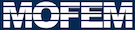 |
| v0.14.0
|
Go to the documentation of this file.
25 using namespace MoFEM;
41 static char help[] =
"Calculate crack release energy and crack propagation"
48 bool CrackPropagation::parallelReadAndBroadcast =
54 int main(
int argc,
char *argv[]) {
56 const char param_file[] =
"param_file.petsc";
61 PetscBool flg = PETSC_FALSE;
63 if (flg == PETSC_TRUE)
83 auto add_last_twenty = [&]() {
89 ->addToDatabaseBitRefLevelByType(MBTET, mask,
BitRefLevel().set());
93 auto add_first_four = [&]() {
96 for (
int ll = 0; ll != 5; ++ll)
99 ->addToDatabaseBitRefLevelByType(MBTET, mask,
BitRefLevel().set());
108 ->writeEntitiesAllBitLevelsByType(
BitRefLevel().set(), MBTET,
109 "all_start.vtk",
"VTK",
"");
121 CHKERR moab.create_meshset(MESHSET_SET, meshset);
122 CHKERR moab.add_entities(meshset, ents);
123 CHKERR moab.write_file(
"ents_not_in_database_to_delete.vtk",
"VTK",
"",
125 CHKERR moab.delete_entities(&meshset, 1);
128 CHKERR moab.get_entities_by_type(0, MBENTITYSET, meshsets,
false);
129 for (
auto m : meshsets)
130 CHKERR moab.remove_entities(
m, ents);
131 CHKERR moab.delete_entities(ents);
134 cp.crackAccelerationFactor,
"cutting_surface.vtk",
QUIET,
143 CHKERR cp.getInterface<
CPMeshCut>()->copySurface(
"cutting_surface.vtk");
145 CHKERR moab.get_entities_by_type(0, MBTET, vol,
false);
153 CHKERR moab.write_mesh(
"cut_mesh_out.h5m");
156 cp.mapBitLevel[
"mesh_cut"],
BitRefLevel().set(), MBTET,
157 "out_level0.vtk",
"VTK",
"");
159 cp.mapBitLevel[
"spatial_domain"],
BitRefLevel().set(), MBTET,
160 "out_level1_tets.vtk",
"VTK",
"");
162 cp.mapBitLevel[
"spatial_domain"],
BitRefLevel().set(), MBPRISM,
163 "out_level1_prisms.vtk",
"VTK",
"");
MoFEMErrorCode getInterface(IFACE *&iface) const
Get interface reference to pointer of interface.
static MoFEMErrorCode Finalize()
Checks for options to be called at the conclusion of the program.
Deprecated interface functions.
DeprecatedCoreInterface Interface
Solvers for crack propagation.
const std::string & getCutSurfMeshName() const
#define CHKERR
Inline error check.
implementation of Data Operators for Forces and Sources
Implementation of linear elastic material.
int main(int argc, char *argv[])
static MoFEMErrorCode Initialize(int *argc, char ***args, const char file[], const char help[])
Initializes the MoFEM database PETSc, MOAB and MPI.
#define CATCH_ERRORS
Catch errors.
#define BITREFLEVEL_SIZE
max number of refinements
PetscErrorCode PetscOptionsGetString(PetscOptions *, const char pre[], const char name[], char str[], size_t size, PetscBool *set)
Implementation of Volume-Length-Quality measure with barrier.
FTensor::Index< 'm', 3 > m
Main class for crack propagation.
std::bitset< BITREFLEVEL_SIZE > BitRefLevel
Bit structure attached to each entity identifying to what mesh entity is attached.
Implementation of Neo-Hookean elastic material.
#define MoFEMFunctionReturn(a)
Last executable line of each PETSc function used for error handling. Replaces return()
#define MoFEMFunctionBegin
First executable line of each MoFEM function, used for error handling. Final line of MoFEM functions ...
PetscErrorCode PetscOptionsGetBool(PetscOptions *, const char pre[], const char name[], PetscBool *bval, PetscBool *set)