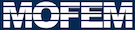 |
| v0.14.0
|
Go to the documentation of this file.
9 #ifndef __COMMINTERFACE_HPP__
10 #define __COMMINTERFACE_HPP__
63 const std::string &fe_name,
90 const std::string &fe_name,
107 const int num_entities,
108 const int owner_proc = 0,
122 const int owner_proc = 0,
136 const int owner_proc = 0,
182 std::map<int, Range> *received_ents,
245 const int adj_dim,
const int n_parts,
246 Tag *th_vertex_weights =
nullptr,
247 Tag *th_edge_weights =
nullptr,
248 Tag *th_part_weights =
nullptr,
257 #endif //__COMMINTERFACE_HPP__
~CommInterface()=default
Destructor.
PetscErrorCode MoFEMErrorCode
MoFEM/PETSc error code.
MoFEMErrorCode partitionMesh(const Range &ents, const int dim, const int adj_dim, const int n_parts, Tag *th_vertex_weights=nullptr, Tag *th_edge_weights=nullptr, Tag *th_part_weights=nullptr, int verb=VERBOSE, const bool debug=false)
Set partition tag to each finite element in the problem.
MoFEMErrorCode resolveParentEntities(const Range &ent, int verb=DEFAULT_VERBOSITY)
Synchronise parent entities.
MoFEMErrorCode makeFieldEntitiesMultishared(const std::string field_name, const int owner_proc=0, int verb=DEFAULT_VERBOSITY)
make field entities multi shared
implementation of Data Operators for Forces and Sources
MoFEMErrorCode query_interface(boost::typeindex::type_index type_index, UnknownInterface **iface) const
MoFEMErrorCode resolveSharedFiniteElements(const Problem *problem_ptr, const std::string &fe_name, int verb=DEFAULT_VERBOSITY)
resolve shared entities for finite elements in the problem
MoFEMErrorCode synchroniseFieldEntities(const std::string name, int verb=DEFAULT_VERBOSITY)
constexpr auto field_name
MoFEMErrorCode synchroniseEntities(Range &ent, std::map< int, Range > *received_ents, int verb=DEFAULT_VERBOSITY)
synchronize entity range on processors (collective)
base class for all interface classes
CommInterface(const MoFEM::Core &core)
MoFEMErrorCode makeEntitiesMultishared(const EntityHandle *entities, const int num_entities, const int owner_proc=0, int verb=DEFAULT_VERBOSITY)
make entities from proc 0 shared on all proc
keeps basic data about problem
MoFEMErrorCode exchangeFieldData(const std::string field_name, int verb=DEFAULT_VERBOSITY)
Exchange field data.