"SMOOTHING_FORCE");
7539 auto get_node_tensor = [](std::vector<double> &data) {
7541 &data[0], &data[1], &data[2]);
7543 auto t_griffith_force = get_node_tensor(griffith_force);
7544 auto t_smoothing_force = get_node_tensor(smoothing_force);
7548 auto get_magnitude = [](
auto t) {
7550 return sqrt(
t(
i) *
t(
i));
7552 const double griffith_mgn = get_magnitude(t_griffith_force);
7553 const double smoothing_mg = get_magnitude(t_smoothing_force);
7555 PetscPrintf(
mField.
get_comm(),
"Node smoothing force fraction %ld %6.4e\n",
7558 ++t_smoothing_force;
◆ calculateSmoothingForcesDM()
MoFEMErrorCode FractureMechanics::CrackPropagation::calculateSmoothingForcesDM |
( |
DM |
dm, |
|
|
Vec |
q, |
|
|
Vec |
f, |
|
|
const int |
verb = QUIET , |
|
|
const bool |
debug = false |
|
) |
| |
assemble smoothing forces, by running material finite element instance
- Parameters
-
dm | crack propagation dm that is composition of elastic DM and material DM |
q | DoF sub-vector for crack propagation DM |
f | Rhs sub-vector for crack propagation DM |
verb | compilation parameter determining the amount of information printed |
debug | flag for debugging |
- Returns
- error code
Definition at line 7476 of file CrackPropagation.cpp.
7481 PetscFunctionReturn(0);
7484 CHKERR VecSetOption(
f, VEC_IGNORE_NEGATIVE_INDICES, PETSC_TRUE);
7486 CHKERR VecGhostUpdateBegin(
f, INSERT_VALUES, SCATTER_FORWARD);
7487 CHKERR VecGhostUpdateEnd(
f, INSERT_VALUES, SCATTER_FORWARD);
7494 CHKERR VecGhostUpdateBegin(
f, ADD_VALUES, SCATTER_REVERSE);
7495 CHKERR VecGhostUpdateEnd(
f, ADD_VALUES, SCATTER_REVERSE);
7496 CHKERR VecGhostUpdateBegin(
f, INSERT_VALUES, SCATTER_FORWARD);
7497 CHKERR VecGhostUpdateEnd(
f, INSERT_VALUES, SCATTER_FORWARD);
7499 CHKERR VecView(
f, PETSC_VIEWER_STDOUT_WORLD);
7503 PostProcVertexMethod ent_method_material_smoothing(
mField,
f,
7505 CHKERR VecGhostUpdateBegin(
f, INSERT_VALUES, SCATTER_FORWARD);
7506 CHKERR VecGhostUpdateEnd(
f, INSERT_VALUES, SCATTER_FORWARD);
7508 ent_method_material_smoothing, 0,
◆ calculateSurfaceProjectionMatrix()
MoFEMErrorCode FractureMechanics::CrackPropagation::calculateSurfaceProjectionMatrix |
( |
DM |
dm_front, |
|
|
DM |
dm_project, |
|
|
const std::vector< int > & |
ids, |
|
|
const int |
verb = QUIET , |
|
|
const bool |
debug = false |
|
) |
| |
assemble projection matrices
Definition at line 7563 of file CrackPropagation.cpp.
7591 Range contact_faces;
7596 for (
auto id : ids) {
7597 bool crack_surface =
7598 (
id == getInterface<CPMeshCut>()->getCrackSurfaceId()) ?
true :
false;
7599 FaceOrientation orientation(crack_surface, contact_faces,
7602 surface_constrain.setOperatorsConstrainOnly(
7604 "LAMBDA_SURFACE" + boost::lexical_cast<std::string>(
id),
7605 "MESH_NODE_POSITIONS");
7606 std::string fe_name = (
id == getInterface<CPMeshCut>()->getCrackSurfaceId())
7611 &surface_constrain.feLhs);
7614 auto get_edges_block_set = [
this]() {
7615 return getInterface<CPMeshCut>()->getEdgesBlockSet();
7617 if (get_edges_block_set()) {
7620 "MESH_NODE_POSITIONS");
◆ cleanSingularElementMatrialPositions()
MoFEMErrorCode FractureMechanics::CrackPropagation::cleanSingularElementMatrialPositions |
( |
| ) |
|
◆ createBcDM()
Create problem to calculate boundary conditions.
- Parameters
-
dm | newly created boundary condition DM |
prb_name | problem name |
bit | auxiliary bit ref level |
mask | mask ref level for problem |
- Returns
- error code
- Note
- BcDM is only useed for testing, to enforce boundary conditions staisifing anlylitical solution to which numerical solution is compared.
Definition at line 3625 of file CrackPropagation.cpp.
3635 #ifdef __ANALITICAL_DISPLACEMENT__
3636 if (meshset_manager_ptr->
checkMeshset(
"ANALITICAL_DISP")) {
3639 &cubit_meshset_ptr);
3643 if (!tris.empty()) {
3655 #endif //__ANALITICAL_DISPLACEMENT__
◆ createCrackFrontAreaDM()
create DM to calculate Griffith energy
- Parameters
-
dm | dm to project material forces on crack surfaces, sub-dm of dm_crack_propagation |
dm_material | material DM |
prb_name | problem name |
verb | compilation parameter determining the amount of information printed |
- Returns
- error code
Definition at line 3776 of file CrackPropagation.cpp.
◆ createCrackPropagationDM()
Create DM by composition of elastic DM and material DM.
- Parameters
-
dm | composition of elastic DM and material DM |
prb_name | problem name |
dm_elastic | elastic DM |
dm_material | material DM |
bit | domain bit ref level |
mask | mask ref level for problem |
- Returns
- error code
Definition at line 3431 of file CrackPropagation.cpp.
3471 for (std::vector<std::string>::const_iterator it = fe_list.begin();
3472 it != fe_list.end(); it++)
3478 #ifdef __ANALITICAL_TRACTION__
3500 PetscSection section;
3502 CHKERR DMSetDefaultSection(dm, section);
3503 CHKERR DMSetDefaultGlobalSection(dm, section);
3505 CHKERR PetscSectionDestroy(§ion);
◆ createDMs()
Crate DMs for all problems and sub problems.
- Parameters
-
dm_elastic | elastic DM |
dm_eiegn_elastci | eigen_elastic DM to solve proble wih eigen stresses |
dm_material | material DM |
dm_crack_propagation | composition of elastic DM and material DM |
dm_material_forces | used to calculate material forces, sub-dm of dm_crack_propagation |
dm_surface_projection | dm to project material forces on surfaces, sub-dm of dm_crack_propagation |
dm_crack_srf_area | dm to project material forces on crack surfaces, sub-dm of dm_crack_propagation |
surface_ids | IDs of surface meshsets |
fe_surf_proj_list | name of elements on surface elements |
- Returns
- error code
Definition at line 9571 of file CrackPropagation.cpp.
9592 fe_surf_proj_list,
false);
9594 dm_elastic, dm_material, bit1,
9597 "MATERIAL_FORCES",
QUIET);
9599 "SURFACE_PROJECTION", surface_ids,
9600 fe_surf_proj_list,
QUIET);
9602 "CRACK_SURFACE_AREA",
QUIET);
◆ createEigenElasticDM()
Create elastic problem DM.
- Parameters
-
dm | elastic DM |
prb_name | problem name |
bit | domain bit ref level |
mask | mask ref level for problem |
- Returns
- error code
Definition at line 3562 of file CrackPropagation.cpp.
3581 #ifdef __ANALITICAL_TRACTION__
3590 auto remove_higher_dofs_than_approx_dofs_from_eigen_elastic = [&]() {
3595 CHKERR prb_mng->removeDofsOnEntities(
"EIGEN_ELASTIC",
"SPATIAL_POSITION",
3599 CHKERR remove_higher_dofs_than_approx_dofs_from_eigen_elastic();
3603 PetscSection section;
3605 CHKERR DMSetDefaultSection(dm, section);
3606 CHKERR DMSetDefaultGlobalSection(dm, section);
3608 CHKERR PetscSectionDestroy(§ion);
3616 "LAMBDA_ARC_LENGTH");
3618 auto arc_snes_ctx = boost::make_shared<ArcLengthSnesCtx>(
◆ createElasticDM()
Create elastic problem DM.
- Parameters
-
dm | elastic DM |
prb_name | problem name |
bit | domain bit ref level |
mask | mask ref level for problem |
- Returns
- error code
Definition at line 3510 of file CrackPropagation.cpp.
3529 #ifdef __ANALITICAL_TRACTION__
3540 PetscSection section;
3542 CHKERR DMSetDefaultSection(dm, section);
3543 CHKERR DMSetDefaultGlobalSection(dm, section);
3545 CHKERR PetscSectionDestroy(§ion);
3552 arcCtx = boost::make_shared<ArcLengthCtx>(
mField, problem_ptr->getName(),
3553 "LAMBDA_ARC_LENGTH");
3555 auto arc_snes_ctx = boost::make_shared<ArcLengthSnesCtx>(
◆ createMaterialDM()
Create DM fto calculate material problem.
- Parameters
-
dm | used to calculate material forces, sub-dm of dm_crack_propagation |
prb_name | problem name |
bit | material domain |
mask | dof mask ref level for problem |
fe_list | name of elements on surface elements |
debug | flag for debugging |
- Returns
- error code
Definition at line 3659 of file CrackPropagation.cpp.
3669 for (
auto &fe : fe_list)
3675 #ifdef __ANALITICAL_TRACTION__
3694 PetscSection section;
3696 CHKERR DMSetDefaultSection(dm, section);
3697 CHKERR DMSetDefaultGlobalSection(dm, section);
3699 CHKERR PetscSectionDestroy(§ion);
3702 std::vector<std::string> fe_lists;
3707 for (FiniteElement_multiIndex::iterator fit = fe_ptr->begin();
3708 fit != fe_ptr->end(); fit++) {
3709 if ((fit->get()->getId() & problem_ptr->
getBitFEId()).any()) {
3710 std::string fe_name = fit->get()->getName();
3713 prb_name, fe_name, &meshset);
3715 (prb_name +
"_" + fe_name +
".h5m").c_str(),
"MOAB",
3716 "PARALLEL=WRITE_PART", &meshset, 1);
◆ createMaterialForcesDM()
Create DM for calculation of material forces (sub DM of DM material)
- Parameters
-
dm | used to calculate material forces, sub-dm of dm_crack_propagation |
prb_name | problem name |
verb | compilation parameter determining the amount of information printed |
- Returns
- error code
Definition at line 3724 of file CrackPropagation.cpp.
3738 #ifdef __ANALITICAL_TRACTION__
◆ createProblemDataStructures()
MoFEMErrorCode FractureMechanics::CrackPropagation::createProblemDataStructures |
( |
const std::vector< int > |
surface_ids, |
|
|
const int |
verb = QUIET , |
|
|
const bool |
debug = false |
|
) |
| |
Construct problem data structures.
- Parameters
-
surface_ids | surface_ids meshsets Ids on body skin |
verb | verbosity level |
debug | |
- Returns
- error code
Definition at line 9525 of file CrackPropagation.cpp.
9537 ->writeEntitiesAllBitLevelsByType(
BitRefLevel().set(), MBTET,
9538 "all_bits.vtk",
"VTK",
"");
◆ createSurfaceProjectionDM()
MoFEMErrorCode FractureMechanics::CrackPropagation::createSurfaceProjectionDM |
( |
SmartPetscObj< DM > & |
dm, |
|
|
SmartPetscObj< DM > |
dm_material, |
|
|
const std::string |
prb_name, |
|
|
const std::vector< int > |
surface_ids, |
|
|
const std::vector< std::string > |
fe_list, |
|
|
const int |
verb = QUIET |
|
) |
| |
create DM to calculate projection matrices (sub DM of DM material)
- Parameters
-
dm | DM to project material forces on surfaces, sub-dm of dm_crack_propagation |
dm_material | material DM |
prb_name | problem name |
surface_ids | IDs of surface meshsets |
fe_list | name of elements on surface elements |
verb | compilation parameter determining the amount of information printed |
- Returns
- error code
Definition at line 3747 of file CrackPropagation.cpp.
3756 for (
auto id : surface_ids) {
3758 dm, (
"LAMBDA_SURFACE" + boost::lexical_cast<std::string>(
id)).c_str());
3761 for (
auto fe : fe_list) {
3764 auto get_edges_block_set = [
this]() {
3765 return getInterface<CPMeshCut>()->getEdgesBlockSet();
3767 if (get_edges_block_set()) {
◆ declareArcLengthFE()
create arc-length element entity and declare elemets
Definition at line 2344 of file CrackPropagation.cpp.
2349 Range arc_fe_meshsets;
2352 arc_fe_meshsets.insert(fit->get()->getEnt());
2353 if (
auto ptr = fit->get()->getPartProcPtr())
2364 "LAMBDA_ARC_LENGTH");
2366 "LAMBDA_ARC_LENGTH");
2369 "LAMBDA_ARC_LENGTH");
2381 "ARC_LENGTH",
false);
2386 if (
auto ptr = fit->get()->getPartProcPtr())
◆ declareBothSidesFE()
Definition at line 3001 of file CrackPropagation.cpp.
3006 auto get_prisms_level = [&](
auto bit) {
3009 bit, mask, MBPRISM, prisms_level);
3011 prisms_level = intersect(
bitProcEnts, prisms_level);
3013 return prisms_level;
3016 auto get_crack_prisms = [&](
auto prisms_level) {
3019 return crack_prisms;
3022 auto add_both_sides_fe = [&](
const std::string fe_name,
3023 const std::string lambda_field_name,
3024 const std::string spatial_field,
Range ents) {
3029 if (!ents.empty()) {
3052 CHKERR add_both_sides_fe(
"CLOSE_CRACK",
"LAMBDA_CLOSE_CRACK",
3054 get_crack_prisms(get_prisms_level(bit_spatial)));
3056 CHKERR add_both_sides_fe(
"BOTH_SIDES_OF_CRACK",
"LAMBDA_BOTH_SIDES",
3057 "MESH_NODE_POSITIONS",
3058 get_crack_prisms(get_prisms_level(bit_material)));
3060 Range contact_prisms =
3062 CHKERR add_both_sides_fe(
"BOTH_SIDES_OF_CONTACT",
3063 "LAMBDA_BOTH_SIDES_CONTACT",
"MESH_NODE_POSITIONS",
◆ declareCrackSurfaceFE()
Definition at line 3289 of file CrackPropagation.cpp.
3299 bit, mask, MBTRI, level_tris);
3300 Range crack_surface;
3305 moab::Interface::UNION);
3306 crack_surface = intersect(crack_surface, proc_tris);
3308 Range crack_front_adj_tris;
3310 crackFrontNodes, 2,
false, crack_front_adj_tris, moab::Interface::UNION);
3311 crack_front_adj_tris = intersect(crack_front_adj_tris,
crackFaces);
3312 crack_front_adj_tris = intersect(crack_front_adj_tris, level_tris);
3315 "LAMBDA_SURFACE" + boost::lexical_cast<std::string>(
3316 getInterface<CPMeshCut>()->getCrackSurfaceId());
3323 "MESH_NODE_POSITIONS");
3327 "MESH_NODE_POSITIONS");
3331 "MESH_NODE_POSITIONS");
3334 crack_surface,
QUIET);
3340 ents = subtract(ents, crack_surface);
3341 if (!ents.empty()) {
3351 std::ostringstream file;
3354 CHKERR moab.create_meshset(MESHSET_SET, meshset);
3355 CHKERR moab.add_entities(meshset, crack_surface);
3356 CHKERR moab.write_file(file.str().c_str(),
"VTK",
"", &meshset, 1);
3357 CHKERR moab.delete_entities(&meshset, 1);
3363 "LAMBDA_CRACKFRONT_AREA");
3365 "MESH_NODE_POSITIONS");
3367 "LAMBDA_CRACKFRONT_AREA");
3369 "MESH_NODE_POSITIONS");
3371 "MESH_NODE_POSITIONS");
3373 "LAMBDA_CRACKFRONT_AREA");
3378 "CRACKFRONT_AREA_TANGENT_ELEM",
"LAMBDA_CRACKFRONT_AREA_TANGENT");
3380 "CRACKFRONT_AREA_TANGENT_ELEM",
"MESH_NODE_POSITIONS");
3382 "CRACKFRONT_AREA_TANGENT_ELEM",
"LAMBDA_CRACKFRONT_AREA_TANGENT");
3384 "CRACKFRONT_AREA_TANGENT_ELEM",
"MESH_NODE_POSITIONS");
3386 "CRACKFRONT_AREA_TANGENT_ELEM",
"MESH_NODE_POSITIONS");
3388 "CRACKFRONT_AREA_TANGENT_ELEM",
"LAMBDA_CRACKFRONT_AREA_TANGENT");
3392 crack_front_adj_tris,
QUIET);
3394 auto remeve_ents_from_fe =
3395 [
this, &crack_front_adj_tris](
const std::string fe_name) {
3399 ents = subtract(ents, crack_front_adj_tris);
3400 if (!ents.empty()) {
3405 CHKERR remeve_ents_from_fe(
"CRACKFRONT_AREA_ELEM");
3406 CHKERR remeve_ents_from_fe(
"CRACKFRONT_AREA_TANGENT_ELEM");
3409 "CRACKFRONT_AREA_ELEM");
3411 crack_front_adj_tris, MBTRI,
"CRACKFRONT_AREA_TANGENT_ELEM");
3414 std::ostringstream file;
3417 CHKERR moab.create_meshset(MESHSET_SET, meshset);
3418 CHKERR moab.add_entities(meshset, crack_front_adj_tris);
3419 CHKERR moab.write_file(file.str().c_str(),
"VTK",
"", &meshset, 1);
3420 CHKERR moab.delete_entities(&meshset, 1);
3424 &crack_front_adj_tris, verb);
3426 &crack_front_adj_tris, verb);
◆ declareEdgeFE()
Definition at line 3179 of file CrackPropagation.cpp.
3186 auto get_edges_block_ents = [
this, meshset_manager_ptr]() {
3187 EntityHandle edge_block_set = getInterface<CPMeshCut>()->getEdgesBlockSet();
3189 if (meshset_manager_ptr->checkMeshset(edge_block_set,
BLOCKSET)) {
3190 CHKERR meshset_manager_ptr->getEntitiesByDimension(
3191 edge_block_set,
BLOCKSET, 1, meshset_ents,
true);
3193 return meshset_ents;
3196 auto get_skin = [
this, bit_ref_manager_ptr]() {
3198 CHKERR bit_ref_manager_ptr->getEntitiesByTypeAndRefLevel(
3202 CHKERR skin.find_skin(0, tets,
false, skin_faces);
3206 auto intersect_with_skin_edges = [
this, get_edges_block_ents, get_skin]() {
3209 moab::Interface::UNION);
3210 return intersect(get_edges_block_ents(), adj_edges);
3212 Range edges_ents = intersect_with_skin_edges();
3214 Range contact_faces;
3219 boost::shared_ptr<SurfaceSlidingConstrains::DriverElementOrientation>(
3220 new FaceOrientation(
false, contact_faces,
mapBitLevel[
"mesh_cut"]));
3229 Range faces = get_skin();
3234 auto intersect_with_bit_level_edges = [bit_ref_manager_ptr, &
bit,
3237 CHKERR bit_ref_manager_ptr->getEntitiesByTypeAndRefLevel(
3239 return intersect(edges_ents, level_edges);
3241 edges_ents = intersect_with_bit_level_edges();
3243 auto intersect_with_proc_edges = [
this, &edges_ents, proc_only]() {
3247 bitProcEnts, 1,
false, proc_edges, moab::Interface::UNION);
3248 return intersect(edges_ents, proc_edges);
3252 edges_ents = intersect_with_proc_edges();
3259 auto add_field = [
this, &fe_name](std::string &&
field_name) {
3266 CHKERR add_field(
"MESH_NODE_POSITIONS");
3267 CHKERR add_field(
"LAMBDA_EDGE");
3272 "edges_normals_on_proc_" +
3278 Range ents_to_remove;
3280 ents_to_remove = subtract(ents_to_remove, edges_ents);
◆ declareElasticFE()
declare elastic finite elements
Definition at line 2205 of file CrackPropagation.cpp.
