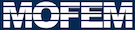 |
| v0.14.0
|
Go to the documentation of this file.
19 #ifndef __CRACK_PROPAGATION_HPP__
20 #define __CRACK_PROPAGATION_HPP__
25 boost::shared_ptr<WrapMPIComm> moab_comm_wrap);
29 const int from_proc,
Range &entities,
30 const bool adjacencies,
const bool tags);
90 version = Version(FM_VERSION_MAJOR, FM_VERSION_MINOR, FM_VERSION_BUILD);
102 UnknownInterface **iface)
const;
192 const int geometry_order = 1);
240 const int verb =
QUIET,
241 const bool debug =
false);
245 const int verb =
QUIET,
246 const bool debug =
false);
264 const bool debug =
false);
286 const int verb =
QUIET,
287 const bool debug =
false);
300 std::vector<int> surface_ids,
301 const int verb =
QUIET,
302 const bool debug =
false);
312 const int verb =
QUIET,
313 const bool debug =
false);
333 SmartPetscObj<DM> &dm_eigen_elastic,
334 SmartPetscObj<DM> &dm_material,
335 SmartPetscObj<DM> &dm_crack_propagation,
336 SmartPetscObj<DM> &dm_material_forces,
337 SmartPetscObj<DM> &dm_surface_projection,
338 SmartPetscObj<DM> &dm_crack_srf_area,
339 std::vector<int> surface_ids,
340 std::vector<std::string> fe_surf_proj_list);
343 const bool debug =
false);
358 const bool proc_only =
true,
const bool build_fields =
true,
359 const int verb =
QUIET,
const bool debug =
false);
367 const bool build_fields =
true,
368 const int verb =
QUIET);
372 const bool proc_only =
true,
373 const bool build_fields =
true,
374 const int verb =
QUIET,
375 const bool debug =
false);
379 const bool proc_only =
true,
380 const bool build_fields =
true,
381 const int verb =
QUIET,
382 const bool debug =
false);
386 const bool proc_only =
true,
387 const bool build_fields =
true,
388 const int verb =
QUIET,
389 const bool debug =
false);
393 const bool build_fields =
true,
394 const int verb =
QUIET,
395 const bool debug =
false);
406 const bool proc_only =
false,
407 const bool build_fields =
true,
408 const int verb =
QUIET,
409 const bool debug =
false);
425 const bool add_forces =
true,
const bool proc_only =
true,
426 const int verb =
QUIET);
430 const int verb =
QUIET);
435 const bool proc_only =
true);
445 const bool proc_only =
true);
455 const bool proc_only =
true);
465 const bool proc_only =
true);
475 const bool proc_only =
true);
480 const bool proc_only =
true,
481 const bool verb =
QUIET);
485 const bool proc_only =
true,
486 const bool verb =
QUIET);
492 const bool proc_only =
true,
const bool verb =
QUIET);
498 const bool proc_only =
true,
const bool verb =
QUIET);
503 const std::vector<int> &ids,
504 const bool proc_only =
true,
505 const int verb =
QUIET,
506 const bool debug =
false);
511 const bool proc_only =
true,
512 const int verb =
QUIET,
513 const bool debug =
false);
517 const BitRefLevel mask,
const bool proc_only =
true,
518 const int verb =
QUIET,
const bool debug =
false);
537 SmartPetscObj<DM> dm_elastic,
540 const std::vector<std::string> fe_list);
550 const std::string prb_name,
590 const std::string prb_name,
592 const std::vector<std::string> fe_list,
593 const bool debug =
false);
605 SmartPetscObj<DM> dm_material,
606 const std::string prb_name,
607 const int verb =
QUIET);
622 SmartPetscObj<DM> &dm, SmartPetscObj<DM> dm_material,
623 const std::string prb_name,
const std::vector<int> surface_ids,
624 const std::vector<std::string> fe_list,
const int verb =
QUIET);
636 SmartPetscObj<DM> dm_material,
637 const std::string prb_name,
638 const bool verb =
QUIET);
655 const int verb =
QUIET,
656 const bool debug =
false);
665 const bool debug =
false);
670 boost::shared_ptr<FEMethod> arc_method = boost::shared_ptr<FEMethod>(),
671 boost::shared_ptr<ArcLengthCtx> arc_ctx =
nullptr,
const int verb =
QUIET,
672 const bool debug =
false);
676 const bool debug =
false);
680 const int verb =
QUIET,
681 const bool debug =
false);
685 const bool debug =
false);
689 const int verb =
QUIET,
690 const bool debug =
false);
694 const bool fix_crack_front,
695 const int verb =
QUIET,
696 const bool debug =
false);
701 boost::shared_ptr<ArcLengthCtx> arc_ctx,
702 const std::vector<int> &surface_ids,
703 const int verb =
QUIET,
704 const bool debug =
false);
716 boost::shared_ptr<CrackFrontElement> &fe_lhs);
720 boost::shared_ptr<CrackFrontElement> &fe_lhs);
724 const bool fix_small_g,
725 const bool debug =
false);
749 const int verb =
QUIET,
750 const bool debug =
false);
764 const int verb =
QUIET,
765 const bool debug =
false);
768 const bool debug =
true);
772 const std::vector<int> &ids,
773 const int verb =
QUIET,
774 const bool debug =
false);
779 const int verb =
QUIET,
780 const bool debug =
false);
794 const int verb =
QUIET,
795 const bool debug =
false);
799 const int verb =
QUIET,
800 const bool debug =
false);
805 const int verb =
QUIET,
806 const bool debug =
