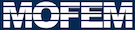 |
| v0.14.0
|
Go to the documentation of this file.
19 #ifndef __SIMPLE_CONTACT__
20 #define __SIMPLE_CONTACT__
29 using ContactEle = ContactPrismElementForcesAndSourcesCore;
46 static inline double Sign(
double x);
48 static inline bool State(
const double cn,
const double g,
const double l);
50 static inline bool StateALM(
const double cn,
const double g,
const double l);
61 static constexpr
double TOL = 1e-8;
71 map<int, SimpleContactPrismsData>
111 " Active Gauss pts: %d out of %d", (
int)array[0],
138 :
public boost::enable_shared_from_this<ConvectSlaveIntegrationPts> {
141 string spat_pos,
string mat_pos)
148 return boost::shared_ptr<MatrixDouble>(shared_from_this(),
153 return boost::shared_ptr<MatrixDouble>(shared_from_this(), &
diffKsiSlave);
184 string mat_pos,
bool newton_cotes =
false)
232 const string element_name,
const string field_name,
233 const string lagrange_field_name,
Range &range_slave_master_prisms,
234 bool eigen_pos_flag =
false,
235 const string eigen_node_field_name =
"EIGEN_SPATIAL_POSITIONS") {
240 if (range_slave_master_prisms.size() > 0) {
243 lagrange_field_name);
249 lagrange_field_name);
255 lagrange_field_name);
259 element_name, eigen_node_field_name);
265 "MESH_NODE_POSITIONS");
271 range_slave_master_prisms, MBPRISM, element_name);
295 const string element_name,
const string field_name,
296 const string mesh_node_field_name,
const string lagrange_field_name,
297 Range &range_slave_master_prisms,
bool eigen_pos_flag =
false,
298 const string eigen_node_field_name =
"EIGEN_SPATIAL_POSITIONS") {
303 if (range_slave_master_prisms.size() > 0) {
306 lagrange_field_name);
311 mesh_node_field_name);
314 lagrange_field_name);
318 mesh_node_field_name);
321 lagrange_field_name);
325 element_name, eigen_node_field_name);
331 mesh_node_field_name);
335 Range ents_to_add = range_slave_master_prisms;
336 Range current_ents_with_fe;
338 current_ents_with_fe);
339 Range ents_to_remove;
340 ents_to_remove = subtract(current_ents_with_fe, ents_to_add);
362 const string spatial_field_name,
363 const string lagrange_field_name,
364 const string mesh_pos_field_name,
370 if (slave_tris.size() > 0) {
374 lagrange_field_name);
381 lagrange_field_name);
388 lagrange_field_name);
394 mesh_pos_field_name);
405 :
public boost::enable_shared_from_this<CommonDataSimpleContact> {
425 boost::shared_ptr<MatrixDouble>
hMat;
426 boost::shared_ptr<MatrixDouble>
FMat;
427 boost::shared_ptr<MatrixDouble>
HMat;
451 gapPtr = boost::make_shared<VectorDouble>();
456 hMat = boost::make_shared<MatrixDouble>();
457 FMat = boost::make_shared<MatrixDouble>();
458 HMat = boost::make_shared<MatrixDouble>();
459 invHMat = boost::make_shared<MatrixDouble>();
460 detHVec = boost::make_shared<VectorDouble>();
488 boost::shared_ptr<double> cn_value,
489 bool newton_cotes =
false)
497 boost::shared_ptr<VolumeElementForcesAndSourcesCoreOnContactPrismSide>
516 EntityType col_type,
EntData &row_data,
527 const string field_name_1,
const string field_name_2,
528 boost::shared_ptr<CommonDataSimpleContact> common_data_contact,
529 const ContactOp::FaceType face_type,
const int rank_row,
531 boost::shared_ptr<VolumeElementForcesAndSourcesCoreOnContactPrismSide>
533 const string side_fe_name =
"")
566 EntityType col_type,
EntData &row_data,
577 const string field_name_1,
const string field_name_2,
578 boost::shared_ptr<CommonDataSimpleContact> common_data_contact,
579 const ContactOp::FaceType face_type,
const int rank_row,
645 const string field_name_1,
const string field_name_2,
646 boost::shared_ptr<CommonDataSimpleContact> common_data_contact,
647 const bool is_master)
648 :
MoFEM::VolumeElementForcesAndSourcesCoreOnContactPrismSide::
654 tangentOne = boost::make_shared<VectorDouble>();
655 tangentTwo = boost::make_shared<VectorDouble>();
669 boost::shared_ptr<CommonDataSimpleContact> common_data_contact)
