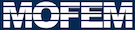 |
| v0.14.0
|
◆ MinimalSurfaceEqn()
◆ assembleSystem()
Definition at line 307 of file minimal_surface_equation.cpp.
309 auto add_domain_base_ops = [&](
auto &pipeline) {
310 auto det_ptr = boost::make_shared<VectorDouble>();
311 auto jac_ptr = boost::make_shared<MatrixDouble>();
312 auto inv_jac_ptr = boost::make_shared<MatrixDouble>();
319 auto add_domain_lhs_ops = [&](
auto &pipeline) {
321 auto grad_u_at_gauss_pts = boost::make_shared<MatrixDouble>();
329 auto add_domain_rhs_ops = [&](
auto &pipeline) {
331 auto grad_u_at_gauss_pts = boost::make_shared<MatrixDouble>();
338 auto add_boundary_base_ops = [&](
auto &pipeline) {};
340 auto add_lhs_base_ops = [&](
auto &pipeline) {
343 "U",
"U", [](
const double,
const double,
const double) {
return 1; }));
346 auto add_rhs_base_ops = [&](
auto &pipeline) {
348 auto u_at_gauss_pts = boost::make_shared<VectorDouble>();
352 [](
const double,
const double,
const double) {
return 1; }));
358 add_domain_base_ops(pipeline_mng->getOpDomainLhsPipeline());
359 add_domain_base_ops(pipeline_mng->getOpDomainRhsPipeline());
360 add_domain_lhs_ops(pipeline_mng->getOpDomainLhsPipeline());
361 add_domain_rhs_ops(pipeline_mng->getOpDomainRhsPipeline());
363 add_boundary_base_ops(pipeline_mng->getOpBoundaryLhsPipeline());
364 add_boundary_base_ops(pipeline_mng->getOpBoundaryRhsPipeline());
365 add_lhs_base_ops(pipeline_mng->getOpBoundaryLhsPipeline());
366 add_rhs_base_ops(pipeline_mng->getOpBoundaryRhsPipeline());
◆ boundaryCondition()
Definition at line 266 of file minimal_surface_equation.cpp.
271 auto get_ents_on_mesh_skin = [&]() {
276 CHKERR skin.find_skin(0, body_ents,
false, skin_ents);
279 ParallelComm *pcomm =
281 pcomm->filter_pstatus(skin_ents, PSTATUS_SHARED | PSTATUS_MULTISHARED,
282 PSTATUS_NOT, -1, &boundary_ents);
285 mField.
get_moab().get_connectivity(boundary_ents, skin_verts,
true);
286 boundary_ents.merge(skin_verts);
288 return boundary_ents;
291 auto mark_boundary_dofs = [&](
Range &&skin_edges) {
293 auto marker_ptr = boost::make_shared<std::vector<unsigned char>>();
295 ProblemsManager::OR, skin_edges, *marker_ptr);
◆ boundaryFunction()
◆ outputResults()
Definition at line 424 of file minimal_surface_equation.cpp.
427 auto post_proc = boost::make_shared<PostProcEle>(
mField);
429 auto u_ptr = boost::make_shared<VectorDouble>();
430 post_proc->getOpPtrVector().push_back(
435 post_proc->getOpPtrVector().push_back(
437 new OpPPMap(post_proc->getPostProcMesh(),
438 post_proc->getMapGaussPts(),
449 auto dm =
simple->getDM();
452 CHKERR post_proc->writeFile(
"out_result.h5m");
◆ readMesh()
◆ runProgram()
◆ setIntegrationRules()
◆ setupProblem()
◆ solveSystem()
Definition at line 371 of file minimal_surface_equation.cpp.