2213 bit1, mask1, MBTET, tets_level1);
2215 tets_level1 = intersect(
bitProcEnts, tets_level1);
2217 Range tets_not_level2;
2219 bit2, mask2, MBTET, tets_not_level2);
2220 tets_not_level2 = subtract(tets_level1, tets_not_level2);
2225 "SPATIAL_POSITION");
2227 "SPATIAL_POSITION");
2229 "SPATIAL_POSITION");
2231 "MESH_NODE_POSITIONS");
2235 Range current_ents_with_fe;
2237 current_ents_with_fe);
2238 Range ents_to_remove;
2239 ents_to_remove = subtract(current_ents_with_fe, tets_level1);
2250 "SPATIAL_POSITION");
2252 "SPATIAL_POSITION");
2254 "SPATIAL_POSITION");
2256 "MESH_NODE_POSITIONS");
2260 Range current_ents_with_fe;
2262 current_ents_with_fe);
2263 Range ents_to_remove;
2264 ents_to_remove = subtract(current_ents_with_fe, tets_not_level2);
2278 Range proc_tris, skin_tris, tets_level;
2280 moab::Interface::UNION);
2283 bit1, mask1, MBTET, tets_level);
2285 CHKERR skin.find_skin(0, tets_level,
false, skin_tris);
2286 skin_tris = intersect(skin_tris, proc_tris);
2291 "SPATIAL_POSITION");
2293 "SPATIAL_POSITION");
2295 "SPATIAL_POSITION");
2297 "MESH_NODE_POSITIONS");
2300 "SKIN",
"EIGEN_SPATIAL_POSITIONS");
2306 "MESH_NODE_POSITIONS");
2309 auto cn_value_ptr = boost::make_shared<double>(
cnValue);
2326 "MORTAR_CONTACT",
"SPATIAL_POSITION",
"LAMBDA_CONTACT",
2328 "EIGEN_SPATIAL_POSITIONS");
2332 Range all_slave_tris;
2337 "CONTACT_POST_PROC",
"SPATIAL_POSITION",
"LAMBDA_CONTACT",
2338 "MESH_NODE_POSITIONS", all_slave_tris);
◆ declareExternalForcesFE()
Definition at line 2392 of file CrackPropagation.cpp.
2400 bit, mask, MBTET, tets_level);
2407 dofs_ents.merge(tets_level);
2408 for (
int dd = 1;
dd != 3;
dd++) {
2409 CHKERR moab.get_adjacencies(tets_level,
dd,
false, dofs_ents,
2410 moab::Interface::UNION);
2413 rval = moab.get_connectivity(tets_level, dofs_nodes,
true);
2415 Range lower_dim_ents = unite(dofs_ents, dofs_nodes);
2417 #ifdef __ANALITICAL_TRACTION__
2421 "SPATIAL_POSITION");
2423 "SPATIAL_POSITION");
2425 "SPATIAL_POSITION");
2427 "MESH_NODE_POSITIONS");
2430 if (meshset_manager_ptr->
checkMeshset(
"ANALITICAL_TRACTION")) {
2433 &cubit_meshset_ptr);
2437 tris = intersect(tris, lower_dim_ents);
2439 "ANALITICAL_TRACTION");
2443 #endif //__ANALITICAL_TRACTION__
2447 "SPATIAL_POSITION");
2449 "SPATIAL_POSITION");
2451 "SPATIAL_POSITION");
2453 "MESH_NODE_POSITIONS");
2464 moab::Interface::UNION);
2472 nodes = subtract(nodes, tris_nodes);
2473 nodes = subtract(nodes, edges_nodes);
2474 nodes = intersect(lower_dim_ents, nodes);
2475 edges = subtract(edges, tris_edges);
2476 edges = intersect(lower_dim_ents, edges);
2477 tris = intersect(lower_dim_ents, tris);
2485 const string block_set_force_name(
"FORCE");
2488 if (it->getName().compare(0, block_set_force_name.length(),
2489 block_set_force_name) == 0) {
2490 std::vector<double> mydata;
2491 CHKERR it->getAttributes(mydata);
2494 for (
unsigned int ii = 0; ii < mydata.size(); ii++) {
2495 force[ii] = mydata[ii];
2497 if (force.size() != 3) {
2499 "Force vector not given");
2513 "SPATIAL_POSITION");
2515 "SPATIAL_POSITION");
2517 "SPATIAL_POSITION");
2519 "MESH_NODE_POSITIONS");
2525 tris = intersect(lower_dim_ents, tris);
2529 const string block_set_linear_pressure_name(
"LINEAR_PRESSURE");
2531 if (it->getName().compare(0, block_set_linear_pressure_name.length(),
2532 block_set_linear_pressure_name) == 0) {
2536 tris = intersect(lower_dim_ents, tris);
2544 const string block_set_pressure_name(
"PRESSURE");
2546 if (it->getName().compare(0, block_set_pressure_name.length(),
2547 block_set_pressure_name) == 0) {
2548 std::vector<double> mydata;
2549 CHKERR it->getAttributes(mydata);
2551 for (
unsigned int ii = 0; ii < mydata.size(); ii++) {
2552 pressure[ii] = mydata[ii];
2554 if (pressure.empty()) {
2556 "Pressure not given");
2569 #ifdef __ANALITICAL_DISPLACEMENT__
2573 if (meshset_manager_ptr->
checkMeshset(
"ANALITICAL_DISP")) {
2576 &cubit_meshset_ptr);
2580 if (!tris.empty()) {
2583 "SPATIAL_POSITION");
2585 "SPATIAL_POSITION");
2587 "SPATIAL_POSITION");
2589 "BC_FE",
"MESH_NODE_POSITIONS");
2590 tris = intersect(lower_dim_ents, tris);
2596 #endif //__ANALITICAL_DISPLACEMENT__
◆ declareFrontFE()
Definition at line 2917 of file CrackPropagation.cpp.
2926 "MESH_NODE_POSITIONS");
2928 "MESH_NODE_POSITIONS");
2930 "MESH_NODE_POSITIONS");
2931 Range crack_front_adj_tris;
2933 crackFrontNodes, 2,
false, crack_front_adj_tris, moab::Interface::UNION);
2934 crack_front_adj_tris = intersect(crack_front_adj_tris,
crackFaces);
2937 bit, mask, MBTRI, tris_level);
2938 crack_front_adj_tris = intersect(crack_front_adj_tris, tris_level);
2940 Range ents_to_remove;
2943 ents_to_remove = subtract(ents_to_remove, crack_front_adj_tris);
2945 ents_to_remove, verb);
2948 "GRIFFITH_FORCE_ELEMENT");
2950 &crack_front_adj_tris, verb);
2952 #ifdef __ANALITICAL_TRACTION__
2956 bit, mask, MBTET, tets_level);
2962 dofs_ents.merge(tets_level);
2963 for (
int dd = 1;
dd != 3;
dd++) {
2964 CHKERR moab.get_adjacencies(tets_level,
dd,
false, dofs_ents,
2965 moab::Interface::UNION);
2968 CHKERR moab.get_connectivity(tets_level, dofs_nodes,
true);
2969 Range lower_dim_ents = unite(dofs_ents, dofs_nodes);
2973 "ANALITICAL_METERIAL_TRACTION",
"MESH_NODE_POSITIONS");
2975 "ANALITICAL_METERIAL_TRACTION",
"MESH_NODE_POSITIONS");
2977 "ANALITICAL_METERIAL_TRACTION",
"SPATIAL_POSITION");
2979 "ANALITICAL_METERIAL_TRACTION",
"MESH_NODE_POSITIONS");
2983 if (meshset_manager_ptr->
checkMeshset(
"ANALITICAL_TRACTION")) {
2986 &cubit_meshset_ptr);
2990 tris = intersect(tris, lower_dim_ents);
2992 tris, MBTRI,
"ANALITICAL_METERIAL_TRACTION");
2997 #endif //__ANALITICAL_TRACTION__
◆ declareMaterialFE()
declare material finite elements
Definition at line 2872 of file CrackPropagation.cpp.
2880 bit, mask, MBTET, tets_level);
2888 "SPATIAL_POSITION");
2890 "SPATIAL_POSITION");
2892 "MESH_NODE_POSITIONS");
2894 "MESH_NODE_POSITIONS");
2896 "SPATIAL_POSITION");
2898 "MESH_NODE_POSITIONS");
2901 Range current_ents_with_fe;
2903 current_ents_with_fe);
2904 Range ents_to_remove;
2905 ents_to_remove = subtract(current_ents_with_fe, tets_level);
◆ declarePressureAleFE()
Declare FE for pressure BC in ALE formulation (in material domain)
- Parameters
-
bit | material domain bit ref level |
mask | bit ref level mask for the problem |
- Returns
- error code
Definition at line 2601 of file CrackPropagation.cpp.
2610 bit, mask, MBTET, tets_level);
2616 dofs_ents.merge(tets_level);
2617 for (
int dd = 1;
dd != 3;
dd++) {
2618 CHKERR moab.get_adjacencies(tets_level,
dd,
false, dofs_ents,
2619 moab::Interface::UNION);
2622 rval = moab.get_connectivity(tets_level, dofs_nodes,
true);
2624 Range lower_dim_ents = unite(dofs_ents, dofs_nodes);
2628 "SPATIAL_POSITION");
2630 "SPATIAL_POSITION");
2632 "SPATIAL_POSITION");
2634 "MESH_NODE_POSITIONS");
2636 "MESH_NODE_POSITIONS");
2638 "MESH_NODE_POSITIONS");
2646 tris = intersect(lower_dim_ents, tris);
2647 ents_to_add.merge(tris);
2650 Range current_ents_with_fe;
2652 current_ents_with_fe);
2653 Range ents_to_remove;
2654 ents_to_remove = subtract(current_ents_with_fe, ents_to_add);
◆ declareSimpleContactAleFE()
Declare FE for pressure BC in ALE formulation (in material domain)
- Parameters
-
bit | material domain bit ref level |
mask | bit ref level mask for the problem |
- Returns
- error code
Definition at line 2799 of file CrackPropagation.cpp.
2807 bit, mask, MBPRISM, prisms_level);
2810 prisms_level = intersect(
bitProcEnts, prisms_level);
2816 "SIMPLE_CONTACT_ALE",
"SPATIAL_POSITION",
"LAMBDA_CONTACT",
2818 "EIGEN_SPATIAL_POSITIONS");
2822 Range face_on_prism;
2823 CHKERR moab.get_adjacencies(contact_prisms, 2,
false, face_on_prism,
2824 moab::Interface::UNION);
2826 Range tris_on_prism = face_on_prism.subset_by_type(MBTRI);
2831 Range adj_vols_to_prisms;
2832 CHKERR moab.get_adjacencies(check_tris, 3,
false, adj_vols_to_prisms,
2833 moab::Interface::UNION);
2837 bit, mask, MBTET, tet_level);
2839 Range contact_tets_from_vols = adj_vols_to_prisms.subset_by_type(MBTET);
2841 Range contact_tets = intersect(tet_level, contact_tets_from_vols);
2845 "SPATIAL_POSITION");
2847 "SPATIAL_POSITION");
2849 "MESH_NODE_POSITIONS");
2851 "MESH_NODE_POSITIONS");
2853 "SPATIAL_POSITION");
2855 "MESH_NODE_POSITIONS");
2857 Range current_ale_tets;
2860 Range ale_tets_to_remove;
2861 ale_tets_to_remove = subtract(current_ale_tets, contact_tets);
2863 ale_tets_to_remove);
◆ declareSmoothingFE()
declare mesh smoothing finite elements
Definition at line 3069 of file CrackPropagation.cpp.
3076 bit, mask, MBTET, tets_level);
3084 "MESH_NODE_POSITIONS");
3086 "MESH_NODE_POSITIONS");
3088 "MESH_NODE_POSITIONS");
3090 "SPATIAL_POSITION");
3092 "SMOOTHING",
"LAMBDA_CRACKFRONT_AREA_TANGENT");
3094 "SMOOTHING",
"LAMBDA_CRACKFRONT_AREA_TANGENT");
3097 Range ents_to_remove;
3100 ents_to_remove = subtract(ents_to_remove, tets_level);
◆ declareSpringsAleFE()
Declare FE for spring BC in ALE formulation (in material domain)
- Parameters
-
bit | material domain bit ref level |
mask | bit ref level mask for the problem |
- Returns
- error code
Definition at line 2736 of file CrackPropagation.cpp.
2745 bit, mask, MBTET, tets_level);
2751 dofs_ents.merge(tets_level);
2752 for (
int dd = 1;
dd != 3;
dd++) {
2753 CHKERR moab.get_adjacencies(tets_level,
dd,
false, dofs_ents,
2754 moab::Interface::UNION);
2757 rval = moab.get_connectivity(tets_level, dofs_nodes,
true);
2759 Range lower_dim_ents = unite(dofs_ents, dofs_nodes);
2763 "SPATIAL_POSITION");
2765 "SPATIAL_POSITION");
2767 "SPATIAL_POSITION");
2769 "MESH_NODE_POSITIONS");
2771 "MESH_NODE_POSITIONS");
2773 "MESH_NODE_POSITIONS");
2777 if (
bit->getName().compare(0, 9,
"SPRING_BC") == 0) {
2781 tris = intersect(lower_dim_ents, tris);
2782 ents_to_add.merge(tris);
2786 Range current_ents_with_fe;
2788 current_ents_with_fe);
2789 Range ents_to_remove;
2790 ents_to_remove = subtract(current_ents_with_fe, ents_to_add);
◆ declareSurfaceFE()
MoFEMErrorCode FractureMechanics::CrackPropagation::declareSurfaceFE |
( |
std::string |
fe_name, |
|
|
const BitRefLevel |
bit, |
|
|
const BitRefLevel |
mask, |
|
|
const std::vector< int > & |
ids, |
|
|
const bool |
proc_only = true , |
|
|
const int |
verb = QUIET , |
|
|
const bool |
debug = false |
|
) |
| |
declare surface sliding elements
Definition at line 3110 of file CrackPropagation.cpp.
3130 moab::Interface::UNION);
3131 surface_ents = intersect(surface_ents, proc_tris);
3136 "MESH_NODE_POSITIONS");
3138 "MESH_NODE_POSITIONS");
3140 "MESH_NODE_POSITIONS");
3142 for (std::vector<int>::const_iterator it = ids.begin(); it != ids.end();
3145 "LAMBDA_SURFACE" + boost::lexical_cast<std::string>(*it);
3150 CHKERR moab.get_adjacencies(&ent, 1, 2,
false, adj_tris);
3151 adj_tris = intersect(adj_tris,
bodySkin);
3152 surface_ents.merge(adj_tris);
3164 Range ents_to_remove;
3167 ents_to_remove = subtract(ents_to_remove, surface_ents);
◆ declareSurfaceForceAleFE()
Declare FE for pressure BC in ALE formulation (in material domain)
- Parameters
-
bit | material domain bit ref level |
mask | bit ref level mask for the problem |
- Returns
- error code
Definition at line 2663 of file CrackPropagation.cpp.
2671 bit, mask, MBTET, tets_level);
2677 dofs_ents.merge(tets_level);
2678 for (
int dd = 1;
dd != 3;
dd++) {
2679 CHKERR moab.get_adjacencies(tets_level,
dd,
false, dofs_ents,
2680 moab::Interface::UNION);
2683 rval = moab.get_connectivity(tets_level, dofs_nodes,
true);
2685 Range lower_dim_ents = unite(dofs_ents, dofs_nodes);
2689 "SPATIAL_POSITION");
2691 "SPATIAL_POSITION");
2693 "SPATIAL_POSITION");
2695 "MESH_NODE_POSITIONS");
2697 "MESH_NODE_POSITIONS");
2699 "MESH_NODE_POSITIONS");
2707 tris = intersect(lower_dim_ents, tris);
2708 ents_to_add.merge(tris);
2711 const string block_set_force_name(
"FORCE");
2713 if (it->getName().compare(0, block_set_force_name.length(),
2714 block_set_force_name) == 0) {
2718 tris = intersect(lower_dim_ents, tris);
2719 ents_to_add.merge(tris);
2723 Range current_ents_with_fe;
2725 current_ents_with_fe);
2726 Range ents_to_remove;
2727 ents_to_remove = subtract(current_ents_with_fe, ents_to_add);
◆ deleteEntities()
MoFEMErrorCode FractureMechanics::CrackPropagation::deleteEntities |
( |
const int |
verb = QUIET , |
|
|
const bool |
debug = false |
|
) |
| |
Definition at line 9615 of file CrackPropagation.cpp.
9634 all_ents = subtract(all_ents, all_ents.subset_by_type(MBENTITYSET));
9635 bit_ents = intersect(bit_ents, all_ents);
9636 all_ents = subtract(all_ents, bit_ents);
9639 for (Range::iterator eit = all_ents.begin(); eit != all_ents.end(); eit++) {
9640 for (RefEntity_multiIndex::iterator eiit =
9641 refined_ents_ptr->get<
Ent_mi_tag>().lower_bound(*eit);
9642 eiit != refined_ents_ptr->get<
Ent_mi_tag>().upper_bound(*eit);
9644 cerr << **eiit <<
" " << eiit->get()->getBitRefLevel() << endl;
9651 "", &out_meshset, 1);
◆ getAnalyticalDirichletBc()
◆ getArcCtx()
boost::shared_ptr<ArcLengthCtx>& FractureMechanics::CrackPropagation::getArcCtx |
( |
| ) |
|
|
inline |
◆ getArcLengthDof()
MoFEMErrorCode FractureMechanics::CrackPropagation::getArcLengthDof |
( |
| ) |
|
set pointer to arc-length DOF
Definition at line 9662 of file CrackPropagation.cpp.
9666 dofs_ptr->get<
Unique_mi_tag>().lower_bound(FieldEntity::getLoBitNumberUId(
9669 dofs_ptr->get<
Unique_mi_tag>().upper_bound(FieldEntity::getHiBitNumberUId(
9672 std::distance(dit, hi_dit) != 1)
9674 "Arc length lambda field not defined, or not unique");
◆ getEigenArcCtx()
boost::shared_ptr<ArcLengthCtx>& FractureMechanics::CrackPropagation::getEigenArcCtx |
( |
| ) |
|
|
inline |
◆ getFrontArcLengthControl()
boost::shared_ptr<FEMethod> FractureMechanics::CrackPropagation::getFrontArcLengthControl |
( |
boost::shared_ptr< ArcLengthCtx > |
arc_ctx | ) |
|
|
inline |
Definition at line 913 of file CrackPropagation.hpp.
914 boost::shared_ptr<FEMethod> arc_method(
915 new GriffithForceElement::FrontArcLengthControl(
◆ getInterfaceVersion()
MoFEMErrorCode FractureMechanics::CrackPropagation::getInterfaceVersion |
( |
Version & |
version | ) |
const |
|
inline |
Definition at line 88 of file CrackPropagation.hpp.
90 version = Version(FM_VERSION_MAJOR, FM_VERSION_MINOR, FM_VERSION_BUILD);
◆ getLoadScale() [1/2]
double& FractureMechanics::CrackPropagation::getLoadScale |
( |
| ) |
|
|
inline |
◆ getLoadScale() [2/2]
double FractureMechanics::CrackPropagation::getLoadScale |
( |
| ) |
const |
|
inline |
◆ getMWLSApprox()
boost::shared_ptr<MWLSApprox>& FractureMechanics::CrackPropagation::getMWLSApprox |
( |
| ) |
|
|
inline |
◆ getNbCutSteps() [1/2]
int& FractureMechanics::CrackPropagation::getNbCutSteps |
( |
| ) |
|
|
inline |
◆ getNbCutSteps() [2/2]
int FractureMechanics::CrackPropagation::getNbCutSteps |
( |
| ) |
const |
|
inline |
◆ getNbLoadSteps() [1/2]
int& FractureMechanics::CrackPropagation::getNbLoadSteps |
( |
| ) |
|
|
inline |
◆ getNbLoadSteps() [2/2]
int FractureMechanics::CrackPropagation::getNbLoadSteps |
( |
| ) |
const |
|
inline |
◆ getOptions()
Get options form command line.
- Returns
- error code
Definition at line 588 of file CrackPropagation.cpp.