false);
810 Vec f_griffith_proj,
Vec f_lambda,
811 const double gc,
const int verb =
QUIET,
812 const bool debug =
true);
829 bool snes_set_up, Mat *shell_m);
854 const int verb =
QUIET,
855 const bool debug =
false);
861 const bool approx_internal_stress);
884 const int verb =
QUIET);
912 inline boost::shared_ptr<FEMethod>
914 boost::shared_ptr<FEMethod> arc_method(
922 inline boost::shared_ptr<AnalyticalDirichletBC::DirichletBC>
968 const std::string lambda_field_name =
"LAMBDA_BOTH_SIDES",
969 const std::string spatial_field_name =
"MESH_NODE_POSITIONS")
973 CHKERRABORT(PETSC_COMM_WORLD,
ierr);
978 ierr = PetscOptionsBegin(PETSC_COMM_WORLD,
"",
979 "Get Booth surface constrains element scaling",
982 CHKERR PetscOptionsScalar(
"-booth_side_alpha",
"scaling parameter",
"",
984 ierr = PetscOptionsEnd();
1019 EntityHandle face = fe_method_ptr->numeredEntFiniteElementPtr->getEnt();
1035 boost::shared_ptr<ArcLengthCtx> &arc_ptr)
1037 MOFEM_LOG(
"CPWorld", Sev::noisy) <<
"ArcLengthSnesCtx create";
1040 MOFEM_LOG(
"CPWorld", Sev::noisy) <<
"ArcLengthSnesCtx destroyed";
1065 boost::shared_ptr<CrackFrontElement>
feLhs;
1066 boost::shared_ptr<CrackFrontElement>
feRhs;
1067 boost::shared_ptr<CrackFrontElement>
1069 boost::shared_ptr<CrackFrontElement>
1072 boost::shared_ptr<ConstrainMatrixCtx>
1074 boost::shared_ptr<ConstrainMatrixCtx>
1076 boost::shared_ptr<MWLSApprox>
1079 boost::shared_ptr<NeumannForcesSurface::MyTriangleFE>
1081 boost::shared_ptr<DirichletSpatialPositionsBc>
1083 boost::shared_ptr<AnalyticalDirichletBC::DirichletBC>
1087 boost::shared_ptr<boost::ptr_map<string, NeumannForcesSurface>>
1089 boost::shared_ptr<boost::ptr_map<string, NeumannForcesSurface>>
1091 boost::shared_ptr<NeumannForcesSurface::DataAtIntegrationPts>
1093 boost::shared_ptr<boost::ptr_map<string, NeumannForcesSurface>>
1095 boost::shared_ptr<boost::ptr_map<string, NeumannForcesSurface>>
1097 boost::shared_ptr<NeumannForcesSurface::DataAtIntegrationPts>
1099 boost::shared_ptr<boost::ptr_map<string, EdgeForce>>
1101 boost::shared_ptr<boost::ptr_map<string, NodalForce>>
1106 boost::shared_ptr<CrackFrontElement>
1108 boost::shared_ptr<CrackFrontElement>
1112 boost::shared_ptr<Smoother::MyVolumeFE>
1114 boost::shared_ptr<Smoother::MyVolumeFE>
1122 boost::shared_ptr<SurfaceSlidingConstrains::DriverElementOrientation>
1124 boost::shared_ptr<SurfaceSlidingConstrains::DriverElementOrientation>
1126 boost::shared_ptr<SurfaceSlidingConstrains::DriverElementOrientation>
1139 boost::shared_ptr<GriffithForceElement::MyTriangleFEConstrainsDelta>
1141 boost::shared_ptr<GriffithForceElement::MyTriangleFEConstrains>
1143 boost::shared_ptr<GriffithForceElement::MyTriangleFEConstrains>
1146 boost::shared_ptr<boost::ptr_map<string, NeumannForcesSurface>>
1190 map<EntityHandle, VectorDouble3>
1192 map<EntityHandle, VectorDouble3>
1249 boost::shared_ptr<ContactSearchKdTree::ContactCommonData_multiIndex>
1261 boost::shared_ptr<FaceElementForcesAndSourcesCore>
1263 boost::shared_ptr<FaceElementForcesAndSourcesCore>
1265 boost::shared_ptr<MetaSpringBC::DataAtIntegrationPtsSprings>
1269 boost::shared_ptr<SimpleContactProblem::SimpleContactElement>
1271 boost::shared_ptr<SimpleContactProblem::SimpleContactElement>
1273 boost::shared_ptr<SimpleContactProblem::CommonDataSimpleContact>
1278 boost::shared_ptr<MortarContactProblem::MortarContactElement>
1280 boost::shared_ptr<MortarContactProblem::MortarContactElement>
1282 boost::shared_ptr<MortarContactProblem::CommonDataMortarContact>
1284 boost::shared_ptr<SimpleContactProblem::SimpleContactElement>
1286 boost::shared_ptr<SimpleContactProblem::SimpleContactElement>
1288 boost::shared_ptr<SimpleContactProblem::SimpleContactElement>
1290 boost::shared_ptr<SimpleContactProblem::CommonDataSimpleContact>
1294 boost::shared_ptr<SimpleContactProblem::SimpleContactElement>
1296 boost::shared_ptr<MortarContactProblem::MortarContactElement>
1336 #endif // __CRACK_PROPAGATION_HPP__
#define MoFEMFunctionReturnHot(a)
Last executable line of each PETSc function used for error handling. Replaces return()
MoFEMErrorCode calculateFrontProjectionMatrix(DM dm_surface, DM dm_project, const int verb=QUIET, const bool debug=false)
assemble crack front projection matrix (that constrains crack area growth)
MoFEMErrorCode cleanSingularElementMatrialPositions()
set maetrial field order to one
boost::shared_ptr< GriffithForceElement::MyTriangleFEConstrains > feGriffithConstrainsLhs
MoFEMErrorCode writeCrackFont(const BitRefLevel &bit, const int step)
MoFEMErrorCode addMaterialFEInstancesToSnes(DM dm, const bool fix_crack_front, const int verb=QUIET, const bool debug=false)
add material elements instances to SNES
MoFEMErrorCode tetsingReleaseEnergyCalculation()
This is run with ctest.
