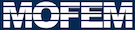 |
| v0.14.0
|
Plane stress elastic dynamic problem
namespace bio = boost::iostreams;
using bio::stream;
using bio::tee_device;
static char help[] =
"...\n\n";
int main(
int argc,
char *argv[]) {
try {
int rank;
MPI_Comm_rank(PETSC_COMM_WORLD, &rank);
PetscBool flg = PETSC_TRUE;
if (flg != PETSC_TRUE) {
SETERRQ(PETSC_COMM_SELF, 1, "*** ERROR -my_file (MESH FILE NEEDED)");
}
const char *option;
option = "";
bit_level0.set(0);
CHKERR moab.create_meshset(MESHSET_SET, meshset_level0);
0, 3, bit_level0);
3);
&flg);
if (flg != PETSC_TRUE) {
}
boost::shared_ptr<
double_kirchhoff_material_ptr(
double>());
boost::shared_ptr<
adouble_kirchhoff_material_ptr(
adouble_kirchhoff_material_ptr);
bit_level0);
"ELASTIC");
double scale_positions = 2;
{
double coords[3];
dof_ptr)) {
if (dof_ptr->get()->getEntType() != MBVERTEX)
continue;
int dof_rank = dof_ptr->get()->getDofCoeffIdx();
double &fval = dof_ptr->get()->getFieldData();
if (node != ent) {
CHKERR moab.get_coords(&ent, 1, coords);
node = ent;
}
fval = scale_positions * coords[dof_rank];
}
}
"ELASTIC_MECHANICS",
COL, &
F);
Mat Aij;
->createMPIAIJWithArrays<PetscGlobalIdx_mi_tag>("ELASTIC_MECHANICS",
&Aij);
elastic.getLoopFeRhs().snes_f =
F;
elastic.getLoopFeLhs().snes_B = Aij;
elastic.getLoopFeRhs());
CHKERR VecGhostUpdateBegin(
F, ADD_VALUES, SCATTER_REVERSE);
CHKERR VecGhostUpdateEnd(
F, ADD_VALUES, SCATTER_REVERSE);
elastic.getLoopFeLhs());
CHKERR MatAssemblyBegin(Aij, MAT_FINAL_ASSEMBLY);
CHKERR MatAssemblyEnd(Aij, MAT_FINAL_ASSEMBLY);
double sum = 0;
CHKERR PetscPrintf(PETSC_COMM_WORLD,
"sum = %4.3e\n", sum);
double fnorm;
CHKERR PetscPrintf(PETSC_COMM_WORLD,
"fnorm = %9.8e\n", fnorm);
double mnorm;
CHKERR MatNorm(Aij, NORM_1, &mnorm);
CHKERR PetscPrintf(PETSC_COMM_WORLD,
"mnorm = %9.8e\n", mnorm);
if (fabs(sum) > 1e-8) {
}
if (fabs(fnorm - 5.12196914e+00) > 1e-6) {
}
if (fabs(mnorm - 5.48280139e+01) > 1e-6) {
}
}
return 0;
}
MoFEMErrorCode getInterface(IFACE *&iface) const
Get interface reference to pointer of interface.
virtual MoFEMErrorCode loop_finite_elements(const std::string problem_name, const std::string &fe_name, FEMethod &method, boost::shared_ptr< NumeredEntFiniteElement_multiIndex > fe_ptr=nullptr, MoFEMTypes bh=MF_EXIST, CacheTupleWeakPtr cache_ptr=CacheTupleSharedPtr(), int verb=DEFAULT_VERBOSITY)=0
Make a loop over finite elements.
MoFEMErrorCode buildProblem(const std::string name, const bool square_matrix, int verb=VERBOSE)
build problem data structures
Problem manager is used to build and partition problems.
structure grouping operators and data used for calculation of nonlinear elastic element
static MoFEMErrorCode Finalize()
Checks for options to be called at the conclusion of the program.
virtual MoFEMErrorCode add_ents_to_field_by_type(const Range &ents, const EntityType type, const std::string &name, int verb=DEFAULT_VERBOSITY)=0
Add entities to field meshset.