376 auto set_fieldsplit_preconditioner = [&](
auto snes) {
379 CHKERR SNESGetKSP(snes, &ksp);
381 CHKERR KSPGetPC(ksp, &pc);
382 PetscBool is_pcfs = PETSC_FALSE;
383 PetscObjectTypeCompare((PetscObject)pc, PCFIELDSPLIT, &is_pcfs);
385 if (is_pcfs == PETSC_TRUE) {
387 auto name_prb =
simple->getProblemName();
392 CHKERR ISGetSize(is_all_bc, &is_all_bc_size);
394 <<
"Field split block size " << is_all_bc_size;
395 CHKERR PCFieldSplitSetIS(pc, PETSC_NULL,
402 auto dm =
simple->getDM();
410 CHKERR SNESSetFromOptions(solver);
411 CHKERR set_fieldsplit_preconditioner(solver);
415 CHKERR SNESSolve(solver, global_rhs, global_solution);
◆ boundaryEnts
Range MinimalSurfaceEqn::boundaryEnts |
|
private |
◆ boundaryMarker
boost::shared_ptr<std::vector<unsigned char> > MinimalSurfaceEqn::boundaryMarker |
|
private |
◆ mField
The documentation for this struct was generated from the following file:
#define MoFEMFunctionReturnHot(a)
Last executable line of each PETSc function used for error handling. Replaces return()
MoFEMErrorCode getInterface(IFACE *&iface) const
Get interface reference to pointer of interface.
MoFEM::Interface & mField
#define MYPCOMM_INDEX
default communicator number PCOMM
SmartPetscObj< SNES > createSNES(SmartPetscObj< DM > dm=nullptr)
Create SNES (nonlinear) solver.
Integrate the domain tangent matrix (LHS)
Problem manager is used to build and partition problems.
FormsIntegrators< BoundaryEleOp >::Assembly< PETSC >::LinearForm< GAUSS >::OpBaseTimesScalar< 1 > OpBoundaryTimeScalarField
MoFEMErrorCode setupProblem()
Integrate the domain residual vector (RHS)
MoFEMErrorCode boundaryCondition()
PipelineManager interface.
MoFEMErrorCode readMesh()
Simple interface for fast problem set-up.
Get field gradients at integration pts for scalar filed rank 0, i.e. vector field.
PetscErrorCode DMCreateGlobalVector_MoFEM(DM dm, Vec *g)
DMShellSetCreateGlobalVector.
MoFEMErrorCode outputResults()
#define CHKERR
Inline error check.
virtual moab::Interface & get_moab()=0
static double boundaryFunction(const double x, const double y, const double z)
Section manager is used to create indexes and sections.
Simple interface for fast problem set-up.
MoFEMErrorCode solveSystem()
void simple(double P1[], double P2[], double P3[], double c[], const int N)
OpPostProcMapInMoab< SPACE_DIM, SPACE_DIM > OpPPMap
PetscErrorCode DMoFEMMeshToGlobalVector(DM dm, Vec g, InsertMode mode, ScatterMode scatter_mode)
set ghosted vector values on all existing mesh entities
Get value at integration points for scalar field.
Modify integration weights on face to take in account higher-order geometry.
boost::shared_ptr< std::vector< unsigned char > > boundaryMarker
MoFEMErrorCode markDofs(const std::string problem_name, RowColData rc, const enum MarkOP op, const Range ents, std::vector< unsigned char > &marker) const
Create vector with marked indices.
MoFEMErrorCode assembleSystem()
@ AINSWORTH_BERNSTEIN_BEZIER_BASE
MoFEMErrorCode setIntegrationRules()
#define MOFEM_LOG(channel, severity)
Log.
SmartPetscObj< Vec > vectorDuplicate(Vec vec)
Create duplicate vector of smart vector.
FormsIntegrators< BoundaryEleOp >::Assembly< PETSC >::BiLinearForm< GAUSS >::OpMass< 1, 1 > OpBoundaryMass
Set indices on entities on finite element.
#define MoFEMFunctionBeginHot
First executable line of each MoFEM function, used for error handling. Final line of MoFEM functions ...
PetscErrorCode DMoFEMLoopFiniteElements(DM dm, const char fe_name[], MoFEM::FEMethod *method, CacheTupleWeakPtr cache_ptr=CacheTupleSharedPtr())
Executes FEMethod for finite elements in DM.
PetscErrorCode PetscOptionsGetInt(PetscOptions *, const char pre[], const char name[], PetscInt *ivalue, PetscBool *set)
#define MoFEMFunctionReturn(a)
Last executable line of each PETSc function used for error handling. Replaces return()
#define MoFEMFunctionBegin
First executable line of each MoFEM function, used for error handling. Final line of MoFEM functions ...
FormsIntegrators< BoundaryEleOp >::Assembly< PETSC >::LinearForm< GAUSS >::OpSource< 1, 1 > OpBoundarySource
Post post-proc data at points from hash maps.