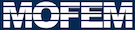 |
| v0.14.0
|
Go to the documentation of this file.
11 using namespace MoFEM;
13 static char help[] =
"...\n\n";
15 int main(
int argc,
char *argv[]) {
24 PetscBool flg = PETSC_TRUE;
26 #if PETSC_VERSION_GE(3, 6, 4)
33 if (flg != PETSC_TRUE)
34 SETERRQ(PETSC_COMM_SELF, 1,
"*** ERROR -my_file (MESH FILE NEEDED)");
48 CHKERR moab.get_number_entities_by_dimension(0, 0, nb_vertices,
true);
52 moab.get_entities_by_type(root_set, MBTET, tets,
false);
56 CHKERR moab.tag_get_handle(
"VERTEX_WEIGHT", 1, MB_TYPE_INTEGER,
57 th_vertex_weight, MB_TAG_CREAT | MB_TAG_DENSE,
62 tets, 3, 2, m_field.
get_comm_size(), &th_vertex_weight, NULL, NULL,
66 CHKERR moab.write_file(
"partitioned_mesh.h5m");
71 PetscBarrier(PETSC_NULL);
83 DMType dm_name =
"DMMOFEM";
87 const char *option =
"DEBUG_IO;"
89 "PARALLEL_RESOLVE_SHARED_ENTS;"
90 "PARTITION=PARALLEL_PARTITION;";
94 "partitioned_mesh.h5m");
105 if(problem_ptr->
nbDofsRow != nb_vertices)
107 "Number of vertices and DOFs is inconstent");
111 <<
"All is good in this test";
MoFEMErrorCode getInterface(IFACE *&iface) const
Get interface reference to pointer of interface.
#define MOFEM_LOG_CHANNEL(channel)
Set and reset channel.
virtual int get_comm_rank() const =0
MoFEMErrorCode partitionMesh(const Range &ents, const int dim, const int adj_dim, const int n_parts, Tag *th_vertex_weights=nullptr, Tag *th_edge_weights=nullptr, Tag *th_part_weights=nullptr, int verb=VERBOSE, const bool debug=false)
Set partition tag to each finite element in the problem.
static MoFEMErrorCode Finalize()
Checks for options to be called at the conclusion of the program.
Simple interface for fast problem set-up.
Deprecated interface functions.
DeprecatedCoreInterface Interface
#define CHKERR
Inline error check.
implementation of Data Operators for Forces and Sources
PetscErrorCode DMRegister_MoFEM(const char sname[])
Register MoFEM problem.
virtual int get_comm_size() const =0
int main(int argc, char *argv[])
static MoFEMErrorCode Initialize(int *argc, char ***args, const char file[], const char help[])
Initializes the MoFEM database PETSc, MOAB and MPI.
#define MOFEM_TAG_AND_LOG(channel, severity, tag)
Tag and log in channel.
#define CATCH_ERRORS
Catch errors.
@ AINSWORTH_LEGENDRE_BASE
Ainsworth Cole (Legendre) approx. base .
DofIdx nbDofsRow
Global number of DOFs in row.
PetscErrorCode PetscOptionsGetString(PetscOptions *, const char pre[], const char name[], char str[], size_t size, PetscBool *set)
PetscErrorCode DMMoFEMGetProblemPtr(DM dm, const MoFEM::Problem **problem_ptr)
Get pointer to problem data structure.
@ MOFEM_ATOM_TEST_INVALID
keeps basic data about problem