boost::shared_ptr< MWLSApprox > & getMWLSApprox()
MoFEMErrorCode declareSurfaceForceAleFE(const BitRefLevel bit, const BitRefLevel mask=BitRefLevel().set(), const bool proc_only=true)
Declare FE for pressure BC in ALE formulation (in material domain)
MoFEMErrorCode createBcDM(SmartPetscObj< DM > &dm, const std::string prb_name, const BitRefLevel bit, const BitRefLevel mask=BitRefLevel().set())
Create problem to calculate boundary conditions.
MoFEMErrorCode addMWLSDensityOperators(boost::shared_ptr< CrackFrontElement > &fe_rhs, boost::shared_ptr< CrackFrontElement > &fe_lhs)
const std::string spatialFieldName
boost::shared_ptr< AnalyticalDirichletBC::DirichletBC > analyticalDirichletBc
boost::shared_ptr< SimpleContactProblem::CommonDataSimpleContact > commonDataSimpleContact
MoFEMErrorCode getElementOrientation(MoFEM::Interface &m_field, const FEMethod *fe_method_ptr)
boost::shared_ptr< SimpleContactProblem::SimpleContactElement > feRhsSimpleContactALEMaterial
boost::shared_ptr< MetaSpringBC::DataAtIntegrationPtsSprings > commonDataSpringsALE
static bool parallelReadAndBroadcast
MoFEMErrorCode resolveSharedBitRefLevel(const BitRefLevel bit, const int verb=QUIET, const bool debug=false)
resole shared entities by bit level
MoFEMErrorCode calculateSmoothingForcesDM(DM dm, Vec q, Vec f, const int verb=QUIET, const bool debug=false)
assemble smoothing forces, by running material finite element instance
MoFEMErrorCode calculateSurfaceProjectionMatrix(DM dm_front, DM dm_project, const std::vector< int > &ids, const int verb=QUIET, const bool debug=false)
assemble projection matrices
int residualStressBlock
Block on which residual stress is applied.
boost::shared_ptr< MortarContactProblem::MortarContactElement > feRhsMortarContact
FaceOrientation(bool crack_front, Range contact_faces, BitRefLevel bit_level)
boost::shared_ptr< CrackFrontElement > feLhs
Integrate elastic FE.
boost::shared_ptr< boost::ptr_map< string, NeumannForcesSurface > > surfaceForces
assemble surface forces
boost::shared_ptr< NeumannForcesSurface::MyTriangleFE > feMaterialAnaliticalTraction
Surface elment to calculate tractions in material space.
MoFEMErrorCode buildCrackFrontFieldId(const BitRefLevel bit, const bool build_fields=true, const int verb=QUIET, const bool debug=false)
declare crack surface files
boost::shared_ptr< boost::ptr_map< string, EdgeForce > > edgeForces
assemble edge forces
MoFEMErrorCode preProcess()
function is run at the beginning of loop
PetscBool fixContactNodes
Range contactBothSidesMasterNodes
MoFEMErrorCode getInterfaceVersion(Version &version) const
MoFEMErrorCode zeroLambdaFields()
Zero fields with lagrange multipliers.
BitRefLevel defaultBitLevel
MoFEMErrorCode declareExternalForcesFE(const BitRefLevel bit, const BitRefLevel mask=BitRefLevel().set(), const bool proc_only=true)
std::map< std::string, BitRefLevel > mapBitLevel
Store variables for ArcLength analysis.
boost::shared_ptr< GriffithForceElement::MyTriangleFEConstrains > feGriffithConstrainsRhs
map< int, boost::shared_ptr< SurfaceSlidingConstrains > > surfaceConstrain
int getNbCutSteps() const
boost::shared_ptr< CrackFrontElement > feMaterialLhs
Integrate material stresses, assemble matrix.
boost::shared_ptr< FaceElementForcesAndSourcesCore > feRhsSpringALEMaterial
MoFEMErrorCode partitionMesh(BitRefLevel bit1, BitRefLevel bit2, int verb=QUIET, const bool debug=false)
partotion mesh
boost::shared_ptr< SimpleContactProblem::SimpleContactElement > fePostProcSimpleContact
PetscErrorCode MoFEMErrorCode
MoFEM/PETSc error code.