593 CHKERR PetscOptionsBool(
"-my_propagate_crack",
598 CHKERR PetscOptionsInt(
"-my_geom_order",
"approximation geometry order",
"",
600 CHKERR PetscOptionsScalar(
"-my_gc",
"release energy",
"",
gC, &
gC,
602 CHKERR PetscOptionsScalar(
"-beta_gc",
"heterogeneous gc beta coefficient",
604 CHKERR PetscOptionsBool(
"-my_is_partitioned",
"true if mesh is partitioned",
606 CHKERR PetscOptionsInt(
"-my_ref",
"crack tip mesh refinement level",
"",
608 CHKERR PetscOptionsInt(
"-my_ref_order",
609 "crack tip refinement approximation order level",
"",
612 MOFEM_LOG_C(
"CPWorld", Sev::inform,
"### Input parameter: -my_order %d",
616 MOFEM_LOG_C(
"CPWorld", Sev::inform,
"### Input parameter: -my_gc %6.4e",
620 MOFEM_LOG_C(
"CPWorld", Sev::inform,
"### Input parameter: -my_ref %d",
622 MOFEM_LOG_C(
"CPWorld", Sev::inform,
"### Input parameter: -my_ref_order %d",
628 "if false force use of nonlinear element for hooke material",
"",
632 CHKERR PetscOptionsBool(
"-my_add_pressure_ale",
633 "if set surface pressure is considered in ALE",
"",
637 CHKERR PetscOptionsBool(
"-my_add_springs_ale",
638 "if set surface springs is considered in ALE",
"",
642 CHKERR PetscOptionsBool(
"-my_add_surface_force_ale",
643 "if set surface force is considered in ALE",
"",
648 "-my_ignore_material_surface_force",
649 "if set material forces arising from surface force are ignored",
"",
653 CHKERR PetscOptionsBool(
"-my_add_singularity",
654 "if set singularity added to crack front",
"",
660 char file_name[255] =
"mwls.med";
661 CHKERR PetscOptionsString(
662 "-my_mwls_approx_file",
663 "file with data from med file (code-aster) with radiation data",
"",
664 file_name, file_name, 255, &flg);
665 if (flg == PETSC_TRUE) {
668 "### Input parameter: -my_mwls_approx_file %s", file_name);
670 char tag_name[255] =
"SIGP";
671 CHKERR PetscOptionsString(
"-my_internal_stress_name",
672 "name of internal stress tag",
"", tag_name,
673 tag_name, 255, &flg);
675 "### Input parameter: -my_internal_stress_name %s", tag_name);
677 CHKERR PetscOptionsString(
"-my_eigen_stress_name",
678 "name of eigen stress tag",
"", tag_name,
679 tag_name, 255, &flg);
681 "### Input parameter: -my_eigen_stress_name %s", tag_name);
685 "-my_residual_stress_block",
686 "block in mechanical (i.e. cracked) mesh to which residual stress "
687 "and density mapping are applied",
690 "### Input parameter: -my_residual_stress_block %d",
693 CHKERR PetscOptionsInt(
"-my_density_block",
694 "block to which density is mapped",
"",
699 char density_tag_name[255] =
"RHO";
700 CHKERR PetscOptionsString(
"-my_density_tag_name",
701 "name of density tag (RHO)",
"", density_tag_name,
702 density_tag_name, 255, PETSC_NULL);
704 "### Input parameter: -my_density_tag_name %s",
710 char file_name[255] =
"add_cubit_meshsets.in";
711 CHKERR PetscOptionsString(
"-meshsets_config",
712 "meshsets config file name",
"", file_name,
713 file_name, 255, &flg);
714 if (flg == PETSC_TRUE) {
715 ifstream
f(file_name);
718 "File configuring meshsets ( %s ) cannot be open\n",
730 MOFEM_LOG_C(
"CPWorld", Sev::inform,
"### Input parameter: -material %s",
735 CHKERR PetscOptionsScalar(
"-my_rho0",
"release energy",
"",
rHo0, &
rHo0,
737 MOFEM_LOG_C(
"CPWorld", Sev::inform,
"### Input parameter: -my_rho0 %6.4e",
740 CHKERR PetscOptionsScalar(
"-my_n_bone",
"release energy",
"",
nBone,
743 "### Input parameter: -my_n_bone %6.4e",
nBone);
747 CHKERR PetscOptionsInt(
"-nb_load_steps",
"number of load steps",
"",
750 CHKERR PetscOptionsInt(
"-nb_cut_steps",
"number of cut mesh steps",
"",
756 "### Input parameter: -load_scale %6.4e",
loadScale);
761 "-other_side_constrain",
762 "Set surface constrain on other side of crack surface",
"",
769 CHKERR PetscOptionsReal(
"-smoother_alpha",
"Control mesh smoother",
"",
771 CHKERR PetscOptionsReal(
"-my_gamma",
"Controls mesh smoother",
"",
775 "### Input parameter: -smoother_alpha %6.4e",
smootherAlpha);
782 CHKERR PetscOptionsReal(
"-arc_alpha",
"Arc length alpha",
"",
arcAlpha,
784 CHKERR PetscOptionsReal(
"-arc_beta",
"Arc length beta",
"",
arcBeta,
786 CHKERR PetscOptionsReal(
"-arc_s",
"Arc length step size",
"",
arcS, &
arcS,
789 "### Input parameter: -arc_alpha %6.4e",
arcAlpha);
791 "### Input parameter: -arc_beta %6.4e",
arcBeta);
792 MOFEM_LOG_C(
"CPWorld", Sev::inform,
"### Input parameter: -arc_s %6.4e",
805 CHKERR PetscOptionsBool(
"-my_ignore_contact",
"If true ignore contact",
807 CHKERR PetscOptionsBool(
"-my_fix_contact_nodes",
810 CHKERR PetscOptionsBool(
"-my_print_contact_state",
811 "If true print contact state",
"",
814 "-my_contact_output_integ_pts",
815 "If true output data at contact integration points",
"",
817 CHKERR PetscOptionsReal(
"-my_r_value",
"Contact regularisation parameter",
819 CHKERR PetscOptionsReal(
"-my_cn_value",
"Contact augmentation parameter",
821 CHKERR PetscOptionsInt(
"-my_contact_order",
"contact approximation order",
824 "-my_contact_lambda_order",
"contact Lagrange multipliers order",
"",
827 "### Input parameter: -my_cn_value %6.4e",
cnValue);
829 "### Input parameter: -my_contact_order %d",
contactOrder);
831 "### Input parameter: -my_cn_value %6.4e",
cnValue);
833 "### Input parameter: -my_contact_order %d",
contactOrder);
839 CHKERR PetscOptionsBool(
"-cut_mesh",
"If true mesh is cut by surface",
"",
842 CHKERR PetscOptionsReal(
"-cut_factor",
"Crack acceleration factor",
"",
847 "-run_elastic_without_crack",
"If true mesh is cut by surface",
"",
850 MOFEM_LOG_C(
"CPWorld", Sev::inform,
"### Input parameter: -cut_mesh %d",
853 "### Input parameter: -cut_factor %6.4e",
856 "### Input parameter: -run_elastic_without_crack %d",
859 CHKERR PetscOptionsInt(
"-post_proc_level",
"level of output files",
"",
862 "### Input parameter: -post_proc_level %6.4e",
postProcLevel);
866 "-add_analytical_internal_stress_operators",
867 "If true add analytical internal stress operators for mwls test",
"",
872 CHKERR PetscOptionsBool(
"-solve_eigen_problem",
"If true solve eigen problem",
877 "-use_eigen_pos_simple_contact",
878 "If true use eigen positions for matching meshes contact",
"",
882 "### Input parameter: -solve_eigen_problem %d",
885 "### Input parameter: -use_eigen_pos_simple_contact %d",
890 CHKERR PetscOptionsScalar(
"-part_weight_power",
"Partitioning weight power",
896 "-calc_mwls_coeffs_every_propagation_step",
897 "If true recaulculate MWLS coefficients every propagation step",
"",
901 ierr = PetscOptionsEnd();
909 char mumps_options[] =
910 "-mat_mumps_icntl_14 800 -mat_mumps_icntl_24 1 -mat_mumps_icntl_13 1 "
911 "-propagation_fieldsplit_0_mat_mumps_icntl_14 800 "
912 "-propagation_fieldsplit_0_mat_mumps_icntl_24 1 "
913 "-propagation_fieldsplit_0_mat_mumps_icntl_13 1 "
914 "-propagation_fieldsplit_1_mat_mumps_icntl_14 800 "
915 "-propagation_fieldsplit_1_mat_mumps_icntl_24 1 "
916 "-propagation_fieldsplit_1_mat_mumps_icntl_13 1 "
917 "-mat_mumps_icntl_20 0 "
918 "-propagation_fieldsplit_0_mat_mumps_icntl_20 0 "
919 "-propagation_fieldsplit_1_mat_mumps_icntl_20 0";
920 CHKERR PetscOptionsInsertString(NULL, mumps_options);
923 CHKERR getInterface<CPMeshCut>()->getOptions();
924 CHKERR getInterface<CPSolvers>()->getOptions();
◆ partitionMesh()
partotion mesh
Definition at line 1112 of file CrackPropagation.cpp.
1121 map<int, Range> layers;
1124 CHKERR moab.get_adjacencies(ref_nodes, 3,
false, tets,
1125 moab::Interface::UNION);
1131 CHKERR moab.get_connectivity(tets, ref_nodes,
false);
1132 CHKERR moab.get_adjacencies(ref_nodes, 3,
false, tets,
1133 moab::Interface::UNION);
1137 layers[ll + 1] = subtract(tets, layers[ll]);
1142 int last = layers.size();
1143 auto rest_elements_in_the_bubble = subtract(tets_bit_2, layers[last - 1]);
1144 if (rest_elements_in_the_bubble.size())
1145 layers[last] = rest_elements_in_the_bubble;
1149 rval = moab.tag_get_handle(
"TETS_WEIGHT", th_tet_weight);
1150 if (
rval == MB_SUCCESS) {
1151 CHKERR moab.tag_delete(th_tet_weight);
1154 CHKERR moab.tag_get_handle(
"TETS_WEIGHT", 1, MB_TYPE_INTEGER, th_tet_weight,
1155 MB_TAG_CREAT | MB_TAG_SPARSE, &def_val);
1157 for (
unsigned int ll = 0; ll != layers.size(); ll++) {
1159 CHKERR moab.tag_clear_data(th_tet_weight, layers[ll], &weight);
1172 CHKERR moab.tag_delete(th_tet_weight);
◆ postProcessDM()
MoFEMErrorCode FractureMechanics::CrackPropagation::postProcessDM |
( |
DM |
dm, |
|
|
const int |
step, |
|
|
const std::string |
fe_name, |
|
|
const bool |
approx_internal_stress |
|
) |
| |
post-process results for elastic solution
Definition at line 9010 of file CrackPropagation.cpp.
9016 "Pointer to elasticFe is NULL");
9019 auto post_proc = boost::make_shared<
9028 post_proc->meshPositionsFieldName =
"NONE";
9030 CHKERR post_proc->generateReferenceElementMesh();
9031 CHKERR post_proc->addFieldValuesPostProc(
"MESH_NODE_POSITIONS");
9032 CHKERR post_proc->addFieldValuesGradientPostProc(
"MESH_NODE_POSITIONS");
9035 boost::shared_ptr<MatrixDouble> mat_pos_at_pts_ptr(
new MatrixDouble());
9037 "MESH_NODE_POSITIONS", mat_pos_at_pts_ptr));
9038 boost::shared_ptr<MatrixDouble> mat_grad_pos_at_pts_ptr;
9041 post_proc->getOpPtrVector().push_back(
9043 post_proc->getOpPtrVector().push_back(
new OpPostProcDisplacements(
9044 post_proc->singularElement, post_proc->singularDisp,
9045 post_proc->postProcMesh, post_proc->mapGaussPts,
H));
9046 post_proc->getOpPtrVector().push_back(
9048 "MESH_NODE_POSITIONS",
elasticFe->commonData));
9049 post_proc->getOpPtrVector().push_back(
new OpTransfromSingularBaseFunctions(
9050 post_proc->singularElement, post_proc->detS, post_proc->invSJac));
9051 CHKERR post_proc->addFieldValuesPostProc(
"SPATIAL_POSITION");
9052 CHKERR post_proc->addFieldValuesGradientPostProc(
"SPATIAL_POSITION");
9053 std::map<int, NonlinearElasticElement::BlockData>::iterator sit =
9055 for (; sit !=
elasticFe->setOfBlocks.end(); sit++) {
9057 post_proc->postProcMesh, post_proc->mapGaussPts,
"SPATIAL_POSITION",
9058 sit->second, post_proc->commonData,
9064 boost::shared_ptr<HookeElement::DataAtIntegrationPts>
9065 data_hooke_element_at_pts(
new HookeElement::DataAtIntegrationPts());
9068 CHKERR post_proc_skin.generateReferenceElementMesh();
9070 CHKERR post_proc_skin.addFieldValuesPostProc(
"MESH_NODE_POSITIONS");
9072 CHKERR post_proc_skin.addFieldValuesPostProc(
"EIGEN_SPATIAL_POSITIONS");
9079 struct OpGetFieldGradientValuesOnSkinWithSingular
9082 const std::string feVolName;
9083 boost::shared_ptr<VolSideFe> sideOpFe;
9085 OpGetFieldGradientValuesOnSkinWithSingular(
9086 const std::string
field_name,
const std::string vol_fe_name,
9087 boost::shared_ptr<VolSideFe> side_fe)
9090 feVolName(vol_fe_name), sideOpFe(side_fe) {}
9095 if (
type != MBVERTEX)
9097 CHKERR loopSideVolumes(feVolName, *sideOpFe);
9102 boost::shared_ptr<VolSideFe> my_vol_side_fe_ptr =
9107 my_vol_side_fe_ptr->getOpPtrVector().push_back(
9109 "MESH_NODE_POSITIONS", data_hooke_element_at_pts->HMat));
9110 my_vol_side_fe_ptr->getOpPtrVector().push_back(
new OpPostProcDisplacements(
9111 my_vol_side_fe_ptr->singularElement, my_vol_side_fe_ptr->singularDisp,
9112 post_proc_skin.postProcMesh, post_proc_skin.mapGaussPts,
9113 data_hooke_element_at_pts->HMat));
9115 my_vol_side_fe_ptr->getOpPtrVector().push_back(
9116 new OpTransfromSingularBaseFunctions(my_vol_side_fe_ptr->singularElement,
9117 my_vol_side_fe_ptr->detS,
9118 my_vol_side_fe_ptr->invSJac));
9120 my_vol_side_fe_ptr->getOpPtrVector().push_back(
9122 "SPATIAL_POSITION", data_hooke_element_at_pts->hMat));
9124 post_proc_skin.getOpPtrVector().push_back(
9125 new OpGetFieldGradientValuesOnSkinWithSingular(
9126 "MESH_NODE_POSITIONS", fe_name.c_str(), my_vol_side_fe_ptr));
9128 CHKERR post_proc_skin.addFieldValuesPostProc(
"SPATIAL_POSITION");
9129 CHKERR post_proc_skin.addFieldValuesPostProc(
"MESH_NODE_POSITIONS");
9131 post_proc_skin.getOpPtrVector().push_back(
9132 new HookeElement::OpPostProcHookeElement<FaceElementForcesAndSourcesCore>(
9133 "MESH_NODE_POSITIONS", data_hooke_element_at_pts,
9134 elasticFe->setOfBlocks, post_proc_skin.postProcMesh,
9135 post_proc_skin.mapGaussPts,
9140 post_proc_skin.getOpPtrVector().push_back(
new OpSetTagRangeOnSkin(
9141 post_proc_skin.postProcMesh, post_proc_skin.mapGaussPts,
9143 post_proc_skin.getOpPtrVector().push_back(
new OpSetTagRangeOnSkin(
9144 post_proc_skin.postProcMesh, post_proc_skin.mapGaussPts,
9153 post_proc->getOpPtrVector().push_back(
9154 new MWLSApprox::OpMWLSStressAtGaussPts(
9158 post_proc->getOpPtrVector().push_back(
9161 post_proc->getOpPtrVector().push_back(
9162 new MWLSApprox::OpMWLSStressAtGaussUsingPrecalulatedCoeffs(
9167 boost::shared_ptr<moab::Interface> post_proc_mesh_ptr(
9169 boost::shared_ptr<std::vector<EntityHandle>> map_gauss_pts_ptr(
9171 post_proc->getOpPtrVector().push_back(
9172 new MWLSApprox::OpMWLSStressPostProcess(
9173 post_proc_mesh_ptr, map_gauss_pts_ptr,
mwlsApprox));
9177 "mwlsApprox not allocated");
9182 approx_internal_stress) {
9186 post_proc->getOpPtrVector().push_back(
9187 new MWLSApprox::OpMWLSRhoAtGaussPts(mat_pos_at_pts_ptr,
H,
9191 post_proc->getOpPtrVector().push_back(
9194 post_proc->getOpPtrVector().push_back(
9195 new MWLSApprox::OpMWLSRhoAtGaussUsingPrecalulatedCoeffs(
9200 boost::shared_ptr<moab::Interface> post_proc_mesh_ptr(
9202 boost::shared_ptr<std::vector<EntityHandle>> map_gauss_pts_ptr(
9204 post_proc->getOpPtrVector().push_back(
9205 new MWLSApprox::OpMWLSRhoPostProcess(
9206 post_proc_mesh_ptr, map_gauss_pts_ptr,
mwlsApprox,
9208 post_proc->commonData)));
9211 "mwlsApprox not allocated");
9218 ss <<
"out_skin_" << step <<
".h5m";
9220 CHKERR post_proc_skin.writeFile(ss.str());
9225 ss <<
"out_spatial_" << step <<
".h5m";
9231 CHKERR post_proc->postProcMesh.tag_get_handle(
"SPATIAL_POSITION_GRAD",
9233 CHKERR post_proc->postProcMesh.tag_delete(
th);
9235 CHKERR post_proc->writeFile(ss.str());
9239 auto fe_post_proc_face_contact =
9240 boost::make_shared<PostProcFaceOnRefinedMesh>(
mField);
9241 CHKERR fe_post_proc_face_contact->generateReferenceElementMesh();
9243 CHKERR fe_post_proc_face_contact->addFieldValuesPostProc(
"LAMBDA_CONTACT");
9244 CHKERR fe_post_proc_face_contact->addFieldValuesPostProc(
9245 "SPATIAL_POSITION");
9246 CHKERR fe_post_proc_face_contact->addFieldValuesPostProc(
9247 "MESH_NODE_POSITIONS");
9250 fe_post_proc_face_contact);
9253 std::ostringstream stm;
9254 stm <<
"out_contact_surface_" << step <<
".h5m";
9256 CHKERR PetscPrintf(PETSC_COMM_WORLD,
"out file %s\n",
9258 CHKERR fe_post_proc_face_contact->postProcMesh.write_file(
9271 std::ostringstream ostrm;
9272 ostrm <<
"out_contact_integ_pts_" << step <<
".h5m";
9274 CHKERR PetscPrintf(PETSC_COMM_WORLD,
"out file %s\n",
9277 "PARALLEL=WRITE_PART");
◆ projectGriffithForce()
MoFEMErrorCode FractureMechanics::CrackPropagation::projectGriffithForce |
( |
DM |
dm, |
|
|
Vec |
f_griffith, |
|
|
Vec |
f_griffith_proj, |
|
|
const int |
verb = QUIET , |
|
|
const bool |
debug = false |
|
) |
| |
project Griffith forces
Definition at line 8011 of file CrackPropagation.cpp.
8024 double nrm2_griffith_force;
8025 CHKERR VecNorm(f_griffith, NORM_2, &nrm2_griffith_force);
8027 nrm2_griffith_force);
8031 CHKERR VecGetSize(f_griffith, &
M);
8032 CHKERR VecGetLocalSize(f_griffith, &
m);
8036 CHKERR MatShellSetOperation(Q, MATOP_MULT,
8038 CHKERR MatMult(Q, f_griffith, f_griffith_proj);
8041 CHKERR VecCopy(f_griffith, f_griffith_proj);
8047 CHKERR VecGhostUpdateBegin(f_griffith_proj, INSERT_VALUES, SCATTER_FORWARD);
8048 CHKERR VecGhostUpdateEnd(f_griffith_proj, INSERT_VALUES, SCATTER_FORWARD);
8049 PostProcVertexMethod ent_method_prj_griffith_force(
8050 mField, f_griffith_proj,
"GRIFFITH_FORCE_PROJECTED");
8052 ent_method_prj_griffith_force);
8054 double nrm2_griffith_force;
8055 CHKERR VecNorm(f_griffith_proj, NORM_2, &nrm2_griffith_force);
8057 nrm2_griffith_force);
◆ projectMaterialForcesDM()
MoFEMErrorCode FractureMechanics::CrackPropagation::projectMaterialForcesDM |
( |
DM |
dm_project, |
|
|
Vec |
f, |
|
|
Vec |
f_proj, |
|
|
const int |
verb = QUIET , |
|
|
const bool |
debug = false |
|
) |
| |
project material forces along the crack elongation direction
- Parameters
-
dm_project | material forces DM, sub-dm of dm_crack_propagation |
f_proj | Rhs sub-vector of projected material forces for material forces DM |
f | Rhs sub-vector for material forces DM |
verb | compilation parameter determining the amount of information printed |
debug | flag for debugging |
- Returns
- error code
Definition at line 7908 of file CrackPropagation.cpp.
7917 CHKERR VecView(
f, PETSC_VIEWER_STDOUT_WORLD);
7927 CHKERR VecView(x, PETSC_VIEWER_STDOUT_WORLD);
7932 CHKERR VecView(Cf, PETSC_VIEWER_STDOUT_WORLD);
7944 CHKERR MatShellSetOperation(Q, MATOP_MULT,
7951 PostProcVertexMethod ent_method_spatial_position(
mField, f_proj,
7952 "MATERIAL_FORCE_PROJECTED");
7953 CHKERR VecGhostUpdateBegin(f_proj, INSERT_VALUES, SCATTER_FORWARD);
7954 CHKERR VecGhostUpdateEnd(f_proj, INSERT_VALUES, SCATTER_FORWARD);
7956 ent_method_spatial_position, 0,
◆ query_interface()
Getting interface of core database.
- Parameters
-
- Returns
- error code
Definition at line 498 of file CrackPropagation.cpp.
503 if (type_index == boost::typeindex::type_id<CrackPropagation>()) {
508 if (type_index == boost::typeindex::type_id<CPSolvers>()) {
513 if (type_index == boost::typeindex::type_id<CPMeshCut>()) {
◆ readMedFile()
MoFEMErrorCode FractureMechanics::CrackPropagation::readMedFile |
( |
| ) |
|
◆ resolveShared()
resolve shared entities
Definition at line 1177 of file CrackPropagation.cpp.
