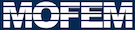 |
| v0.14.0
|
Make a loop over entities
static char help[] =
"...\n\n";
entsIt = allEnts.begin();
}
if (entPtr->getEnt() != *entsIt)
"Entity and entity iterator should be the same");
++entsIt;
}
if(entsIt != allEnts.end())
"All entities should be iterated");
}
private:
Range::const_iterator entsIt;
};
int main(
int argc,
char *argv[]) {
try {
DMType dm_name = "DMMOFEM";
{
all_ents = subtract(all_ents, all_ents.subset_by_type(MBENTITYSET));
auto testingEntitiesInDatabase = [&]() {
};
CHKERR testingEntitiesInDatabase();
auto testingEntitiesInDatabaseSubRange = [&]() {
Range edges = all_ents.subset_by_type(MBEDGE);
};
CHKERR testingEntitiesInDatabaseSubRange();
auto dm = simple_interface->
getDM();
auto testingEntitiesInDM = [&]() {
};
}
}
return 0;
}
#define MoFEMFunctionReturnHot(a)
Last executable line of each PETSc function used for error handling. Replaces return()
MoFEMErrorCode getInterface(IFACE *&iface) const
Get interface reference to pointer of interface.
int main(int argc, char *argv[])
Data structure to exchange data between mofem and User Loop Methods on entities.
PetscErrorCode MoFEMErrorCode
MoFEM/PETSc error code.
MoFEMErrorCode loadFile(const std::string options, const std::string mesh_file_name, LoadFileFunc loadFunc=defaultLoadFileFunc)
Load mesh file.
static MoFEMErrorCode Finalize()
Checks for options to be called at the conclusion of the program.
Simple interface for fast problem set-up.
Deprecated interface functions.
DeprecatedCoreInterface Interface
MoFEMErrorCode getOptions()
get options
MoFEMErrorCode getDM(DM *dm)
Get DM.
#define CHKERR
Inline error check.
virtual moab::Interface & get_moab()=0
implementation of Data Operators for Forces and Sources
PetscErrorCode DMRegister_MoFEM(const char sname[])
Register MoFEM problem.
virtual int get_comm_size() const =0
MoFEMErrorCode setFieldOrder(const std::string field_name, const int order, const Range *ents=NULL)
Set field order.
static MoFEMErrorCode Initialize(int *argc, char ***args, const char file[], const char help[])
Initializes the MoFEM database PETSc, MOAB and MPI.
#define CATCH_ERRORS
Catch errors.
@ AINSWORTH_LEGENDRE_BASE
Ainsworth Cole (Legendre) approx. base .
PetscErrorCode DMMoFEMGetProblemPtr(DM dm, const MoFEM::Problem **problem_ptr)
Get pointer to problem data structure.
@ MOFEM_ATOM_TEST_INVALID
#define MoFEMFunctionBeginHot
First executable line of each MoFEM function, used for error handling. Final line of MoFEM functions ...
keeps basic data about problem
MoFEMErrorCode setUp(const PetscBool is_partitioned=PETSC_TRUE)
Setup problem.
#define MoFEMFunctionReturn(a)
Last executable line of each PETSc function used for error handling. Replaces return()
MoFEMErrorCode addDomainField(const std::string name, const FieldSpace space, const FieldApproximationBase base, const FieldCoefficientsNumber nb_of_coefficients, const TagType tag_type=MB_TAG_SPARSE, const enum MoFEMTypes bh=MF_ZERO, int verb=-1)
Add field on domain.
#define MoFEMFunctionBegin
First executable line of each MoFEM function, used for error handling. Final line of MoFEM functions ...
virtual MoFEMErrorCode loop_entities(const std::string field_name, EntityMethod &method, Range const *const ents=nullptr, int verb=DEFAULT_VERBOSITY)=0
Loop over field entities.