Deprecated interface functions.
DeprecatedCoreInterface Interface
#define CHKERR
Inline error check.
implementation of Data Operators for Forces and Sources
virtual MoFEMErrorCode build_finite_elements(int verb=DEFAULT_VERBOSITY)=0
Build finite elements.
int main(int argc, char *argv[])
MoFEMErrorCode addElement(const std::string element_name, const std::string spatial_position_field_name, const std::string material_position_field_name="MESH_NODE_POSITIONS", const bool ale=false)
MoFEMErrorCode partitionFiniteElements(const std::string name, bool part_from_moab=false, int low_proc=-1, int hi_proc=-1, int verb=VERBOSE)
partition finite elements
virtual MoFEMErrorCode modify_problem_ref_level_add_bit(const std::string &name_problem, const BitRefLevel &bit)=0
add ref level to problem
Vector manager is used to create vectors \mofem_vectors.
MoFEMErrorCode setOperators(const std::string spatial_position_field_name, const std::string material_position_field_name="MESH_NODE_POSITIONS", const bool ale=false, const bool field_disp=false)
Set operators to calculate left hand tangent matrix and right hand residual.
Matrix manager is used to build and partition problems.
static MoFEMErrorCode Initialize(int *argc, char ***args, const char file[], const char help[])
Initializes the MoFEM database PETSc, MOAB and MPI.
#define CATCH_ERRORS
Catch errors.
#define _IT_GET_DOFS_FIELD_BY_NAME_FOR_LOOP_(MFIELD, NAME, IT)
@ AINSWORTH_LEGENDRE_BASE
Ainsworth Cole (Legendre) approx. base .
const FTensor::Tensor2< T, Dim, Dim > Vec
PetscErrorCode PetscOptionsGetString(PetscOptions *, const char pre[], const char name[], char str[], size_t size, PetscBool *set)
static char help[]
[Check]
virtual MoFEMErrorCode build_fields(int verb=DEFAULT_VERBOSITY)=0
virtual MoFEMErrorCode modify_problem_add_finite_element(const std::string name_problem, const std::string &fe_name)=0
add finite element to problem, this add entities assigned to finite element to a particular problem
std::bitset< BITREFLEVEL_SIZE > BitRefLevel
Bit structure attached to each entity identifying to what mesh entity is attached.
@ MOFEM_ATOM_TEST_INVALID
virtual MoFEMErrorCode build_adjacencies(const Range &ents, int verb=DEFAULT_VERBOSITY)=0
build adjacencies
virtual MoFEMErrorCode set_field_order(const EntityHandle meshset, const EntityType type, const std::string &name, const ApproximationOrder order, int verb=DEFAULT_VERBOSITY)=0
Set order approximation of the entities in the field.
MoFEMErrorCode partitionGhostDofs(const std::string name, int verb=VERBOSE)
determine ghost nodes
virtual MoFEMErrorCode add_problem(const std::string &name, enum MoFEMTypes bh=MF_EXCL, int verb=DEFAULT_VERBOSITY)=0
Add problem.
PetscErrorCode PetscOptionsGetInt(PetscOptions *, const char pre[], const char name[], PetscInt *ivalue, PetscBool *set)
virtual MoFEMErrorCode add_field(const std::string name, const FieldSpace space, const FieldApproximationBase base, const FieldCoefficientsNumber nb_of_coefficients, const TagType tag_type=MB_TAG_SPARSE, const enum MoFEMTypes bh=MF_EXCL, int verb=DEFAULT_VERBOSITY)=0
Add field.
MoFEMErrorCode setBlocks(boost::shared_ptr< FunctionsToCalculatePiolaKirchhoffI< double >> materialDoublePtr, boost::shared_ptr< FunctionsToCalculatePiolaKirchhoffI< adouble >> materialAdoublePtr)
MoFEMErrorCode partitionProblem(const std::string name, int verb=VERBOSE)
partition problem dofs (collective)