1184 ParallelComm *pcomm = ParallelComm::get_pcomm(&moab,
MYPCOMM_INDEX);
1187 Range all_proc_ents;
1190 Tag part_tag = pcomm->part_tag();
1193 0, MBENTITYSET, &part_tag, NULL, 1, tagged_sets, moab::Interface::UNION);
1194 for (Range::iterator mit = tagged_sets.begin(); mit != tagged_sets.end();
1197 CHKERR moab.tag_get_data(part_tag, &*mit, 1, &part);
1199 CHKERR moab.get_entities_by_dimension(*mit, 3, proc_ents,
true);
1200 CHKERR moab.get_entities_by_handle(*mit, all_proc_ents,
true);
1203 proc_ents = intersect(proc_ents, tets);
1207 CHKERR skin.find_skin(0, tets,
false, tets_skin);
1210 Range proc_ents_skin[4];
1217 bit2_again,
BitRefLevel().set(), MBPRISM, prisms_level);
1221 Range contact_prisms_to_remove =
1223 prisms_level = subtract(prisms_level, contact_prisms_to_remove);
1225 Range face_on_prism;
1226 CHKERR moab.get_adjacencies(contact_prisms, 2,
false, face_on_prism,
1227 moab::Interface::UNION);
1229 Range tris_on_prism = face_on_prism.subset_by_type(MBTRI);
1231 Range adj_vols_to_prisms;
1232 CHKERR moab.get_adjacencies(tris_on_prism, 3,
false, adj_vols_to_prisms,
1233 moab::Interface::UNION);
1237 bit2_again,
BitRefLevel().set(), MBTET, tet_level);
1239 Range contact_tets_from_vols = adj_vols_to_prisms.subset_by_type(MBTET);
1241 contact_tets = intersect(tet_level, contact_tets_from_vols);
1244 ParallelComm *pcomm = ParallelComm::get_pcomm(&moab,
MYPCOMM_INDEX);
1245 Range contact_tets_container = contact_tets;
1274 proc_ents.merge(contact_tets);
1276 Range contact_tets_vert;
1277 CHKERR moab.get_adjacencies(contact_tets, 0,
false, contact_tets_vert,
1278 moab::Interface::UNION);
1279 Range contact_tets_edges;
1280 CHKERR moab.get_adjacencies(contact_tets, 1,
false, contact_tets_edges,
1281 moab::Interface::UNION);
1282 Range contact_tets_faces;
1283 CHKERR moab.get_adjacencies(contact_tets, 2,
false, contact_tets_faces,
1284 moab::Interface::UNION);
1285 proc_ents_skin[2].merge(contact_tets_faces);
1286 proc_ents_skin[1].merge(contact_tets_edges);
1287 proc_ents_skin[0].merge(contact_tets_vert);
1290 proc_ents_skin[3] = proc_ents;
1291 CHKERR skin.find_skin(0, proc_ents,
false, proc_ents_skin[2]);
1293 proc_ents_skin[2] = subtract(proc_ents_skin[2], tets_skin);
1301 CHKERR moab.get_adjacencies(proc_ents_skin[2], 1,
false, proc_ents_skin[1],
1302 moab::Interface::UNION);
1303 CHKERR moab.get_connectivity(proc_ents_skin[1], proc_ents_skin[0],
true);
1305 Range crack_faces_nodes;
1307 proc_ents_skin[0].merge(crack_faces_nodes);
1312 Range arc_length_vertices;
1313 CHKERR moab.get_entities_by_type(lambda_meshset, MBVERTEX,
1314 arc_length_vertices,
true);
1315 if (arc_length_vertices.size() != 1) {
1317 "Should be one vertex in <LAMBDA_ARC_LENGTH> field but is %d",
1318 arc_length_vertices.size());
1321 proc_ents_skin[0].merge(arc_length_vertices);
1323 const double coords[] = {0, 0, 0};
1335 Range all_ents_no_on_part;
1336 CHKERR moab.get_entities_by_handle(0, all_ents_no_on_part,
true);
1337 all_ents_no_on_part = subtract(all_ents_no_on_part, all_proc_ents);
1338 for (
int dd = 0;
dd != 4; ++
dd) {
1339 all_ents_no_on_part = subtract(all_ents_no_on_part, proc_ents_skin[
dd]);
1341 all_ents_no_on_part = subtract(
1342 all_ents_no_on_part, all_ents_no_on_part.subset_by_type(MBENTITYSET));
1348 CHKERR moab.get_entities_by_handle(0, all_ents);
1349 std::vector<unsigned char> pstat_tag(all_ents.size(), 0);
1350 CHKERR moab.tag_set_data(pcomm->pstatus_tag(), all_ents,
1351 &*pstat_tag.begin());
1354 CHKERR pcomm->resolve_shared_ents(0, proc_ents, 3, -1, proc_ents_skin);
1359 std::ostringstream file_skin;
1362 CHKERR moab.create_meshset(MESHSET_SET, meshset_skin);
1363 CHKERR moab.add_entities(meshset_skin, proc_ents_skin[2]);
1364 CHKERR moab.add_entities(meshset_skin, proc_ents_skin[1]);
1365 CHKERR moab.add_entities(meshset_skin, proc_ents_skin[0]);
1367 CHKERR moab.write_file(file_skin.str().c_str(),
"VTK",
"", &meshset_skin,
1369 std::ostringstream file_owned;
1372 CHKERR moab.create_meshset(MESHSET_SET, meshset_owned);
1373 CHKERR moab.add_entities(meshset_owned, proc_ents);
1374 CHKERR moab.write_file(file_owned.str().c_str(),
"VTK",
"", &meshset_owned,
1376 CHKERR moab.delete_entities(&meshset_owned, 1);
1382 CHKERR pcomm->get_shared_entities(-1, shared_ents);
1383 std::ostringstream file_shared_owned;
1387 CHKERR moab.create_meshset(MESHSET_SET, meshset_shared_owned);
1388 CHKERR moab.add_entities(meshset_shared_owned, shared_ents);
1389 CHKERR moab.write_file(file_shared_owned.str().c_str(),
"VTK",
"",
1390 &meshset_shared_owned, 1);
1391 CHKERR moab.delete_entities(&meshset_shared_owned, 1);
◆ resolveSharedBitRefLevel()
resole shared entities by bit level
Definition at line 1397 of file CrackPropagation.cpp.
1401 ParallelComm *pcomm = ParallelComm::get_pcomm(&moab,
MYPCOMM_INDEX);
1409 std::ostringstream file_owned;
1412 CHKERR moab.create_meshset(MESHSET_SET, meshset);
1413 CHKERR moab.add_entities(meshset,
bitEnts.subset_by_dimension(3));
1414 CHKERR moab.write_file(file_owned.str().c_str(),
"VTK",
"", &meshset, 1);
1415 CHKERR moab.delete_entities(&meshset, 1);
1419 Tag part_tag = pcomm->part_tag();
1422 0, MBENTITYSET, &part_tag, NULL, 1, tagged_sets,
1423 moab::Interface::UNION);
1432 tris_level = intersect(tris_level, prisms_sides);
1437 for (Range::iterator mit = tagged_sets.begin(); mit != tagged_sets.end();
1440 CHKERR moab.get_entities_by_type(*mit, MBTRI, part_tris);
1441 part_tris = intersect(part_tris, tris_level);
1443 CHKERR moab.get_adjacencies(part_tris, 3,
false, adj_prisms,
1444 moab::Interface::UNION);
1445 adj_prisms = intersect(adj_prisms, prisms_level);
1446 prisms_level = subtract(prisms_level, adj_prisms);
1449 CHKERR moab.tag_get_data(part_tag, &*mit, 1, &part);
1450 std::vector<int> tag(adj_prisms.size(), part);
1451 CHKERR moab.tag_set_data(part_tag, adj_prisms, &*tag.begin());
1452 CHKERR moab.add_entities(*mit, adj_prisms);
◆ saveEachPart()
MoFEMErrorCode FractureMechanics::CrackPropagation::saveEachPart |
( |
const std::string |
prefix, |
|
|
const Range & |
ents |
|
) |
| |
Save entities on ech processor.
Usually used for debugging, to check if meshes are consistent on all processors.
- Parameters
-
prefix | file name prefix |
ents | entities to save |
- Returns
- MoFEMErrorCode error code
Definition at line 1098 of file CrackPropagation.cpp.
1101 std::ostringstream file;
1107 &meshset_out, 1, NULL, 0);
◆ savePositionsOnCrackFrontDM()
MoFEMErrorCode FractureMechanics::CrackPropagation::savePositionsOnCrackFrontDM |
( |
DM |
dm, |
|
|
Vec |
q, |
|
|
const int |
verb = QUIET , |
|
|
const bool |
debug = false |
|
) |
| |
Definition at line 8064 of file CrackPropagation.cpp.
8068 if (q != PETSC_NULL) {
8069 CHKERR VecGhostUpdateBegin(q, INSERT_VALUES, SCATTER_FORWARD);
8070 CHKERR VecGhostUpdateEnd(q, INSERT_VALUES, SCATTER_FORWARD);
8074 PostProcVertexMethod ent_method_spatial_position(
mField, q,
8075 "SPATIAL_POSITION");
8077 ent_method_spatial_position, 0,
8084 std::ostringstream file_name;
8085 file_name <<
"out_crack_spatial_position.h5m";
8087 "PARALLEL=WRITE_PART", &meshset, 1);
◆ setCoordsFromField()
MoFEMErrorCode FractureMechanics::CrackPropagation::setCoordsFromField |
( |
const std::string |
field_name = "MESH_NODE_POSITIONS" | ) |
|
set coordinates from field
Definition at line 9304 of file CrackPropagation.cpp.
9309 MBVERTEX, dof_ptr)) {
9311 int dof_rank = dof_ptr->get()->getDofCoeffIdx();
9312 double fval = dof_ptr->get()->getFieldData();
9317 coords[dof_rank] = fval;
9318 if (dof_ptr->get()->getName() !=
field_name) {
◆ setCrackFrontBitLevel()
Set bit ref level for entities adjacent to crack front.
- Parameters
-
from_bit | |
bit | |
nb_levels | adjacency bridge levels |
- Returns
- MoFEMErrorCode
Definition at line 9413 of file CrackPropagation.cpp.
9427 for (
int ll = 0; ll !=
nb_levels; ll++) {
9429 CHKERR moab.get_adjacencies(ents, 3,
false, adj_tets,
9430 moab::Interface::UNION);
9432 adj_tets = intersect(bit_tets, adj_tets);
9434 CHKERR moab.get_connectivity(adj_tets, ents,
true);
9437 CHKERR moab.get_adjacencies(adj_tets.subset_by_type(MBTET), 1,
false, ents,
9438 moab::Interface::UNION);
9439 CHKERR moab.get_adjacencies(adj_tets.subset_by_type(MBTET), 2,
false, ents,
9440 moab::Interface::UNION);
9441 ents.merge(adj_tets);
9447 bit,
BitRefLevel().set(), MBTET,
"bit2_at_crack_front.vtk",
"VTK",
"");
9449 bit,
BitRefLevel().set(), MBPRISM,
"bit2_at_crack_front_prisms.vtk",
◆ setFieldFromCoords()
MoFEMErrorCode FractureMechanics::CrackPropagation::setFieldFromCoords |
( |
const std::string |
field_name | ) |
|
set field from node positions
Definition at line 9285 of file CrackPropagation.cpp.
9290 if (dof_ptr->get()->getEntType() != MBVERTEX) {
9291 dof_ptr->get()->getFieldData() = 0;
9293 int dof_rank = dof_ptr->get()->getDofCoeffIdx();
9294 double &fval = dof_ptr->get()->getFieldData();
9297 fval = coords[dof_rank];
◆ setMaterialPositionFromCoords()
MoFEMErrorCode FractureMechanics::CrackPropagation::setMaterialPositionFromCoords |
( |
| ) |
|
◆ setSingularDofs()
MoFEMErrorCode FractureMechanics::CrackPropagation::setSingularDofs |
( |
const string |
field_name, |
|
|
const int |
verb = QUIET |
|
) |
| |
set singular dofs (on edges adjacent to crack front) from geometry
Definition at line 9334 of file CrackPropagation.cpp.
9345 CHKERR moab.get_connectivity(*eit, conn, num_nodes,
false);
9347 CHKERR moab.get_coords(conn, num_nodes, coords);
9348 const double dir[3] = {coords[3] - coords[0], coords[4] - coords[1],
9349 coords[5] - coords[2]};
9350 double dof[3] = {0, 0, 0};
9352 for (
int dd = 0;
dd != 3;
dd++) {
9356 for (
int dd = 0;
dd != 3;
dd++) {
9362 const int idx = dit->get()->getEntDofIdx();
9364 dit->get()->getFieldData() = 0;
9367 cerr << **dit << endl;
9368 cerr << dof[idx] << endl;
9373 dit->get()->getFieldData() = dof[idx];
◆ setSingularElementMatrialPositions()
MoFEMErrorCode FractureMechanics::CrackPropagation::setSingularElementMatrialPositions |
( |
const int |
verb = QUIET | ) |
|
set singular dofs of material field (on edges adjacent to crack front) from geometry
Definition at line 9381 of file CrackPropagation.cpp.
◆ setSpatialPositionFromCoords()
MoFEMErrorCode FractureMechanics::CrackPropagation::setSpatialPositionFromCoords |
( |
| ) |
|
◆ solveElasticDM()
MoFEMErrorCode FractureMechanics::CrackPropagation::solveElasticDM |
( |
DM |
dm, |
|
|
SNES |
snes, |
|
|
Mat |
m, |
|
|
Vec |
q, |
|
|
Vec |
f, |
|
|
bool |
snes_set_up, |
|
|
Mat * |
shell_m |
|
) |
| |
solve elastic problem
- Parameters
-
dm | elastic DM |
snes | snes solver for elastic DM |
m | stiffness sub-matrix for elastic DM |
q | DoF sub-vector for elastic DM |
f | Rhs sub-vector for material DM |
snes_set_up | boolean flag wheather to determin snes solver |
shell_m | stiffness sub-matrix for elastic DM |
- Returns
- error code
Definition at line 8224 of file CrackPropagation.cpp.
8232 auto check_vec_size = [&](
auto q) {
8235 CHKERR VecGetSize(q, &q_size);
8238 "MoFEM vector size incomatibile %d != d", q_size,
8243 CHKERR check_vec_size(q);
8246 decltype(
arcCtx) arc_ctx;
8247 if (problem_ptr->
getName() ==
"ELASTIC")
8249 if (problem_ptr->
getName() ==
"EIGEN_ELASTIC")
8254 "Ctx for arc-length is not created");
8259 dynamic_cast<CrackPropagation::ArcLengthSnesCtx *
>(snes_ctx);
8261 arc_snes_ctx->setVecDiagM(diag_m);
8262 CHKERR VecSet(arc_snes_ctx->getVecDiagM(), 1.);
8265 boost::make_shared<ArcLengthMatShell>(
m, arc_ctx, problem_ptr->
getName());
8267 auto create_shell_matrix = [&]() {
8272 CHKERR MatGetLocalSize(
m, &mm, &nn);
8274 static_cast<void *
>(arc_mat_ctx.get()), shellM);
8275 CHKERR MatShellSetOperation(*shellM, MATOP_MULT,
8281 auto get_pc = [&]() {
8283 CHKERR PCAppendOptionsPrefix(pc,
"elastic_");
8284 CHKERR PCSetFromOptions(pc);
8288 boost::shared_ptr<PCArcLengthCtx> pc_arc_length_ctx;
8290 auto get_pc_arc = [&](
auto pc_mg) {
8292 boost::make_shared<PCArcLengthCtx>(pc_mg, *shellM,
m, arc_ctx);
8294 CHKERR PCSetType(pc_arc, PCSHELL);
8295 CHKERR PCShellSetContext(pc_arc, pc_arc_length_ctx.get());
8301 auto setup_snes = [&](
auto pc_mg,
auto pc_arc) {
8305 CHKERR SNESSetDM(snes, dm);
8308 CHKERR SNESAppendOptionsPrefix(snes,
"elastic_");
8309 CHKERR SNESSetFromOptions(snes);
8310 CHKERR SNESGetKSP(snes, &ksp);
8311 CHKERR KSPSetPC(ksp, pc_arc);
8313 #if PETSC_VERSION_GE(3, 8, 0)
8314 CHKERR PCFactorSetMatSolverType(pc_mg, MATSOLVERMUMPS);
8316 CHKERR PCFactorSetMatSolverPackage(pc_mg, MATSOLVERMUMPS);
8323 auto calculate_diagonal_scale = [&]() {
8329 const double *f_array;
8330 CHKERR VecGetArrayRead(
f, &f_array);
8335 constexpr
size_t nb_fields = 2;
8336 std::array<std::string, nb_fields> fields = {
"SPATIAL_POSITION",
8337 "LAMBDA_CLOSE_CRACK"};
8338 std::array<double, nb_fields> lnorms = {0, 0};
8339 std::array<double, nb_fields> norms = {0, 0};
8340 std::array<double, nb_fields> loc_nb_dofs = {0, 0};
8341 std::array<double, nb_fields> glob_nb_dofs = {0, 0};
8345 for (
size_t f = 0;
f != nb; ++
f) {
8348 rows_index.lower_bound(FieldEntity::getLoBitNumberUId(bit_number));
8350 rows_index.upper_bound(FieldEntity::getHiBitNumberUId(bit_number));
8351 for (
auto lo = lo_uid; lo != hi_uid; ++lo) {
8352 const int local_idx = (*lo)->getPetscLocalDofIdx();
8353 if (local_idx >= 0 && local_idx < nb_local) {
8354 const double val = f_array[local_idx];
8355 lnorms[
f] += val * val;
8361 CHKERR VecRestoreArrayRead(
f, &f_array);
8362 CHKERR MPIU_Allreduce(lnorms.data(), norms.data(), nb, MPIU_REAL, MPIU_SUM,
8363 PetscObjectComm((PetscObject)dm));
8364 CHKERR MPIU_Allreduce(loc_nb_dofs.data(), glob_nb_dofs.data(), nb,
8365 MPIU_REAL, MPIU_SUM,
8366 PetscObjectComm((PetscObject)dm));
8368 for (
auto &
v : norms)
8371 for (
size_t f = 0;
f != nb; ++
f)
8373 "Scaling diaginal for field [ %s ] by %9.8e nb. of dofs %d",
8374 fields[
f].c_str(), norms[
f],
8375 static_cast<double>(glob_nb_dofs[
f]));
8377 CHKERR VecSet(arc_snes_ctx->getVecDiagM(), 1.);
8378 double *diag_m_array;
8379 CHKERR VecGetArray(arc_snes_ctx->getVecDiagM(), &diag_m_array);
8381 for (
size_t f = 0;
f != nb; ++
f) {
8384 const auto lo_uid = FieldEntity::getLoBitNumberUId(bit_number);
8385 const auto hi_uid = FieldEntity::getHiBitNumberUId(bit_number);
8386 for (
auto lo = rows_index.lower_bound(lo_uid);
8387 lo != rows_index.upper_bound(hi_uid); ++lo) {
8388 const int local_idx = (*lo)->getPetscLocalDofIdx();
8389 if (local_idx >= 0 && local_idx < nb_local)
8390 diag_m_array[local_idx] = 1. / norms[
f];
8395 CHKERR VecGetArray(arc_snes_ctx->getVecDiagM(), &diag_m_array);
8401 CHKERR create_shell_matrix();
8402 auto pc_base = get_pc();
8403 auto pc_arc = get_pc_arc(pc_base);
8404 CHKERR setup_snes(pc_base, pc_arc);
8409 CHKERR VecGhostUpdateBegin(
f, INSERT_VALUES, SCATTER_FORWARD);
8410 CHKERR VecGhostUpdateEnd(
f, INSERT_VALUES, SCATTER_FORWARD);
8418 CHKERR calculate_diagonal_scale();
8420 auto create_section = [&]() {
8422 PetscSection section;
8423 CHKERR DMGetDefaultSection(dm, §ion);
8425 CHKERR PetscSectionGetNumFields(section, &num_fields);
8426 for (
int f = 0;
f != num_fields;
f++) {
8435 auto set_monitor = [&]() {
8437 PetscViewerAndFormat *vf;
8438 CHKERR PetscViewerAndFormatCreate(PETSC_VIEWER_STDOUT_WORLD,
8439 PETSC_VIEWER_DEFAULT, &vf);
8451 CHKERR SNESSolve(snes, PETSC_NULL, q);
8452 CHKERR VecGhostUpdateBegin(q, INSERT_VALUES, SCATTER_FORWARD);
8453 CHKERR VecGhostUpdateEnd(q, INSERT_VALUES, SCATTER_FORWARD);
8454 CHKERR SNESMonitorCancel(snes);
8457 CHKERR VecNorm(q, NORM_2, &fnorm);
8458 MOFEM_LOG_C(
"CPWorld", Sev::verbose,
"solution fnorm = %9.8e", fnorm);
8460 if (problem_ptr->
getName() ==
"EIGEN_ELASTIC") {
8462 "EIGEN_ELASTIC",
"SPATIAL_POSITION",
"EIGEN_SPATIAL_POSITIONS",
ROW, q,
8463 INSERT_VALUES, SCATTER_REVERSE);
◆ solvePropagationDM()
MoFEMErrorCode FractureMechanics::CrackPropagation::solvePropagationDM |
( |
DM |
dm, |
|
|
DM |
dm_elastic, |
|
|
SNES |
snes, |
|
|
Mat |
m, |
|
|
Vec |
q, |
|
|
Vec |
f |
|
) |
| |
solve crack propagation problem
- Parameters
-
dm | crack propagation DM that is composed of elastic DM and material DM |
dm_elastic | elastic DM |
snes | snes solver for crack propagation DM |
m | stiffness sub-matrix for crack propagation DM |
q | DoF sub-vector for crack propagation DM |
f | Rhs sub-vector for crack propagation DM |
snes_set_up | boolean flag wheather to determin snes solver |
- Returns
- error code
Definition at line 8494 of file CrackPropagation.cpp.