MoFEMErrorCode declareMaterialFE(const BitRefLevel bit, const BitRefLevel mask=BitRefLevel().set(), const bool proc_only=true, const bool verb=QUIET)
declare material finite elements
boost::shared_ptr< BothSurfaceConstrains > bothSidesConstrains
boost::shared_ptr< NeumannForcesSurface::DataAtIntegrationPts > commonDataSurfacePressureAle
common data at integration points (ALE)
MoFEMErrorCode setSingularDofs(const string field_name, const int verb=QUIET)
set singular dofs (on edges adjacent to crack front) from geometry
PetscBool solveEigenStressProblem
Solve eigen problem.
MoFEMErrorCode postProcess()
boost::shared_ptr< ArcLengthCtx > & getArcCtx()
MoFEMErrorCode broadcast_entities(moab::Interface &moab, moab::Interface &moab_tmp, const int from_proc, Range &entities, const bool adjacencies, const bool tags)
MoFEMErrorCode assembleCouplingForcesDM(DM dm, const int verb=QUIET, const bool debug=false)
assemble coupling element instances
double smootherGamma
Controls mesh smoothing.
const std::string lambdaFieldName
boost::shared_ptr< GriffithForceElement::MyTriangleFE > feGriffithForceRhs
map< EntityHandle, double > mapJ
hashmap of J - release energy at nodes
MoFEMErrorCode createEigenElasticDM(SmartPetscObj< DM > &dm, const std::string prb_name, const BitRefLevel bit, const BitRefLevel mask=BitRefLevel().set())
Create elastic problem DM.
double initialSmootherAlpha
const boost::scoped_ptr< UnknownInterface > cpSolversPtr
MoFEMErrorCode saveEachPart(const std::string prefix, const Range &ents)
Save entities on ech processor.
virtual ~FaceOrientation()
MoFEMErrorCode addPropagationFEInstancesToSnes(DM dm, boost::shared_ptr< FEMethod > arc_method, boost::shared_ptr< ArcLengthCtx > arc_ctx, const std::vector< int > &surface_ids, const int verb=QUIET, const bool debug=false)
add finite element to SNES for crack propagation problem
boost::shared_ptr< MortarContactProblem::MortarContactElement > fePostProcMortarContact
boost::shared_ptr< ArcLengthCtx > & getEigenArcCtx()
MoFEMErrorCode setSpatialPositionFromCoords()
set spatial field from nodes
PetscBool contactOutputIntegPts
Range contactMeshsetFaces
Data structure to exchange data between mofem and User Loop Methods on entities.
tangent_tests
Names of tangent matrices tests.
boost::shared_ptr< CrackFrontElement > feEnergy
Integrate energy.
boost::shared_ptr< DirichletSpatialPositionsBc > spatialDirichletBc
apply Dirichlet BC to sparial positions
MoFEMErrorCode buildSurfaceFields(const BitRefLevel bit, const bool proc_only=true, const bool build_fields=true, const int verb=QUIET, const bool debug=false)
build fields with Lagrange multipliers to constrain surfaces
MoFEMErrorCode calculateGriffithForce(DM dm, const double gc, Vec f_griffith, const int verb=QUIET, const bool debug=false)
calculate Griffith (driving) force
boost::shared_ptr< VolumeLengthQuality< adouble > > volumeLengthAdouble
EntityHandle meshsetFaces
boost::shared_ptr< SimpleContactProblem::SimpleContactElement > feLhsSimpleContactALEMaterial
Deprecated interface functions.
boost::shared_ptr< ContactSearchKdTree::ContactCommonData_multiIndex > contactSearchMultiIndexPtr
bool onlyHooke
True if only Hooke material is applied.
bool isStressTagSavedOnNodes
Range mortarContactElements
PetscBool areSpringsAle
If true surface spring is considered in ALE.
DeprecatedCoreInterface Interface
MoFEM::Interface & mField
boost::shared_ptr< NeumannForcesSurface::DataAtIntegrationPts > commonDataSurfaceForceAle
common data at integration points (ALE)
MoFEMErrorCode solvePropagationDM(DM dm, DM dm_elastic, SNES snes, Mat m, Vec q, Vec f)
solve crack propagation problem
static constexpr int approx_order
virtual ~ArcLengthSnesCtx()
BothSurfaceConstrains(MoFEM::Interface &m_field, const std::string lambda_field_name="LAMBDA_BOTH_SIDES", const std::string spatial_field_name="MESH_NODE_POSITIONS")
Construct a new Both Surface Constrains object.
