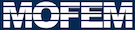 |
| v0.14.0
|
Atom test testing calculation of element residual vectors and tangent matrices.
More...
Go to the source code of this file.
|
int | main (int argc, char *argv[]) |
|
|
static char | help [] = "...\n\n" |
|
Atom test testing calculation of element residual vectors and tangent matrices.
Definition in file damper_jacobian_test.cpp.
◆ main()
int main |
( |
int |
argc, |
|
|
char * |
argv[] |
|
) |
| |
Definition at line 17 of file damper_jacobian_test.cpp.
27 PetscBool flg = PETSC_TRUE;
32 if (flg != PETSC_TRUE) {
33 SETERRQ(PETSC_COMM_SELF, 1,
"*** ERROR -my_file (MESH FILE NEEDED)");
57 bool check_if_spatial_field_exist =
75 if (flg != PETSC_TRUE) {
95 if (!check_if_spatial_field_exist) {
130 damper.blockMaterialDataMap[id];
131 damper.constitutiveEquationMap.insert(
136 CHKERR moab.get_entities_by_type(0, MBTET, material_data.
tEts);
137 material_data.
gBeta = 1;
138 material_data.
vBeta = 0.3;
144 for (
auto &&fe_ptr : {&damper.feRhs, &damper.feLhs}) {
149 fe_ptr->getOpPtrVector().push_back(
153 fe_ptr->getOpPtrVector().push_back(
156 fe_ptr->getOpPtrVector().push_back(
160 fe_ptr->getOpPtrVector().push_back(
166 std::vector<int> tags;
170 damper.constitutiveEquationMap.at(
id);
174 "SPATIAL_POSITION", tags, ce, damper.commonData,
true,
false));
175 damper.feRhs.getOpPtrVector().push_back(
180 "SPATIAL_POSITION", tags, ce, damper.commonData,
false,
true));
181 damper.feLhs.getOpPtrVector().push_back(
187 DMType dm_name =
"DMDAMPER";
190 CHKERR DMCreate(PETSC_COMM_WORLD, &dm);
191 CHKERR DMSetType(dm, dm_name);
193 CHKERR DMSetFromOptions(dm);
207 CHKERR VecGhostUpdateBegin(
F, INSERT_VALUES, SCATTER_FORWARD);
208 CHKERR VecGhostUpdateEnd(
F, INSERT_VALUES, SCATTER_FORWARD);
209 CHKERR VecZeroEntries(U_t);
210 CHKERR VecGhostUpdateBegin(U_t, INSERT_VALUES, SCATTER_FORWARD);
211 CHKERR VecGhostUpdateEnd(U_t, INSERT_VALUES, SCATTER_FORWARD);
213 damper.feRhs.ts_F =
F;
214 damper.feRhs.ts_u_t = U_t;
215 damper.feRhs.ts_ctx = TSMethod::CTX_TSSETIFUNCTION;
217 damper.feLhs.ts_B =
M;
218 damper.feLhs.ts_a = 1.0;
219 damper.feLhs.ts_u_t = U_t;
223 CHKERR MatAssemblyBegin(
M, MAT_FINAL_ASSEMBLY);
224 CHKERR MatAssemblyEnd(
M, MAT_FINAL_ASSEMBLY);
231 CHKERR PetscViewerASCIIOpen(PETSC_COMM_WORLD,
"damper_jacobian_test.txt",
233 CHKERR PetscViewerDestroy(&viewer);
◆ help
MoFEMErrorCode getInterface(IFACE *&iface) const
Get interface reference to pointer of interface.
Assemble internal force vector.
virtual MoFEMErrorCode loop_dofs(const Problem *problem_ptr, const std::string &field_name, RowColData rc, DofMethod &method, int lower_rank, int upper_rank, int verb=DEFAULT_VERBOSITY)=0
Make a loop over dofs.
string spatialPositionNameDot
double gBeta
Sheer modulus spring alpha.
virtual MoFEMErrorCode modify_finite_element_add_field_row(const std::string &fe_name, const std::string name_row)=0
set field row which finite element use
Implementation of Kelvin Voigt Damper.