8500 Mat m_elastic = PETSC_NULL;
8509 dynamic_cast<CrackPropagation::ArcLengthSnesCtx *
>(snes_ctx);
8511 arc_snes_ctx->setVecDiagM(diag_m);
8512 CHKERR VecSet(arc_snes_ctx->getVecDiagM(), 1.);
8519 mwlsApprox->F_lambda = arc_snes_ctx->getArcPtr()->F_lambda;
8525 for (boost::ptr_map<string, NeumannForcesSurface>::iterator fit =
8533 for (boost::ptr_map<string, NeumannForcesSurface>::iterator fit =
8540 auto arc_mat_ctx = boost::make_shared<ArcLengthMatShell>(
8541 m, arc_snes_ctx->getArcPtr(), problem_ptr->
getName());
8543 auto create_shell_matrix = [&]() {
8548 CHKERR MatGetLocalSize(
m, &mm, &nn);
8550 static_cast<void *
>(arc_mat_ctx.get()), &shell_m);
8551 CHKERR MatShellSetOperation(shell_m, MATOP_MULT,
8556 auto set_monitor = [&]() {
8558 PetscViewerAndFormat *vf;
8559 CHKERR PetscViewerAndFormatCreate(PETSC_VIEWER_STDOUT_WORLD,
8560 PETSC_VIEWER_DEFAULT, &vf);
8569 auto zero_matrices_and_vectors = [&]() {
8573 CHKERR VecGhostUpdateBegin(
f, INSERT_VALUES, SCATTER_FORWARD);
8574 CHKERR VecGhostUpdateEnd(
f, INSERT_VALUES, SCATTER_FORWARD);
8578 auto create_section = [&]() {
8580 PetscSection section;
8581 CHKERR DMGetDefaultSection(dm, §ion);
8583 CHKERR PetscSectionGetNumFields(section, &num_fields);
8584 for (
int f = 0;
f != num_fields;
f++) {
8593 boost::shared_ptr<PCArcLengthCtx> pc_arc_length_ctx;
8595 auto create_pc_arc = [&](
auto pc_fs) {
8596 pc_arc_length_ctx = boost::make_shared<PCArcLengthCtx>(
8597 pc_fs, shell_m,
m, arc_snes_ctx->getArcPtr());
8599 CHKERR PCSetType(pc_arc, PCSHELL);
8600 CHKERR PCShellSetContext(pc_arc, pc_arc_length_ctx.get());
8606 auto set_up_snes = [&](
auto pc_arc,
auto pc_fs,
auto is_pcfs) {
8611 CHKERR SNESSetDM(snes, dm);
8614 CHKERR SNESSetFromOptions(snes);
8617 CHKERR SNESGetKSP(snes, &ksp);
8618 CHKERR KSPSetPC(ksp, pc_arc);
8622 CHKERR PCFieldSplitGetSubKSP(pc_fs, &
n, &sub_ksp);
8624 CHKERR KSPGetPC(sub_ksp[0], &pc_0);
8625 CHKERR KSPGetPC(sub_ksp[1], &pc_1);
8626 #if PETSC_VERSION_GE(3, 8, 0)
8627 CHKERR PCFactorSetMatSolverType(pc_0, MATSOLVERMUMPS);
8628 CHKERR PCFactorSetMatSolverType(pc_1, MATSOLVERMUMPS);
8630 CHKERR PCFactorSetMatSolverPackage(pc_0, MATSOLVERMUMPS);
8631 CHKERR PCFactorSetMatSolverPackage(pc_1, MATSOLVERMUMPS);
8635 #if PETSC_VERSION_GE(3, 8, 0)
8636 CHKERR PCFactorSetMatSolverType(pc_fs, MATSOLVERMUMPS);
8638 CHKERR PCFactorSetMatSolverPackage(pc_fs, MATSOLVERMUMPS);
8645 auto create_pc_fs = [&](
auto &is_pcfs) {
8647 CHKERR PCAppendOptionsPrefix(pc_fs,
"propagation_");
8648 CHKERR PCSetFromOptions(pc_fs);
8649 PetscObjectTypeCompare((PetscObject)pc_fs, PCFIELDSPLIT, &is_pcfs);
8653 boost::shared_ptr<ComposedProblemsData> cmp_data_ptr =
8655 for (
int ff = 0; ff !=
static_cast<int>(cmp_data_ptr->rowIs.size());
8657 CHKERR PCFieldSplitSetIS(pc_fs, NULL, cmp_data_ptr->rowIs[ff]);
8659 CHKERR PCSetOperators(pc_fs,
m,
m);
8665 auto calculate_diagonal_scale = [&](
bool debug =
false) {
8675 auto f_lambda = arc_snes_ctx->getArcPtr()->F_lambda;
8682 CHKERR VecSetOption(f_griffith, VEC_IGNORE_NEGATIVE_INDICES, PETSC_TRUE);
8684 auto assemble_mat = [&](DM dm, std::string name,
8685 boost::shared_ptr<FEMethod> fe, Mat
m) {
8687 fe->snes_ctx = SnesMethod::CTX_SNESSETJACOBIAN;
8691 fe->snes_ctx = SnesMethod::CTX_SNESNONE;
8695 auto assemble_vec = [&](DM dm, std::string name,
8696 boost::shared_ptr<FEMethod> fe,
Vec f) {
8698 fe->snes_ctx = SnesMethod::CTX_SNESSETFUNCTION;
8702 fe->snes_ctx = SnesMethod::CTX_SNESNONE;
8706 auto calculate_mat_and_vec = [&](
double set_arc_alpha,
8707 double set_smoothing_alpha,
double set_gc,
8710 bool stabilised =
smootherFe->smootherData.sTabilised;
8711 double arc_alpha = arc_snes_ctx->getArcPtr()->alpha;
8719 arc_snes_ctx->getArcPtr()->alpha = set_arc_alpha;
8725 CHKERR VecZeroEntries(f_griffith);
8728 CHKERR VecGhostUpdateBegin(f_griffith, ADD_VALUES, SCATTER_REVERSE);
8729 CHKERR VecGhostUpdateEnd(f_griffith, ADD_VALUES, SCATTER_REVERSE);
8730 CHKERR VecAssemblyBegin(f_griffith);
8731 CHKERR VecAssemblyEnd(f_griffith);
8735 CHKERR MatAssemblyBegin(
m, MAT_FINAL_ASSEMBLY);
8736 CHKERR MatAssemblyEnd(
m, MAT_FINAL_ASSEMBLY);
8737 CHKERR MatGetDiagonal(
m, diag_griffith);
8742 CHKERR MatAssemblyBegin(
m, MAT_FINAL_ASSEMBLY);
8743 CHKERR MatAssemblyEnd(
m, MAT_FINAL_ASSEMBLY);
8744 CHKERR MatGetDiagonal(
m, diag_smoothing);
8746 smootherFe->smootherData.sTabilised = stabilised;
8747 arc_snes_ctx->getArcPtr()->alpha = arc_alpha;
8755 auto get_norm_from_vec = [&](
Vec vec, std::string field,
Range *ents,
8759 const double *v_array;
8760 CHKERR VecGetArrayRead(vec, &v_array);
8763 const auto lo_uid = FieldEntity::getLoBitNumberUId(bit_number);
8764 const auto hi_uid = FieldEntity::getHiBitNumberUId(bit_number);
8766 for (
auto lo = rows_index.lower_bound(lo_uid);
8767 lo != rows_index.upper_bound(hi_uid); ++lo) {
8769 const int local_idx = (*lo)->getPetscLocalDofIdx();
8770 if (local_idx >= 0 && local_idx < nb_local) {
8772 bool no_skip =
true;
8774 bool contain = ents->find((*lo)->getEnt()) != ents->end();
8785 const double val = v_array[local_idx];
8786 ret_val += val * val;
8791 CHKERR VecRestoreArrayRead(vec, &v_array);
8792 return std::pair<double, double>(ret_val, size);
8795 auto set_norm_from_vec = [&](
Vec vec, std::string field,
const double norm,
8796 Range *ents,
bool not_contain) {
8799 if (!std::isnormal(norm))
8801 "Wrong scaling value");
8804 CHKERR VecGetArray(vec, &v_array);
8807 const auto lo_uid = FieldEntity::getLoBitNumberUId(bit_number);
8808 const auto hi_uid = FieldEntity::getHiBitNumberUId(bit_number);
8810 for (
auto lo = rows_index.lower_bound(lo_uid);
8811 lo != rows_index.upper_bound(hi_uid); ++lo) {
8813 const int local_idx = (*lo)->getPetscLocalDofIdx();
8814 if (local_idx >= 0 && local_idx < nb_local) {
8816 bool no_skip =
true;
8818 bool contain = ents->find((*lo)->getEnt()) != ents->end();
8829 v_array[local_idx] /= norm;
8832 CHKERR VecRestoreArray(vec, &v_array);
8836 auto calulate_norms = [&](
auto &lnorms,
auto &lsizes,
auto &norms,
8839 const double lambda = arc_snes_ctx->getArcPtr()->getFieldData();
8840 std::pair<double &, double &>(lnorms[0], lsizes[0]) =
8841 get_norm_from_vec(
f,
"LAMBDA_ARC_LENGTH",
nullptr,
false);
8842 std::pair<double &, double &>(lnorms[1], lsizes[1]) =
8843 get_norm_from_vec(f_lambda,
"SPATIAL_POSITION",
nullptr,
false);
8844 std::pair<double &, double &>(lnorms[2], lsizes[2]) = get_norm_from_vec(
8846 std::pair<double &, double &>(lnorms[3], lsizes[3]) = get_norm_from_vec(
8848 std::pair<double &, double &>(lnorms[4], lsizes[4]) = get_norm_from_vec(
8851 CHKERR MPIU_Allreduce(lnorms.data(), norms.data(), lnorms.size(),
8852 MPIU_REAL, MPIU_SUM,
8853 PetscObjectComm((PetscObject)dm));
8854 CHKERR MPIU_Allreduce(lsizes.data(), sizes.data(), lnorms.size(),
8855 MPIU_REAL, MPIU_SUM,
8856 PetscObjectComm((PetscObject)dm));
8858 for (
auto &
v : norms)
8862 norms[3] /= norms[1];
8863 norms[4] /= norms[1];
8864 norms[3] /= sizes[3];
8865 norms[4] /= sizes[4];
8870 auto test_scale = [&](
auto &fields,
auto &lnorms,
auto &lsizes,
auto &norms,
8873 CHKERR calculate_mat_and_vec(arc_snes_ctx->getArcPtr()->alpha,
8878 for (
auto &
v : lnorms)
8880 for (
auto &
v : lsizes)
8882 for (
auto &
v : norms)
8884 for (
auto &
v : sizes)
8887 CHKERR VecPointwiseMult(
f,
f, arc_snes_ctx->getVecDiagM());
8888 CHKERR VecPointwiseMult(f_lambda, f_lambda, arc_snes_ctx->getVecDiagM());
8889 CHKERR VecPointwiseMult(f_griffith, f_griffith,
8890 arc_snes_ctx->getVecDiagM());
8891 CHKERR VecPointwiseMult(diag_griffith, diag_griffith,
8892 arc_snes_ctx->getVecDiagM());
8893 CHKERR VecPointwiseMult(diag_smoothing, diag_smoothing,
8894 arc_snes_ctx->getVecDiagM());
8896 CHKERR calulate_norms(lnorms, lsizes, norms, sizes);
8901 for (
size_t f = 0;
f != fields.size(); ++
f)
8903 "Norms for field [ %s ] = %9.8e (%6.0f)", fields[
f].c_str(),
8904 norms[
f], sizes[
f]);
8908 CHKERR calculate_mat_and_vec(1, 1,
gC, 1);
8910 constexpr
size_t nb_fields = 5;
8911 std::array<std::string, nb_fields> fields = {
8912 "LAMBDA_ARC_LENGTH",
"FLAMBDA",
"GRIFFITH_FORCE",
"GRIFFITH_FORCE_LHS",
8913 "SMOOTHING_CONSTRAIN_LHS"};
8914 std::array<double, nb_fields> lnorms = {0, 0, 0, 0, 0};
8915 std::array<double, nb_fields> norms = {0, 0, 0, 0, 0};
8916 std::array<double, nb_fields> lsizes = {0, 0, 0, 0, 0};
8917 std::array<double, nb_fields> sizes = {0, 0, 0, 0, 0};
8919 CHKERR calulate_norms(lnorms, lsizes, norms, sizes);
8921 constexpr
size_t nb_fields_contact = 2;
8922 std::array<std::string, nb_fields> fields_contact = {
"LAMBDA_CONTACT"};
8923 std::array<double, nb_fields> lnorms_contact = {0, 0};
8924 std::array<double, nb_fields> norms_contact = {0, 0};
8925 std::array<double, nb_fields> lsizes_contact = {0, 0};
8926 std::array<double, nb_fields> sizes_contact = {0, 0};
8930 for (
size_t f = 0;
f != nb_fields; ++
f)
8932 "Norms for field [ %s ] = %9.8e (%6.0f)", fields[
f].c_str(),
8933 norms[
f], sizes[
f]);
8935 arc_snes_ctx->getArcPtr()->alpha =
arcAlpha / norms[0];
8936 const double scaled_smoother_alpha =
smootherAlpha * norms[3] / norms[4];
8940 CHKERR set_norm_from_vec(arc_snes_ctx->getVecDiagM(),
"SPATIAL_POSITION",
8941 norms[1],
nullptr,
false);
8942 CHKERR set_norm_from_vec(arc_snes_ctx->getVecDiagM(),
"MESH_NODE_POSITIONS",
8943 norms[1],
nullptr,
false);
8947 MOFEM_LOG_C(
"CPWorld", Sev::inform,
"Set scaled arc length alpha = %4.3e",
8948 arc_snes_ctx->getArcPtr()->alpha);
8949 MOFEM_LOG_C(
"CPWorld", Sev::inform,
"Set scaled smoothing alpha = %4.3e",
8951 MOFEM_LOG_C(
"CPWorld", Sev::inform,
"Set scaled griffith E = %4.3e",
8955 CHKERR test_scale(fields, lnorms, lsizes, norms, sizes);
8962 PetscBool is_pcfs = PETSC_FALSE;
8964 CHKERR create_shell_matrix();
8965 auto pc_fs = create_pc_fs(is_pcfs);
8966 auto pc_arc = create_pc_arc(pc_fs);
8967 CHKERR set_up_snes(pc_arc, pc_fs, is_pcfs);
8971 CHKERR zero_matrices_and_vectors();
8980 CHKERR calculate_diagonal_scale(
false);
8985 <<
"Resest MWLS approximation coefficients. Coefficients will be "
8986 "recalulated for current material positions.";
8993 CHKERR SNESSolve(snes, PETSC_NULL, q);
8994 CHKERR VecGhostUpdateBegin(q, INSERT_VALUES, SCATTER_FORWARD);
8995 CHKERR VecGhostUpdateEnd(q, INSERT_VALUES, SCATTER_FORWARD);
8996 CHKERR SNESMonitorCancel(snes);
8999 CHKERR VecNorm(q, NORM_2, &fnorm);
9002 CHKERR MatDestroy(&shell_m);
9004 CHKERR MatDestroy(&m_elastic);
◆ testJacobians()
test LHS Jacobians
- Parameters
-
bit | bit level |
mask | bitref mask |
test_nb | name of the test |
Definition at line 5279 of file CrackPropagation.cpp.
5285 boost::shared_ptr<CrackFrontElement> fe_rhs;
5286 boost::shared_ptr<CrackFrontElement> fe_lhs;
5299 "There is no such test defined");
5304 CHKERR DMCreate(PETSC_COMM_WORLD, &dm);
5305 CHKERR DMSetType(dm,
"MOFEM");
5320 Vec front_f, tangent_front_f;
5321 CHKERR DMCreateGlobalVector(dm, &front_f);
5322 CHKERR VecDuplicate(front_f, &tangent_front_f);
5323 CHKERR VecSetOption(front_f, VEC_IGNORE_NEGATIVE_INDICES, PETSC_TRUE);
5324 CHKERR VecSetOption(tangent_front_f, VEC_IGNORE_NEGATIVE_INDICES, PETSC_TRUE);
5326 if (
smootherFe->smootherData.frontF != PETSC_NULL)
5328 if (
smootherFe->smootherData.tangentFrontF != PETSC_NULL)
5332 smootherFe->smootherData.tangentFrontF = tangent_front_f;
5349 VEC_IGNORE_NEGATIVE_INDICES, PETSC_TRUE);
5353 boost::shared_ptr<FEMethod>
null;
5371 if (
m.first != getInterface<CPMeshCut>()->getCrackSurfaceId()) {
5402 if (
m.first != getInterface<CPMeshCut>()->getCrackSurfaceId()) {
5427 "There is no such test defined");
5432 "MESH_NODE_POSITIONS");
5438 CHKERR VecSetOption(
f, VEC_IGNORE_NEGATIVE_INDICES, PETSC_TRUE);
5439 CHKERR VecSetOption(q, VEC_IGNORE_NEGATIVE_INDICES, PETSC_TRUE);
5443 CHKERR VecCopy(q, q_copy);
5451 CHKERR VecGhostUpdateBegin(
f, INSERT_VALUES, SCATTER_FORWARD);
5452 CHKERR VecGhostUpdateEnd(
f, INSERT_VALUES, SCATTER_FORWARD);
5454 PetscBool calculate_fd = PETSC_FALSE;
5456 &calculate_fd, PETSC_NULL);
5459 CHKERR SNESCreate(PETSC_COMM_WORLD, &snes);
5460 CHKERR SNESSetDM(snes, dm);
5463 CHKERR SNESAppendOptionsPrefix(snes,
"test_mwls_");
5465 if (calculate_fd == PETSC_FALSE) {
5466 char testing_options[] =
5467 "-test_mwls_snes_test_jacobian "
5468 "-test_mwls_snes_test_jacobian_display "
5469 "-test_mwls_snes_no_convergence_test -test_mwls_snes_atol 0 "
5470 "-test_mwls_snes_rtol 0 -test_mwls_snes_max_it 1 -test_mwls_pc_type "
5472 CHKERR PetscOptionsInsertString(NULL, testing_options);
5474 char testing_options[] =
5475 "-test_mwls_snes_no_convergence_test -test_mwls_snes_atol 0 "
5476 "-test_mwls_snes_rtol 0 -test_mwls_snes_max_it 1 -test_mwls_pc_type "
5478 CHKERR PetscOptionsInsertString(NULL, testing_options);
5483 CHKERR SNESSetFromOptions(snes);
5492 CHKERR SNESSolve(snes, PETSC_NULL, q);
5494 if (calculate_fd == PETSC_TRUE) {
5496 CHKERR MatNorm(
m, NORM_INFINITY, &nrm_m0);
5498 char testing_options_fd[] =
"-test_mwls_snes_fd";
5499 CHKERR PetscOptionsInsertString(NULL, testing_options_fd);
5503 CHKERR SNESSetJacobian(snes, fd_m, fd_m,
SnesMat, snes_ctx);
5504 CHKERR SNESSetFromOptions(snes);
5508 CHKERR SNESSolve(snes, NULL, q_copy);
5510 CHKERR MatAXPY(
m, -1, fd_m, SUBSET_NONZERO_PATTERN);
5513 CHKERR MatNorm(
m, NORM_INFINITY, &nrm_m);
5514 PetscPrintf(PETSC_COMM_WORLD,
"Matrix norms %3.4e %3.4e %3.4e\n", nrm_m0,
5515 nrm_m, nrm_m / nrm_m0);
5518 const double tol = 1e-6;
5521 "Difference between hand-calculated tangent matrix and finite "
5522 "difference matrix is too big");
5526 CHKERR SNESDestroy(&snes);
◆ tetsingReleaseEnergyCalculation()
MoFEMErrorCode FractureMechanics::CrackPropagation::tetsingReleaseEnergyCalculation |
( |
| ) |
|
This is run with ctest.
- Returns
- error code
Definition at line 929 of file CrackPropagation.cpp.
