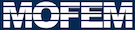 |
| v0.14.0
|
Go to the documentation of this file.
18 static char help[] =
"...\n\n";
33 double operator()(
const double x,
const double y,
const double z)
const {
34 return 1 + x * x + y * y + z * z * z;
43 const double z)
const {
65 double operator()(
const double x,
const double y,
const double z)
const {
70 int main(
int argc,
char *argv[]) {
83 PetscBool flg_test = PETSC_FALSE;
84 CHKERR PetscOptionsBegin(PETSC_COMM_WORLD,
"",
"Poisson's problem options",
90 CHKERR PetscOptionsBool(
"-test",
"if true is ctest",
"", flg_test,
91 &flg_test, PETSC_NULL);
92 ierr = PetscOptionsEnd();
112 boost::shared_ptr<ForcesAndSourcesCore> domain_lhs_fe;
113 boost::shared_ptr<ForcesAndSourcesCore> boundary_lhs_fe;
114 boost::shared_ptr<ForcesAndSourcesCore> domain_rhs_fe;
115 boost::shared_ptr<ForcesAndSourcesCore> boundary_rhs_fe;
116 boost::shared_ptr<ForcesAndSourcesCore> domain_error;
117 boost::shared_ptr<PoissonExample::PostProcFE>
119 boost::shared_ptr<ForcesAndSourcesCore>
null;
125 boundary_lhs_fe, domain_rhs_fe, boundary_rhs_fe);
130 global_error, domain_error);
207 domain_rhs_fe,
null,
null);
209 boundary_rhs_fe,
null,
null);
217 CHKERR DMCreateGlobalVector(dm,&
F);
224 CHKERR KSPCreate(PETSC_COMM_WORLD,&solver);
225 CHKERR KSPSetFromOptions(solver);
226 CHKERR KSPSetDM(solver,dm);
238 CHKERR KSPDestroy(&solver);
251 if (flg_test == PETSC_TRUE) {
262 post_proc_volume->writeFile(
"out_vol.h5m");
269 CHKERR VecDestroy(&global_error);
MoFEMErrorCode getInterface(IFACE *&iface) const
Get interface reference to pointer of interface.
PetscErrorCode DMMoFEMKSPSetComputeRHS(DM dm, const char fe_name[], MoFEM::FEMethod *method, MoFEM::BasicMethod *pre_only, MoFEM::BasicMethod *post_only)
set KSP right hand side evaluation function
@ L2
field with C-1 continuity
MoFEMErrorCode loadFile(const std::string options, const std::string mesh_file_name, LoadFileFunc loadFunc=defaultLoadFileFunc)
Load mesh file.
static MoFEMErrorCode Finalize()
Checks for options to be called at the conclusion of the program.
Create finite elements instances.
PetscErrorCode DMMoFEMKSPSetComputeOperators(DM dm, const char fe_name[], MoFEM::FEMethod *method, MoFEM::BasicMethod *pre_only, MoFEM::BasicMethod *post_only)
Set KSP operators and push mofem finite element methods.
Simple interface for fast problem set-up.
Deprecated interface functions.
DeprecatedCoreInterface Interface
MoFEMErrorCode getOptions()
get options
MoFEMErrorCode createGhostVec(Vec *ghost_vec) const
double operator()(const double x, const double y, const double z) const
double operator()(const double x, const double y, const double z) const
MoFEMErrorCode getDM(DM *dm)
Get DM.
#define CHKERR
Inline error check.
MoFEMErrorCode creatFEToPostProcessResults(boost::shared_ptr< PostProcFE > &post_proc_volume) const
Create finite element to post-process results.
MoFEMErrorCode testError(Vec ghost_vec)
Test error.
PetscErrorCode DMoFEMMeshToGlobalVector(DM dm, Vec g, InsertMode mode, ScatterMode scatter_mode)
set ghosted vector values on all existing mesh entities
MoFEMErrorCode addDomainField(const std::string &name, const FieldSpace space, const FieldApproximationBase base, const FieldCoefficientsNumber nb_of_coefficients, const TagType tag_type=MB_TAG_SPARSE, const enum MoFEMTypes bh=MF_ZERO, int verb=-1)
Add field on domain.
PetscErrorCode DMRegister_MoFEM(const char sname[])
Register MoFEM problem.
const std::string getDomainFEName() const
Get the Domain FE Name.
MoFEMErrorCode printError(Vec ghost_vec)
Print error.
int main(int argc, char *argv[])
MoFEMErrorCode addDataField(const std::string &name, const FieldSpace space, const FieldApproximationBase base, const FieldCoefficientsNumber nb_of_coefficients, const TagType tag_type=MB_TAG_SPARSE, const enum MoFEMTypes bh=MF_ZERO, int verb=-1)
Add field on domain.
MoFEMErrorCode setFieldOrder(const std::string field_name, const int order, const Range *ents=NULL)
Set field order.
static MoFEMErrorCode Initialize(int *argc, char ***args, const char file[], const char help[])
Initializes the MoFEM database PETSc, MOAB and MPI.
#define CATCH_ERRORS
Catch errors.
MoFEMErrorCode createFEToEvaluateError(boost::function< double(const double, const double, const double)> f_u, boost::function< FTensor::Tensor1< double, 3 >(const double, const double, const double)> g_u, Vec global_error, boost::shared_ptr< ForcesAndSourcesCore > &domain_error) const
Create finite element to calculate error.
static MoFEMErrorCodeGeneric< PetscErrorCode > ierr
@ AINSWORTH_LEGENDRE_BASE
Ainsworth Cole (Legendre) approx. base .
const std::string getBoundaryFEName() const
Get the Boundary FE Name.
const FTensor::Tensor2< T, Dim, Dim > Vec
MoFEMErrorCode addBoundaryField(const std::string &name, const FieldSpace space, const FieldApproximationBase base, const FieldCoefficientsNumber nb_of_coefficients, const TagType tag_type=MB_TAG_SPARSE, const enum MoFEMTypes bh=MF_ZERO, int verb=-1)
Add field on boundary.
const double D
diffusivity
MoFEMErrorCode createFEToAssembleMatrixAndVector(boost::function< double(const double, const double, const double)> f_u, boost::function< double(const double, const double, const double)> f_source, boost::shared_ptr< ForcesAndSourcesCore > &domain_lhs_fe, boost::shared_ptr< ForcesAndSourcesCore > &boundary_lhs_fe, boost::shared_ptr< ForcesAndSourcesCore > &domain_rhs_fe, boost::shared_ptr< ForcesAndSourcesCore > &boundary_rhs_fe, bool trans=true) const
Create finite element to calculate matrix and vectors.
MoFEMErrorCode setUp(const PetscBool is_partitioned=PETSC_TRUE)
Setup problem.
PetscErrorCode DMoFEMLoopFiniteElements(DM dm, const char fe_name[], MoFEM::FEMethod *method, CacheTupleWeakPtr cache_ptr=CacheTupleSharedPtr())
Executes FEMethod for finite elements in DM.
#define CHKERRG(n)
Check error code of MoFEM/MOAB/PETSc function.
MoFEMErrorCode assembleGhostVector(Vec ghost_vec) const
Assemble error vector.
FTensor::Tensor1< double, 3 > operator()(const double x, const double y, const double z) const