MoFEMErrorCode setSingularElementMatrialPositions(const int verb=QUIET)
set singular dofs of material field (on edges adjacent to crack front) from geometry
boost::shared_ptr< boost::ptr_map< string, NeumannForcesSurface > > surfacePressure
assemble surface pressure
PetscBool ignoreMaterialForce
If true surface pressure is considered in ALE.
moab::Interface & contactPostProcMoab
MoFEMErrorCode buildBothSidesFieldId(const BitRefLevel bit_spatial, const BitRefLevel bit_material, const bool proc_only=false, const bool build_fields=true, const int verb=QUIET, const bool debug=false)
Lagrange multipliers field which constrains material displacements.
operator to post-process results on crack front nodes
MoFEMErrorCode getOptions()
MoFEMErrorCode setMaterialPositionFromCoords()
set material field from nodes
#define CHKERR
Inline error check.
MoFEMErrorCode calculateMaterialForcesDM(DM dm, Vec q, Vec f, const int verb=QUIET, const bool debug=false)
assemble material forces, by running material finite element instance
MoFEMErrorCode buildProblemFields(const BitRefLevel &bit1, const BitRefLevel &mask1, const BitRefLevel &bit2, const int verb=QUIET, const bool debug=false)
Build problem fields.
MoFEMErrorCode updateMaterialFixedNode(const bool fix_front, const bool fix_small_g, const bool debug=false)
Update fixed nodes.
boost::shared_ptr< SimpleContactProblem::SimpleContactElement > feLhsSimpleContactALE
boost::shared_ptr< DirichletFixFieldAtEntitiesBc > fixMaterialEnts
PetscBool isSurfaceForceAle
If true surface pressure is considered in ALE.
MoFEMErrorCode declareBothSidesFE(const BitRefLevel bit_spatial, const BitRefLevel bit_material, const BitRefLevel mask=BitRefLevel().set(), const bool proc_only=true, const bool verb=QUIET)
MoFEMErrorCode setCrackFrontBitLevel(BitRefLevel from_bit, BitRefLevel bit, const int nb_levels=2, const bool debug=false)
Set bit ref level for entities adjacent to crack front.
MoFEMErrorCode calculateSmoothingForceFactor(const int verb=QUIET, const bool debug=true)
boost::shared_ptr< DofEntity > arcLengthDof
boost::shared_ptr< GriffithForceElement::MyTriangleFE > feGriffithForceLhs
Range contactBothSidesSlaveFaces
bool useProjectionFromCrackFront
boost::shared_ptr< BothSurfaceConstrains > bothSidesContactConstrains
boost::shared_ptr< BothSurfaceConstrains > closeCrackConstrains
double betaGc
heterogeneous Griffith energy exponent
map< EntityHandle, double > mapG3
hashmap of g3 - release energy at nodes
MoFEMErrorCode declareElasticFE(const BitRefLevel bit1, const BitRefLevel mask1, const BitRefLevel bit2, const BitRefLevel mask2, const bool add_forces=true, const bool proc_only=true, const int verb=QUIET)
declare elastic finite elements
MoFEMErrorCode declareSurfaceFE(std::string fe_name, const BitRefLevel bit, const BitRefLevel mask, const std::vector< int > &ids, const bool proc_only=true, const int verb=QUIET, const bool debug=false)
declare surface sliding elements
double nBone
Exponent parameter in bone density.
boost::shared_ptr< SimpleContactProblem > contactProblem
MoFEMErrorCode declareFrontFE(const BitRefLevel bit, const BitRefLevel mask=BitRefLevel().set(), const bool proc_only=true, const bool verb=QUIET)
MoFEMErrorCode assembleSmootherForcesDM(DM dm, const std::vector< int > ids, const int verb=QUIET, const bool debug=false)
create smoothing element instance
boost::shared_ptr< Smoother > smootherFe
MoFEMErrorCode createCrackFrontAreaDM(SmartPetscObj< DM > &dm, SmartPetscObj< DM > dm_material, const std::string prb_name, const bool verb=QUIET)
create DM to calculate Griffith energy
double griffithR
Griffith regularisation parameter.
Range contactBothSidesMasterFaces
MoFEMErrorCode buildProblemFiniteElements(BitRefLevel bit1, BitRefLevel bit2, std::vector< int > surface_ids, const int verb=QUIET, const bool debug=false)
Build problem finite elements.
MoFEMErrorCode addSmoothingFEInstancesToSnes(DM dm, const bool fix_crack_front, const int verb=QUIET, const bool debug=false)
add softening elements instances to SNES
MoFEMErrorCode createMaterialForcesDM(SmartPetscObj< DM > &dm, SmartPetscObj< DM > dm_material, const std::string prb_name, const int verb=QUIET)
Create DM for calculation of material forces (sub DM of DM material)
moab::Core contactPostProcCore
PetscBool addAnalyticalInternalStressOperators
boost::shared_ptr< FEMethod > getFrontArcLengthControl(boost::shared_ptr< ArcLengthCtx > arc_ctx)
MoFEMErrorCode readMedFile()
read mesh file
boost::shared_ptr< FaceElementForcesAndSourcesCore > feSpringLhsPtr
AdolcTags
Tapes numbers used by ADOL-C.
boost::shared_ptr< GriffithForceElement > griffithForceElement
MoFEMErrorCode createCrackPropagationDM(SmartPetscObj< DM > &dm, const std::string prb_name, SmartPetscObj< DM > dm_elastic, SmartPetscObj< DM > dm_material, const BitRefLevel bit, const BitRefLevel mask, const std::vector< std::string > fe_list)
Create DM by composition of elastic DM and material DM.