933 CIRCULAR_PLATE_IN_INFINITE_BODY,
934 ANGLE_ANALYTICAL_MODE_1,
935 MWLS_INTERNAL_STRESS,
936 MWLS_INTERNAL_STRESS_ANALYTICAL,
941 const char *list[] = {
"analytical_mode_1",
942 "circular_plate_in_infinite_body",
943 "angle_analytical_mode_1",
944 "mwls_internal_stress",
945 "mwls_internal_stress_analytical",
947 "notch_with_density"};
949 int choice_value = ANALYTICAL_MODE_1;
954 &choice_value, &flg);
955 CHKERR PetscOptionsScalar(
"-test_tol",
"test tolerance",
"", test_tol,
956 &test_tol, PETSC_NULL);
957 ierr = PetscOptionsEnd();
960 if (flg == PETSC_TRUE) {
964 switch (choice_value) {
965 case ANALYTICAL_MODE_1: {
967 for (map<EntityHandle, double>::iterator mit =
mapG1.begin();
968 mit !=
mapG1.end(); mit++) {
969 ave_g1 += mit->second;
971 ave_g1 /=
mapG1.size();
973 const double gc = 1e-5;
975 const double error = fabs(ave_g1 - gc) / gc;
978 "ave_gc = %6.4e gc = %6.4e tolerance = %6.4e error = %6.4e\n",
979 ave_g1, gc, test_tol, error);
980 if (error > test_tol || error != error) {
982 "ANALYTICAL_MODE_1 test failed");
985 case CIRCULAR_PLATE_IN_INFINITE_BODY: {
986 const double gc = 1.1672e+01;
987 double max_error = 0;
988 for (map<EntityHandle, double>::iterator mit =
mapG1.begin();
989 mit !=
mapG1.end(); mit++) {
990 const double g = mit->second;
991 const double error = fabs(
g - gc) / gc;
992 max_error = (max_error < error) ? error : max_error;
995 "g = %6.4e gc = %6.4e tolerance = %6.4e error = %6.4e\n",
g, gc,
998 if (max_error > test_tol || max_error != max_error)
1000 "CIRCULAR_PLATE_IN_INFINITE_BODY test failed");
1003 case ANGLE_ANALYTICAL_MODE_1: {
1004 const double gc = 1e-5;
1005 double max_error = 0;
1006 for (map<EntityHandle, double>::iterator mit =
mapG1.begin();
1007 mit !=
mapG1.end(); mit++) {
1008 const double g = mit->second;
1009 const double error = fabs(
g - gc) / gc;
1010 max_error = (max_error < error) ? error : max_error;
1013 "g = %6.4e gc = %6.4e tolerance = %6.4e error = %6.4e\n",
g, gc,
1016 if (max_error > test_tol || max_error != max_error) {
1018 "ANGLE_ANALYTICAL_MODE_1 test failed %6.4e > %6.4e",
1019 max_error, test_tol);
1022 case MWLS_INTERNAL_STRESS: {
1025 double max_error = 0;
1026 for (map<EntityHandle, double>::iterator mit =
mapG1.begin();
1027 mit !=
mapG1.end(); mit++) {
1028 const double g = mit->second;
1029 const double error = fabs(
g - gc) / gc;
1030 max_error = (max_error < error) ? error : max_error;
1033 "g = %6.4e gc = %6.4e tolerance = %6.4e error = %6.4e\n",
g, gc,
1036 if (max_error > test_tol || max_error != max_error) {
1038 "MWLS_INTERNAL_STRESS test failed");
1041 case MWLS_INTERNAL_STRESS_ANALYTICAL: {
1044 double max_error = 0;
1045 for (map<EntityHandle, double>::iterator mit =
mapG1.begin();
1046 mit !=
mapG1.end(); mit++) {
1047 const double g = mit->second;
1048 const double error = fabs(
g - gc) / gc;
1049 max_error = (max_error < error) ? error : max_error;
1052 "g = %6.4e gc = %6.4e tolerance = %6.4e error = %6.4e\n",
g, gc,
1055 if (max_error > test_tol || max_error != max_error) {
1057 "MWLS_INTERNAL_STRESS_ANALYTICAL test failed");
1060 case CUT_CIRCLE_PLATE: {
1064 const double g =
m.second;
1065 max_g = fmax(
g, max_g);
1069 double error = fabs(max_g - gc) / gc;
1070 if (error > test_tol || error != error) {
1072 "CUT_CIRCLE_PLATE test failed");
1075 case NOTCH_WITH_DENSITY: {
1078 for (map<EntityHandle, double>::iterator mit =
mapG1.begin();
1079 mit !=
mapG1.end(); mit++) {
1080 const double g = mit->second;
1081 max_g = fmax(
g, max_g);
1083 const double gc = 1.6642e-04;
1085 double error = fabs(max_g - gc) / gc;
1086 if (error > test_tol || error != error) {
1088 "NOTCH_WITH_DENSITY test failed");
◆ unsetSingularElementMatrialPositions()
MoFEMErrorCode FractureMechanics::CrackPropagation::unsetSingularElementMatrialPositions |
( |
| ) |
|
remove singularity
Definition at line 9388 of file CrackPropagation.cpp.
9396 mField,
"MESH_NODE_POSITIONS", *eit, dit)) {
9397 dit->get()->getFieldData() = 0;
◆ updateMaterialFixedNode()
MoFEMErrorCode FractureMechanics::CrackPropagation::updateMaterialFixedNode |
( |
const bool |
fix_front, |
|
|
const bool |
fix_small_g, |
|
|
const bool |
debug = false |
|
) |
| |
Update fixed nodes.
Definition at line 6898 of file CrackPropagation.cpp.
6902 Range fix_material_nodes;
6903 CHKERR GetSmoothingElementsSkin (*
this)(fix_material_nodes, fix_small_g,
6913 "Number of fixed nodes %d, number of fixed crack front nodes %d "
6915 fix_material_nodes.size(),
◆ writeCrackFont()
Definition at line 8093 of file CrackPropagation.cpp.
8097 auto make_file_name = [step](
const std::string prefix,
8098 const std::string surfix =
".vtk") {
8099 return prefix + boost::lexical_cast<std::string>(step) + surfix;
8102 auto write_file = [
this, make_file_name](
const std::string prefix,
8107 std::string file_name = make_file_name(prefix);
8121 if (!crack_front_edge.empty())
8122 CHKERR write_file(
"out_crack_front_", crack_front_edge);
8126 double edge_node_coords[6];
8129 &edge_node_coords[2]),
8131 &edge_node_coords[5])};
8135 double sum_crack_front_length = 0;
8136 for (
auto edge : crack_front_edge) {
8141 t_node_edge[0](
i) -= t_node_edge[1](
i);
8142 double l = sqrt(t_node_edge[0](
i) * t_node_edge[0](
i));
8143 sum_crack_front_length +=
l;
8146 sum_crack_front_length);
8148 double rate_cf = 0.;
8151 PetscPrintf(
mField.
get_comm(),
" | Change rate = %6.4e\n", rate_cf);
8161 CHKERR write_file(
"out_crack_surface_", crack_surface);
8171 CHKERR Tools::getTriNormal(coords, &t_normal(0));
8173 return sqrt(t_normal(
i) * t_normal(
i)) * 0.5;
8176 double sum_crack_area = 0;
8177 for (
auto tri : crack_surface) {
8178 sum_crack_area += get_tri_area(tri);
8180 PetscPrintf(
mField.
get_comm(),
"Crack surface area = %6.4e", sum_crack_area);
8182 double rate_cs = 0.;
8185 PetscPrintf(
mField.
get_comm(),
" | Change rate = %6.4e\n", rate_cs);
8195 CHKERR write_file(
"out_volume_", bit_tets);
8199 CHKERR skin.find_skin(0, bit_tets,
false, tets_skin);
8200 CHKERR write_file(
"out_skin_volume_", tets_skin);
◆ zeroLambdaFields()
MoFEMErrorCode FractureMechanics::CrackPropagation::zeroLambdaFields |
( |
| ) |
|
Zero fields with lagrange multipliers.
Definition at line 9553 of file CrackPropagation.cpp.
9558 for (
auto f : *fields_ptr) {
9559 if (
f->getName().compare(0, 6,
"LAMBDA") == 0 &&
9560 f->getName() !=
"LAMBDA_ARC_LENGTH" &&
9561 f->getName() !=
"LAMBDA_CONTACT" &&
9562 f->getName() !=
"LAMBDA_CLOSE_CRACK") {
◆ addAnalyticalInternalStressOperators
PetscBool FractureMechanics::CrackPropagation::addAnalyticalInternalStressOperators |
◆ addSingularity
PetscBool FractureMechanics::CrackPropagation::addSingularity |
◆ analiticalSurfaceElement
boost::shared_ptr<boost::ptr_map<string, NeumannForcesSurface> > FractureMechanics::CrackPropagation::analiticalSurfaceElement |
◆ analyticalDirichletBc
◆ approxOrder
int FractureMechanics::CrackPropagation::approxOrder |
◆ arcAlpha
double FractureMechanics::CrackPropagation::arcAlpha |
◆ arcBeta
double FractureMechanics::CrackPropagation::arcBeta |
◆ arcCtx
boost::shared_ptr<ArcLengthCtx> FractureMechanics::CrackPropagation::arcCtx |
◆ arcEigenCtx
boost::shared_ptr<ArcLengthCtx> FractureMechanics::CrackPropagation::arcEigenCtx |
◆ arcLengthDof
boost::shared_ptr<DofEntity> FractureMechanics::CrackPropagation::arcLengthDof |
◆ arcLengthVertex
EntityHandle FractureMechanics::CrackPropagation::arcLengthVertex |
◆ arcS
double FractureMechanics::CrackPropagation::arcS |
◆ areSpringsAle
PetscBool FractureMechanics::CrackPropagation::areSpringsAle |
◆ assembleFlambda
boost::shared_ptr<FEMethod> FractureMechanics::CrackPropagation::assembleFlambda |
◆ betaGc
double FractureMechanics::CrackPropagation::betaGc |
◆ bitEnts
Range FractureMechanics::CrackPropagation::bitEnts |
◆ bitProcEnts
Range FractureMechanics::CrackPropagation::bitProcEnts |
◆ bodySkin
Range FractureMechanics::CrackPropagation::bodySkin |
◆ bothSidesConstrains
◆ bothSidesContactConstrains
◆ closeCrackConstrains
◆ cnValue
double FractureMechanics::CrackPropagation::cnValue |
Augmentation parameter in the complementarity function used to enforce KKT constraints
Definition at line 1230 of file CrackPropagation.hpp.
◆ commonDataMortarContact
◆ commonDataSimpleContact
◆ commonDataSimpleContactALE
◆ commonDataSpringsALE
◆ commonDataSurfaceForceAle
◆ commonDataSurfacePressureAle
◆ configFile
std::string FractureMechanics::CrackPropagation::configFile |
◆ constrainedInterface
Range FractureMechanics::CrackPropagation::constrainedInterface |
◆ contactBothSidesMasterFaces
Range FractureMechanics::CrackPropagation::contactBothSidesMasterFaces |
◆ contactBothSidesMasterNodes
Range FractureMechanics::CrackPropagation::contactBothSidesMasterNodes |
◆ contactBothSidesSlaveFaces
Range FractureMechanics::CrackPropagation::contactBothSidesSlaveFaces |
◆ contactElements
Range FractureMechanics::CrackPropagation::contactElements |
◆ contactLambdaOrder
int FractureMechanics::CrackPropagation::contactLambdaOrder |
Approximation order for field of Lagrange multipliers used to enforce contact
Definition at line 1226 of file CrackPropagation.hpp.
◆ contactMasterFaces
Range FractureMechanics::CrackPropagation::contactMasterFaces |
◆ contactMeshsetFaces
Range FractureMechanics::CrackPropagation::contactMeshsetFaces |
◆ contactOrder
int FractureMechanics::CrackPropagation::contactOrder |
Approximation order for spatial positions used in contact elements
Definition at line 1224 of file CrackPropagation.hpp.
◆ contactOrientation
◆ contactOutputIntegPts
PetscBool FractureMechanics::CrackPropagation::contactOutputIntegPts |
◆ contactPostProcCore
moab::Core FractureMechanics::CrackPropagation::contactPostProcCore |
◆ contactPostProcMoab
moab::Interface& FractureMechanics::CrackPropagation::contactPostProcMoab |
◆ contactProblem
◆ contactSearchMultiIndexPtr
◆ contactSlaveFaces
Range FractureMechanics::CrackPropagation::contactSlaveFaces |
◆ contactTets
Range FractureMechanics::CrackPropagation::contactTets |
◆ cpMeshCutPtr
const boost::scoped_ptr<UnknownInterface> FractureMechanics::CrackPropagation::cpMeshCutPtr |
◆ cpSolversPtr
const boost::scoped_ptr<UnknownInterface> FractureMechanics::CrackPropagation::cpSolversPtr |
◆ crackAccelerationFactor
double FractureMechanics::CrackPropagation::crackAccelerationFactor |
◆ crackFaces
Range FractureMechanics::CrackPropagation::crackFaces |
◆ crackFront
Range FractureMechanics::CrackPropagation::crackFront |
◆ crackFrontElements
Range FractureMechanics::CrackPropagation::crackFrontElements |
◆ crackFrontLength
double FractureMechanics::CrackPropagation::crackFrontLength |
◆ crackFrontNodes
Range FractureMechanics::CrackPropagation::crackFrontNodes |
◆ crackFrontNodesEdges
Range FractureMechanics::CrackPropagation::crackFrontNodesEdges |
◆ crackFrontNodesTris
Range FractureMechanics::CrackPropagation::crackFrontNodesTris |
◆ crackOrientation
◆ crackSurfaceArea
double FractureMechanics::CrackPropagation::crackSurfaceArea |
◆ debug
bool CrackPropagation::debug = false |
|
static |
◆ defaultMaterial
int FractureMechanics::CrackPropagation::defaultMaterial |
◆ densityMapBlock
int FractureMechanics::CrackPropagation::densityMapBlock |
Block on which density is applied (Note: if used with internal stress, it has to be the same ID!)
Definition at line 137 of file CrackPropagation.hpp.
◆ doCutMesh
PetscBool FractureMechanics::CrackPropagation::doCutMesh |
◆ doElasticWithoutCrack
PetscBool FractureMechanics::CrackPropagation::doElasticWithoutCrack |
◆ doSurfaceProjection
bool FractureMechanics::CrackPropagation::doSurfaceProjection |
◆ edgeConstrains
◆ edgeForces
boost::shared_ptr<boost::ptr_map<string, EdgeForce> > FractureMechanics::CrackPropagation::edgeForces |
◆ elasticFe
Integrate spatial stresses, matrix and vector (not only element but all data structure)
Definition at line 1063 of file CrackPropagation.hpp.
◆ feCouplingElasticLhs
boost::shared_ptr<CrackFrontElement> FractureMechanics::CrackPropagation::feCouplingElasticLhs |
◆ feCouplingMaterialLhs
boost::shared_ptr<CrackFrontElement> FractureMechanics::CrackPropagation::feCouplingMaterialLhs |
◆ feEnergy
◆ feGriffithConstrainsDelta
◆ feGriffithConstrainsLhs
◆ feGriffithConstrainsRhs
◆ feGriffithForceLhs
◆ feGriffithForceRhs
◆ feLhs
◆ feLhsMortarContact
◆ feLhsSimpleContact
◆ feLhsSimpleContactALE
◆ feLhsSimpleContactALEMaterial
◆ feLhsSpringALE
◆ feLhsSpringALEMaterial
◆ feLhsSurfaceForceALEMaterial
◆ feMaterialAnaliticalTraction
◆ feMaterialLhs
boost::shared_ptr<CrackFrontElement> FractureMechanics::CrackPropagation::feMaterialLhs |
◆ feMaterialRhs
boost::shared_ptr<CrackFrontElement> FractureMechanics::CrackPropagation::feMaterialRhs |
◆ fePostProcMortarContact
◆ fePostProcSimpleContact
◆ feRhs
◆ feRhsMortarContact
◆ feRhsSimpleContact
◆ feRhsSimpleContactALEMaterial
◆ feRhsSpringALEMaterial
◆ feRhsSurfaceForceALEMaterial
◆ feSmootherLhs
◆ feSmootherRhs
◆ feSpringLhsPtr
◆ feSpringRhsPtr
◆ fileVersion
Version FractureMechanics::CrackPropagation::fileVersion |
◆ fixContactNodes
PetscBool FractureMechanics::CrackPropagation::fixContactNodes |
If set true, contact nodes are fixed in the material configuration
Definition at line 1235 of file CrackPropagation.hpp.
◆ fixMaterialEnts
◆ fractionOfFixedNodes
double FractureMechanics::CrackPropagation::fractionOfFixedNodes |
◆ gC
double FractureMechanics::CrackPropagation::gC |
◆ geometryOrder
int FractureMechanics::CrackPropagation::geometryOrder |
◆ griffithE
double FractureMechanics::CrackPropagation::griffithE |
◆ griffithForceElement
◆ griffithR
double FractureMechanics::CrackPropagation::griffithR |
◆ ignoreContact
PetscBool FractureMechanics::CrackPropagation::ignoreContact |
If set true, contact interfaces are ignored and prism interfaces are not created
Definition at line 1233 of file CrackPropagation.hpp.
◆ ignoreMaterialForce
PetscBool FractureMechanics::CrackPropagation::ignoreMaterialForce |
◆ initialSmootherAlpha
double FractureMechanics::CrackPropagation::initialSmootherAlpha |
◆ isGcHeterogeneous
PetscBool FractureMechanics::CrackPropagation::isGcHeterogeneous |
◆ isPartitioned
PetscBool FractureMechanics::CrackPropagation::isPartitioned |
◆ isPressureAle
PetscBool FractureMechanics::CrackPropagation::isPressureAle |
◆ isStressTagSavedOnNodes
bool FractureMechanics::CrackPropagation::isStressTagSavedOnNodes |
◆ isSurfaceForceAle
PetscBool FractureMechanics::CrackPropagation::isSurfaceForceAle |
◆ loadScale
double FractureMechanics::CrackPropagation::loadScale |
◆ mapBitLevel
std::map<std::string, BitRefLevel> FractureMechanics::CrackPropagation::mapBitLevel |
Map associating string literals to bit refinement levels The following convention is currently used: "mesh_cut" : level 0 "spatial_domain" : level 1 "material_domain" : level 2
Definition at line 180 of file CrackPropagation.hpp.
◆ mapG1
◆ mapG3
◆ mapGriffith
map<EntityHandle, VectorDouble3> FractureMechanics::CrackPropagation::mapGriffith |
◆ mapJ
◆ mapMatForce
map<EntityHandle, VectorDouble3> FractureMechanics::CrackPropagation::mapMatForce |
◆ mapSmoothingForceFactor
◆ materialFe
◆ maxG1
double FractureMechanics::CrackPropagation::maxG1 |
◆ maxJ
double FractureMechanics::CrackPropagation::maxJ |
◆ meshsetFaces
EntityHandle FractureMechanics::CrackPropagation::meshsetFaces |
◆ mField
◆ moabCommWorld
boost::shared_ptr<WrapMPIComm> FractureMechanics::CrackPropagation::moabCommWorld |
◆ mortarContactElements
Range FractureMechanics::CrackPropagation::mortarContactElements |
◆ mortarContactMasterFaces
Range FractureMechanics::CrackPropagation::mortarContactMasterFaces |
◆ mortarContactProblemPtr
boost::shared_ptr<MortarContactProblem> FractureMechanics::CrackPropagation::mortarContactProblemPtr |
◆ mortarContactSlaveFaces
Range FractureMechanics::CrackPropagation::mortarContactSlaveFaces |
◆ mwlsApprox
boost::shared_ptr<MWLSApprox> FractureMechanics::CrackPropagation::mwlsApprox |
◆ mwlsApproxFile
std::string FractureMechanics::CrackPropagation::mwlsApproxFile |
◆ mwlsEigenStressTagName
std::string FractureMechanics::CrackPropagation::mwlsEigenStressTagName |
◆ mwlsRhoTagName
std::string FractureMechanics::CrackPropagation::mwlsRhoTagName |
◆ mwlsStressTagName
std::string FractureMechanics::CrackPropagation::mwlsStressTagName |
◆ nbCutSteps
int FractureMechanics::CrackPropagation::nbCutSteps |
◆ nbLoadSteps
int FractureMechanics::CrackPropagation::nbLoadSteps |
◆ nBone
double FractureMechanics::CrackPropagation::nBone |
◆ nodalForces
boost::shared_ptr<boost::ptr_map<string, NodalForce> > FractureMechanics::CrackPropagation::nodalForces |
◆ oneSideCrackFaces
Range FractureMechanics::CrackPropagation::oneSideCrackFaces |
◆ onlyHooke
bool FractureMechanics::CrackPropagation::onlyHooke |
◆ onlyHookeFromOptions
PetscBool FractureMechanics::CrackPropagation::onlyHookeFromOptions |
◆ otherSideConstrains
PetscBool FractureMechanics::CrackPropagation::otherSideConstrains |
◆ otherSideCrackFaces
Range FractureMechanics::CrackPropagation::otherSideCrackFaces |
◆ parallelReadAndBroadcast
bool CrackPropagation::parallelReadAndBroadcast |
|
static |
Initial value:That is unstable development, for some meshses (propably generated by cubit) this is not working. Error can be attributed to bug in MOAB.
Definition at line 81 of file CrackPropagation.hpp.
◆ partitioningWeightPower
double FractureMechanics::CrackPropagation::partitioningWeightPower |
Weight controling load balance. Elements at the crack front are higher order, also have more fildes, associated with ALE formulation. Higher number put more weight on those elements while partitioning.
Definition at line 143 of file CrackPropagation.hpp.
◆ postProcLevel
int FractureMechanics::CrackPropagation::postProcLevel |
◆ printContactState
PetscBool FractureMechanics::CrackPropagation::printContactState |
◆ projFrontCtx
◆ projSurfaceCtx
◆ propagateCrack
PetscBool FractureMechanics::CrackPropagation::propagateCrack |
◆ refAtCrackTip
int FractureMechanics::CrackPropagation::refAtCrackTip |
◆ refOrderAtTip
int FractureMechanics::CrackPropagation::refOrderAtTip |
◆ resetMWLSCoeffsEveryPropagationStep
PetscBool FractureMechanics::CrackPropagation::resetMWLSCoeffsEveryPropagationStep |
If true, MWLS coefficients are recalulated every propagaton step.
