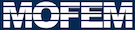 |
| v0.14.0
|
Go to the documentation of this file.
10 using namespace MoFEM;
12 static char help[] =
"...\n\n";
14 int main(
int argc,
char *argv[]) {
21 ParallelComm *pcomm = ParallelComm::get_pcomm(&moab,
MYPCOMM_INDEX);
23 pcomm =
new ParallelComm(&moab, PETSC_COMM_WORLD);
29 char mesh_out_file[255] =
"out.h5m";
32 CHKERR PetscOptionsBegin(m_field.
get_comm(),
"",
"Read MED tool",
"none");
33 CHKERR PetscOptionsInt(
"-med_time_step",
"time step",
"", time_step,
34 &time_step, PETSC_NULL);
35 CHKERR PetscOptionsString(
"-output_file",
"output mesh file name",
"",
36 "out.h5m", mesh_out_file, 255, PETSC_NULL);
37 ierr = PetscOptionsEnd();
45 for (std::map<std::string, MedInterface::FieldData>::iterator fit =
47 fit != med_interface_ptr->
fieldNames.end(); fit++) {
49 fit->first,
false, time_step);
60 <<
"Print all meshsets (old and added from meshsets "
61 "configurational file)";
62 for (
auto cit = meshsets_interface_ptr->
getBegin();
63 cit != meshsets_interface_ptr->
getEnd(); cit++)
66 CHKERR moab.write_file(mesh_out_file);
MoFEMErrorCode getInterface(IFACE *&iface) const
Get interface reference to pointer of interface.
#define MYPCOMM_INDEX
default communicator number PCOMM
#define MOFEM_LOG_CHANNEL(channel)
Set and reset channel.
Interface for load MED files.
virtual MPI_Comm & get_comm() const =0
std::string medFileName
MED file name.
MoFEMErrorCode readMed(const string &file, int verb=1)
read MED file
std::map< std::string, FieldData > fieldNames
static MoFEMErrorCode Finalize()
Checks for options to be called at the conclusion of the program.
Deprecated interface functions.
DeprecatedCoreInterface Interface
#define CHKERR
Inline error check.
CubitMeshSet_multiIndex::iterator getEnd() const
get begin iterator of cubit mehset of given type (instead you can use IT_CUBITMESHSETS_TYPE_FOR_LOOP(...
implementation of Data Operators for Forces and Sources
CubitMeshSet_multiIndex::iterator getBegin() const
get begin iterator of cubit mehset of given type (instead you can use IT_CUBITMESHSETS_TYPE_FOR_LOOP(...
MoFEMErrorCode setMeshsetFromFile(const string file_name, const bool clean_file_options=true)
add blocksets reading config file
MoFEMErrorCode medGetFieldNames(const string &file, int verb=1)
Get field names in MED file.
MoFEMErrorCode readFields(const std::string &file_name, const std::string &field_name, const bool load_series=false, const int only_step=-1, int verb=1)
int main(int argc, char *argv[])
#define MOFEM_LOG_TAG(channel, tag)
Tag channel.
static MoFEMErrorCode Initialize(int *argc, char ***args, const char file[], const char help[])
Initializes the MoFEM database PETSc, MOAB and MPI.
#define MOFEM_LOG(channel, severity)
Log.
#define CATCH_ERRORS
Catch errors.
static MoFEMErrorCodeGeneric< PetscErrorCode > ierr
Interface for managing meshsets containing materials and boundary conditions.