712 boost::shared_ptr<CommonDataSimpleContact> common_data_contact)
760 boost::shared_ptr<CommonDataSimpleContact> common_data_contact)
778 boost::shared_ptr<CommonDataSimpleContact> common_data_contact)
796 boost::shared_ptr<CommonDataSimpleContact> common_data_contact)
814 boost::shared_ptr<CommonDataSimpleContact> common_data_contact)
832 boost::shared_ptr<CommonDataSimpleContact> common_data_contact)
850 boost::shared_ptr<CommonDataSimpleContact> common_data_contact)
868 boost::shared_ptr<CommonDataSimpleContact> common_data_contact)
901 const string lagrange_field_name,
902 boost::shared_ptr<CommonDataSimpleContact> common_data_contact)
923 boost::shared_ptr<CommonDataSimpleContact> common_data_contact,
946 const string lagrange_field_name,
947 boost::shared_ptr<CommonDataSimpleContact> common_data_contact)
966 boost::shared_ptr<CommonDataSimpleContact> common_data_contact)
1011 boost::shared_ptr<CommonDataSimpleContact> common_data_contact)
1056 boost::shared_ptr<CommonDataSimpleContact> common_data_contact)
1109 boost::shared_ptr<CommonDataSimpleContact> common_data_contact)
1163 const string lagrange_field_name,
1164 boost::shared_ptr<CommonDataSimpleContact> common_data_contact,
1165 boost::shared_ptr<double> cn)
1218 const string lagrange_field_name,
1219 boost::shared_ptr<CommonDataSimpleContact> common_data_contact,
1280 const string field_name,
const string lagrange_field_name,
1281 boost::shared_ptr<CommonDataSimpleContact> common_data_contact)
1315 EntityType col_type,
EntData &row_data,
1335 const string field_name,
const string lagrange_field_name,
1336 boost::shared_ptr<CommonDataSimpleContact> common_data_contact)
1369 EntityType col_type,
EntData &row_data,
1389 const string lagrange_field_name,
1390 boost::shared_ptr<CommonDataSimpleContact> common_data_contact,
1391 boost::shared_ptr<double> cn)
1430 EntityType col_type,
EntData &row_data,
1451 const string lagrange_field_name,
const string field_name,
1452 boost::shared_ptr<CommonDataSimpleContact> common_data_contact,
1453 boost::shared_ptr<double> cn)
1494 EntityType col_type,
EntData &row_data,
1515 const string lagrange_field_name,
const string field_name,
1516 boost::shared_ptr<CommonDataSimpleContact> common_data_contact,
1517 boost::shared_ptr<double> cn)
1558 EntityType col_type,
EntData &row_data,
1581 const string field_name,
const string lagrange_field_name,
1582 boost::shared_ptr<CommonDataSimpleContact> common_data_contact)
1625 EntityType col_type,
EntData &row_data,
1646 const string field_name,
const string lagrange_field_name,
1647 boost::shared_ptr<CommonDataSimpleContact> common_data_contact)
1689 EntityType col_type,
EntData &row_data,
1710 const string field_name,
const string field_name_2,
1711 const double cn_value,
1712 boost::shared_ptr<CommonDataSimpleContact> common_data_contact)
1757 EntityType col_type,
1780 const string field_name,
const string field_name_2,
1781 const double cn_value,
1782 boost::shared_ptr<CommonDataSimpleContact> common_data_contact)
1827 EntityType col_type,
1850 const string field_name,
const string field_name_2,
1851 const double cn_value,
1852 boost::shared_ptr<CommonDataSimpleContact> common_data_contact)
1897 EntityType col_type,
1921 const string field_name,
const string field_name_2,
1922 const double cn_value,
1923 boost::shared_ptr<CommonDataSimpleContact> common_data_contact)
1968 EntityType col_type,
1990 const string lagrange_field_name,
1991 boost::shared_ptr<CommonDataSimpleContact> common_data_contact,
2034 EntityType col_type,
EntData &row_data,
2054 const string field_name,
const string lagrange_field_name,
2055 boost::shared_ptr<CommonDataSimpleContact> common_data_contact,
2099 EntityType col_type,
EntData &row_data,
2119 const string field_name,
const string lagrange_field_name,
2120 boost::shared_ptr<CommonDataSimpleContact> common_data_contact,
2165 EntityType col_type,
EntData &row_data,