Definition at line 149 of file CrackPropagation.hpp.
◆ residualStressBlock
int FractureMechanics::CrackPropagation::residualStressBlock |
◆ rHo0
double FractureMechanics::CrackPropagation::rHo0 |
◆ rValue
double FractureMechanics::CrackPropagation::rValue |
Parameter for regularizing absolute value function in the complementarity function
Definition at line 1228 of file CrackPropagation.hpp.
◆ setSingularCoordinates
bool FractureMechanics::CrackPropagation::setSingularCoordinates |
◆ skinOrientation
◆ smootherAlpha
double FractureMechanics::CrackPropagation::smootherAlpha |
◆ smootherFe
boost::shared_ptr<Smoother> FractureMechanics::CrackPropagation::smootherFe |
◆ smootherGamma
double FractureMechanics::CrackPropagation::smootherGamma |
◆ solveEigenStressProblem
PetscBool FractureMechanics::CrackPropagation::solveEigenStressProblem |
◆ spatialDirichletBc
◆ startStep
int FractureMechanics::CrackPropagation::startStep |
◆ surfaceConstrain
◆ surfaceForceAle
boost::shared_ptr<boost::ptr_map<string, NeumannForcesSurface> > FractureMechanics::CrackPropagation::surfaceForceAle |
◆ surfaceForces
boost::shared_ptr<boost::ptr_map<string, NeumannForcesSurface> > FractureMechanics::CrackPropagation::surfaceForces |
◆ surfacePressure
boost::shared_ptr<boost::ptr_map<string, NeumannForcesSurface> > FractureMechanics::CrackPropagation::surfacePressure |
◆ surfacePressureAle
boost::shared_ptr<boost::ptr_map<string, NeumannForcesSurface> > FractureMechanics::CrackPropagation::surfacePressureAle |
◆ tangentConstrains
◆ useEigenPositionsSimpleContact
PetscBool FractureMechanics::CrackPropagation::useEigenPositionsSimpleContact |
◆ volumeLengthAdouble
◆ volumeLengthDouble
◆ zeroFlambda
boost::shared_ptr<FEMethod> FractureMechanics::CrackPropagation::zeroFlambda |
The documentation for this struct was generated from the following files:
#define MoFEMFunctionReturnHot(a)
Last executable line of each PETSc function used for error handling. Replaces return()
MoFEMErrorCode getInterface(IFACE *&iface) const
Get interface refernce to pointer of interface.
boost::shared_ptr< GriffithForceElement::MyTriangleFEConstrains > feGriffithConstrainsLhs
MoFEMErrorCode addHOOpsFace3D(const std::string field, E &e, bool hcurl, bool hdiv)
PetscErrorCode DMMoFEMSetSnesCtx(DM dm, boost::shared_ptr< MoFEM::SnesCtx > snes_ctx)
Set MoFEM::SnesCtx data structure.
MoFEMErrorCode declareSurfaceForceAleFE(const BitRefLevel bit, const BitRefLevel mask=BitRefLevel().set(), const bool proc_only=true)
Declare FE for pressure BC in ALE formulation (in material domain)
static MoFEMErrorCode getElementOptions(BlockData &block_data)
MoFEMErrorCode addMWLSDensityOperators(boost::shared_ptr< CrackFrontElement > &fe_rhs, boost::shared_ptr< CrackFrontElement > &fe_lhs)
#define MYPCOMM_INDEX
default communicator number PCOMM
virtual MoFEMErrorCode add_ents_to_finite_element_by_MESHSET(const EntityHandle meshset, const std::string &name, const bool recursive=false)=0
add MESHSET element to finite element database given by name
RHS-operator for pressure element (spatial configuration)
boost::shared_ptr< AnalyticalDirichletBC::DirichletBC > analyticalDirichletBc
virtual MoFEMErrorCode loop_dofs(const Problem *problem_ptr, const std::string &field_name, RowColData rc, DofMethod &method, int lower_rank, int upper_rank, int verb=DEFAULT_VERBOSITY)=0
Make a loop over dofs.
boost::shared_ptr< SimpleContactProblem::CommonDataSimpleContact > commonDataSimpleContact
Force on edges and lines.
PetscErrorCode DMMoFEMAddSubFieldCol(DM dm, const char field_name[])
boost::shared_ptr< SimpleContactProblem::SimpleContactElement > feRhsSimpleContactALEMaterial
boost::shared_ptr< MetaSpringBC::DataAtIntegrationPtsSprings > commonDataSpringsALE
MoFEMErrorCode resolveSharedBitRefLevel(const BitRefLevel bit, const int verb=QUIET, const bool debug=false)
resole shared entities by bit level
int residualStressBlock
Block on which residual stress is applied.
#define MOFEM_LOG_CHANNEL(channel)
Set and reset channel.
boost::shared_ptr< MortarContactProblem::MortarContactElement > feRhsMortarContact
boost::shared_ptr< CrackFrontElement > feLhs
Integrate elastic FE.
Problem manager is used to build and partition problems.
boost::shared_ptr< boost::ptr_map< string, NeumannForcesSurface > > surfaceForces
assemble surface forces
boost::shared_ptr< NeumannForcesSurface::MyTriangleFE > feMaterialAnaliticalTraction
Surface elment to calculate tractions in material space.
DofIdx getNbDofsCol() const
MoFEMErrorCode buildCrackFrontFieldId(const BitRefLevel bit, const bool build_fields=true, const int verb=QUIET, const bool debug=false)
declare crack surface files
boost::shared_ptr< boost::ptr_map< string, EdgeForce > > edgeForces
assemble edge forces
Implementation of elastic (non-linear) St. Kirchhoff equation.
Operator performs automatic differentiation.
PetscBool fixContactNodes
Range contactBothSidesMasterNodes
MoFEMErrorCode getInterfaceVersion(Version &version) const
#define CHKERRQ_MOAB(a)
check error code of MoAB function
MoFEMErrorCode declareExternalForcesFE(const BitRefLevel bit, const BitRefLevel mask=BitRefLevel().set(), const bool proc_only=true)
virtual MPI_Comm & get_comm() const =0
std::map< std::string, BitRefLevel > mapBitLevel
virtual MoFEMErrorCode modify_finite_element_add_field_row(const std::string &fe_name, const std::string name_row)=0
set field row which finite element use
static MoFEMErrorCode setTags(moab::Interface &moab, Range edges, Range tris, bool number_pathes=true, boost::shared_ptr< SurfaceSlidingConstrains::DriverElementOrientation > surface_orientation=nullptr, MoFEM::Interface *m_field_ptr=nullptr)
boost::shared_ptr< GriffithForceElement::MyTriangleFEConstrains > feGriffithConstrainsRhs
map< int, boost::shared_ptr< SurfaceSlidingConstrains > > surfaceConstrain
structure grouping operators and data used for calculation of nonlinear elastic element
Elastic material data structure.
PetscErrorCode DMMoFEMSetSquareProblem(DM dm, PetscBool square_problem)
set squared problem
boost::shared_ptr< CrackFrontElement > feMaterialLhs
Integrate material stresses, assemble matrix.
DEPRECATED auto createSmartDM(MPI_Comm comm, const std::string dm_type_name)
boost::shared_ptr< FaceElementForcesAndSourcesCore > feRhsSpringALEMaterial
MoFEMErrorCode partitionMesh(BitRefLevel bit1, BitRefLevel bit2, int verb=QUIET, const bool debug=false)
partotion mesh
boost::shared_ptr< SimpleContactProblem::SimpleContactElement > fePostProcSimpleContact
PetscErrorCode MoFEMErrorCode
MoFEM/PETSc error code.
MoFEMErrorCode declareMaterialFE(const BitRefLevel bit, const BitRefLevel mask=BitRefLevel().set(), const bool proc_only=true, const bool verb=QUIET)
declare material finite elements
this struct keeps basic methods for moab meshset about material and boundary conditions
boost::shared_ptr< BothSurfaceConstrains > bothSidesConstrains
boost::shared_ptr< NeumannForcesSurface::DataAtIntegrationPts > commonDataSurfacePressureAle
common data at integration points (ALE)
Get values at integration pts for tensor filed rank 1, i.e. vector field.
#define _IT_CUBITMESHSETS_BY_BCDATA_TYPE_FOR_LOOP_(MESHSET_MANAGER, CUBITBCTYPE, IT)
Iterator that loops over a specific Cubit MeshSet in a moFEM field.
#define _IT_GET_DOFS_FIELD_BY_NAME_AND_ENT_FOR_LOOP_(MFIELD, NAME, ENT, IT)
loop over all dofs from a moFEM field and particular field
MoFEMErrorCode setSingularDofs(const string field_name, const int verb=QUIET)
set singular dofs (on edges adjacent to crack front) from geometry
UBlasMatrix< double > MatrixDouble
Operator for force element.
PetscBool solveEigenStressProblem
Solve eigen problem.
Struct keeps handle to refined handle.
virtual MoFEMErrorCode remove_ents_from_field(const std::string name, const EntityHandle meshset, const EntityType type, int verb=DEFAULT_VERBOSITY)=0
remove entities from field
virtual FieldBitNumber get_field_bit_number(const std::string name) const =0
get field bit number
virtual int get_comm_rank() const =0
double smootherGamma
Controls mesh smoothing.
boost::shared_ptr< GriffithForceElement::MyTriangleFE > feGriffithForceRhs
BitFEId getBitFEId() const
map< EntityHandle, double > mapJ
hashmap of J - release energy at nodes
MoFEMErrorCode createEigenElasticDM(SmartPetscObj< DM > &dm, const std::string prb_name, const BitRefLevel bit, const BitRefLevel mask=BitRefLevel().set())
Create elastic problem DM.
double initialSmootherAlpha
multi_index_container< boost::shared_ptr< FieldEntity >, indexed_by< ordered_unique< tag< Unique_mi_tag >, member< FieldEntity, UId, &FieldEntity::localUId > >, ordered_non_unique< tag< Ent_mi_tag >, const_mem_fun< FieldEntity::interface_type_RefEntity, EntityHandle, &FieldEntity::getEnt > > > > FieldEntity_multiIndex
MultiIndex container keeps FieldEntity.
const boost::scoped_ptr< UnknownInterface > cpSolversPtr
MoFEMErrorCode saveEachPart(const std::string prefix, const Range &ents)
Save entities on ech processor.
PetscErrorCode DMoFEMMeshToLocalVector(DM dm, Vec l, InsertMode mode, ScatterMode scatter_mode)
set local (or ghosted) vector values on mesh for partition only
virtual const FiniteElement_multiIndex * get_finite_elements() const =0
Get the finite elements object.
boost::shared_ptr< MortarContactProblem::MortarContactElement > fePostProcMortarContact
PetscBool contactOutputIntegPts
virtual EntityHandle get_field_meshset(const std::string name) const =0
get field meshset
multi_index_container< boost::shared_ptr< RefEntity >, indexed_by< ordered_unique< tag< Ent_mi_tag >, const_mem_fun< RefEntity, EntityHandle, &RefEntity::getEnt > >, ordered_non_unique< tag< Ent_Ent_mi_tag >, const_mem_fun< RefEntity, EntityHandle, &RefEntity::getParentEnt > >, ordered_non_unique< tag< Composite_EntType_and_ParentEntType_mi_tag >, composite_key< RefEntity, const_mem_fun< RefEntity, EntityType, &RefEntity::getEntType >, const_mem_fun< RefEntity, EntityType, &RefEntity::getParentEntType > > >, ordered_non_unique< tag< Composite_ParentEnt_And_EntType_mi_tag >, composite_key< RefEntity, const_mem_fun< RefEntity, EntityType, &RefEntity::getEntType >, const_mem_fun< RefEntity, EntityHandle, &RefEntity::getParentEnt > > > > > RefEntity_multiIndex
boost::shared_ptr< CrackFrontElement > feEnergy
Integrate energy.
boost::shared_ptr< DirichletSpatialPositionsBc > spatialDirichletBc
apply Dirichlet BC to sparial positions
MoFEMErrorCode buildSurfaceFields(const BitRefLevel bit, const bool proc_only=true, const bool build_fields=true, const int verb=QUIET, const bool debug=false)
build fields with Lagrange multipliers to constrain surfaces
static MoFEMErrorCode propagation_snes_rhs(SNES snes, Vec x, Vec f, void *ctx)
virtual MoFEMErrorCode add_ents_to_field_by_type(const Range &ents, const EntityType type, const std::string &name, int verb=DEFAULT_VERBOSITY)=0
Add entities to field meshset.
PetscErrorCode DMMoFEMAddElement(DM dm, std::string fe_name)
add element to dm
boost::shared_ptr< VolumeLengthQuality< adouble > > volumeLengthAdouble
virtual bool check_problem(const std::string name)=0
check if problem exist
boost::shared_ptr< SimpleContactProblem::SimpleContactElement > feLhsSimpleContactALEMaterial
boost::shared_ptr< ContactSearchKdTree::ContactCommonData_multiIndex > contactSearchMultiIndexPtr
bool onlyHooke
True if only Hooke material is applied.
Range mortarContactElements
PetscBool areSpringsAle
If true surface spring is considered in ALE.
virtual const DofEntity_multiIndex * get_dofs() const =0
Get the dofs object.
DeprecatedCoreInterface Interface
MoFEM::Interface & mField
boost::shared_ptr< NeumannForcesSurface::DataAtIntegrationPts > commonDataSurfaceForceAle
common data at integration points (ALE)
static constexpr int approx_order
#define MOFEM_LOG_FUNCTION()
Set scope.
boost::shared_ptr< boost::ptr_map< string, NeumannForcesSurface > > surfacePressure
assemble surface pressure
MoFEMErrorCode ConstrainMatrixMultOpRT(Mat RT, Vec x, Vec f)
Multiplication operator for RT = (CCT)^-TC.
PetscBool ignoreMaterialForce
If true surface pressure is considered in ALE.
moab::Interface & contactPostProcMoab
MoFEMErrorCode buildBothSidesFieldId(const BitRefLevel bit_spatial, const BitRefLevel bit_material, const bool proc_only=false, const bool build_fields=true, const int verb=QUIET, const bool debug=false)
Lagrange multipliers field which constrains material displacements.
PetscErrorCode DMMoFEMSetDestroyProblem(DM dm, PetscBool destroy_problem)
virtual MoFEMErrorCode add_ents_to_finite_element_by_type(const EntityHandle entities, const EntityType type, const std::string &name, const bool recursive=true)=0
add entities to finite element
static MoFEMErrorCodeGeneric< moab::ErrorCode > rval
FTensor::Index< 'M', 3 > M
PetscErrorCode DMMoFEMAddRowCompositeProblem(DM dm, const char prb_name[])
Add problem to composite DM on row.
#define CHKERR
Inline error check.
static MoFEMErrorCode snes_monitor_fields(SNES snes, PetscInt its, PetscReal fgnorm, PetscViewerAndFormat *vf)
MoFEMErrorCode buildProblemFields(const BitRefLevel &bit1, const BitRefLevel &mask1, const BitRefLevel &bit2, const int verb=QUIET, const bool debug=false)
Build problem fields.
virtual MoFEMErrorCode remove_ents_from_finite_element(const std::string name, const EntityHandle meshset, const EntityType type, int verb=DEFAULT_VERBOSITY)=0
remove entities from given refinement level to finite element database
MoFEMErrorCode updateMaterialFixedNode(const bool fix_front, const bool fix_small_g, const bool debug=false)
Update fixed nodes.
boost::shared_ptr< SimpleContactProblem::SimpleContactElement > feLhsSimpleContactALE
boost::shared_ptr< DirichletFixFieldAtEntitiesBc > fixMaterialEnts
virtual MoFEMErrorCode add_finite_element(const std::string &fe_name, enum MoFEMTypes bh=MF_EXCL, int verb=DEFAULT_VERBOSITY)=0
add finite element
virtual moab::Interface & get_moab()=0
PetscBool isSurfaceForceAle
If true surface pressure is considered in ALE.
static MoFEMErrorCode saveEdges(moab::Interface &moab, std::string name, Range edges, Range *faces=nullptr)
virtual MoFEMErrorCode modify_finite_element_add_field_col(const std::string &fe_name, const std::string name_row)=0
set field col which finite element use
MoFEMErrorCode declareBothSidesFE(const BitRefLevel bit_spatial, const BitRefLevel bit_material, const BitRefLevel mask=BitRefLevel().set(), const bool proc_only=true, const bool verb=QUIET)
MoFEMErrorCode clearLoops()
Clear loops.
Section manager is used to create indexes and sections.
RHS-operator for the surface force element (material configuration)
MoFEMErrorCode setCrackFrontBitLevel(BitRefLevel from_bit, BitRefLevel bit, const int nb_levels=2, const bool debug=false)
Set bit ref level for entities adjacent to crack front.
Operator for force element.
virtual MoFEMErrorCode build_finite_elements(int verb=DEFAULT_VERBOSITY)=0
Build finite elements.
boost::shared_ptr< DofEntity > arcLengthDof
MoFEMErrorCode getEntitiesByDimension(const int ms_id, const unsigned int cubit_bc_type, const int dimension, Range &entities, const bool recursive=true) const
get entities from CUBIT/meshset of a particular entity dimension
Structure used to enforce analytical boundary conditions.
boost::shared_ptr< GriffithForceElement::MyTriangleFE > feGriffithForceLhs
default operator for TRI element
#define MOFEM_LOG_C(channel, severity, format,...)
boost::shared_ptr< BothSurfaceConstrains > bothSidesContactConstrains
boost::shared_ptr< BothSurfaceConstrains > closeCrackConstrains
virtual MoFEMErrorCode add_field(const std::string &name, const FieldSpace space, const FieldApproximationBase base, const FieldCoefficientsNumber nb_of_coefficients, const TagType tag_type=MB_TAG_SPARSE, const enum MoFEMTypes bh=MF_EXCL, int verb=DEFAULT_VERBOSITY)=0
Add field.
PetscErrorCode DMMoFEMCreateSubDM(DM subdm, DM dm, const char problem_name[])
Must be called by user to set Sub DM MoFEM data structures.
double betaGc
heterogeneous Griffith energy exponent
DofIdx getNbDofsRow() const
map< EntityHandle, double > mapG3
hashmap of g3 - release energy at nodes
virtual boost::shared_ptr< BasicEntityData > & get_basic_entity_data_ptr()=0
Get pointer to basic entity data.
MoFEMErrorCode declareElasticFE(const BitRefLevel bit1, const BitRefLevel mask1, const BitRefLevel bit2, const BitRefLevel mask2, const bool add_forces=true, const bool proc_only=true, const int verb=QUIET)
declare elastic finite elements
MoFEMErrorCode declareSurfaceFE(std::string fe_name, const BitRefLevel bit, const BitRefLevel mask, const std::vector< int > &ids, const bool proc_only=true, const int verb=QUIET, const bool debug=false)
declare surface sliding elements
double nBone
Exponent parameter in bone density.
boost::shared_ptr< SimpleContactProblem > contactProblem
@ MAT_ELASTICSET
block name is "MAT_ELASTIC"
#define MOFEM_LOG_SYNCHRONISE(comm)
Synchronise "SYNC" channel.
std::string problemName
problem name
MoFEMErrorCode declareFrontFE(const BitRefLevel bit, const BitRefLevel mask=BitRefLevel().set(), const bool proc_only=true, const bool verb=QUIET)
boost::shared_ptr< Smoother > smootherFe
MoFEMErrorCode createCrackFrontAreaDM(SmartPetscObj< DM > &dm, SmartPetscObj< DM > dm_material, const std::string prb_name, const bool verb=QUIET)
create DM to calculate Griffith energy
double griffithR
Griffith regularisation parameter.
Range contactBothSidesMasterFaces
#define _IT_GET_ENT_FIELD_BY_NAME_FOR_LOOP_(MFIELD, NAME, IT)
loop over all dofs from a moFEM field and particular field
MoFEMErrorCode buildProblemFiniteElements(BitRefLevel bit1, BitRefLevel bit2, std::vector< int > surface_ids, const int verb=QUIET, const bool debug=false)
Build problem finite elements.
PetscErrorCode DMMoFEMGetSnesCtx(DM dm, MoFEM::SnesCtx **snes_ctx)
get MoFEM::SnesCtx data structure
auto createPC(MPI_Comm comm)
MoFEMErrorCode createMaterialForcesDM(SmartPetscObj< DM > &dm, SmartPetscObj< DM > dm_material, const std::string prb_name, const int verb=QUIET)
Create DM for calculation of material forces (sub DM of DM material)
auto & getNumeredRowDofsPtr() const
get access to numeredRowDofsPtr storing DOFs on rows
virtual int get_comm_size() const =0
moab::Core contactPostProcCore
PetscBool addAnalyticalInternalStressOperators
auto & getComposedProblemsData() const
Het composed problems data structure.
DEPRECATED auto smartCreateDMMatrix(DM dm)
virtual const FieldEntity_multiIndex * get_field_ents() const =0
Get the field ents object.