MoFEMErrorCode createSurfaceProjectionDM(SmartPetscObj< DM > &dm, SmartPetscObj< DM > dm_material, const std::string prb_name, const std::vector< int > surface_ids, const std::vector< std::string > fe_list, const int verb=QUIET)
create DM to calculate projection matrices (sub DM of DM material)
MoFEMErrorCode query_interface(boost::typeindex::type_index type_index, UnknownInterface **iface) const
Getting interface of core database.
MoFEMErrorCode addMWLSStressOperators(boost::shared_ptr< CrackFrontElement > &fe_rhs, boost::shared_ptr< CrackFrontElement > &fe_lhs)
MoFEMErrorCode clean_pcomms(moab::Interface &moab, boost::shared_ptr< WrapMPIComm > moab_comm_wrap)
Range constrainedInterface
Range of faces on the constrained interface.
boost::shared_ptr< CrackFrontElement > feCouplingElasticLhs
FE instance to assemble coupling terms.
double gC
Griffith energy.
PetscBool printContactState
MoFEMErrorCode declareSimpleContactAleFE(const BitRefLevel bit, const BitRefLevel mask=BitRefLevel().set(), const bool proc_only=true)
Declare FE for pressure BC in ALE formulation (in material domain)
PetscBool propagateCrack
If true crack propagation is calculated.
MoFEMErrorCode getElementOrientation(MoFEM::Interface &m_field, const EntityHandle face)
MoFEMErrorCode assembleElasticDM(const std::string mwls_stress_tag_name, const int verb=QUIET, const bool debug=false)
create elastic finite element instance for spatial assembly
MoFEMErrorCode calculateElasticEnergy(DM dm, const std::string msg="")
assemble elastic part of matrix, by running elastic finite element instance
Range crackFrontNodesTris
MoFEM::Interface & mField
! Node on which lagrange multiplier is set
double crackAccelerationFactor
MoFEMErrorCode postProcessDM(DM dm, const int step, const std::string fe_name, const bool approx_internal_stress)
post-process results for elastic solution
ArcLengthSnesCtx(MoFEM::Interface &m_field, const std::string problem_name, boost::shared_ptr< ArcLengthCtx > &arc_ptr)
const boost::scoped_ptr< UnknownInterface > cpMeshCutPtr
MoFEMErrorCode deleteEntities(const int verb=QUIET, const bool debug=false)
boost::shared_ptr< SurfaceSlidingConstrains::DriverElementOrientation > crackOrientation
MoFEMErrorCode declareSmoothingFE(const BitRefLevel bit, const BitRefLevel mask=BitRefLevel().set(), const bool proc_only=true, const bool verb=QUIET)
declare mesh smoothing finite elements
boost::shared_ptr< CrackFrontElement > feRhs
Integrate elastic FE.
double griffithE
Griffith stability parameter.
boost::shared_ptr< Smoother::MyVolumeFE > feSmootherLhs
Integrate smoothing operators.
static FTensor::Tensor2_symmetric< double, 3 > analyticalStrainFunction(FTensor::Tensor1< FTensor::PackPtr< double *, 1 >, 3 > &t_coords)
constexpr auto field_name
void setVecDiagM(Vec diag_m)
MoFEMErrorCode calculateReleaseEnergy(DM dm, Vec f_material_proj, Vec f_griffith_proj, Vec f_lambda, const double gc, const int verb=QUIET, const bool debug=true)
calculate release energy
boost::shared_ptr< SimpleContactProblem::CommonDataSimpleContact > commonDataSimpleContactALE
boost::shared_ptr< FaceElementForcesAndSourcesCore > feLhsSurfaceForceALEMaterial
double partitioningWeightPower
std::string mwlsEigenStressTagName
Name of tag with eigen stresses.
base class for all interface classes
PetscBool otherSideConstrains
MoFEMErrorCode solveElasticDM(DM dm, SNES snes, Mat m, Vec q, Vec f, bool snes_set_up, Mat *shell_m)
solve elastic problem
MoFEMErrorCode savePositionsOnCrackFrontDM(DM dm, Vec q, const int verb=QUIET, const bool debug=false)
boost::shared_ptr< ConstrainMatrixCtx > projSurfaceCtx
Data structure to project on the body surface.
boost::shared_ptr< GriffithForceElement::MyTriangleFEConstrainsDelta > feGriffithConstrainsDelta
map< EntityHandle, VectorDouble3 > mapGriffith
hashmap of Griffith energy at nodes
boost::shared_ptr< FaceElementForcesAndSourcesCore > feSpringRhsPtr
PetscBool useEigenPositionsSimpleContact
#define MOFEM_LOG(channel, severity)
Log.