2195 boost::shared_ptr<CommonDataSimpleContact> common_data,
2214 boost::shared_ptr<CommonDataSimpleContact> common_data,
2215 ofstream &_my_split)
2219 mySplit << fixed << setprecision(8);
2220 mySplit <<
"[0] Lagrange multiplier [1] Gap" << endl;
2247 boost::shared_ptr<SimpleContactElement> fe_rhs_simple_contact,
2248 boost::shared_ptr<CommonDataSimpleContact> common_data_simple_contact,
2249 string field_name,
string lagrange_field_name,
bool is_alm =
false,
2250 bool is_eigen_pos_field =
false,
2251 string eigen_pos_field_name =
"EIGEN_SPATIAL_POSITIONS",
2252 bool use_reference_coordinates =
false);
2277 boost::shared_ptr<SimpleContactElement> fe_lhs_simple_contact,
2278 boost::shared_ptr<CommonDataSimpleContact> common_data_simple_contact,
2279 string field_name,
string lagrange_field_name,
bool is_alm =
false,
2280 bool is_eigen_pos_field =
false,
2281 string eigen_pos_field_name =
"EIGEN_SPATIAL_POSITIONS",
2282 bool use_reference_coordinates =
false);
2292 boost::shared_ptr<ConvectMasterContactElement> fe_lhs_simple_contact,
2293 boost::shared_ptr<CommonDataSimpleContact> common_data_simple_contact,
2294 string field_name,
string lagrange_field_name,
bool is_alm =
false,
2295 bool is_eigen_pos_field =
false,
2296 string eigen_pos_field_name =
"EIGEN_SPATIAL_POSITIONS",
2297 bool use_reference_coordinates =
false);
2300 boost::shared_ptr<SimpleContactElement> fe_lhs_simple_contact,
2301 boost::shared_ptr<CommonDataSimpleContact> common_data_simple_contact,
2302 string field_name,
string lagrange_field_name,
bool is_alm =
false,
2303 bool is_eigen_pos_field =
false,
2304 string eigen_pos_field_name =
"EIGEN_SPATIAL_POSITIONS",
2305 bool use_reference_coordinates =
false);
2308 boost::shared_ptr<SimpleContactElement> fe_lhs_simple_contact,
2309 boost::shared_ptr<CommonDataSimpleContact> common_data_simple_contact,
2310 string field_name,
string lagrange_field_name,
bool is_alm =
false,
2311 bool is_eigen_pos_field =
false,
2312 string eigen_pos_field_name =
"EIGEN_SPATIAL_POSITIONS",
2313 bool use_reference_coordinates =
false);
2316 boost::shared_ptr<ConvectSlaveContactElement> fe_lhs_simple_contact,
2317 boost::shared_ptr<CommonDataSimpleContact> common_data_simple_contact,
2318 string field_name,
string lagrange_field_name,
bool is_alm =
false,
2319 bool is_eigen_pos_field =
false,
2320 string eigen_pos_field_name =
"EIGEN_SPATIAL_POSITIONS",
2321 bool use_reference_coordinates =
false);
2347 boost::shared_ptr<SimpleContactElement> fe_post_proc_simple_contact,
2348 boost::shared_ptr<CommonDataSimpleContact> common_data_simple_contact,
2351 bool is_eigen_pos_field =
false,
2352 string eigen_pos_field_name =
"EIGEN_SPATIAL_POSITIONS",
2353 bool use_reference_coordinates =
false,
2366 const string row_field_name,
const string col_field_name,
2367 boost::shared_ptr<CommonDataSimpleContact> common_data_contact,
2368 const ContactOp::FaceType face_type,
2369 boost::shared_ptr<MatrixDouble> diff_convect)
2378 EntityType col_type,
EntData &row_data,
2394 boost::shared_ptr<CommonDataSimpleContact> common_data_contact)
2412 boost::shared_ptr<CommonDataSimpleContact> common_data_contact)
2430 const string lagrange_field_name,
const string field_name,
2431 boost::shared_ptr<CommonDataSimpleContact> common_data_contact,
2432 boost::shared_ptr<double> cn,
const ContactOp::FaceType face_type,
2433 boost::shared_ptr<MatrixDouble> diff_convect)
2442 EntityType col_type,
EntData &row_data,
2457 :
public VolumeElementForcesAndSourcesCoreOnContactPrismSide::
2467 boost::shared_ptr<CommonDataSimpleContact> common_data_contact,
2468 bool ho_geometry =
false)
2489 boost::shared_ptr<VolumeElementForcesAndSourcesCoreOnContactPrismSide>
2500 boost::shared_ptr<VolumeElementForcesAndSourcesCoreOnContactPrismSide>
2502 const string &side_fe_name,
const ContactOp::FaceType face_type)
2571 boost::shared_ptr<CommonDataSimpleContact> common_data_contact)
2638 boost::shared_ptr<CommonDataSimpleContact> common_data_contact)