DEPRECATED SmartPetscObj< Vec > smartVectorDuplicate(Vec vec)
boost::shared_ptr< FaceElementForcesAndSourcesCore > feSpringLhsPtr
void temp(int x, int y=10)
boost::shared_ptr< GriffithForceElement > griffithForceElement
MoFEMErrorCode createCrackPropagationDM(SmartPetscObj< DM > &dm, const std::string prb_name, SmartPetscObj< DM > dm_elastic, SmartPetscObj< DM > dm_material, const BitRefLevel bit, const BitRefLevel mask, const std::vector< std::string > fe_list)
Create DM by composition of elastic DM and material DM.
PetscErrorCode DMMoFEMSNESSetJacobian(DM dm, const char fe_name[], MoFEM::FEMethod *method, MoFEM::BasicMethod *pre_only, MoFEM::BasicMethod *post_only)
set SNES Jacobian evaluation function
MoFEMErrorCode createSurfaceProjectionDM(SmartPetscObj< DM > &dm, SmartPetscObj< DM > dm_material, const std::string prb_name, const std::vector< int > surface_ids, const std::vector< std::string > fe_list, const int verb=QUIET)
create DM to calculate projection matrices (sub DM of DM material)
DEPRECATED auto smartCreateDMVector(DM dm)
MoFEMErrorCode addMWLSStressOperators(boost::shared_ptr< CrackFrontElement > &fe_rhs, boost::shared_ptr< CrackFrontElement > &fe_lhs)
virtual MoFEMErrorCode get_field_entities_by_handle(const std::string name, Range &ents) const =0
get entities in the field by handle
MoFEMErrorCode clean_pcomms(moab::Interface &moab, boost::shared_ptr< WrapMPIComm > moab_comm_wrap)
virtual bool check_field(const std::string &name) const =0
check if field is in database
boost::shared_ptr< CrackFrontElement > feCouplingElasticLhs
FE instance to assemble coupling terms.
virtual MoFEMErrorCode delete_ents_by_bit_ref(const BitRefLevel bit, const BitRefLevel mask, const bool remove_parent=false, int verb=DEFAULT_VERBOSITY, MoFEMTypes mf=MF_ZERO)=0
delete entities form mofem and moab database
Shape preserving constrains, i.e. nodes sliding on body surface.
double gC
Griffith energy.
PetscBool printContactState
MoFEMErrorCode declareSimpleContactAleFE(const BitRefLevel bit, const BitRefLevel mask=BitRefLevel().set(), const bool proc_only=true)
Declare FE for pressure BC in ALE formulation (in material domain)
#define MOFEM_LOG_TAG(channel, tag)
Tag channel.
DofIdx getNbLocalDofsRow() const
PetscBool propagateCrack
If true crack propagation is calculated.
MoFEMErrorCode assembleElasticDM(const std::string mwls_stress_tag_name, const int verb=QUIET, const bool debug=false)
create elastic finite element instance for spatial assembly
Range crackFrontNodesTris
constexpr double t
plate stiffness
double crackAccelerationFactor
virtual EntFiniteElement_multiIndex::index< Unique_mi_tag >::type::iterator get_fe_by_name_end(const std::string &fe_name) const =0
get end iterator of finite elements of given name (instead you can use IT_GET_FES_BY_NAME_FOR_LOOP(MF...
PetscErrorCode DMMoFEMCreateMoFEM(DM dm, MoFEM::Interface *m_field_ptr, const char problem_name[], const MoFEM::BitRefLevel bit_level, const MoFEM::BitRefLevel bit_mask=MoFEM::BitRefLevel().set())
Must be called by user to set MoFEM data structures.
Volume finite element base.
FTensor::Index< 'i', SPACE_DIM > i
const boost::scoped_ptr< UnknownInterface > cpMeshCutPtr
boost::shared_ptr< SurfaceSlidingConstrains::DriverElementOrientation > crackOrientation
MoFEMErrorCode declareSmoothingFE(const BitRefLevel bit, const BitRefLevel mask=BitRefLevel().set(), const bool proc_only=true, const bool verb=QUIET)
declare mesh smoothing finite elements
Operator to assemble nodal force into right hand side vector.
boost::shared_ptr< CrackFrontElement > feRhs
Integrate elastic FE.
RHS-operator for the pressure element (material configuration)
Vector manager is used to create vectors \mofem_vectors.
double griffithE
Griffith stability parameter.
boost::shared_ptr< Smoother::MyVolumeFE > feSmootherLhs
Integrate smoothing operators.
static FTensor::Tensor2_symmetric< double, 3 > analyticalStrainFunction(FTensor::Tensor1< FTensor::PackPtr< double *, 1 >, 3 > &t_coords)
EntitiesFieldData::EntData EntData
constexpr auto field_name
virtual MoFEMErrorCode get_finite_element_entities_by_handle(const std::string name, Range &ents) const =0
get entities in the finite element by handle
boost::shared_ptr< SimpleContactProblem::CommonDataSimpleContact > commonDataSimpleContactALE
Get field gradients at integration pts for scalar filed rank 0, i.e. vector field.
virtual const Field_multiIndex * get_fields() const =0
Get the fields object.
double partitioningWeightPower
std::string mwlsEigenStressTagName
Name of tag with eigen stresses.
PetscBool otherSideConstrains
PetscErrorCode SnesRhs(SNES snes, Vec x, Vec f, void *ctx)
This is MoFEM implementation for the right hand side (residual vector) evaluation in SNES solver.
const double v
phase velocity of light in medium (cm/ns)
boost::shared_ptr< ConstrainMatrixCtx > projSurfaceCtx
Data structure to project on the body surface.
#define LOBATTO_PHI0(x)
Definitions taken from Hermes2d code.
boost::shared_ptr< GriffithForceElement::MyTriangleFEConstrainsDelta > feGriffithConstrainsDelta
MoFEMErrorCode ProjectionMatrixMultOpQ(Mat Q, Vec x, Vec f)
Multiplication operator for Q = I-CTC(CCT)^-1C.
const Tensor2_symmetric_Expr< const ddTensor0< T, Dim, i, j >, typename promote< T, double >::V, Dim, i, j > dd(const Tensor0< T * > &a, const Index< i, Dim > index1, const Index< j, Dim > index2, const Tensor1< int, Dim > &d_ijk, const Tensor1< double, Dim > &d_xyz)
static MoFEMErrorCode elastic_snes_rhs(SNES snes, Vec x, Vec f, void *ctx)
map< EntityHandle, VectorDouble3 > mapGriffith
hashmap of Griffith energy at nodes
PetscBool useEigenPositionsSimpleContact
boost::shared_ptr< FaceElementForcesAndSourcesCore > feSpringRhsPtr
#define MOFEM_LOG(channel, severity)
Log.
map< EntityHandle, double > mapG1
hashmap of g1 - release energy at nodes
boost::shared_ptr< WrapMPIComm > moabCommWorld
MoFEMErrorCode createElasticDM(SmartPetscObj< DM > &dm, const std::string prb_name, const BitRefLevel bit, const BitRefLevel mask=BitRefLevel().set())
Create elastic problem DM.
boost::shared_ptr< VolumeLengthQuality< double > > volumeLengthDouble
MoFEMErrorCode setFieldFromCoords(const std::string field_name)
set field from node positions
boost::shared_ptr< FaceElementForcesAndSourcesCore > feLhsSpringALEMaterial
bool setSingularCoordinates
#define _IT_GET_DOFS_FIELD_BY_NAME_FOR_LOOP_(MFIELD, NAME, IT)
boost::shared_ptr< boost::ptr_map< string, NeumannForcesSurface > > surfaceForceAle
assemble surface pressure (ALE)
Range otherSideCrackFaces
map< EntityHandle, VectorDouble3 > mapMatForce
hashmap of material force at nodes
#define _IT_CUBITMESHSETS_BY_SET_TYPE_FOR_LOOP_(MESHSET_MANAGER, CUBITBCTYPE, IT)
Iterator that loops over a specific Cubit MeshSet having a particular BC meshset in a moFEM field.
static MoFEMErrorCode numberSurfaces(moab::Interface &moab, Range edges, Range tris)
boost::shared_ptr< boost::ptr_map< string, NodalForce > > nodalForces
assemble nodal forces
boost::shared_ptr< NonlinearElasticElement > elasticFe
std::string mwlsApproxFile
Name of file with internal stresses.
MoFEMErrorCode buildElasticFields(const BitRefLevel bit, const BitRefLevel mask=BitRefLevel().set(), const bool proc_only=true, const bool build_fields=true, const int verb=QUIET, const bool debug=false)
Declate fields for elastic analysis.
MoFEMErrorCode buildCrackSurfaceFieldId(const BitRefLevel bit, const bool proc_only=true, const bool build_fields=true, const int verb=QUIET, const bool debug=false)
declare crack surface files
multi_index_container< boost::shared_ptr< Field >, indexed_by< hashed_unique< tag< BitFieldId_mi_tag >, const_mem_fun< Field, const BitFieldId &, &Field::getId >, HashBit< BitFieldId >, EqBit< BitFieldId > >, ordered_unique< tag< Meshset_mi_tag >, member< Field, EntityHandle, &Field::meshSet > >, ordered_unique< tag< FieldName_mi_tag >, const_mem_fun< Field, boost::string_ref, &Field::getNameRef > >, ordered_non_unique< tag< BitFieldId_space_mi_tag >, const_mem_fun< Field, FieldSpace, &Field::getSpace > > > > Field_multiIndex
Field_multiIndex for Field.
boost::shared_ptr< SurfaceSlidingConstrains::DriverElementOrientation > contactOrientation
virtual MoFEMErrorCode clear_inactive_dofs(int verb=DEFAULT_VERBOSITY)=0
@ GRIFFITH_CONSTRAINS_TAG
boost::shared_ptr< MortarContactProblem::MortarContactElement > feLhsMortarContact
int getMeshsetId() const
get meshset id as it set in preprocessing software
boost::shared_ptr< CrackFrontElement > feMaterialRhs
Integrate material stresses, assemble vector.
virtual EntFiniteElement_multiIndex::index< Unique_mi_tag >::type::iterator get_fe_by_name_begin(const std::string &fe_name) const =0
get begin iterator of finite elements of given name (instead you can use IT_GET_FES_BY_NAME_FOR_LOOP(...
MoFEMErrorCode declareSpringsAleFE(const BitRefLevel bit, const BitRefLevel mask=BitRefLevel().set(), const bool proc_only=true)
Declare FE for spring BC in ALE formulation (in material domain)
FTensor::Index< 'j', 3 > j
#define BITREFLEVEL_SIZE
max number of refinements
MoFEMErrorCode buildEdgeFields(const BitRefLevel bit, const bool proc_only=true, const bool build_fields=true, const int verb=QUIET, const bool debug=false)
build fields with Lagrange multipliers to constrain edges
map< EntityHandle, double > mapSmoothingForceFactor
std::string mwlsStressTagName
Name of tag with internal stresses.
virtual const RefEntity_multiIndex * get_ref_ents() const =0
Get the ref ents object.
double smootherAlpha
Controls mesh smoothing.
static MoFEMErrorCodeGeneric< PetscErrorCode > ierr
MoFEMErrorCode buildArcLengthField(const BitRefLevel bit, const bool build_fields=true, const int verb=QUIET)
Declate field for arc-length.
@ AINSWORTH_LEGENDRE_BASE
Ainsworth Cole (Legendre) approx. base .
DofIdx nbDofsRow
Global number of DOFs in row.
double rHo0
Reference density if bone is analyzed.
boost::shared_ptr< MortarContactProblem > mortarContactProblemPtr
PetscBool onlyHookeFromOptions
True if only Hooke material is applied.
const FTensor::Tensor2< T, Dim, Dim > Vec
@ MOFEM_DATA_INCONSISTENCY
boost::shared_ptr< SurfaceSlidingConstrains::DriverElementOrientation > skinOrientation
boost::shared_ptr< FEMethod > assembleFlambda
assemble F_lambda vector
MoFEMErrorCode declareArcLengthFE(const BitRefLevel bits, const int verb=QUIET)
create arc-length element entity and declare elemets
Range mortarContactMasterFaces
PetscErrorCode DMMoFEMGetProblemPtr(DM dm, const MoFEM::Problem **problem_ptr)
Get pointer to problem data structure.
Interface for managing meshsets containing materials and boundary conditions.
PetscErrorCode PetscOptionsGetEList(PetscOptions *, const char pre[], const char name[], const char *const *list, PetscInt next, PetscInt *value, PetscBool *set)
const char * materials_list[]
virtual MoFEMErrorCode build_fields(int verb=DEFAULT_VERBOSITY)=0
int postProcLevel
level of postprocessing (amount of output files)
virtual MoFEMErrorCode modify_finite_element_add_field_data(const std::string &fe_name, const std::string name_filed)=0
set finite element field data
boost::shared_ptr< FEMethod > zeroFlambda
assemble F_lambda vector
boost::shared_ptr< SimpleContactProblem::SimpleContactElement > feRhsSimpleContact
MoFEMErrorCode getArcLengthDof()
set pointer to arc-length DOF
boost::shared_ptr< CrackFrontElement > feCouplingMaterialLhs
FE instance to assemble coupling terms.
UBlasVector< double > VectorDouble
DofIdx getNbLocalDofsCol() const
auto & getNumeredColDofsPtr() const
get access to numeredColDofsPtr storing DOFs on cols
multi_index_container< boost::shared_ptr< FiniteElement >, indexed_by< hashed_unique< tag< FiniteElement_Meshset_mi_tag >, member< FiniteElement, EntityHandle, &FiniteElement::meshset > >, hashed_unique< tag< BitFEId_mi_tag >, const_mem_fun< FiniteElement, BitFEId, &FiniteElement::getId >, HashBit< BitFEId >, EqBit< BitFEId > >, ordered_unique< tag< FiniteElement_name_mi_tag >, const_mem_fun< FiniteElement, boost::string_ref, &FiniteElement::getNameRef > > > > FiniteElement_multiIndex
MultiIndex for entities for FiniteElement.
boost::shared_ptr< FaceElementForcesAndSourcesCore > feLhsSpringALE
FTensor::Index< 'm', 3 > m
Kronecker Delta class symmetric.
boost::shared_ptr< boost::ptr_map< string, NeumannForcesSurface > > analiticalSurfaceElement
std::bitset< BITREFLEVEL_SIZE > BitRefLevel
Bit structure attached to each entity identifying to what mesh entity is attached.
@ MOFEM_ATOM_TEST_INVALID
virtual MoFEMErrorCode build_adjacencies(const Range &ents, int verb=DEFAULT_VERBOSITY)=0
build adjacencies
#define MoFEMFunctionBeginHot
First executable line of each MoFEM function, used for error handling. Final line of MoFEM functions ...
boost::shared_ptr< ArcLengthCtx > arcCtx
Interface for nonlinear (SNES) solver.
keeps basic data about problem
virtual bool check_finite_element(const std::string &name) const =0
Check if finite element is in database.
OpMWLSCalculateBaseCoeffcientsAtGaussPtsTmpl< VolumeElementForcesAndSourcesCore > OpMWLSCalculateBaseCoeffcientsAtGaussPts
CrackPropagation(MoFEM::Interface &m_field, const int approx_order=2, const int geometry_order=1)
Constructor of Crack Propagation, prints fracture module version and registers the three interfaces u...
PetscErrorCode DMMoFEMAddSubFieldRow(DM dm, const char field_name[])
boost::shared_ptr< ObosleteUsersModules::TangentWithMeshSmoothingFrontConstrain > tangentConstrains
Constrains crack front in tangent directiona.
Base volume element used to integrate on skeleton.
PetscBool resetMWLSCoeffsEveryPropagationStep
virtual MoFEMErrorCode set_field_order(const EntityHandle meshset, const EntityType type, const std::string &name, const ApproximationOrder order, int verb=DEFAULT_VERBOSITY)=0
Set order approximation of the entities in the field.
MoFEMErrorCode getCubitMeshsetPtr(const int ms_id, const CubitBCType cubit_bc_type, const CubitMeshSets **cubit_meshset_ptr) const
get cubit meshset
multi_index_container< boost::shared_ptr< DofEntity >, indexed_by< ordered_unique< tag< Unique_mi_tag >, const_mem_fun< DofEntity, UId, &DofEntity::getLocalUniqueId > >, ordered_non_unique< tag< Ent_mi_tag >, const_mem_fun< DofEntity, EntityHandle, &DofEntity::getEnt > > > > DofEntity_multiIndex
MultiIndex container keeps DofEntity.
Finite element and operators to apply force/pressures applied to surfaces.
boost::shared_ptr< ArcLengthCtx > arcEigenCtx
structure for projection matrices
Range crackFrontNodesEdges
boost::shared_ptr< SimpleContactProblem::SimpleContactElement > feLhsSimpleContact
PetscErrorCode SnesMat(SNES snes, Vec x, Mat A, Mat B, void *ctx)
This is MoFEM implementation for the left hand side (tangent matrix) evaluation in SNES solver.
MoFEMErrorCode declareEdgeFE(std::string fe_name, const BitRefLevel bit, const BitRefLevel mask, const bool proc_only=true, const int verb=QUIET, const bool debug=false)
bool checkMeshset(const int ms_id, const CubitBCType cubit_bc_type) const
check for CUBIT Id and CUBIT type
MoFEMErrorCode createMaterialDM(SmartPetscObj< DM > &dm, const std::string prb_name, const BitRefLevel bit, const BitRefLevel mask, const std::vector< std::string > fe_list, const bool debug=false)
Create DM fto calculate material problem.
std::string mwlsRhoTagName
Name of tag with density.
EntityHandle arcLengthVertex
Vertex associated with dof for arc-length control.
#define _IT_GET_DOFS_FIELD_BY_NAME_AND_TYPE_FOR_LOOP_(MFIELD, NAME, TYPE, IT)
loop over all dofs from a moFEM field and particular field
PetscErrorCode DMoFEMLoopFiniteElements(DM dm, const char fe_name[], MoFEM::FEMethod *method, CacheTupleWeakPtr cache_ptr=CacheTupleSharedPtr())
Executes FEMethod for finite elements in DM.
#define MoFEMFunctionReturn(a)
Last executable line of each PETSc function used for error handling. Replaces return()
MoFEMErrorCode declareCrackSurfaceFE(std::string fe_name, const BitRefLevel bit, const BitRefLevel mask, const bool proc_only=true, const int verb=QUIET, const bool debug=false)
boost::shared_ptr< NonlinearElasticElement > materialFe
map< int, boost::shared_ptr< EdgeSlidingConstrains > > edgeConstrains
boost::shared_ptr< MWLSApprox > mwlsApprox
multi_index_container< boost::shared_ptr< EntFiniteElement >, indexed_by< ordered_unique< tag< Unique_mi_tag >, const_mem_fun< EntFiniteElement, UId, &EntFiniteElement::getLocalUniqueId > >, ordered_non_unique< tag< Ent_mi_tag >, const_mem_fun< EntFiniteElement::interface_type_RefEntity, EntityHandle, &EntFiniteElement::getEnt > > > > EntFiniteElement_multiIndex
MultiIndex container for EntFiniteElement.
PetscBool isGcHeterogeneous
flag for heterogeneous gc
#define CHKERRG(n)
Check error code of MoFEM/MOAB/PETSc function.
Range mortarContactSlaveFaces
#define MoFEMFunctionBegin
First executable line of each MoFEM function, used for error handling. Final line of MoFEM functions ...
PetscBool doElasticWithoutCrack
FTensor::Index< 'l', 3 > l
MoFEMErrorCode declarePressureAleFE(const BitRefLevel bit, const BitRefLevel mask=BitRefLevel().set(), const bool proc_only=true)
Declare FE for pressure BC in ALE formulation (in material domain)
MoFEMErrorCode resolveShared(const Range &tets, Range &proc_ents, const int verb=QUIET, const bool debug=false)
resolve shared entities
boost::shared_ptr< ConstrainMatrixCtx > projFrontCtx
Data structure to project on crack front.
boost::shared_ptr< boost::ptr_map< string, NeumannForcesSurface > > surfacePressureAle
assemble surface pressure (ALE)
static MoFEMErrorCode elastic_snes_mat(SNES snes, Vec x, Mat A, Mat B, void *ctx)
PetscErrorCode DMMoFEMSetVerbosity(DM dm, const int verb)
Set verbosity level.
NonlinearElasticElement::BlockData BlockData
boost::shared_ptr< MortarContactProblem::CommonDataMortarContact > commonDataMortarContact
@ NOFIELD
scalar or vector of scalars describe (no true field)
PetscErrorCode DMMoFEMSetIsPartitioned(DM dm, PetscBool is_partitioned)
PetscErrorCode DMMoFEMSNESSetFunction(DM dm, const char fe_name[], MoFEM::FEMethod *method, MoFEM::BasicMethod *pre_only, MoFEM::BasicMethod *post_only)
set SNES residual evaluation function
Set Dirichlet boundary conditions on spatial displacements.
static MoFEMErrorCode propagation_snes_mat(SNES snes, Vec x, Mat A, Mat B, void *ctx)
PetscErrorCode PetscOptionsGetBool(PetscOptions *, const char pre[], const char name[], PetscBool *bval, PetscBool *set)
@ BARRIER_AND_CHANGE_QUALITY_SCALED_BY_VOLUME
PetscBool isPressureAle
If true surface pressure is considered in ALE.
boost::shared_ptr< Smoother::MyVolumeFE > feSmootherRhs
Integrate smoothing operators.