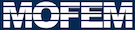 |
| v0.14.0
|
Go to the documentation of this file.
30 using namespace MoFEM;
31 using namespace boost::multi_index;
32 using namespace boost::multiprecision;
33 using namespace boost::numeric;
37 #include <boost/python.hpp>
38 #include <boost/python/def.hpp>
39 #include <boost/python/numpy.hpp>
40 namespace bp = boost::python;
41 namespace np = boost::python::numpy;
48 static char help[] =
"...\n\n";
50 int main(
int argc,
char *argv[]) {
53 const char param_file[] =
"param_file.petsc";
57 auto core_log = logging::core::get();
65 MOFEM_LOG(
"EP", Sev::inform) <<
"Python initialised";
67 MOFEM_LOG(
"EP", Sev::inform) <<
"Python NOT initialised";
78 PetscBool flg = PETSC_TRUE;
84 char restart_file[255];
85 PetscBool restart_flg = PETSC_TRUE;
92 DMType dm_name =
"DMMOFEM";
94 DMType dm_name_mg =
"DMMOFEM_MG";
101 ParallelComm *pcomm = ParallelComm::get_pcomm(&moab,
MYPCOMM_INDEX);
103 pcomm =
new ParallelComm(&moab, PETSC_COMM_WORLD);
106 if (pcomm->proc_config().proc_size() == 1)
109 option =
"PARALLEL=READ_PART;"
110 "PARALLEL_RESOLVE_SHARED_ENTS;"
111 "PARTITION=PARALLEL_PARTITION";
146 const char *list_materials[LastMaterial] = {
147 "stvenant_kirchhoff",
"mooney_rivlin",
"hencky",
"neo_hookean"};
148 PetscInt choice_material = MooneyRivlin;
150 LastMaterial, &choice_material, PETSC_NULL);
152 switch (choice_material) {
153 case StVenantKirchhoff:
154 MOFEM_LOG(
"EP", Sev::inform) <<
"StVenantKirchhoff material model";
159 MOFEM_LOG(
"EP", Sev::inform) <<
"MooneyRivlin material model";
160 MOFEM_LOG(
"EP", Sev::inform) <<
"MU(1, 0)/2 = " <<
MU(1, 0) / 2;
166 MOFEM_LOG(
"EP", Sev::inform) <<
"Hencky material model";
170 MOFEM_LOG(
"EP", Sev::inform) <<
"Neohookean material model";
184 CHKERR VecSetOption(f_elastic, VEC_IGNORE_NEGATIVE_INDICES, PETSC_TRUE);
188 CHKERR PetscViewerBinaryOpen(PETSC_COMM_WORLD, restart_file,
189 FILE_MODE_READ, &viewer);
190 CHKERR VecLoad(x_elastic, viewer);
191 CHKERR PetscViewerDestroy(&viewer);
196 auto ts_elastic =
createTS(PETSC_COMM_WORLD);
197 CHKERR TSSetType(ts_elastic, TSBEULER);
200 CHKERR TSGetAdapt(ts_elastic, &adapt);
204 CHKERR TSSetTime(ts_elastic, time);
213 if (Py_FinalizeEx() < 0) {
MoFEMErrorCode getInterface(IFACE *&iface) const
Get interface reference to pointer of interface.
#define MYPCOMM_INDEX
default communicator number PCOMM
MoFEMErrorCode addDMs(const BitRefLevel bit=BitRefLevel().set(0), const EntityHandle meshset=0)
MoFEMErrorCode projectGeometry(const EntityHandle meshset=0)
auto createTS(MPI_Comm comm)
virtual MPI_Comm & get_comm() const =0
MoFEMErrorCode addMaterial_Hencky(double E, double nu)
MoFEMErrorCode addBoundaryFiniteElement(const EntityHandle meshset=0)
MoFEMErrorCode setElasticElementToTs(DM dm)
MoFEMErrorCode setElasticElementOps(const int tag)
PetscErrorCode DMoFEMMeshToLocalVector(DM dm, Vec l, InsertMode mode, ScatterMode scatter_mode)
set local (or ghosted) vector values on mesh for partition only
MoFEMErrorCode getSpatialTractionFreeBc(const EntityHandle meshset=0)
static MoFEMErrorCode Finalize()
Checks for options to be called at the conclusion of the program.
static boost::shared_ptr< SinkType > createSink(boost::shared_ptr< std::ostream > stream_ptr, std::string comm_filter)
Create a sink object.
Deprecated interface functions.
DeprecatedCoreInterface Interface
PetscErrorCode DMCreateGlobalVector_MoFEM(DM dm, Vec *g)
DMShellSetCreateGlobalVector.
#define CHKERR
Inline error check.
implementation of Data Operators for Forces and Sources
MoFEMErrorCode addVolumeFiniteElement(const EntityHandle meshset=0)
SmartPetscObj< DM > dmElastic
Elastic problem.
MoFEMErrorCode DMRegister_MGViaApproxOrders(const char sname[])
Register DM for Multi-Grid via approximation orders.
PetscErrorCode TSAdaptCreate_EP(TSAdapt adapt)
MoFEMErrorCode addMaterial_HMHHStVenantKirchhoff(const int tape, const double lambda, const double mu, const double sigma_y)
static boost::shared_ptr< std::ostream > getStrmSync()
Get the strm sync object.
static boost::shared_ptr< std::ostream > getStrmWorld()
Get the strm world object.
PetscErrorCode DMRegister_MoFEM(const char sname[])
Register MoFEM problem.
MoFEMErrorCode addMaterial_HMHNeohookean(const int tape, const double c10, const double K)
MoFEMErrorCode solveElastic(TS ts, Vec x)
Eshelbian plasticity interface.
#define MOFEM_LOG_TAG(channel, tag)
Tag channel.
static MoFEMErrorCode Initialize(int *argc, char ***args, const char file[], const char help[])
Initializes the MoFEM database PETSc, MOAB and MPI.
#define MOFEM_LOG(channel, severity)
Log.
SmartPetscObj< Vec > vectorDuplicate(Vec vec)
Create duplicate vector of smart vector.
#define CATCH_ERRORS
Catch errors.
@ MOFEM_DATA_INCONSISTENCY
PetscErrorCode PetscOptionsGetString(PetscOptions *, const char pre[], const char name[], char str[], size_t size, PetscBool *set)
PetscErrorCode PetscOptionsGetEList(PetscOptions *, const char pre[], const char name[], const char *const *list, PetscInt next, PetscInt *value, PetscBool *set)
MoFEMErrorCode addFields(const EntityHandle meshset=0)
int main(int argc, char *argv[])
std::bitset< BITREFLEVEL_SIZE > BitRefLevel
Bit structure attached to each entity identifying to what mesh entity is attached.
PetscErrorCode PetscOptionsGetScalar(PetscOptions *, const char pre[], const char name[], PetscScalar *dval, PetscBool *set)
static LoggerType & setLog(const std::string channel)
Set ans resset chanel logger.
MoFEMErrorCode getSpatialTractionBc()
MoFEMErrorCode addMaterial_HMHMooneyRivlin(const int tape, const double alpha, const double beta, const double lambda, const double sigma_y)
MoFEMErrorCode getSpatialRotationBc()
MoFEMErrorCode getSpatialDispBc()
[Getting norms]