2650 boost::shared_ptr<CommonDataSimpleContact> common_data_contact)
2694 boost::shared_ptr<CommonDataSimpleContact> common_data_contact)
2783 const string mesh_nodes_field_row,
const string mesh_nodes_field_col,
2784 boost::shared_ptr<CommonDataSimpleContact> common_data_contact,
2785 const int row_rank,
const int col_rank)
2787 common_data_contact,
ContactOp::FACEMASTERMASTER,
2788 row_rank, col_rank) {
2845 const string mesh_nodes_field_row,
const string mesh_nodes_field_col,
2846 boost::shared_ptr<CommonDataSimpleContact> common_data_contact,
2847 const int row_rank,
const int col_rank)
2849 common_data_contact,
ContactOp::FACESLAVESLAVE,
2850 row_rank, col_rank) {
2925 const string mesh_nodes_field_row,
const string lagrange_field_name,
2926 boost::shared_ptr<CommonDataSimpleContact> common_data_contact,
2927 const int row_rank,
const int col_rank)
2929 common_data_contact,
ContactOp::FACEMASTERSLAVE,
2930 row_rank, col_rank) {
3004 const string mesh_nodes_field_row,
const string lagrange_field_name,
3005 boost::shared_ptr<CommonDataSimpleContact> common_data_contact,
3006 const int row_rank,
const int col_rank)
3008 common_data_contact,
ContactOp::FACESLAVESLAVE,
3009 row_rank, col_rank) {
3067 const string field_name,
const string mesh_nodes_field,
3068 boost::shared_ptr<CommonDataSimpleContact> common_data_contact,
3069 const int row_rank,
const int col_rank)
3071 ContactOp::FACESLAVESLAVE, row_rank, col_rank) {
3149 const string field_name,
const string mesh_nodes_field,
3150 boost::shared_ptr<CommonDataSimpleContact> common_data_contact,
3151 const int row_rank,
const int col_rank)
3153 ContactOp::FACEMASTERSLAVE, row_rank, col_rank) {
3217 const string field_name,
const string mesh_nodes_field,
3218 boost::shared_ptr<CommonDataSimpleContact> common_data_contact,
3219 const int row_rank,
const int col_rank)
3221 ContactOp::FACEMASTERMASTER, row_rank, col_rank) {
3316 const string lagrange_field_name,
const string mesh_nodes_field,
3317 boost::shared_ptr<double> cn,
3318 boost::shared_ptr<CommonDataSimpleContact> common_data_contact,
3319 int row_rank,
const int col_rank)
3321 common_data_contact,
ContactOp::FACESLAVESLAVE,
3322 row_rank, col_rank),
3380 const string field_name_1,
const string field_name_2,
3381 boost::shared_ptr<CommonDataSimpleContact> common_data_contact,
3382 const bool is_master)
3384 common_data_contact, is_master) {
3442 const string field_name_1,
const string field_name_2,
3443 boost::shared_ptr<CommonDataSimpleContact> common_data_contact,
3444 const bool is_master)
3446 common_data_contact, is_master) {
3471 boost::shared_ptr<SimpleContactElement> fe_rhs_simple_contact_ale,
3472 boost::shared_ptr<CommonDataSimpleContact> common_data_simple_contact,
3473 const string field_name,
const string mesh_node_field_name,
3474 const string lagrange_field_name,
const string side_fe_name);
3495 boost::shared_ptr<SimpleContactElement> fe_lhs_simple_contact_ale,
3496 boost::shared_ptr<CommonDataSimpleContact> common_data_simple_contact,
3497 const string field_name,
const string mesh_node_field_name,
3498 const string lagrange_field_name,
const string side_fe_name);
3517 boost::shared_ptr<SimpleContactElement> fe_lhs_simple_contact_ale,
3518 boost::shared_ptr<CommonDataSimpleContact> common_data_simple_contact,
3519 const string field_name,
const string mesh_node_field_name,
3520 const string lagrange_field_name,
bool is_eigen_pos_field =
false,
3521 string eigen_pos_field_name =
"EIGEN_SPATIAL_POSITIONS");
3526 const string lagrange_field_name,
3527 boost::shared_ptr<CommonDataSimpleContact> common_data_contact,
3528 const double cn,
const bool alm_flag =
false)
3546 const string lagrange_field_name,
3547 boost::shared_ptr<CommonDataSimpleContact> common_data_contact,
3548 const double cn,
const bool alm_flag =
false,
3549 Range post_proc_surface =
Range(),
double post_proc_gap_tol = 0.)