Projection of edge entities with one mid-node on hierarchical basis.
static MoFEMErrorCode Finalize()
Checks for options to be called at the conclusion of the program.
virtual MoFEMErrorCode add_ents_to_field_by_type(const Range &ents, const EntityType type, const std::string &name, int verb=DEFAULT_VERBOSITY)=0
Add entities to field meshset.
PetscErrorCode DMMoFEMAddElement(DM dm, std::string fe_name)
add element to dm
boost::shared_ptr< MatrixDouble > gradDataAtGaussTmpPtr
Deprecated interface functions.
DeprecatedCoreInterface Interface
PetscErrorCode DMCreateGlobalVector_MoFEM(DM dm, Vec *g)
DMShellSetCreateGlobalVector.
virtual MoFEMErrorCode add_ents_to_finite_element_by_type(const EntityHandle entities, const EntityType type, const std::string &name, const bool recursive=true)=0
add entities to finite element
FTensor::Index< 'M', 3 > M
PetscErrorCode DMCreateMatrix_MoFEM(DM dm, Mat *M)
#define CHKERR
Inline error check.
virtual MoFEMErrorCode add_finite_element(const std::string &fe_name, enum MoFEMTypes bh=MF_EXCL, int verb=DEFAULT_VERBOSITY)=0
add finite element
virtual MoFEMErrorCode modify_finite_element_add_field_col(const std::string &fe_name, const std::string name_row)=0
set field col which finite element use
virtual MoFEMErrorCode build_finite_elements(int verb=DEFAULT_VERBOSITY)=0
Build finite elements.
PetscErrorCode DMRegister_MoFEM(const char sname[])
Register MoFEM problem.
virtual bool check_field(const std::string &name) const =0
check if field is in database
PetscErrorCode DMMoFEMCreateMoFEM(DM dm, MoFEM::Interface *m_field_ptr, const char problem_name[], const MoFEM::BitRefLevel bit_level, const MoFEM::BitRefLevel bit_mask=MoFEM::BitRefLevel().set())
Must be called by user to set MoFEM data structures.
virtual MoFEMErrorCode modify_finite_element_add_field_data(const std::string &fe_name, const std::string name_field)=0
set finite element field data
double vBeta
Poisson ration spring alpha.
Get field gradients at integration pts for scalar filed rank 0, i.e. vector field.
static MoFEMErrorCode Initialize(int *argc, char ***args, const char file[], const char help[])
Initializes the MoFEM database PETSc, MOAB and MPI.
#define CATCH_ERRORS
Catch errors.
Common data for nonlinear_elastic_elem model.
@ AINSWORTH_LEGENDRE_BASE
Ainsworth Cole (Legendre) approx. base .
const FTensor::Tensor2< T, Dim, Dim > Vec
PetscErrorCode PetscOptionsGetString(PetscOptions *, const char pre[], const char name[], char str[], size_t size, PetscBool *set)
virtual MoFEMErrorCode build_fields(int verb=DEFAULT_VERBOSITY)=0
std::bitset< BITREFLEVEL_SIZE > BitRefLevel
Bit structure attached to each entity identifying to what mesh entity is attached.
virtual MoFEMErrorCode build_adjacencies(const Range &ents, int verb=DEFAULT_VERBOSITY)=0
build adjacencies
string spatialPositionName
virtual MoFEMErrorCode set_field_order(const EntityHandle meshset, const EntityType type, const std::string &name, const ApproximationOrder order, int verb=DEFAULT_VERBOSITY)=0
Set order approximation of the entities in the field.
PetscErrorCode DMoFEMLoopFiniteElements(DM dm, const char fe_name[], MoFEM::FEMethod *method, CacheTupleWeakPtr cache_ptr=CacheTupleSharedPtr())
Executes FEMethod for finite elements in DM.
Get field gradients time derivative at integration pts for scalar filed rank 0, i....
PetscErrorCode PetscOptionsGetInt(PetscOptions *, const char pre[], const char name[], PetscInt *ivalue, PetscBool *set)
virtual MoFEMErrorCode add_field(const std::string name, const FieldSpace space, const FieldApproximationBase base, const FieldCoefficientsNumber nb_of_coefficients, const TagType tag_type=MB_TAG_SPARSE, const enum MoFEMTypes bh=MF_EXCL, int verb=DEFAULT_VERBOSITY)=0
Add field.
Dumper material parameters.