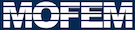 |
| v0.14.0
|
Testing problem for partitioned mesh with NOFIELD field
static char help[] =
"...\n\n";
constexpr
bool debug =
false;
int main(
int argc,
char *argv[]) {
try {
PetscBool flg = PETSC_TRUE;
255, &flg);
if (flg != PETSC_TRUE)
SETERRQ(PETSC_COMM_SELF, 1, "*** ERROR -my_file (MESH FILE NEEDED)");
DMType dm_name = "DMMOFEM";
DM dm;
CHKERR DMCreate(PETSC_COMM_WORLD, &dm);
CHKERR DMSetType(dm, dm_name);
ParallelComm *pcomm = ParallelComm::get_pcomm(&moab,
MYPCOMM_INDEX);
auto moab_comm_wrap =
boost::make_shared<WrapMPIComm>(PETSC_COMM_WORLD, false);
if (pcomm == NULL)
pcomm = new ParallelComm(&moab, moab_comm_wrap->get_comm());
const std::string options = "PARALLEL=READ_PART;"
"PARALLEL_RESOLVE_SHARED_ENTS;"
"PARTITION=PARALLEL_PARTITION;";
std::array<double, 6> coords = {0, 0, 0, 0, 0, 0};
CHKERR comm_interface_ptr->makeFieldEntitiesMultishared(
"LAMBDA", 0,
CHKERR bit_ref_ptr->setFieldEntitiesBitRefLevel(
"LAMBDA",
auto print_field_ents = [&](
const std::string
field_name) {
for (auto it = lo; it != hi; ++it) {
std::ostringstream ss;
ss <<
"Rank " << m_field.
get_comm_rank() <<
" -> " << **it << endl;
PetscSynchronizedPrintf(m_field.
get_comm(),
"%s", ss.str().c_str());
PetscSynchronizedFlush(m_field.
get_comm(), PETSC_STDOUT);
}
};
print_field_ents("LAMBDA");
}
CHKERR comm_interface_ptr->exchangeFieldData(
"LAMBDA");
CHKERR comm_interface_ptr->exchangeFieldData(
"FIELD");
auto check_exchanged_values = [&](
const std::string
field_name) {
for (auto it = lo; it != hi; ++it) {
for (
auto v : field_data)
"Wrong value on field %4.3f",
v);
}
}
};
CHKERR check_exchanged_values(
"LAMBDA");
dm, PETSC_FALSE);
->checkMPIAIJWithArraysMatrixFillIn<PetscGlobalIdx_mi_tag>(
"TEST_PROBLEM", -1, -1, 1);
CHKERR moab.write_file(
"test_out.h5m",
"MOAB",
"PARALLEL=WRITE_PART");
}
return 0;
}
MoFEMErrorCode getInterface(IFACE *&iface) const
Get interface reference to pointer of interface.
#define MYPCOMM_INDEX
default communicator number PCOMM
int main(int argc, char *argv[])
virtual MPI_Comm & get_comm() const =0
virtual MoFEMErrorCode modify_finite_element_add_field_row(const std::string &fe_name, const std::string name_row)=0
set field row which finite element use
PetscErrorCode DMMoFEMSetSquareProblem(DM dm, PetscBool square_problem)
set squared problem
virtual MoFEMErrorCode create_vertices_and_add_to_field(const std::string name, const double coords[], int size, int verb=DEFAULT_VERBOSITY)=0
Create a vertices and add to field object.
virtual FieldBitNumber get_field_bit_number(const std::string name) const =0
get field bit number
virtual int get_comm_rank() const =0
static MoFEMErrorCode Finalize()
Checks for options to be called at the conclusion of the program.
virtual MoFEMErrorCode add_ents_to_field_by_type(const Range &ents, const EntityType type, const std::string &name, int verb=DEFAULT_VERBOSITY)=0
Add entities to field meshset.
PetscErrorCode DMMoFEMAddElement(DM dm, std::string fe_name)
add element to dm
Deprecated interface functions.
DeprecatedCoreInterface Interface
#define CHKERR
Inline error check.
virtual MoFEMErrorCode add_finite_element(const std::string &fe_name, enum MoFEMTypes bh=MF_EXCL, int verb=DEFAULT_VERBOSITY)=0
add finite element
virtual MoFEMErrorCode add_ents_to_finite_element_by_type(const EntityHandle entities, const EntityType type, const std::string name, const bool recursive=true)=0
add entities to finite element
implementation of Data Operators for Forces and Sources
virtual MoFEMErrorCode modify_finite_element_add_field_col(const std::string &fe_name, const std::string name_row)=0
set field col which finite element use
virtual MoFEMErrorCode build_finite_elements(int verb=DEFAULT_VERBOSITY)=0
Build finite elements.
static UId getLoBitNumberUId(const FieldBitNumber bit_number)
PetscErrorCode DMRegister_MoFEM(const char sname[])
Register MoFEM problem.
virtual const FieldEntity_multiIndex * get_field_ents() const =0
Get the field ents object.
PetscErrorCode DMMoFEMCreateMoFEM(DM dm, MoFEM::Interface *m_field_ptr, const char problem_name[], const MoFEM::BitRefLevel bit_level, const MoFEM::BitRefLevel bit_mask=MoFEM::BitRefLevel().set())
Must be called by user to set MoFEM data structures.
virtual MoFEMErrorCode modify_finite_element_add_field_data(const std::string &fe_name, const std::string name_field)=0
set finite element field data
constexpr auto field_name
Matrix manager is used to build and partition problems.
const double v
phase velocity of light in medium (cm/ns)
static MoFEMErrorCode Initialize(int *argc, char ***args, const char file[], const char help[])
Initializes the MoFEM database PETSc, MOAB and MPI.
#define CATCH_ERRORS
Catch errors.
@ AINSWORTH_LEGENDRE_BASE
Ainsworth Cole (Legendre) approx. base .
PetscErrorCode PetscOptionsGetString(PetscOptions *, const char pre[], const char name[], char str[], size_t size, PetscBool *set)
virtual MoFEMErrorCode build_fields(int verb=DEFAULT_VERBOSITY)=0
static UId getHiBitNumberUId(const FieldBitNumber bit_number)
UBlasVector< double > VectorDouble
FTensor::Index< 'm', 3 > m
std::bitset< BITREFLEVEL_SIZE > BitRefLevel
Bit structure attached to each entity identifying to what mesh entity is attached.
@ MOFEM_ATOM_TEST_INVALID
virtual MoFEMErrorCode build_adjacencies(const Range &ents, int verb=DEFAULT_VERBOSITY)=0
build adjacencies
virtual MoFEMErrorCode set_field_order(const EntityHandle meshset, const EntityType type, const std::string &name, const ApproximationOrder order, int verb=DEFAULT_VERBOSITY)=0
Set order approximation of the entities in the field.
#define MoFEMFunctionReturn(a)
Last executable line of each PETSc function used for error handling. Replaces return()
virtual MoFEMErrorCode add_field(const std::string name, const FieldSpace space, const FieldApproximationBase base, const FieldCoefficientsNumber nb_of_coefficients, const TagType tag_type=MB_TAG_SPARSE, const enum MoFEMTypes bh=MF_EXCL, int verb=DEFAULT_VERBOSITY)=0
Add field.
#define MoFEMFunctionBegin
First executable line of each MoFEM function, used for error handling. Final line of MoFEM functions ...
@ NOFIELD
scalar or vector of scalars describe (no true field)