map< EntityHandle, double > mapG1
hashmap of g1 - release energy at nodes
boost::shared_ptr< WrapMPIComm > moabCommWorld
MoFEMErrorCode createElasticDM(SmartPetscObj< DM > &dm, const std::string prb_name, const BitRefLevel bit, const BitRefLevel mask=BitRefLevel().set())
Create elastic problem DM.
boost::shared_ptr< VolumeLengthQuality< double > > volumeLengthDouble
MoFEMErrorCode setFieldFromCoords(const std::string field_name)
set field from node positions
boost::shared_ptr< FaceElementForcesAndSourcesCore > feLhsSpringALEMaterial
bool setSingularCoordinates
boost::shared_ptr< boost::ptr_map< string, NeumannForcesSurface > > surfaceForceAle
assemble surface pressure (ALE)
Range otherSideCrackFaces
map< EntityHandle, VectorDouble3 > mapMatForce
hashmap of material force at nodes
MoFEMErrorCode operator()()
boost::shared_ptr< boost::ptr_map< string, NodalForce > > nodalForces
assemble nodal forces
MoFEMErrorCode projectMaterialForcesDM(DM dm_project, Vec f, Vec f_proj, const int verb=QUIET, const bool debug=false)
project material forces along the crack elongation direction
PostProcVertexMethod(MoFEM::Interface &m_field, Vec f, std::string tag_name)
boost::shared_ptr< NonlinearElasticElement > elasticFe
std::string mwlsApproxFile
Name of file with internal stresses.
MoFEMErrorCode buildElasticFields(const BitRefLevel bit, const BitRefLevel mask=BitRefLevel().set(), const bool proc_only=true, const bool build_fields=true, const int verb=QUIET, const bool debug=false)
Declate fields for elastic analysis.
MoFEMErrorCode buildCrackSurfaceFieldId(const BitRefLevel bit, const bool proc_only=true, const bool build_fields=true, const int verb=QUIET, const bool debug=false)
declare crack surface files
double getLoadScale() const
boost::shared_ptr< SurfaceSlidingConstrains::DriverElementOrientation > contactOrientation
@ GRIFFITH_CONSTRAINS_TAG
boost::shared_ptr< MortarContactProblem::MortarContactElement > feLhsMortarContact
boost::shared_ptr< CrackFrontElement > feMaterialRhs
Integrate material stresses, assemble vector.
MoFEMErrorCode declareSpringsAleFE(const BitRefLevel bit, const BitRefLevel mask=BitRefLevel().set(), const bool proc_only=true)
Declare FE for spring BC in ALE formulation (in material domain)
MoFEMErrorCode testJacobians(const BitRefLevel bit, const BitRefLevel mask, tangent_tests test)
test LHS Jacobians
MoFEMErrorCode buildEdgeFields(const BitRefLevel bit, const bool proc_only=true, const bool build_fields=true, const int verb=QUIET, const bool debug=false)
build fields with Lagrange multipliers to constrain edges
map< EntityHandle, double > mapSmoothingForceFactor
std::string mwlsStressTagName
Name of tag with internal stresses.
MoFEMErrorCode assembleMaterialForcesDM(DM dm, const int verb=QUIET, const bool debug=false)
create material element instance
Constrains material displacement on both sides.
double smootherAlpha
Controls mesh smoothing.
static MoFEMErrorCodeGeneric< PetscErrorCode > ierr
MoFEMErrorCode buildArcLengthField(const BitRefLevel bit, const bool build_fields=true, const int verb=QUIET)
Declate field for arc-length.
double rHo0
Reference density if bone is analyzed.
boost::shared_ptr< MortarContactProblem > mortarContactProblemPtr
PetscBool onlyHookeFromOptions
True if only Hooke material is applied.
const FTensor::Tensor2< T, Dim, Dim > Vec
boost::shared_ptr< SurfaceSlidingConstrains::DriverElementOrientation > skinOrientation
boost::shared_ptr< FEMethod > assembleFlambda
assemble F_lambda vector
MoFEMErrorCode declareArcLengthFE(const BitRefLevel bits, const int verb=QUIET)
create arc-length element entity and declare elemets
Range mortarContactMasterFaces
virtual ~CrackPropagation()
int postProcLevel
level of postprocessing (amount of output files)
boost::shared_ptr< FEMethod > zeroFlambda
assemble F_lambda vector
boost::shared_ptr< SimpleContactProblem::SimpleContactElement > feRhsSimpleContact
MoFEMErrorCode getArcLengthDof()
set pointer to arc-length DOF
boost::shared_ptr< FaceElementForcesAndSourcesCore > feRhsSurfaceForceALEMaterial
MoFEMErrorCode getElementOrientation(MoFEM::Interface &m_field, const EntityHandle face, const BitRefLevel bit)
boost::shared_ptr< CrackFrontElement > feCouplingMaterialLhs
FE instance to assemble coupling terms.
boost::shared_ptr< FaceElementForcesAndSourcesCore > feLhsSpringALE
Determine face orientation.
MoFEM::Interface & mField
FTensor::Index< 'm', 3 > m
MoFEMErrorCode preProcess()
MoFEMErrorCode operator()()
function is run for every finite element
boost::shared_ptr< boost::ptr_map< string, NeumannForcesSurface > > analiticalSurfaceElement
std::bitset< BITREFLEVEL_SIZE > BitRefLevel
Bit structure attached to each entity identifying to what mesh entity is attached.
