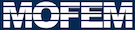 |
| v0.14.0
|
Go to the documentation of this file.
17 #ifdef WITH_MODULE_MORTAR_CONTACT
21 #ifdef WITH_MODULE_MFRONT_INTERFACE
22 #include <MFrontMoFEMInterface.hpp>
25 #ifdef WITH_MODULE_HDIV_CONTACT
27 #include <AnalyticalSurfaces.hpp>
28 #include <RigidBodies.hpp>
29 #include <BasicFeTools.hpp>
30 #include <ContactOperators.hpp>
31 using namespace OpContactTools;
32 #include <HdivContactInterface.hpp>
45 static char help[] =
"...\n\n";
50 int main(
int argc,
char *argv[]) {
52 const string default_options =
"-ksp_type fgmres \n"
54 "-pc_factor_mat_solver_type mumps \n"
59 "-snes_linesearch_type bt \n"
60 "-snes_linesearch_max_it 3 \n"
64 "-ts_alpha_radius 1 \n"
66 "-mat_mumps_icntl_20 0 \n"
67 "-mat_mumps_icntl_14 800 \n"
68 "-mat_mumps_icntl_24 1 \n"
69 "-mat_mumps_icntl_13 1 \n";
71 string param_file =
"param_file.petsc";
72 if (!
static_cast<bool>(ifstream(param_file))) {
73 std::ofstream file(param_file.c_str(), std::ios::ate);
75 file << default_options;
83 auto core_log = logging::core::get();
102 if (
md.isPartitioned)
106 simple->getProblemName() =
"MoFEM Manager module";
107 simple->getDomainFEName() =
"ELASTIC";
108 vector<string> volume_fe_elements{
simple->getDomainFEName()};
109 simple->getBoundaryFEName() =
"BOUNDARY_ELEMENT";
112 boost::ptr_vector<GenericElementInterface> m_modules;
116 m_field,
"U",
"MESH_NODE_POSITIONS",
simple->getProblemName(),
117 simple->getDomainFEName(),
true,
md.isQuasiStatic,
nullptr,
120 #ifdef WITH_MODULE_MORTAR_CONTACT
122 m_field,
"U",
"MESH_NODE_POSITIONS",
true,
md.isQuasiStatic));
127 m_field,
"U",
"MESH_NODE_POSITIONS",
true,
md.isQuasiStatic));
129 #ifdef WITH_MODULE_MFRONT_INTERFACE
130 m_modules.push_back(
new MFrontMoFEMInterface(
131 m_field,
"U",
"MESH_NODE_POSITIONS",
true,
md.isQuasiStatic));
132 volume_fe_elements.push_back(
"MFRONT_EL");
135 #ifdef WITH_MODULE_HDIV_CONTACT
136 m_modules.push_back(
new HdivContactInterface(
137 m_field,
"U",
"MESH_NODE_POSITIONS",
simple->getProblemName(),
138 volume_fe_elements, {simple->getBoundaryFEName()}, PETSC_TRUE,
139 md.isQuasiStatic,
md.isPartitioned));
144 for (
auto &&mod : m_modules) {
145 CHKERR mod.getCommandLineParameters();
146 CHKERR mod.addElementFields();
147 CHKERR mod.createElements();
153 for (
auto &&mod : m_modules)
163 md.monitorPtr->preProcessHook = []() {
return 0; };
164 md.monitorPtr->operatorHook = []() {
return 0; };
165 md.monitorPtr->postProcessHook = [&]() {
167 auto ts_time =
md.monitorPtr->ts_t;
168 auto ts_step =
md.monitorPtr->ts_step;
169 auto &ts_u =
md.monitorPtr->ts_u;
171 for (
auto &&mod : m_modules) {
172 CHKERR mod.updateElementVariables();
173 if (ts_step %
md.saveEveryNthStep == 0)
174 CHKERR mod.postProcessElement(ts_step);
177 if (
md.doPrintMaxMin == PETSC_TRUE) {
178 CHKERR md.printMaxMin(
md.vScatter[0],
"Ux", ts_u, ts_time);
179 CHKERR md.printMaxMin(
md.vScatter[1],
"Uy", ts_u, ts_time);
180 CHKERR md.printMaxMin(
md.vScatter[2],
"Uz", ts_u, ts_time);
186 auto t_type =
md.getTSType();
187 for (
auto &&mod : m_modules) {
188 CHKERR mod.setMonitorPtr(
md.monitorPtr);
189 CHKERR mod.setOperators();
190 CHKERR mod.setupSolverFunctionTS(t_type);
191 CHKERR mod.setupSolverJacobianTS(t_type);
#define MoFEMFunctionReturnHot(a)
Last executable line of each PETSc function used for error handling. Replaces return()
MoFEMErrorCode getInterface(IFACE *&iface) const
Get interface reference to pointer of interface.
ElementsAndOps< SPACE_DIM >::BoundaryEle BoundaryEle
virtual MoFEMErrorCode loop_dofs(const Problem *problem_ptr, const std::string &field_name, RowColData rc, DofMethod &method, int lower_rank, int upper_rank, int verb=DEFAULT_VERBOSITY)=0
Make a loop over dofs.
Set of functions declaring elements and setting operators for basic boundary conditions interface.
Header file for BasicBoundaryConditionsInterface element implementation.
Projection of edge entities with one mid-node on hierarchical basis.
static MoFEMErrorCode Finalize()
Checks for options to be called at the conclusion of the program.
static boost::shared_ptr< SinkType > createSink(boost::shared_ptr< std::ostream > stream_ptr, std::string comm_filter)
Create a sink object.
Simple interface for fast problem set-up.
Deprecated interface functions.
DeprecatedCoreInterface Interface
#define CHKERR
Inline error check.
constexpr size_t SPACE_DIM
implementation of Data Operators for Forces and Sources
virtual MoFEMErrorCode build_finite_elements(int verb=DEFAULT_VERBOSITY)=0
Build finite elements.
void simple(double P1[], double P2[], double P3[], double c[], const int N)
static boost::shared_ptr< std::ostream > getStrmWorld()
Get the strm world object.
#define MOFEM_LOG_TAG(channel, tag)
Tag channel.
Volume finite element base.
int main(int argc, char *argv[])
static MoFEMErrorCode Initialize(int *argc, char ***args, const char file[], const char help[])
Initializes the MoFEM database PETSc, MOAB and MPI.
#define CATCH_ERRORS
Catch errors.
ForcesAndSourcesCore::UserDataOperator UserDataOperator
virtual MoFEMErrorCode build_fields(int verb=DEFAULT_VERBOSITY)=0
virtual MoFEMErrorCode build_adjacencies(const Range &ents, int verb=DEFAULT_VERBOSITY)=0
build adjacencies
#define MoFEMFunctionBeginHot
First executable line of each MoFEM function, used for error handling. Final line of MoFEM functions ...
ElementsAndOps< SPACE_DIM >::DomainEle DomainEle
static LoggerType & setLog(const std::string channel)
Set ans resset chanel logger.
Set of functions declaring elements and setting operators for generic element interface.