3579 return ((cn *
g) <=
l);
3584 return ((
l + cn *
g) < 0. || abs(
l + cn *
g) <
ALM_TOL);
3598 return cn * (1 +
Sign(
l - cn *
g)) /
static_cast<double>(2);
3604 return (1 +
Sign(cn *
g -
l)) /
static_cast<double>(2);
#define MoFEMFunctionReturnHot(a)
Last executable line of each PETSc function used for error handling. Replaces return()
Data on single entity (This is passed as argument to DataOperator::doWork)
virtual MPI_Comm & get_comm() const =0
virtual MoFEMErrorCode modify_finite_element_add_field_row(const std::string &fe_name, const std::string name_row)=0
set field row which finite element use
DEPRECATED auto createSmartVectorMPI(MPI_Comm comm, PetscInt n, PetscInt N)
PetscErrorCode MoFEMErrorCode
MoFEM/PETSc error code.
UBlasMatrix< double > MatrixDouble
virtual int get_comm_rank() const =0
Deprecated interface functions.
MoFEMErrorCode doWork(int side, EntityType type, EntData &data)
DeprecatedCoreInterface Interface
virtual MoFEMErrorCode add_ents_to_finite_element_by_type(const EntityHandle entities, const EntityType type, const std::string &name, const bool recursive=true)=0
add entities to finite element
#define CHKERR
Inline error check.
virtual MoFEMErrorCode remove_ents_from_finite_element(const std::string name, const EntityHandle meshset, const EntityType type, int verb=DEFAULT_VERBOSITY)=0
remove entities from given refinement level to finite element database
virtual MoFEMErrorCode add_finite_element(const std::string &fe_name, enum MoFEMTypes bh=MF_EXCL, int verb=DEFAULT_VERBOSITY)=0
add finite element
implementation of Data Operators for Forces and Sources
virtual MoFEMErrorCode modify_finite_element_add_field_col(const std::string &fe_name, const std::string name_row)=0
set field col which finite element use
OpMakeTestTextFile(MoFEM::Interface &m_field, string field_name, boost::shared_ptr< CommonDataSimpleContact > common_data, ofstream &_my_split)
#define MOFEM_LOG_C(channel, severity, format,...)
boost::shared_ptr< CommonDataSimpleContact > commonDataSimpleContact
Class used to scale loads, f.e. in arc-length control.
EntitiesFieldData::EntData EntData
constexpr auto field_name
virtual MoFEMErrorCode get_finite_element_entities_by_handle(const std::string name, Range &ents) const =0
get entities in the finite element by handle
MoFEM::Interface & mField
ForcesAndSourcesCore::UserDataOperator UserDataOperator
UBlasVector< int > VectorInt
virtual MoFEMErrorCode modify_finite_element_add_field_data(const std::string &fe_name, const std::string name_filed)=0
set finite element field data
UBlasVector< double > VectorDouble
#define MoFEMFunctionReturn(a)
Last executable line of each PETSc function used for error handling. Replaces return()
#define MoFEMFunctionBegin
First executable line of each MoFEM function, used for error handling. Final line of MoFEM functions ...
FTensor::Index< 'l', 3 > l