#define MoFEMFunctionBeginHot
First executable line of each MoFEM function, used for error handling. Final line of MoFEM functions ...
boost::shared_ptr< ArcLengthCtx > arcCtx
MoFEMErrorCode projectGriffithForce(DM dm, Vec f_griffith, Vec f_griffith_proj, const int verb=QUIET, const bool debug=false)
project Griffith forces
CrackPropagation(MoFEM::Interface &m_field, const int approx_order=2, const int geometry_order=1)
Constructor of Crack Propagation, prints fracture module version and registers the three interfaces u...
boost::shared_ptr< ObosleteUsersModules::TangentWithMeshSmoothingFrontConstrain > tangentConstrains
Constrains crack front in tangent directiona.
PetscBool resetMWLSCoeffsEveryPropagationStep
@ EXTERIOR_DERIVATIVE_TAG
boost::shared_ptr< ArcLengthCtx > arcEigenCtx
Range crackFrontNodesEdges
double fractionOfFixedNodes
boost::shared_ptr< SimpleContactProblem::SimpleContactElement > feLhsSimpleContact
MoFEMErrorCode createDMs(SmartPetscObj< DM > &dm_elastic, SmartPetscObj< DM > &dm_eigen_elastic, SmartPetscObj< DM > &dm_material, SmartPetscObj< DM > &dm_crack_propagation, SmartPetscObj< DM > &dm_material_forces, SmartPetscObj< DM > &dm_surface_projection, SmartPetscObj< DM > &dm_crack_srf_area, std::vector< int > surface_ids, std::vector< std::string > fe_surf_proj_list)
Crate DMs for all problems and sub problems.
MoFEMErrorCode declareEdgeFE(std::string fe_name, const BitRefLevel bit, const BitRefLevel mask, const bool proc_only=true, const int verb=QUIET, const bool debug=false)
MoFEMErrorCode createMaterialDM(SmartPetscObj< DM > &dm, const std::string prb_name, const BitRefLevel bit, const BitRefLevel mask, const std::vector< std::string > fe_list, const bool debug=false)
Create DM fto calculate material problem.
std::string mwlsRhoTagName
Name of tag with density.
EntityHandle arcLengthVertex
Vertex associated with dof for arc-length control.
MoFEMErrorCode setCoordsFromField(const std::string field_name="MESH_NODE_POSITIONS")
set coordinates from field
#define MoFEMFunctionReturn(a)
Last executable line of each PETSc function used for error handling. Replaces return()
MoFEMErrorCode declareCrackSurfaceFE(std::string fe_name, const BitRefLevel bit, const BitRefLevel mask, const bool proc_only=true, const int verb=QUIET, const bool debug=false)
boost::shared_ptr< NonlinearElasticElement > materialFe
MoFEMErrorCode unsetSingularElementMatrialPositions()
remove singularity
map< int, boost::shared_ptr< EdgeSlidingConstrains > > edgeConstrains
MoFEMErrorCode addElasticFEInstancesToSnes(DM dm, Mat m, Vec q, Vec f, boost::shared_ptr< FEMethod > arc_method=boost::shared_ptr< FEMethod >(), boost::shared_ptr< ArcLengthCtx > arc_ctx=nullptr, const int verb=QUIET, const bool debug=false)
boost::shared_ptr< MWLSApprox > mwlsApprox
PetscBool isGcHeterogeneous
flag for heterogeneous gc
MoFEMErrorCode getOptions()
Get options form command line.
MoFEMErrorCode createProblemDataStructures(const std::vector< int > surface_ids, const int verb=QUIET, const bool debug=false)
Construct problem data structures.
Range mortarContactSlaveFaces
Class implemented by user to detect face orientation.
#define MoFEMFunctionBegin
First executable line of each MoFEM function, used for error handling. Final line of MoFEM functions ...
Range masterNodes
! Scaling parameter
PetscBool doElasticWithoutCrack
MoFEMErrorCode declarePressureAleFE(const BitRefLevel bit, const BitRefLevel mask=BitRefLevel().set(), const bool proc_only=true)
Declare FE for pressure BC in ALE formulation (in material domain)
MoFEMErrorCode resolveShared(const Range &tets, Range &proc_ents, const int verb=QUIET, const bool debug=false)
resolve shared entities
boost::shared_ptr< ConstrainMatrixCtx > projFrontCtx
Data structure to project on crack front.
boost::shared_ptr< boost::ptr_map< string, NeumannForcesSurface > > surfacePressureAle
assemble surface pressure (ALE)
boost::shared_ptr< MortarContactProblem::CommonDataMortarContact > commonDataMortarContact
MoFEMErrorCode postProcess()
function is run at the end of loop
int getNbLoadSteps() const
PetscBool isPressureAle
If true surface pressure is considered in ALE.
boost::shared_ptr< AnalyticalDirichletBC::DirichletBC > getAnalyticalDirichletBc()
boost::shared_ptr< Smoother::MyVolumeFE > feSmootherRhs
Integrate smoothing operators.